- 在自己项目各层在vs里按图中设置(原理:编译后运行控制台程序,copy工作是让控制台程序完成的。注意:start 后跟上exe文件路径,然后是空格)
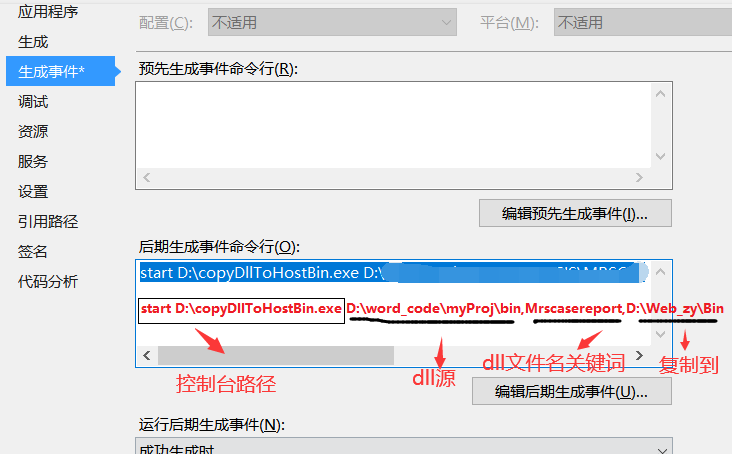
- 控制台程序源码
public class Program
{
public static void Main(string[] args)
{
//string para1 = @"D:\word_code\Server\myProj\\bin,casereport,D:\Web_zy\Bin";// 依次是源,dll文件关键词,目标目录
string para1 =args[0];
string[] ar = para1.Split(',', '|', '、');//源项目bin路径、移动dll文件的关键词、目标服务器路径
Console.WriteLine("源:" + ar[0]);
Console.WriteLine("目标:" + ar[2]);
var moveFile = new List<string>();
if (!string.IsNullOrWhiteSpace(ar[0]) && !string.IsNullOrWhiteSpace(ar[1]))
{
var list=GetFiles(ar[0],"", true);
moveFile = (from p in list
where Path.GetFileName(p).ToLower().Contains(ar[1].ToLower())
select p).ToList();
}
if (!string.IsNullOrWhiteSpace(ar[2]))
{
foreach (var item in moveFile)
{
CopyFile(item, $@"{ar[2]}\{Path.GetFileName(item)}");
}
}
Console.ForegroundColor = ConsoleColor.Green;
Console.Write("【成功】 按任意键退出...");
Console.ReadKey(true);
}
#region 查找目录下包含子目录的全部文件
/// <summary>
/// 获得目录下所有文件或指定文件类型文件地址
/// </summary>
public static List<string> fileList = new List<string>();
public static string[] GetFiles(string fullPath, string extName, bool isFullName = false)
{
try
{
fileList.Clear();
DirectoryInfo dirs = new DirectoryInfo(fullPath); //获得程序所在路径的目录对象
DirectoryInfo[] dir = dirs.GetDirectories();//获得目录下文件夹对象
FileInfo[] file = dirs.GetFiles();//获得目录下文件对象
int dircount = dir.Count();//获得文件夹对象数量
int filecount = file.Count();//获得文件对象数量
//循环文件夹
for (int i = 0; i < dircount; i++)
{
string pathNode = fullPath + "\\" + dir[i].Name;
GetMultiFile(pathNode, isFullName);
}
//循环文件
for (int j = 0; j < filecount; j++)
{
if (isFullName)
{
fileList.Add(file[j].FullName);
}
else
{
fileList.Add(file[j].Name);
}
}
return fileList.ToArray();
}
catch (Exception ex)
{
Console.WriteLine (ex.Message + "\r\n出错的位置为:Form1.PaintTreeView()");
}
return null;
}
private static bool GetMultiFile(string path, bool isFullName = false)
{
if (Directory.Exists(path) == false)
{ return false; }
DirectoryInfo dirs = new DirectoryInfo(path); //获得程序所在路径的目录对象
DirectoryInfo[] dir = dirs.GetDirectories();//获得目录下文件夹对象
FileInfo[] file = dirs.GetFiles();//获得目录下文件对象
int dircount = dir.Count();//获得文件夹对象数量
int filecount = file.Count();//获得文件对象数量
int sumcount = dircount + filecount;
if (sumcount == 0)
{ return false; }
//循环文件夹
for (int j = 0; j < dircount; j++)
{
string pathNodeB = path + "\\" + dir[j].Name;
GetMultiFile(pathNodeB, isFullName);
}
//循环文件
for (int j = 0; j < filecount; j++)
{
if (isFullName)
{
fileList.Add(file[j].FullName);
}
else
{
fileList.Add(file[j].Name);
}
}
return true;
}
/// <summary>
/// 大文件多次复制文件 true:复制成功 false:复制失败
/// </summary>
/// <param name="soucrePath">原始文件路径</param>
/// <param name="targetPath">复制目标文件路径</param>
/// <returns></returns>
public static bool CopyFile(string soucrePath, string targetPath)
{
try
{
//读取复制文件流
using (FileStream fsRead = new FileStream(soucrePath, FileMode.Open, FileAccess.Read))
{
//写入文件复制流
using (FileStream fsWrite = new FileStream(targetPath, FileMode.OpenOrCreate, FileAccess.Write))
{
byte[] buffer = new byte[1024 * 1024 * 2]; //每次读取2M
while (true)//文件大,每次读取2M
{
int n = fsRead.Read(buffer, 0, buffer.Count());//每次读取的数据 n:是每次读取到的实际数据大小
//如果n=0说明读取的数据为空,已经读取到最后了,跳出循环
if (n == 0)
{
break;
}
//写入每次读取的实际数据大小
fsWrite.Write(buffer, 0, n);
}
}
}
return true;
}
catch (Exception ex)
{
return false;
}
}
#endregion
}