小组成员
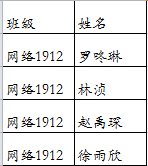
小组分工:
任务 |
姓名 |
前期调查和编码规范 |
徐雨欣 |
功能设计 |
林浈,罗咚琳 |
面向对象设计与UML制作 |
林浈,罗咚琳 |
PPT制作、演示 |
赵禹琛 |
前期调查:
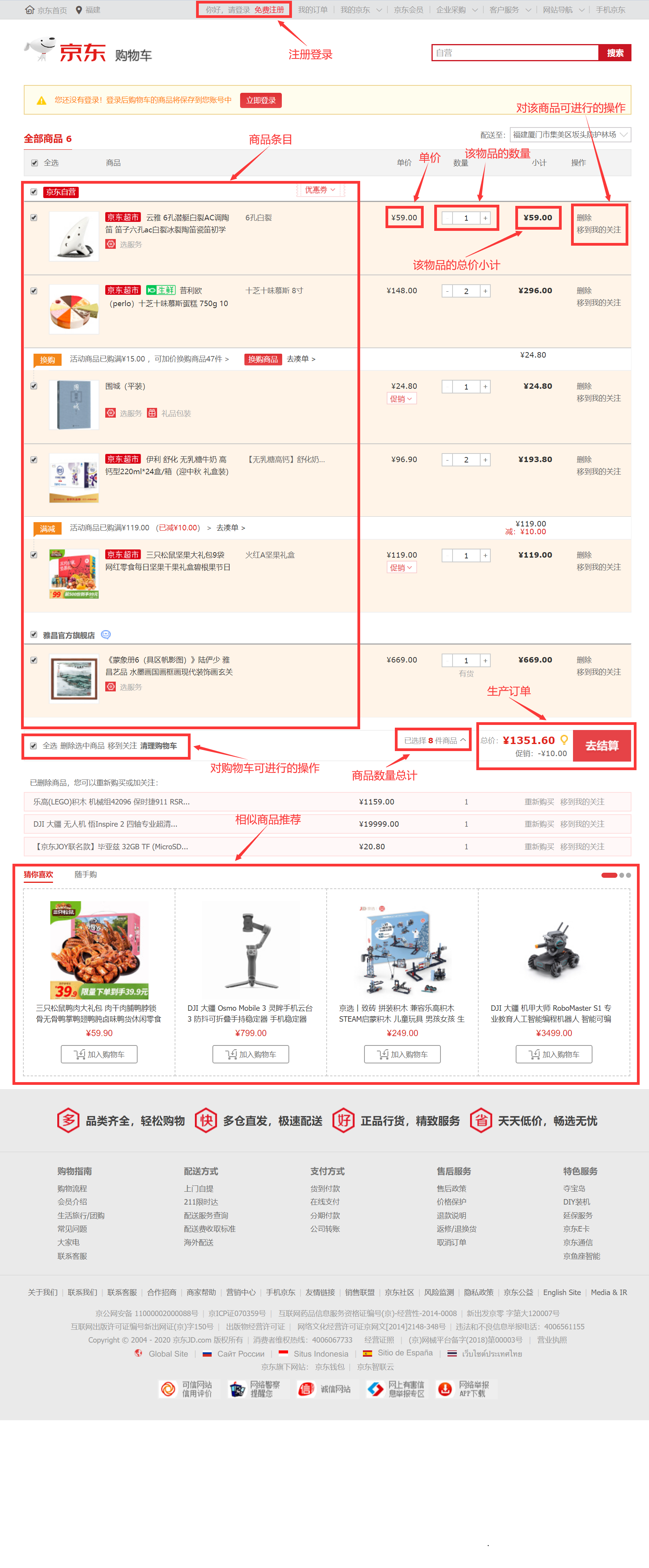
系统功能结构图:
流程图:
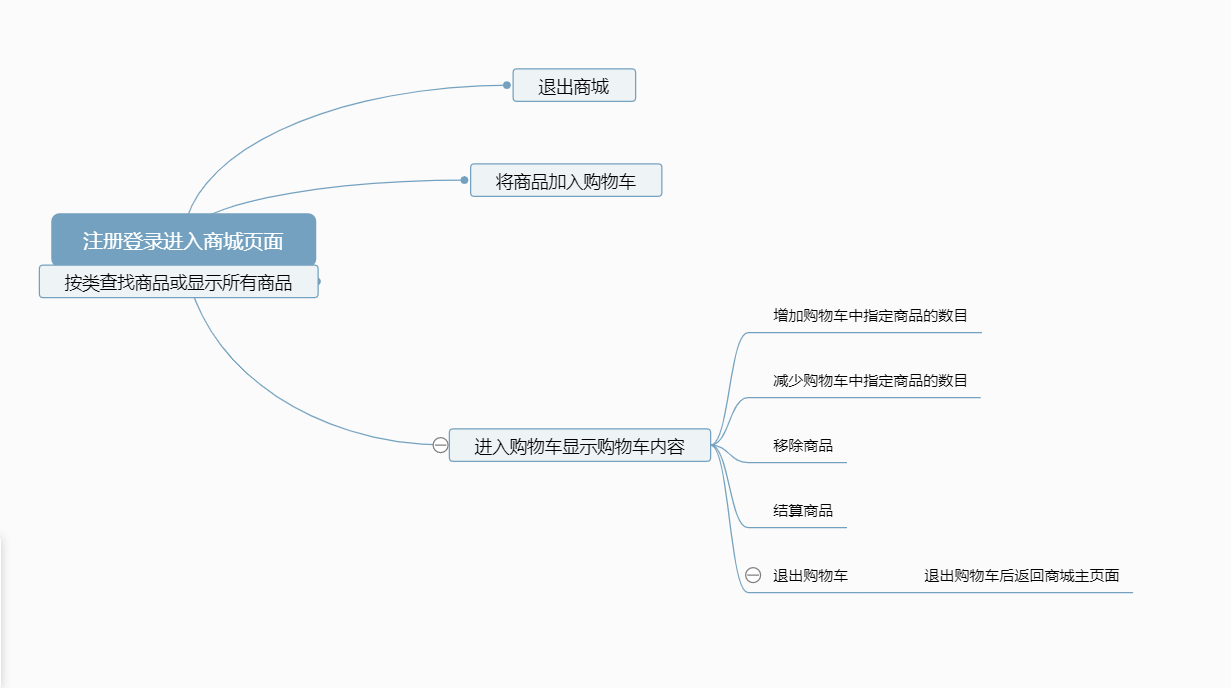
UML类图:
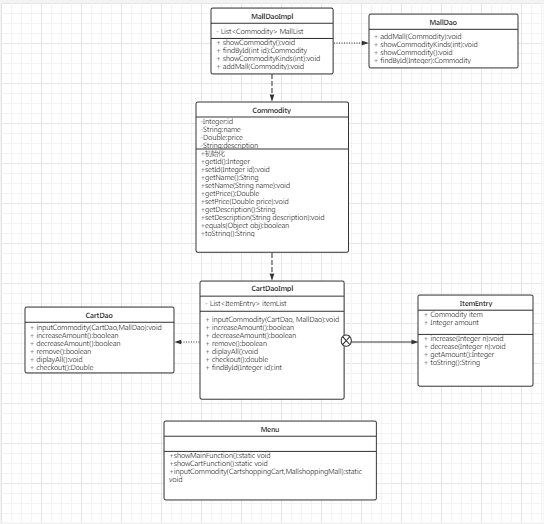
运行结果:
1.按类别查找商品:
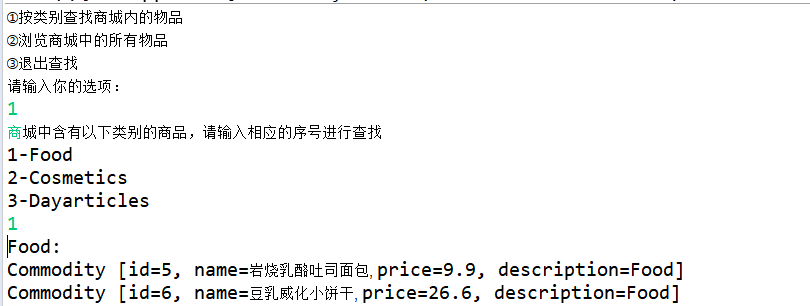
2.显示商城中所有商品:
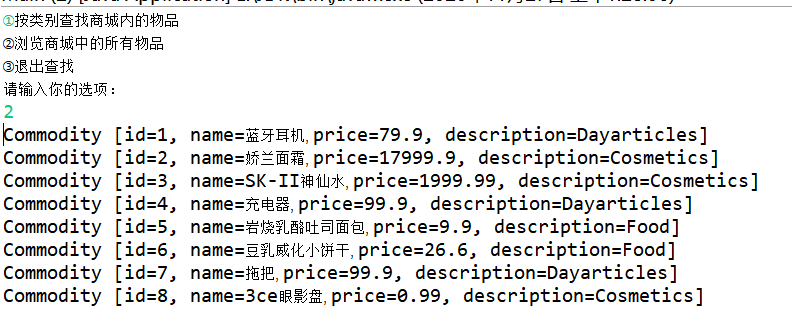
3.将选定的商品加入购物车:
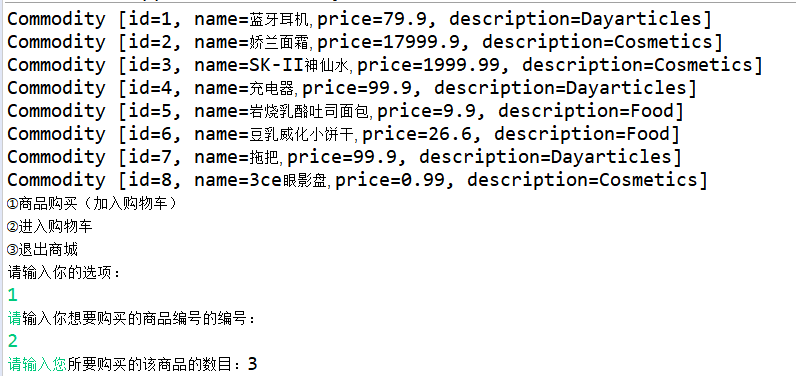
4.增加购物车中指定商品的数目:
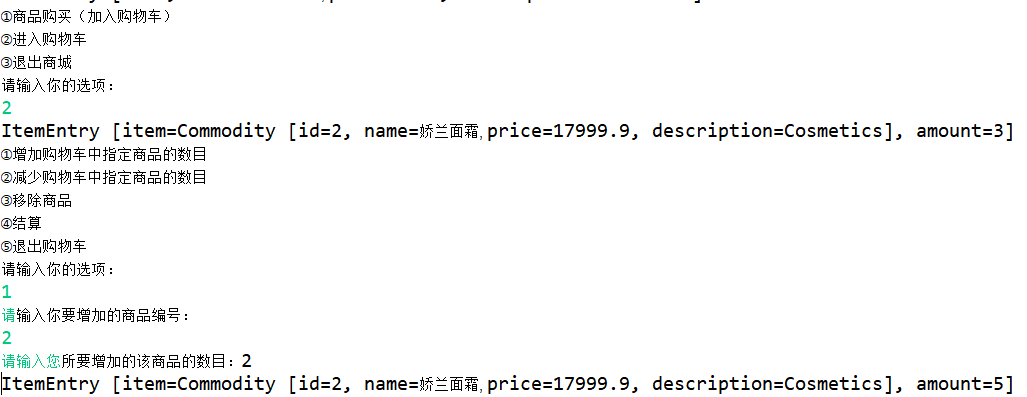
5.减少购物车中指定商品的数目:
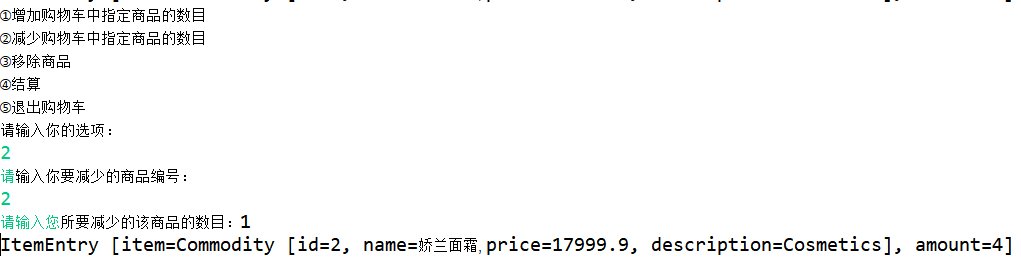
6.移除商品
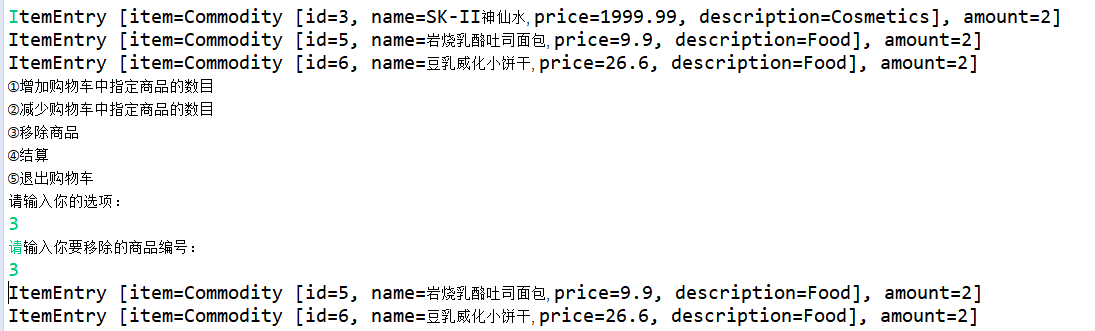
7.结算页面:
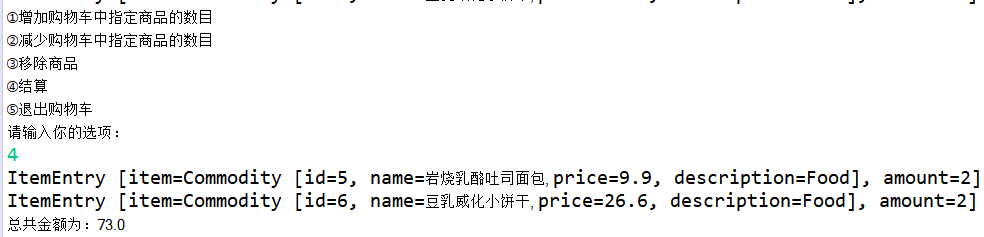
关键代码:
1.商品类:定义商品属性
/**
* 商品类
*/
public class Commodity {
private Integer id;//商品编号
private String name;//商品名称
private Double price;//商品价格
private String description;//商品类别
public Commodity() {
}
public Commodity(Integer id, String name,Double price,String description) {
this.id = id;
this.name = name;
this.price = price;
this.description=description;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Double getPrice() {
return price;
}
public void setPrice(Double price) {
this.price = price;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((id == null) ? 0 : id.hashCode());
return result;
}
@Override
public boolean equals(Object obj) { //只比较id,id相同则说明两个商品相同
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
Commodity other = (Commodity) obj;
if (id == null) {
if (other.id != null)
return false;
} else if (!id.equals(other.id))
return false;
return true;
}
@Override
public String toString() {
return "Commodity [id=" + id + ", name=" + name + ", price=" + price + ", description=" + description + "]";
}
}
2.DAO接口:
MallDAO商城接口:
package shopping;
import java.util.Scanner;
public interface MallDao {
public void addMall(Commodity e);
public void showCommodityKinds(int choice);
public void showCommodity();
public Commodity findById(Integer id);
}
CartDAO购物车接口:
package shopping;
import java.util.Scanner;
public interface CartDao {
public void inputCommodity(CartDao shoppingCart, MallDao shoppingMall);
public boolean increaseAmount();
public boolean decreaseAmount();
public boolean remove();
public void diplayAll();
public double checkout();
}
3.MallDaoImpl商城类
对商城卖家进行进行商品的添加,查找商品以及展示货品。
/**
* 商城类
*/
public class MallDaoImpl implements MallDao {
private List<Commodity> MallList = new ArrayList<>();
public void addMall(Commodity e){
//添加商品至商城
if (e == null){
return ;
}
MallList.add(e);//加入商品到商城
}
public void showCommodityKinds(int choice) {
// 按类展示货架上的商品
switch(choice) {
case 1:
System.out.println("Food:");
for(Commodity x:MallList) {
if(x.getDescription()=="Food")
System.out.println(x);
}
break;
case 2:
System.out.println("Cosmetics:");
for(Commodity x:MallList) {
if(x.getDescription()=="Cosmetics")
System.out.println(x);
}
break;
case 3:
System.out.println("Dayarticles:");
for(Commodity x:MallList) {
if(x.getDescription()=="Dayarticles")
System.out.println(x);
break;
}
}
}
public void showCommodity() {
// 展示货架上所有的商品
for(Commodity x:MallList) {
System.out.println(x);
}
}
public Commodity findById(Integer id){
//查找在该商场中是否存在该商品
if(id<1||id>MallList.size())
{
System.out.println("商城中不存在该商品");
return null;
}
return MallList.get(id-1);
}
}
4.CartDaoImpl购物车类
对购物车内的商品进行增加,减少,移除,结算等操作,还可以展示购物车
/**
* 购物车类
*/
public class CartDaoImpl implements CartDao {
private List<ItemEntry> itemList=new ArrayList<>();
public CartDaoImpl(){
}
public void inputCommodity(CartDao shoppingCart, MallDao shoppingMall) {
//从商城中向购物车添加商品
Scanner sc = new Scanner(System.in);
Commodity e=new Commodity();
System.out.println("请输入你想要购买的商品编号的编号:");
int id = sc.nextInt();
e=shoppingMall.findById(id);
if (e == null)
{
return ;
}
int index = findById(e.getId());
System.out.print("请输入您所要购买的该商品的数目:");
Integer n=sc.nextInt();
if (index == -1)//如果购物车不包含该商品,添加
{
itemList.add(new ItemEntry(e,n));
}
else//否则,增加该商品数量
{
itemList.get(index).increase(n);
}
}
public boolean increaseAmount(){
//增加购物车原有商品数量
Scanner sc=new Scanner(System.in);
int id;
System.out.println("请输入你要增加的商品编号:");
id=sc.nextInt();
if (id<1||id>8)
{
return false;
}
int index = findById(id);
if (index == -1)//如果购物车不包含该商品,输入错误
{
return false;
}
else//否则,增加该商品在购物车中的数量
{
System.out.print("请输入您所要增加的该商品的数目:");
Integer n=sc.nextInt();
itemList.get(index).increase(n);
}
return true;
}
public boolean decreaseAmount(){
//从购物车减少商品数目
Scanner sc=new Scanner(System.in);
int id;
System.out.println("请输入你要减少的商品编号:");
id=sc.nextInt();
int index = findById(id);
if (index == -1)//未找到该商品编号
{
return false;
}
else//购物车存在该商品
{
ItemEntry entry = itemList.get(index);
if (entry.getAmount() <= 1)//若相关商品条目数量amount<=0,删除条目
{
itemList.remove(index);
}
else
{
System.out.print("请输入您所要减少的该商品的数目:");
Integer n=sc.nextInt();
entry.decrease(n);
}
}
return true;
}
public boolean remove(){
//从购物车移除商品相关条目
Scanner sc=new Scanner(System.in);
int id;
System.out.println("请输入你要移除的商品编号:");
id=sc.nextInt();
int index = findById(id);
if (index == -1)//未找到该商品编号
{
return false;
}
else//购物车存在该商品
{
itemList.remove(index);
}
return true;
}
public void diplayAll(){
//展示购物车
for (ItemEntry itemEntry : itemList)
{
System.out.println(itemEntry);
}
}
public double checkout() {
//结算金额
double x = 0;
for (ItemEntry item : itemList) {
x = x + item.getItem().getPrice() * item.getAmount();// 价格*数量
}
return x;
}
private int findById(int id){
//通过id在购物车中查找是否已存在相关条目。
for (int i = 0; i < itemList.size(); i++) {
if (itemList.get(i).getItem().getId().equals(id))
return i;
}
return -1;
}
/*
* 内部类:购物车商品条目类
*/
private class ItemEntry{
Commodity item;//商品
Integer amount;//商品数量
public ItemEntry(Commodity item,Integer amount) {
this.item = item;
this.amount = amount;
}
//数量增加
public void increase(Integer n){
amount+=n;
}
//商品数量减少
public void decrease(Integer n){
amount-=n;
}
public Commodity getItem() {
return item;
}
public Integer getAmount() {
return amount;
}
@Override
public String toString() {
return "ItemEntry [item=" + item + ", amount=" + amount + "]";
}
}
}
5.菜单类
商城主页面引导买家进行购物
/**
* 菜单类
*/
public class Menu {
public static void showMainFunction() {
System.out.println("①商品购买(加入购物车)");
System.out.println("②进入购物车");
System.out.println("③退出商城");
}
public static void showCartFunction() {
System.out.println("①增加购物车中指定商品的数目");
System.out.println("②减少购物车中指定商品的数目");
System.out.println("③移除商品");
System.out.println("④结算");
System.out.println("⑤退出购物车");
}
public static void showSearchFunction() {
System.out.println("①按类别查找商城内的物品");
System.out.println("②浏览商城中的所有物品");
System.out.println("③退出查找");
}
public static void showCommodityKinds() {
System.out.println("商城中含有以下类别的商品,请输入相应的序号进行查找");
System.out.println("1-Food");
System.out.println("2-Cosmetics");
System.out.println("3-Dayarticles");
}
}
GUI购物车登录界面:
登录界面
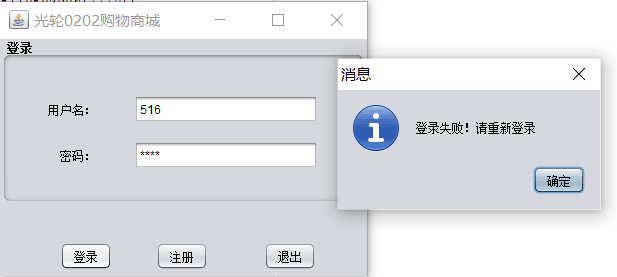
点击注册跳转到注册界面:
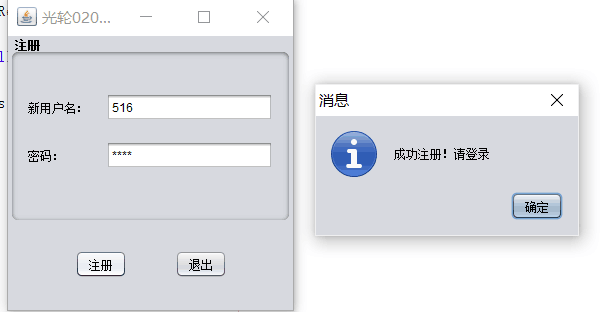
点击确定自动跳转到登录界面:
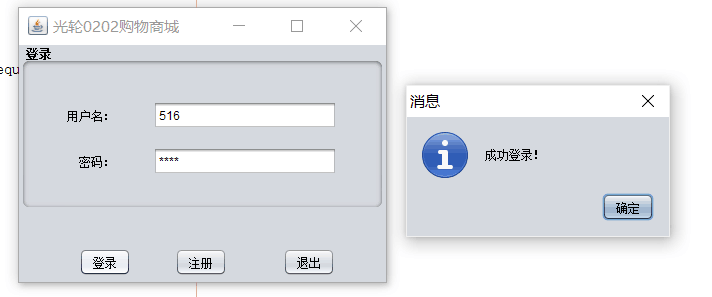
商城购物车界面:
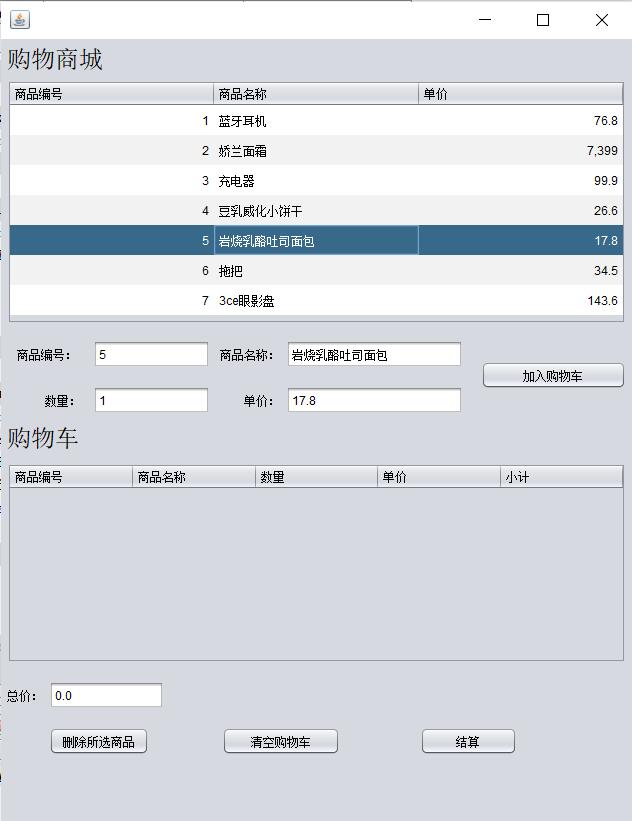
按加入购物车按钮将商品加入购物车,数量可改变:
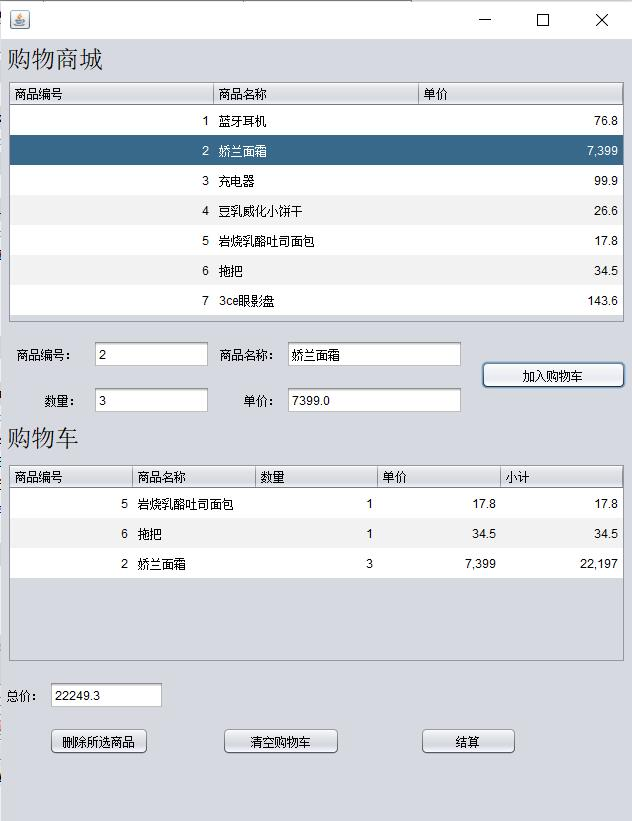
删除购物车中所选中的商品:
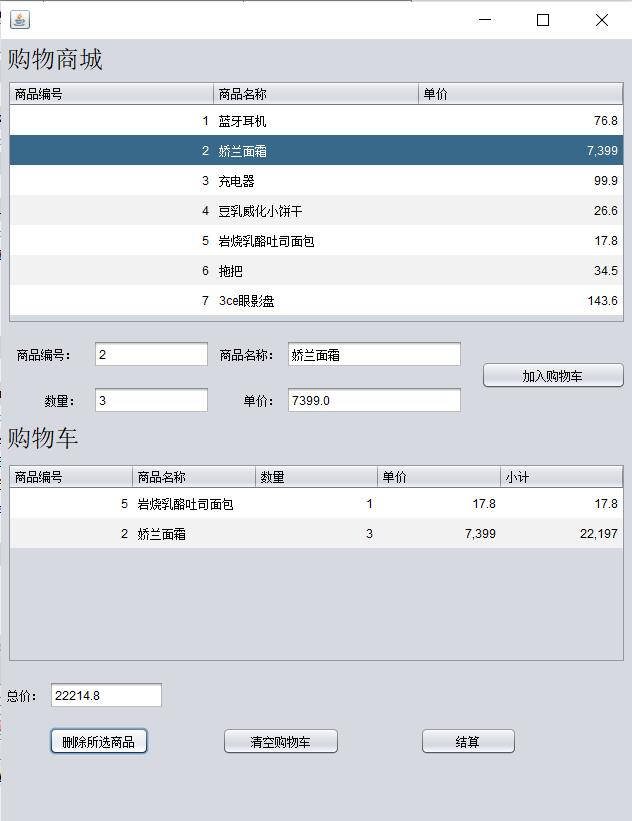
清空购物车:
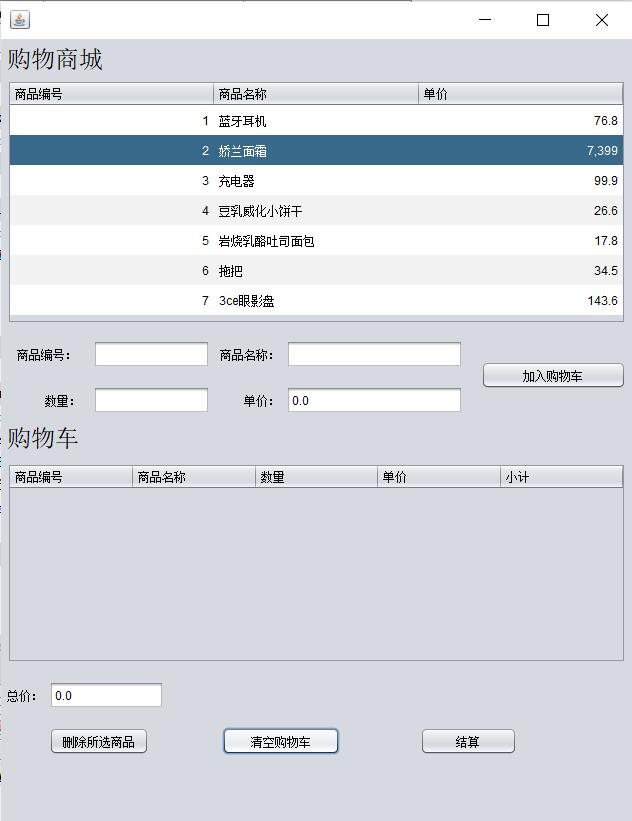
结算订单界面;
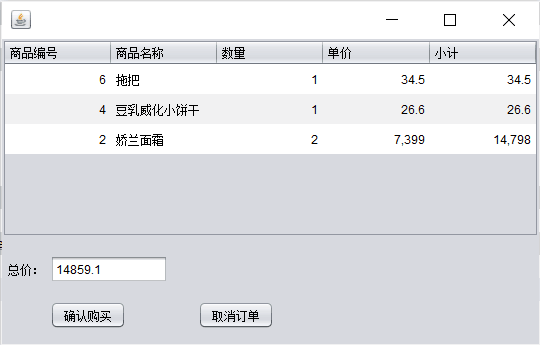
确认购买后直接退出商城,取消订单可回到商城界面:
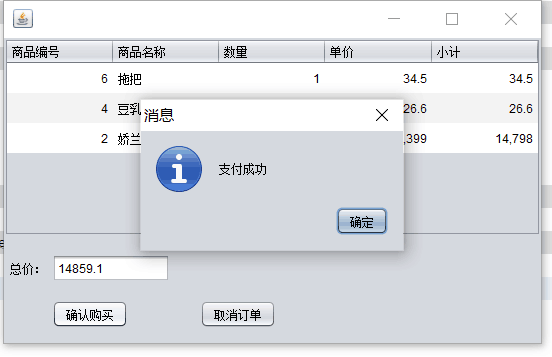
GUI关键代码:
登录界面登录按钮代码:
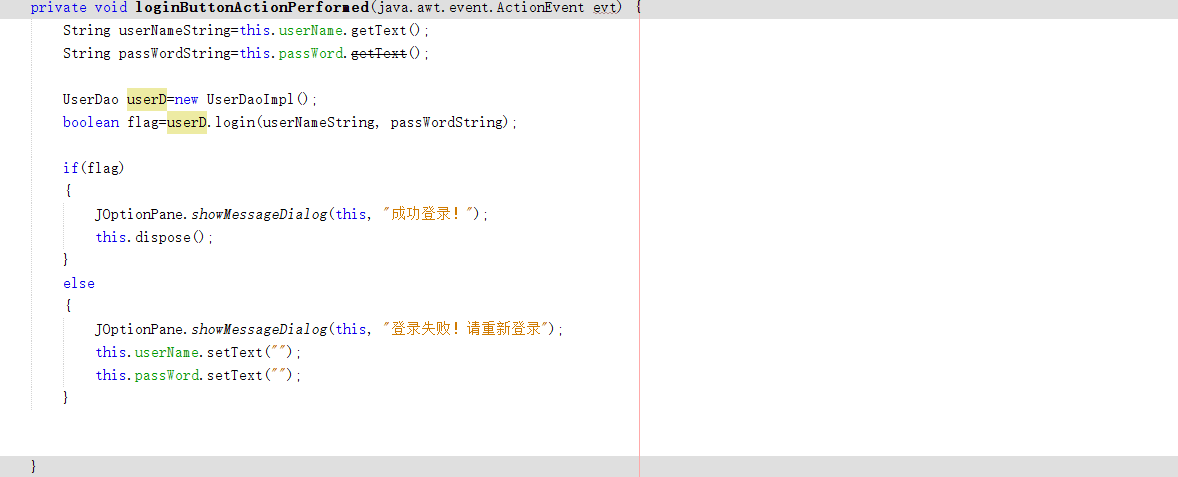
文件处理登录用户名密码代码:
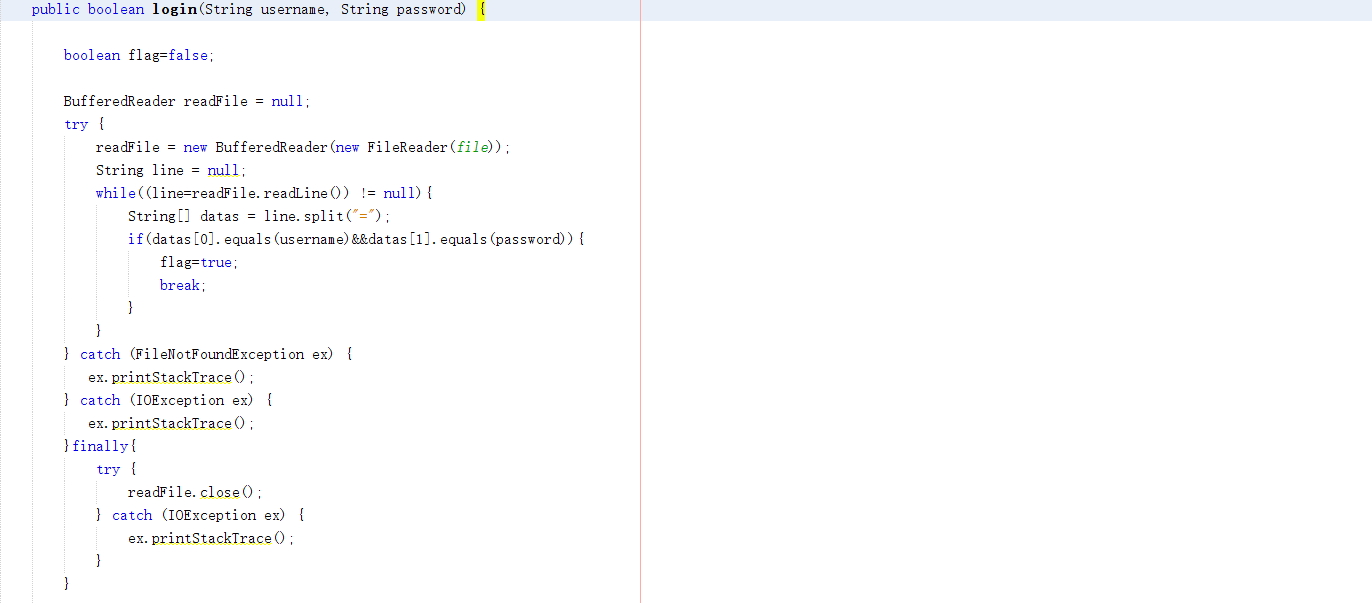
商城购物车功能代码:
//商城表格按行读取
private void MallTableMouseClicked(java.awt.event.MouseEvent evt) {
// TODO add your handling code here:
int row=MallTable.getSelectedRow();
idTextField.setText(MallTable.getValueAt(row, 0).toString());
countTextField.setText("1");
nameTextField.setText(MallTable.getValueAt(row, 1).toString());
priceTextField.setText(MallTable.getValueAt(row, 2).toString());
countTextField.setEditable(true);//数量可写入
}
//添加商品到购物车
private void addCommodityButtonActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
DefaultTableModel model=(DefaultTableModel)CartTable.getModel();
Vector v=new Vector();
int row=MallTable.getSelectedRow();
v.add(0,idTextField.getText());
v.add(1,nameTextField.getText());
v.add(2,countTextField.getText());
v.add(3,Double.valueOf(priceTextField.getText()));
Double totalPrice=(Double.valueOf(countTextField.getText())*Double.valueOf(priceTextField.getText()));
v.add(4,totalPrice);
model.addRow(v);
//总价修改
Double total=0.00;
for (int i = 0; i < CartTable.getRowCount(); i++) {
total+=Double.valueOf(CartTable.getValueAt(i, 4).toString());
}
totalPriceTextField.setText(String.valueOf(total));
}
//删除购物车中选中的商品
private void deleteButtonActionPerformed(java.awt.event.ActionEvent evt) {
// TODO add your handling code here:
DefaultTableModel model=(DefaultTableModel)CartTable.getModel();
int row=CartTable.getSelectedRow();
model.removeRow(row);
//总价修改
Double total=0.00;
for (int i = 0; i < CartTable.getRowCount(); i++) {
total+=Double.valueOf(CartTable.getValueAt(i, 4).toString());
}
totalPriceTextField.setText(String.valueOf(total));
}