数据结构之字典树
Trie又被称为前缀树、字典树,所以当然是一棵树。上面这棵Trie树包含的字符串集合是{in, inn, int, tea, ten, to}。每个节点的编号是我们为了描述方便加上去的。树中的每一条边上都标识有一个字符。这些字符可以是任意一个字符集中的字符。比如对于都是小写字母的字符串,字符集就是’a’-‘z’;对于都是数字的字符串,字符集就是’0’-‘9’;对于二进制字符串,字符集就是0和1。
比如上图中3号节点对应的路径0123上的字符串是inn,8号节点对应的路径0568上的字符串是ten。终结点与集合中的字符串是一一对应的。
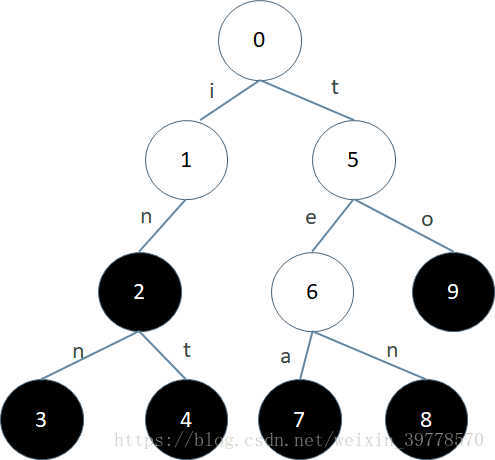
具体来说,Trie一般支持两个操作:
1. Trie.insert(W):第一个操作是插入操作,就是将一个字符串W加入到集合中。
2. Trie.search(S):第二个操作是查询操作,就是查询一个字符串S是不是在集合中
1. Trie.insert(W):第一个操作是插入操作,就是将一个字符串W加入到集合中。
2. Trie.search(S):第二个操作是查询操作,就是查询一个字符串S是不是在集合中
1 #include"字典树Trie.h" 2 #include<iostream> 3 using namespace std; 4 5 Trie* Trie::create_Trie() { 6 Trie *root = (Trie*)malloc(sizeof(Trie)); 7 root->count = 0; 8 for (int i = 0; i < MAX; i++) { 9 root->next[i] = NULL; 10 } 11 return root; 12 } 13 14 void Trie::insert_word(Trie *root, char *str) { 15 int len = strlen(str); 16 Trie *tmp = root; 17 for (int i = 0; i < len; i++) { 18 int index = str[i] - 'a'; 19 if (root->next[index] == NULL) { 20 Trie *tmp = new Trie(); 21 root->next[index] = tmp; 22 root = root->next[index]; 23 } 24 else { 25 root = root->next[index]; 26 root->count += 1; 27 } 28 } 29 } 30 int Trie::count_Trie(Trie *root, char *str) { 31 int len = strlen(str); 32 for (int i = 0; i < len; i++) { 33 int index = str[i] - 'a'; 34 if (!root->next[index])return 0; 35 else 36 root = root->next[index]; 37 } 38 return root->count; 39 } 40 41 void Trie::destory_Trie(Trie *root) { 42 for (int i = 0; i < MAX; i++) { 43 if (root->next[i])destory_Trie(root->next[i]); 44 } 45 free(root); 46 }