LeetCode - 24. Swap Nodes in Pairs
Given a linked list, swap every two adjacent nodes and return its head.
Example:
Given1->2->3->4
, you should return the list as2->1->4->3
.
Note:
- Your algorithm should use only constant extra space.
- You may not modify the values in the list's nodes, only nodes itself may be changed.
交换链表中的节点,题目不难,需要细心,有两种解法。
1.递归,逻辑清晰。用临时节点保存交换中的中间节点,以防链表断裂,节点丢失。
class Solution { public ListNode swapPairs(ListNode head) { if (head == null || head.next == null) return head; ListNode temp = head.next; head.next = swapPairs(head.next.next); temp.next = head; return temp; } }
2.直接循环做,需要一个假的头节点来保存交换之后的头节点,同样需要临时节点保存交换中的中间节点。
class Solution { public ListNode swapPairs(ListNode head) { if (head == null || head.next == null) return head; ListNode fakeHead = new ListNode(0), pre = fakeHead, temp = null; fakeHead.next = head; while (pre.next!=null && pre.next.next!=null) { temp = pre.next.next; pre.next.next = temp.next; temp.next = pre.next; pre.next = temp; pre = temp.next; } return fakeHead.next; } }
在LeetCode上循环比递归耗时减少1ms
作者:Pickle
声明:对于转载分享我是没有意见的,出于对博客园社区和作者的尊重一定要保留原文地址哈。
致读者:坚持写博客不容易,写高质量博客更难,我也在不断的学习和进步,希望和所有同路人一道用技术来改变生活。觉得有点用就点个赞哈。
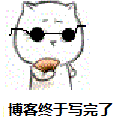

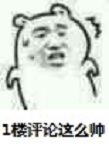
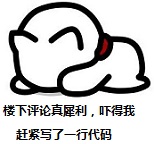
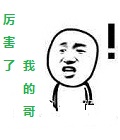
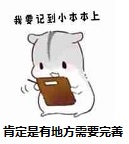

