2022.5.11 JavaBean
9、JavaBean
实体类 JavaBean有特定的写法:
-
必须要有一一个无参构造
-
属性必须私有化
-
必须有对应的get/set方法;
一般用来和数据库的字段做映射ORM;
ORM :对象关系映射
-
表-->类
-
字段-->属性
-
行记录--->对象
package com.xing.pojo;
//一个实体类对应数据库的一张表
public class Person {
private String name;
private int age;
private int id;
private String address;
public Person() {
}
public Person(String name, int age, int id, String address) {
this.name = name;
this.age = age;
this.id = id;
this.address = address;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
<%
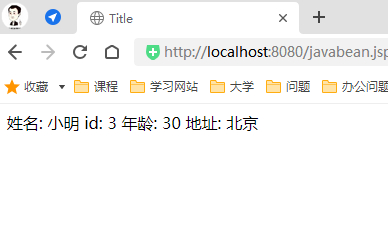