#!/usr/bin/env python
# coding: utf-8
# # Think Python 学习笔记
# 1.关于异或计算符
# In[2]:
6^2
# 2.关于函数
# 注意:变量名称不能用数字来开头
# In[3]:
import math
degree = 45
radians = degree/180 * math.pi
math.sin(radians)
# 3.关于自定义函数,关于形参和实参
# In[4]:
def print_twice(words):
print(words)
print(words)
print_twice('whao')
# 练习1 写一个名叫right_justify的函数,形参是s的字符串,前面留出足够的空格,让字符串最后一个字幕在第70列显示。
# In[5]:
def right_justify(s):
print(' '*(70-len(s)),s)
s1='I am whao.'
s2='I have a handsome son.'
right_justify(s1)
right_justify(s2)
# 练习2 修改一下do_twice这个函数, 让它接收两个实际参数, 一个是函数对象, 一个是值, 调用对象函数两次, 并且赋这个值给对象函数作为实际参数。
# In[6]:
def do_twice(right_justifi,s):
right_justifi(s)
right_justifi(s)
do_twice(right_justify,s1)
# 4.关于乌龟模块,用乌龟模块画个正方形
# In[7]:
import turtle
bob = turtle.Turtle()
bob.pd
for i in range(4):
bob.fd(100)
bob.lt(90)
# 练习3
# 下面是一系列使用TurtleWorld的练习。
# 1.写一个函数叫做square( 译者注: 就是正方形的意思) , 有一个名叫t的参数, 这个t是一个
# turtle。 用这个turtle来画一个正方形。 写一个函数调用, 把bob作为参数传递给square, 然后
# 再运行这个程序。
# 2.给这个square函数再加一个参数, 叫做length( 译者注: 长度) 。 把函数体修改一下, 让长
# 度length赋值给各个边的长度, 然后修改一下调用函数的代码, 再提供一个这个对应长度的参
# 数。 再次运行一下, 用一系列不同的长度值来测试一下你的程序。
# 3.复制一下square这个函数, 把名字改成polygon( 译者注: 意思为多边形) 。 另外添加一个
# 参数叫做n, 然后修改函数体, 让函数实现画一个正n边的多边形。 提示: 正n多边形的外角为
# 360/n度。
# 4.在写一个叫做circle( 译者注: 圆) 的函数, 也用一个turtle类的对象t, 以及一个半径r, 作
# 为参数, 画一个近似的圆, 通过调用polygon函数来近似实现, 用适当的边长和边数。 用不同
# 的半径值来测试一下你的函数。
# 提示: 算出圆的周长, 确保边长乘以边数的值( 近似) 等于圆周长。
# 5.在circle基础上做一个叫做arc的函数, 在circle的基础上添加一个angle( 译者注: 角度) 变
# 量, 用这个角度值来确定画多大的一个圆弧。 用度做单位, 当angle等于360度的时候, arc函
# 数就应当画出一个整团了。
# In[8]:
def square(t):
t.pd
for i in range(4):
t.fd(100)
t.lt(90)
import turtle
bob = turtle.Turtle()
square(bob)
# In[9]:
def square(t,length):
t.pd
for i in range(4):
t.fd(length)
t.lt(90)
import turtle
bob = turtle.Turtle()
square(bob,200)
# In[10]:
def polygon(t,length,n):
t.pd
for i in range(n):
t.fd(length)
t.lt(360/n)
import turtle
bob = turtle.Turtle()
polygon(bob,100,5)
# In[11]:
def polygon(t,length,n):
t.pd
for i in range(n):
t.fd(length)
t.lt(360/n)
def circle(t,r):
z=6.28*r
length=10
n=int(z/length)
polygon(t,length,n)
import turtle
bob = turtle.Turtle()
circle(bob,100)
# In[12]:
import math
def polygon(t,length,n,angle):
t.pd
for i in range(int(n*angle/360)):
t.fd(length)
t.lt(360/n)
def arc(t,r,angle):
z=2*math.pi*r
length=10
n=int(z/length)
polygon(t,length,n,angle)
import turtle
bob = turtle.Turtle()
arc(bob,100,120)
# 5.关于三目条件句
# In[13]:
def is_more(a,b):
#print('1') if n>0 else print('2')
print('%d>%d'%(a,b))if a>b else print('%d<=%d'%(a,b))
is_more(-8,7)
# 6.关于递归运算
# In[14]:
def count_down(n):
if n<=0:
print("downoff")
else:
print(n)
count_down(n-1)
count_down(3)
# 7.关于键盘输入
# In[ ]:
name = input('please input you name:\n')
name
# 8.关于有返回值的函数
# In[ ]:
def area(radius):
a = math.pi * radius**2
return a
area(1)
# In[ ]:
def factorial(n):
"""n阶乘法计算"""
if n==0:
return 1
else:
m = factorial(n-1)
result = n*m
return result
def fibonacci(n):
"""斐波拉契数列"""
if n==0:
return 0
elif n == 1:
return 1
else:
return fibonacci(n-1)+fibonacci(n-2)
r1 = factorial(3)
r2 = fibonacci(4)
print('factorial(3) is %d.\nfibonacci(2) is %d.'%(r1, r2))
# 9.关于while语句
# 练习4 写一个叫做eval_loop的函数, 交互地提醒用户, 获取输入, 然后用eval对输入进行运算, 把
# 结果打印出来。这个程序要一直运行, 直到用户输入『done』 才停止, 然后输出最后一次计算的表达式的值。
# In[6]:
def eval_loop(s):
return eval(s)
import math
while True:
inputV = input('please input the value(must be data): \n')
if inputV != 'done':
the_last = eval_loop(inputV)
else:
print('the last input value is %d'%the_last)
break
# 10.关于字符串
# 字符串是有序排列,不可修改
# In[ ]:
例1.在 Robert McCloskey 的一本名叫《Make Way for Ducklings》 的书中, 小鸭子的名字依次
为: Jack, Kack, Lack, Mack, Nack, Ouack, Pack, 和Quack。 下面这个循环会依次输出他们
的名字:
# In[7]:
prefixes = 'JKLMNOPQ'
suffix = 'ack'
for letter in prefixes:
print(letter + 'u'+suffix) if letter == 'O'or letter == 'Q' else print(letter+suffix)
# if letter == 'O'or letter == 'Q':
# print(letter + 'u'+suffix)
# else:
# print(letter+suffix)
# In[ ]:
11.关于基于字符串的循环和计数
# In[2]:
words = 'banana baaaala'
count = 0
for letter in words:
if letter == 'a':
count = count +1
print(count)
# 练习5 凯撒密码是一种简单的加密方法, 用的方法是把每个字母进行特定数量的移位。 对一个字母移位就是把它根据字母表的顺序来增减对应, 如果到末尾位数不够就从开头算剩余的位数,『A』 移位3就是『D』 , 而『Z』移位1就是『A』了。
# In[32]:
"""先搭建框架,再丰富完善"""
import string
def rotate_word(s,n):
lc = string.ascii_lowercase
uc = string.ascii_uppercase
r=''
for i in s:
if i in lc:
print('%s is lowercase'%i)
elif i in uc:
print('%s is upercase'%i)
else:
print('%s is other'%i)
return r
#s = input('please input the word:\n')
#n = input('please input the moved step num(1-26):\n')
s = 'U00pkiip'
n = 13
r = rotate_word(s,n)
print('加密前文字为:',s)
print('加密后文字为:',r)
# In[56]:
def rotate_word_enc(s,n):
"""凯撒古典加密对称算法"""
lc = string.ascii_lowercase
uc = string.ascii_uppercase
r=''
for i in s:
if i in lc:
#print('%s is lowercase'%i)
pos = ord(i) + n - 1 - ord('z')+ord('a') if (ord(i) + n) > ord('z') else (ord(i) + n)
r = r + chr(pos)
elif i in uc:
#print('%s is ur = r + chr(pos)percase'%i)
pos = ord(i) + n - 1 - ord('Z')+ord('A') if (ord(i) + n) > ord('Z') else (ord(i) + n)
r = r + chr(pos)
else:
#print('%s is other'%i)
r = r + i
return r
def rotate_word_dec(r,n):
"""凯撒古典解密对称算法"""
lc = string.ascii_lowercase
uc = string.ascii_uppercase
s=''
for i in r:
if i in lc:
pos = (ord('z') - (ord('a') - ord(i) + n - 1)) if (ord(i) - n) < ord('a') else (ord(i) - n)
s = s + chr(pos)
elif i in uc:
pos = (ord('Z') - (ord('A') - ord(i) + n - 1)) if (ord(i) - n) < ord('A') else (ord(i) - n)
s = s + chr(pos)
else:
s = s + i
return s
#主程序
import string
s = input('please input the word:\n')
n = int(input('please input the moved step num(1-26):\n')) #注意进行格式转换
#s = 'U00pkiip'
#n = 13
r = rotate_word_enc(s,n)
s1 = rotate_word_dec(r,n)
print('加密前文字为:',s)
print('加密后文字为:',r)
print('解密后文字为:',s1)
# In[ ]:
11.关于列表
列表是可修改,有序排列
# In[57]:
numbers = [2, 9, 3, 7]
for i in range(len(numbers)): #从0开始计数 下标范围为0- (len-1)
numbers[i] = numbers[i] * 2
numbers
# In[27]:
"""大多数列表的方法都是无返回值的; 这些方法都对列表进行修改, 返回的是空。
如果你不小心写出了一个 t=t.sort(), 得到的结果恐怕让你很失望。"""
t = ['a','c','z','b','e','p']
t1 = t.sort()
t2 = sorted(t)
print('t,t1,t2: ',t,t1,t2)
# Map, filter, reduce 列表中最重要的三种运算
# In[60]:
#将某一函数(如 capitalize)应用到一个序列中的每个元素上称为一个map
def map_capitalize(t):
res = []
for s in t:
res.append(s.capitalize())
return res
t=['a','BaNana','U00','wHa']
map_capitalize(t)
# In[61]:
#reduce
t = [1, 2, 4]
sum(t)
# In[63]:
#filter
def filter_upper(t):
res = []
for s in t:
if s.isupper():
res.append(s)
return res
t = ['A','Baa','CEF','aD']
filter_upper(t)
# In[70]:
#删除列表项的几种方法区别:pop返回删除项,del不返回删除项,remove不知道项数时
t = ['a', 'b', 'c','d']
t1 = t+['e', 'f', 'g']
x = t.pop(2)
print(x)
del t[0]
del t1[1:5]
print('t is ',t)
print('t1 is ',t1)
t.remove('d')
print('t is ',t)
# In[1]:
s = 'whao'
t = list(s)
t
# In[15]:
s = 'pining for the fjords'
s1 = 'spam-spam-spam'
t = s.split()
delimiter = '-'
t1 = s1.split(delimiter)
delimiter1 =' '
s3 = delimiter1.join(t)
s3
# In[23]:
"""一定要区分修改列表的运算和产生新列表的运算, 这特别重要。 比如 append 方法修改一个列
表, 但加号+运算符是产生一个新的列表"""
t1 = [1,2]
t2 = t1.append(3)
print('t1 and t2:',t1,t2)
t3=t1+[4]
print('t1 and t3:',t1,t3)
# 12.关于字典
# 字典是可修改,无序排列
# In[ ]:
d = dict()
d = {'one': 'uno', 'two': 'dos', 'three': 'tres'}
# 例:用字典作为计数器
# 假设你得到一个字符串, 然后你想要查一下每个字母出现了多少次。 你可以通过以下方法来实现:
# 1. 你可以建立26个变量, 每一个代表一个字母。 然后你遍历整个字符串, 每个字母的个数都累加到对应的计数器里面, 可能会用到分支条件判断。
# 2. 你可以建立一个有26个元素的列表。 然后你把每个字母转换成一个数字( 用内置的 ord函数) , 用这些数字作为这个列表的索引, 然后累加相应的计数器。
# 3. 你可以建立一个字典, 用字母作为键, 用该字母出现的次数作为对应的键值。 第一次遇到一个字母, 就在字典里面加一个项。 此后再遇到这个字母, 就每次在已有的项上进行累加即可。
# In[8]:
def histogram(s):
"""频次统计函数"""
d = dict()
for c in s:
if c not in d:
d[c]= 1
else:
d[c]+= 1
return d
s = 'agdecgbadgwersdghvxdoiadec'
histogram(s)
# In[7]:
def histogram(s):
"""频次统计函数V2.0,用get方法简化"""
d = dict()
for c in s:
d[c] = d.get(c, 0) + 1
# if c not in d:
# d[c]= 1
# else:
# d[c]+= 1
return d
s = 'agdecgbadgwersdghvxdoiadec'
histogram(s)
# In[13]:
def histogram(s):
"""频次统计函数V2.0,用get方法简化"""
d = dict()
for c in s:
d[c] = d.get(c, 0) + 1
return d
s = 'agdecgbadgwersdghvxdoiadec'
t=[]
for c in s:
t +=[str(ord(c))]
histogram(t)
# In[ ]:
13.关于元组
是不可修改,有序的
# In[14]:
"""要建立一个单个元素构成的元组,必须要在结尾加上逗号:"""
t1 = ('a',)
type(t1)
# In[15]:
"""只用括号放一个值则并不是元组:"""
t2 = ('a')
type(t2)
# In[17]:
"""如果参数为一个序列(比如字符串、列表或者元组),结果就会得到一个以该序列元素组成的元组。"""
t3 = tuple('whao')
t3
# In[18]:
"""函数的参数可以有任意多个。用星号*开头来作为形式参数名,可以将所有实际参数收录到一
个元组中。例如 printall 就可以获取任意多个数的参数,然后把它们都打印输出:"""
def printall(*args):
print(args)
printall(1, 2.0,'3')
# In[6]:
#元组比较,会按顺序对元素进行依次比较
t1 = (3,3)
t2 = (4,0)
print(t1>t2)
# 第13章 练习1
# 写一个读取文件的程序,把每一行拆分成一个个词,去掉空白和标点符号,然后把所有单词
# 都转换成小写字母的。
# 提示:字符串模块 string 提供了一个名为 whitespace 的字符串,包含了空格、跳表符、另起
# 一行等等,然后还有个 punctuation 模块,包含了各种标点符号的字符。咱们可以试试让
# Python 把标点符号都给显示一下:
# >>> import string
# >>>string.punctuation
# '!"#$%&'()*+,-./:;<=>?@[\]^_`{|}~'
# 另外你也可以试试字符串模块的其他方法,比如 strip、replace 以及 translate。
# 第13章 练习2
# 访问[古登堡计划网站] (http://gutenberg.org),然后下载一个你最喜欢的公有领域的书,要下
# 载纯文本格式的哈。
# 修改一下刚才上一个练习你写的程序,让这个程序能读取你下载的这本书,跳过文件开头部
# 分的信息,继续以上个练习中的方式来处理一下整本书的正文。
# 然后再修改一下程序,让程序能统计一下整本书的总单词数目,以及每个单词出现的次数。
# 输出一下这本书中不重复的单词的个数。对比一下不同作者、不同地域的书籍。哪个作者的
# 词汇量最丰富?
# 第13章 练习3
# 再接着修改程序,输出一下每本书中最频繁出现的20个词。
# In[ ]:
练习4
接着修改,让程序能读取一个单词列表(参考9.1),然后输出一下所有包含在书中,但不包
含于单词列表中的单词。看看这些单词中有多少是排版错误的?有多少是本应被单词列表包
get_ipython().set_next_input('含的常用单词?有多少是很晦涩艰深的罕见词汇');get_ipython().run_line_magic('pinfo', '有多少是很晦涩艰深的罕见词汇')
# In[ ]:
14.随机数
# In[20]:
import random
t = [1, 2, 3]
random.choice(t)
# In[22]:
"""randint函数接收两个参数作为下界和上界,然后返回一个二者之间的整数,这个整数可以是下界或者上界。"""
import random
random.randint(1, 10)
# In[25]:
"""函数random返回一个在0.0到1.0的前闭后开区间"""
import random
for i in range(10):
r = random.random()
print(r)
# In[ ]:
15.关于文件读写
# In[15]:
fo = open('output.txt', 'w')
line1 = 'I am whao.\n'
line2 = 'I live in Guiyang.\n'
r = fo.write(line1)
r1 = fo.write(line2)
print(r,'\t',r1)
fo.close()
fi = open('output.txt')
r2 = fi.readline().strip()
r3 = fi.readline().strip()
print(r2,'\t',r3)
fi.close()
# 例:下面这个例子中,walks 这个函数遍历一个目录,然后输出所有该目录下的文件的名字,并且在该目录下的所有子目录中递归调用自身。
# In[17]:
"""This module contains a code example related to
Think Python, 2nd Edition
by Allen Downey
http://thinkpython2.com
Copyright 2015 Allen Downey
License: http://creativecommons.org/licenses/by/4.0/
"""
import os
def walk(dirname):
"""Prints the names of all files in dirname and its subdirectories.
This is the version in the book.
dirname: string name of directory
"""
for name in os.listdir(dirname):
path = os.path.join(dirname, name)
if os.path.isfile(path):
print(path)
else:
walk(path)
def walk2(dirname):
"""Prints the names of all files in dirname and its subdirectories.
This is the exercise solution, which uses os.walk.
dirname: string name of directory
"""
for root, dirs, files in os.walk(dirname):
for filename in files:
print(os.path.join(root, filename))
if __name__ == '__main__':
#walk('.')
walk2('.')
# 16.关于数据库
# In[23]:
import dbm
db = dbm.open('captions','c') #c模式表示如果该数据库不存在就创建一个新的。得到的返回结果就是一个数据库对象了。
db['cleese.png']= 'Photo of John Cleese.'
db['whao.png']= 'Photo of whao.'
for key in db:
print(key, db[key])
db.close()
# 16.1 Pickle模块
# dbm 的局限就在于键和键值必须是字符串或者二进制。如果用其他类型数据,就得到错误了。这时候就可以用 pickle 模块了。该模块可以把几乎所有类型的对象翻译成字符串模式,以便存储在数据库中,然后用的时候还可以把字符串再翻译回来。
# In[26]:
import pickle
t = [1, 2, 3]
s = pickle.dumps(t)
t1 = pickle.loads(s)
t1
# 17.关于编写模块
# 一般情况你导入一个模块,模块只是定义了新的函数,但不会去主动运行自己内部的函数。
# if __name__ == '__main__':
# print(linecount('wc.py')) #函数测试用语句,作为模块导入时不希望被执行。
# name是一个内置变量,当程序开始运行的时候被设置。如果程序是作为脚本来运行的,name的值就是'main';这样的话,if条件满足,测试代码就会运行。而如果该代码被用作模块导入了,if条件不满足,测试的代码就不会运行了。
# 18.关于类
# 例:牌桌上面一共有52张扑克牌,每一张都属于四种花色之一,并且是十三张牌之一。花色为黑桃,红心,方块,梅花(在桥牌中按照降序排列)。排列顺序为 A,2,3,4,5,6,7,8,9,10,J,Q,K。根据具体玩的游戏的不同,A可以比K大,也可以比2还小。
# 一种思路是用整数来编码,以表示点数和花色。在这里,『编码』的意思就是我们要建立一个从数值到花色或者从数值到点数的映射。这种编码并不是为了安全的考虑(那种情况下用的词是『encryption(也是编码的意思,专用于安全领域)』)。
# 例如,下面这个表格就表示了花色与整数编码之间的映射关系:
# Spades ↦ 3
# Hearts ↦ 2
# Diamonds ↦ 1
# Clubs ↦ 0
# 这样的编码就比较易于比较牌的大小;因为高花色对应着大数值,我们对比一下编码大小就能比较花色顺序。牌面大小的映射就很明显了;每一张牌都对应着相应大小的整数,对于有人像的几张映射如下所示:
# Jack ↦ 11
# Queen ↦ 12
# King ↦ 13
# 我这里用箭头符号↦来表示映射关系,但这个符号并不是 Python 所支持的。这些符号是程序设计的一部分,但最终并不以这种形式出现在代码里。
# In[52]:
import random
class Card: #单张牌
def __init__(self, rank = 1, suit = 0): #默认最小方片Ace, rank输入范围(0-13),suit输入范围(0-3)
self.rank = rank
self.suit = suit
rank_names = [None,'Acs','2','3','4','5','6','7','8','9','10','Jack','Queen','King']
suit_names = ['Clubs', 'Diamonds', 'Hearts', 'Spades']
def __str__(self):
return '%s of %s'%(Card.rank_names[self.rank], Card.suit_names[self.suit])
def __lt__(self, other):
#print('execute __lt__')
t1 = (self.rank, self.suit)
t2 = (other.rank,other.suit)
return(t1<t2)
# if self.rank < other.rank:
# return True
# elif self.rank > other.rank:
# return False
# else:
# return True if self.suit < other.suit else False
class Deck: #整副牌
def __init__(self):
self.cards = []
for rank in range(1,14):
for suit in range(4):
card = Card(rank, suit)
self.cards.append(card)
def __str__(self):
r = []
for c in self.cards:
r.append(str(c))
return('\n'.join(r))
def pop_card(self):
return self.cards.pop()
def add_card(self, card):
self.cards.append(card)
def shuffle_cards(self):
random.shuffle(self.cards)
def sort_cards(self):
cards_len = len(self.cards)
r = []
c = Card()
for i in range(cards_len):
for j in range((i+1),cards_len):
if not self.cards[i]<self.cards[j]:
c = self.cards[i]
self.cards[i]=self.cards[j]
self.cards[j]=c
r.append(self.cards[i])
class Hand(Deck):
"""Hand类继承于父类Deck"""
def __init__(self, label='new hand'):
self.cards = []
self.label = label
def move_cards(self, deck, num):#拿牌 deck指定从哪副牌里,num拿几张牌
for i in range(num):
self.add_card(deck.pop_card())
#if '__name__' == '__main__':
# card1 = Card(13,2)
# print(card1)
# card2 = Card(1,3)
# print('%s<%s?:%s'%(card1, card2, card1<card2))
deck = Deck()
hand = Hand("whao's new hand")
deck.shuffle_cards()
hand.move_cards(deck,13) #从洗好的一套牌里依次抽出13张组成一手牌
print('shuffled cards list:\n',deck)
print('the hand cards list:',hand)
deck.sort_cards()
print('sorted cards list:\n',deck)
# In[54]:
def factorial(n):
return 1 if n==0 else n*factorial(n-1)
factorial(3)
# 19.关于列表推导
# In[ ]:
def capitalize_all(t):
return[s.capitalize() for s in t]
# In[55]:
def capitalize_all(t):
# res = []
# for s in t:
# res.append(s.capitalize())
# return res
return[s.capitalize() for s in t]
t = ['aB','BAAs','esA']
print(capitalize_all(t))
# In[57]:
def only_upper(t):
# res = []
# for s in t:
# if s.isupper():
# res.append(s)
# return res
return[s for s in t if s.isupper()]
t = ['s','A','c','D']
only_upper(t)
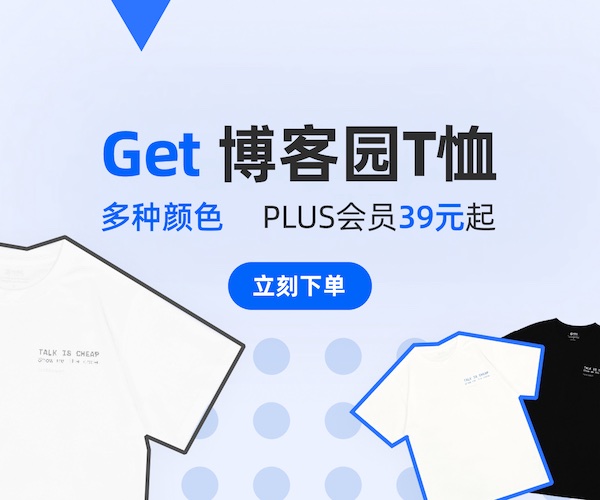