Python学习-终端字体高亮显示
1、采用原生转义字符序列,对Windows有的版本不支持(比如win7),完美支持Linux
实现过程:
终端的字符颜色是用转义序列控制的,是文本模式下的系统显示功能,和具体的语言无关。
转义序列是以ESC开头,即用\033来完成(ESC的ASCII码用十进制表示是27,用八进制表示就是033)。
格式:
开头部分:\033[显示方式;前景色;背景色m + 结尾部分:\033[0m
前景色 | 背景色 | 颜色 |
30 | 40 | 黑色 |
31 | 41 | 红色 |
32 | 42 | 绿色 |
33 | 43 | 黄色 |
34 | 44 | 蓝色 |
35 | 45 | 紫红色 |
36 | 46 | 青蓝色 |
37 | 47 | 白色 |
显示方式:
显示方式 | 意义 |
0 | 终端默认设置 |
1 | 高亮显示 |
4 | 使用下划线 |
5 | 闪烁 |
7 | 反白显示 |
8 | 不可见 |
1 #!/usr/bin/env python3 2 # -*- coding: utf-8 -*- 3 # @Time : 2018/4/29 10:27 4 # @Author : yang 5 # @File : Colored_Escape_character.py 6 # @Software: PyCharm 7 #-------------------------------- 8 #显示格式:\033[显示方式;前景色;背景色m 9 #-------------------------------- 10 #显示方式 说明 11 # 0 终端默认设置 12 # 1 高亮显示 13 # 4 使用下划线 14 # 5 闪烁 15 # 7 反白显示 16 # 8 不可见 17 # 22 非粗体 18 # 24 非下划线 19 # 25 非闪烁 20 # 21 #前景色 背景色 颜色 22 # 30 40 黑色 23 # 31 41 红色 24 # 32 42 绿色 25 # 33 43 黄色 26 # 34 44 蓝色 27 # 35 45 紫红色 28 # 36 46 青蓝色 29 # 37 47 白色 30 #--------------------------------------- 31 class Colored(object): 32 RED = '\033[31m' #红色 33 GREEN = '\033[32m' #绿色 34 YELLOW = '\033[33m' #黄色 35 BLUE = '\033[34m' #蓝色 36 FUCHSIA = '\033[35m' #紫红色 37 CYAN = '\033[36m' #青蓝色 38 WHITE = '\033[37m' #白色 39 #:no color 40 RESET = '\033[0m' #终端默认颜色 41 def color_str(self,color,s): 42 return '{}{}{}'.format(getattr(self,color),s,self.RESET) 43 44 def red(self,s): 45 return self.color_str('RED',s) 46 def green(self,s): 47 return self.color_str('GREEN',s) 48 def yellow(self,s): 49 return self.color_str('YELLOW',s) 50 def blue(self,s): 51 return self.color_str('BLUE',s) 52 def fuchsia(self,s): 53 return self.color_str('FUCHSIA',s) 54 def cyan(self,s): 55 return self.color_str('CYAN',s) 56 def white(self,s): 57 return self.color_str('WHITE',s) 58 #-----------使用示例如下-------- 59 color = Colored() 60 print(color.red('I am red!')) 61 print(color.green('I am green!')) 62 print(color.yellow('I am yellow!')) 63 print(color.blue('I am blue!')) 64 print(color.fuchsia('I am fuchsia!')) 65 print(color.cyan('I am cyan!')) 66 print(color.white('I am white!'))
输出结果:
2、采用Python标准库colorama模块--兼容linux和windows各个版本:
1 #!/usr/bin/env python3 2 # -*- coding: utf-8 -*- 3 # @Time : 2018/4/29 10:57 4 # @Author : yang 5 # @File : Colored_Colorama_module.py 6 # @Software: PyCharm 7 #--------------colorama模块的一些常量------- 8 #colorama是一个python专门用来在控制台、命令行输出彩色文字的模块,可以跨平台使用 9 # 在windows下linux下都工作良好,如果你想让控制台的输出信息更漂亮一些,可以使用给这个模块。 10 # Fore: BLACK, RED, GREEN, YELLOW, BLUE, MAGENTA, CYAN, WHITE, RESET. 11 # Back: BLACK, RED, GREEN, YELLOW, BLUE, MAGENTA, CYAN, WHITE, RESET. 12 # Style: DIM, NORMAL, BRIGHT, RESET_ALL 13 from colorama import init,Fore,Back,Style 14 #init(autoreset=True) 15 class Colored(object): 16 def red(self,s): 17 return Fore.RED + s + Fore.RESET 18 def green(self,s): 19 return Fore.GREEN + s + Fore.RESET 20 def yellow(self,s): 21 return Fore.YELLOW + s + Fore.RESET 22 def blue(self,s): 23 return Fore.BLUE + s + Fore.RESET 24 def magenta(self,s): 25 return Fore.MAGENTA + s + Fore.RESET 26 def cyan(self,s): 27 return Fore.CYAN + s + Fore.RESET 28 def white(self,s): 29 return Fore.WHITE + s + Fore.RESET 30 def balck(self,s): 31 return Fore.BLACK 32 def white_green(self,s): 33 return Fore.WHITE + Back.GREEN + s + Fore.RESET + Back.RESET 34 color = Colored() 35 print(color.red('I am red!')) 36 print(color.green('I am green!')) 37 print(color.yellow('I am yellow!')) 38 print(color.blue('I am blue!')) 39 print(color.magenta('I am magenta!')) 40 print(color.cyan('I am cyan!')) 41 print(color.white('I am white!')) 42 print(color.white_green('I am white green!'))
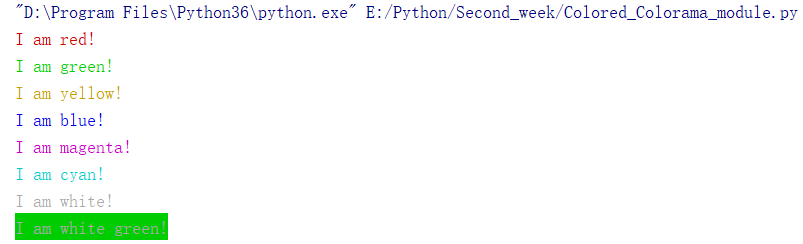
termcolor是一个python包,可以改变控制台输出的颜色,支持各种terminal(WINDOWS的cmd.exe除外)。
支持下列的文字颜色:
grey, red, green, yellow, blue, magenta, cyan, white
支持下列的背景高亮:
on_grey, on_red, on_green, on_yellow, on_blue, on_magenta, on_cyan, on_white
支持下列属性:
bold, dark, underline, blink, reverse, concealed
1 #!/usr/bin/env python3 2 # -*- coding: utf-8 -*- 3 # @Time : 2018/4/29 16:49 4 # @Author : yang 5 # @File : Colored_Termcolor_module.py 6 # @Software: PyCharm 7 import sys 8 from termcolor import colored,cprint 9 text = colored('Hello,World!','red',attrs=['reverse','blink']) 10 11 #colored(text, color=None, on_color=None, attrs=None) 12 # Available text colors: 13 # red, green, yellow, blue, magenta, cyan, white. 14 15 # Available text highlights: 16 # on_red, on_green, on_yellow, on_blue, on_magenta, on_cyan, on_white. 17 18 # Available attributes: 19 # bold, dark, underline, blink, reverse, concealed. 20 #print('\033[5;7;31mHello,World!\033[0m') 21 22 print(text) 23 24 cprint('Hello,World!','green','on_red') 25 #cprint('Hello,World!','green','on_red',attrs=['bold']) 26 #def cprint(text, color=None, on_color=None, attrs=None, **kwargs) 27 28 print_red_on_cyan = lambda x:cprint(x,'red','on_cyan') 29 print_red_on_cyan('Hello,World!') 30 print_red_on_cyan('Hello,Universe!') 31 for i in range(10): 32 cprint(i,'magenta',end=' ') 33 cprint('Attention!','red',attrs=['bold'],file = sys.stderr)
输出结果:
参考:
1、https://pypi.org/project/colorama/
2、https://pypi.org/project/termcolor/#description
3、https://www.cnblogs.com/hellojesson/p/5961570.html
4、https://stackoverflow.com/questions/287871/print-in-terminal-with-colors/3332860#3332860