LeetCode - 768. Max Chunks To Make Sorted II
This question is the same as "Max Chunks to Make Sorted" except the integers of the given array are not necessarily distinct, the input array could be up to length 2000
, and the elements could be up to 10**8
.
Given an array arr
of integers (not necessarily distinct), we split the array into some number of "chunks" (partitions), and individually sort each chunk. After concatenating them, the result equals the sorted array.
What is the most number of chunks we could have made?
Example 1:
Input: arr = [5,4,3,2,1] Output: 1 Explanation: Splitting into two or more chunks will not return the required result. For example, splitting into [5, 4], [3, 2, 1] will result in [4, 5, 1, 2, 3], which isn't sorted.
Example 2:
Input: arr = [2,1,3,4,4] Output: 4 Explanation: We can split into two chunks, such as [2, 1], [3, 4, 4]. However, splitting into [2, 1], [3], [4], [4] is the highest number of chunks possible.
class Solution { public int maxChunksToSorted(int[] arr) { if (arr == null) return 0; int[] arr2 = new int[arr.length]; for (int i=0; i<arr.length; i++) { arr2[i] = arr[i]; } Arrays.sort(arr2); int sum1 = 0, sum2 = 0, ret = 0; for (int i=0; i<arr.length; i++) { sum1 += arr[i]; sum2 += arr2[i]; if (sum1 == sum2) ret ++; } return ret; } }
作者:Pickle
声明:对于转载分享我是没有意见的,出于对博客园社区和作者的尊重一定要保留原文地址哈。
致读者:坚持写博客不容易,写高质量博客更难,我也在不断的学习和进步,希望和所有同路人一道用技术来改变生活。觉得有点用就点个赞哈。
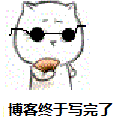

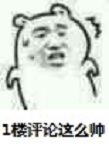
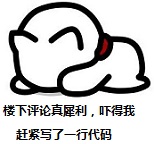
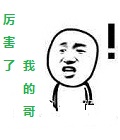
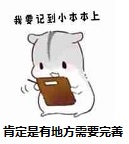

