C#学习笔记(十二):构造函数、属性和静态类
面向对象
简写重载的方法:重载中如果逻辑重复的情况下,用参数少的调用参数多
参数空缺,可以用null填补
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace m1w3d3_attribute_inherit_visit { #region 属性 class Student { public Student(string name, int age, char sex) { this.name = name; this.age = age; this.sex = sex; } string name;//可以读,不能写 int age;//年龄不能小于10,不能大于150 char sex; //1、和方法大体一样,返回类型不能是void,没有参数列表 //如果你保护哪个字段,建议属性名用字段的帕斯卡命名法 //2、属性块中由两个块组成get,set //get块在被使用(取值)时是被调用 //set块在赋值时才会被调用 //set get块可以由空语句替代 //必须全部由空语句替代,或者没有set //get块和set块可以只有一个 //3、get块必须有返回值,值类型与属性返回类型一致 //4、在对应字段写入相应逻辑 public string Name { get { return " "; }//读 //set { }//写 } public int Age { get { return age; } set { //1、外部传入的值,在set块中用value表示 //age = value; //if (age < 0) age = 0; //else if (age > 150) age = 150; if (value < 0) value = 0; else if (value > 150) value = 150; age = value; } } } #endregion #region 属性的练习1 class Student1 { public Student1(string name, char sex, int age, float cSharp, float unity) { this.name = name; this.sex = sex; this.age = age; this.cSharp = cSharp; this.unity = unity; } //public string name; //public char sex; //public int age; //public float cSharp; //public float unity; string name; char sex; int age; float cSharp; float unity; public void SayHello() { Console.WriteLine("我叫{0},今年{1}岁了,是{2}生,我的CSharp成绩{3},我的Unity成绩{4}", Name, Age, Sex, CSharp, Unity); } //public void SaySorce(float cSharp, float unity) //{ // Console.WriteLine("我的CSharp成绩{0},我的Unity成绩{1}", unity, cSharp); // Console.WriteLine("我的总分是{0},我的平均分是{1}", (unity + cSharp), (unity + cSharp) / 2); //} public string Name { get { return name; } set { } } public int Age { get { if (age < 0) age = 0; else if (age > 150) age = 150; return age; } set { age = value; } } public float CSharp { get { if (cSharp <= 0) cSharp = 0; else if (cSharp >= 100) cSharp = 100; return cSharp; } set { cSharp = value; } } public float Unity { get { return unity; } set { unity = value; if (unity <= 0) unity = 0; else if (unity >= 100) unity = 100; } } public char Sex { get { if (sex == '男' || sex == '女') { } else { sex = '错'; } return sex; } set { sex = value; } } } #endregion #region 属性的练习2 class Ticket { float price;//写在这里,节约CPU运算 float distance; public Ticket() { } public Ticket(float distance) { //float price;//写在这里,节约内存空间 if (distance < 0) distance = 0; this.distance = distance; if (distance <= 100) price = distance; else if (distance <= 200) price = distance * 0.95f; else if (distance <= 300) price = distance * 0.9f; else price = distance * 0.8f; } public float Price { get { return price; } set { } } public float Distance { get { return distance; } } //在C#中,我们可以通过重写ToString(),去修改一个对象的打印信息 //ToString()必须要返回一个字符串 //Console.WriteLine()默认是用了 格式化字符串 //我们可以通过调用 格式化字符串方法 //String.Format(); public override string ToString() { return String.Format("这张票的票价是{0},距离{1}", Price, Distance); } } #endregion #region 属性的简写 //get set块 可以是空语句,set块可以没有 //这样的属性我们叫做自动属性,自动属性可以帮助我们快速实现一个自动属性 //自动属性有保护的字段 class Student2 { //public string name;//隐藏字段 public char sex; public int age; public float cSharp; public float unity; public string Name { get;//隐藏字段 set; } //public string Name => name;//只读属性的另一种写法 public void SayHello() { Console.WriteLine("我叫{0},今年{1}岁了,是{2}生,我的CSharp成绩{3},我的Unity成绩{4}", Name, age, sex, cSharp, unity); } } #endregion #region 静态类 static class Manager { public static int money; public static Random roll = new Random(); } class Fighter { string name; public Fighter(string name) { this.name = name; } int health; public void FindMoney() { int num = Manager.roll.Next(0, 100); if (num > 50) { num -= 50; Manager.money += num; Console.WriteLine("我是{0},我帮主人捡到了{1},我好开心!!", name, num); } else { Console.WriteLine("主人我没捡到钱,我的肉不好吃!!"); } } public void SayHello() { Console.WriteLine("主人,我是{0}", name); } } #endregion class Program { static void Main(string[] args) { #region 属性 Student xiaoMing = new Student("小明", 18, '男'); Console.WriteLine(xiaoMing.Name); xiaoMing.Age = 1000; Console.WriteLine(xiaoMing.Age); #endregion #region 属性的练习1 Student1 xiaoMing1 = new Student1("小明",'男', 18, 80, 70); xiaoMing1.SayHello(); Console.WriteLine(xiaoMing1.Age); Console.WriteLine(xiaoMing1.CSharp); Console.WriteLine(xiaoMing1.Unity); Console.WriteLine(xiaoMing1.Sex); Console.WriteLine(); Student1 xiaoMing2 = new Student1("小明", '大', 180, 500, 700); xiaoMing2.SayHello(); Console.WriteLine(xiaoMing2.Age); Console.WriteLine(xiaoMing2.CSharp); Console.WriteLine(xiaoMing2.Unity); Console.WriteLine(xiaoMing2.Sex); Console.WriteLine(); #endregion #region 属性的练习2 Ticket t = new Ticket(300); Console.WriteLine(t.Price); Console.WriteLine(t); #endregion #region 属性的简写 Student2 xiaoMing2 = new Student2(); xiaoMing2.SayHello(); #endregion #region 静态类 string ming = "赵钱孙李周吴郑王冯陈褚卫蒋沈韩杨"; Fighter[] monsters = new Fighter[10]; for (int i = 0; i < monsters.Length; i++) { monsters[i] = new Fighter("小" + ming[Manager.roll.Next(0, ming.Length)]); } foreach (var item in monsters) { item.FindMoney(); } Console.WriteLine(Manager.money); #endregion } } }
如何判定一个对象是被使用了?
结构体,在栈里读取数据
类,在栈里读取成员,在堆里读取数据(堆相当于内存条的大小)
结构体放在结构体里面,在栈里打开
结构体放在类里面,在堆里面打开
类,引用类型,直接储存的是地址,由CSharp的垃圾回收机制释放
结构体,值类型,直接存储的是数据
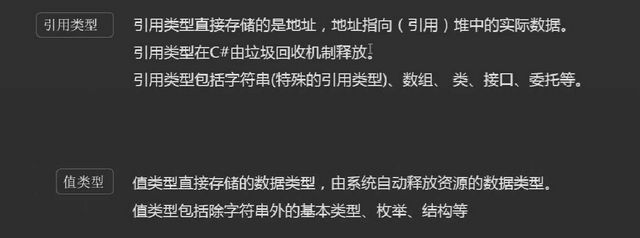
字符串,是特殊的引用类型,传参数时需要加ref
数组,在栈里存的是首元素的地址
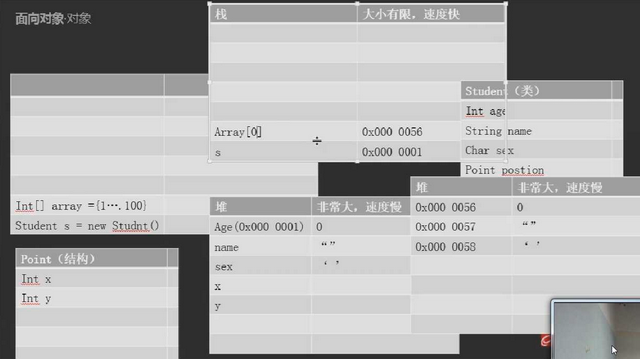
堆里存储的对象数据,没有栈里的地址来指向(直接、间接),数据就会被垃圾回收机制回收
类里引用的是对象,结构体引用的不叫对象
析构函数,类似C++
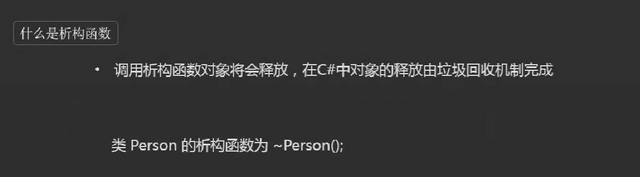
析构函数可以监视,不可调用
参照《C#公共语言运行时》
拓展练习:乘客随着载具移动,乘客放在数组里面
属性
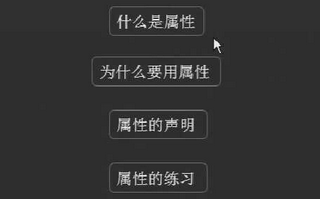
静态类
静态类占用静态空间
静态类,在程序开始时,会一直占用空间,直到程序结束时释放