LeetCode - 141. Linked List Cycle
Given a linked list, determine if it has a cycle in it.
Follow up:
Can you solve it without using extra space?
经典问题,判断一个单向链表是否有环,快慢指针,有相遇即有环。
/** * Definition for singly-linked list. * class ListNode { * int val; * ListNode next; * ListNode(int x) { * val = x; * next = null; * } * } */ public class Solution { public boolean hasCycle(ListNode head) { if (head == null) return false; ListNode fast = head, slow = head; int cnt = 0; while (true) { if (fast == null) return false; if (cnt > 0 && fast == slow) return true; slow = slow.next; fast = fast.next; if (slow == null || fast == null) return false; fast = fast.next; cnt ++; } } }
作者:Pickle
声明:对于转载分享我是没有意见的,出于对博客园社区和作者的尊重一定要保留原文地址哈。
致读者:坚持写博客不容易,写高质量博客更难,我也在不断的学习和进步,希望和所有同路人一道用技术来改变生活。觉得有点用就点个赞哈。
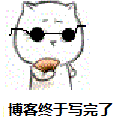

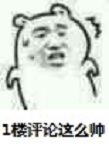
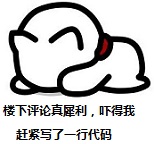
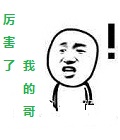
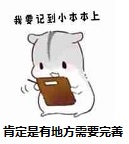

