Python 协程、伪并发、yield、迭代器、for循环与__iter__方法
1.并发:指多个动作同时发生
#author: wylkjj
#date:2019/1/28
import time
def consumer(name):
print("%s 准备吃包子啦!" %name)
while True:
baozi = yield#接收send(i)传的值然后返回
print("包子[%s]来了,被[%s]吃了!"%(baozi,name))
def producer(name):
c=consumer('A')#yield对象,生成器对象
c2=consumer('B')#yield对象,生成器对象
c.__next__()#传值
c2.__next__()#传值
print("开始准备做包子啦!")
for i in range(1,10):
time.sleep(1)
print("做了2个包子!")
c.send(i)
c2.send(i)
producer("eric")
2.迭代器
(1)迭代器:生成器都是迭代器,迭代器不一定是生成器
list,tuple,dict,string:Iterable(可迭代对象)
l=[1,2,3,4,5]
d=iter(l) #l.__iter__()
print(d) #<list_iterator object at 0x000002D3D2F286A0>
(2)迭代器协议:
什么是迭代器?
满足两个条件:1.有iter方法;2.有next方法
(3)for循环内三件事:(while是没有的)
1.调用可迭代对象iter方法返回一个迭代器对象
2.不断调用迭代器对象的next方法
3.处理StopIteartion
for i in [1,2,3,4]:
iter([1,2,3,4])
(4)
from collections import Iterator
print(isinstance([1,2,3],list))#判断[1,2,3]是不是list类型
l=[1,2,3,4,5]
d=iter(l)
print(d)
print(isinstance(l,list))#是列表
print(isinstance(l,Iterator))#不是迭代器
print(isinstance(d,Iterator))#是迭代器
原文链接:https://blog.csdn.net/sinat_41672927/article/details/86681292
容器、可迭代对象、迭代器、生成器之间的关系:
协程:
yield实现生产者-消费者模型
# 消费者 def customer(): r = "" while True: n = yield r # 接受生产者的消息n,并且发送r print("customer 接受:", n) r = "ok" # 生产者 def producer(c): c.send(None) # 第一次返回None,不然会报错 for i in range(6): print("开始发送给消费者:", i) r = c.send(i) # 向消费者发送值 print("接受到消费者:", r) print("------------------------") c=customer() producer(c)
打印效果:
开始发送给消费者: 0
customer 接受: 0
接受到消费者: ok
------------------------
开始发送给消费者: 1
customer 接受: 1
接受到消费者: ok
------------------------
开始发送给消费者: 2
customer 接受: 2
接受到消费者: ok
------------------------
开始发送给消费者: 3
customer 接受: 3
接受到消费者: ok
------------------------
开始发送给消费者: 4
customer 接受: 4
接受到消费者: ok
------------------------
开始发送给消费者: 5
customer 接受: 5
接受到消费者: ok
------------------------

图解过程
- 这里的 n = yield r , 不只是发送数据r,还进行接受n
- 传统的生产者-消费者模型是一个线程写消息,一个线程取消息,通过锁机制控制队列和等待,但一不小心就可能死锁。
- 如果该用yield实现协程,生产者生产消息后,直接通过yield跳转到消费者接受执行,等待消费者消耗完毕后,生产者继续生成,提高了效率。
作者:时间煮菜
链接:https://www.jianshu.com/p/7747a268dfa8
来源:简书
著作权归作者所有。商业转载请联系作者获得授权,非商业转载请注明出处。
如果这篇文章帮助到了你,你可以请作者喝一杯咖啡
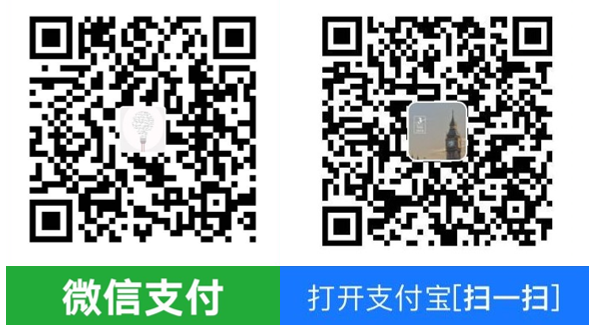