jQuery学习(二) jQuery选择器
jQuery选择器$()是jQuery的核心部分,他能够精确查找页面中的元素。
$()函数不需要像普通的JavaScript代码来遍历一组元素,它能够帮我们自动遍历,下面来看看jQuery常用的选择器。
- CSS选择器类型
- 标签形式:$('p'),这将获得页面中所有的p元素,可以在jQuery中这样写:$('p').addClass('.para'),这样就为整个页面的p元素都添加了样式类.para。
- CSS的ID选择器形式:$('#thisid'),将会获得id='thisid'的元素,同样可以通过addClass()方法为该元素添加样式规则。
- 类似CSS的类选择器形式:$('.content'),将会得到class="content"的所有元素(比如<div class="content"></div>)。
- XPath选择器类型
- XML 路径语言(XPath)是一种在 XML 文档中指定不同元素或者它们的值的语言,它与CSS 在 HTML 文档中指定元素的方法相类似。jQuery 库支持支持一套基本的 XPath 选择器,如果我们想的话,我们可以让它与 CSS 选择器一起工作。使用 jQuery,不管文档的类型如何,我们都可使用 XPath 和 CSS 选择器。
- • jQuery 使用 XPath 指定属性的约定,属性通过在方括号里用 @ 符号作为前缀指定,而不是用 CSS 的方法,它缺乏灵活性。例如,选择所有带有 title 属性的链接,我们会这样写:$('a[@title]')
- • XPath 语法允许方括号不使用 @ 的用法来指定一个元素。例如,我们可用下面的选择器表达式来得到所有包含至少一个 ol 元素的 div 元素:$('div[ol]')
3. 自定义选择器
对于各种的CSS和XPath选择器,jQuery加入了它自定义的选择器,大部分的自定义选择器能让我们在一个队列外选择一些元素。它的语法和CSS伪类语法类似(不知道Learning Jquery这本书为什么不把这种形式也归结到CSS类型选择器中),即选择器以冒号开头。例如我们想从一组匹配选择带有horziontal类的div中选择第二项,可以这样写:
$('div.horziontal:eq(1)'); //eq(1)是获得第二项
通过上述3种jQuery选择器,我们很容易就能找到页面中的元素,而且代码也不会长。这样就很利于我们操作页面元素,也节省了时间。
1
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
2
<html xmlns="http://www.w3.org/1999/xhtml">
3
<head>
4
<title></title>
5
<style type="text/css">
6
html, body
7
{
8
margin: 0;
9
padding: 0;
10
}
11
body
12
{
13
font-family: Arial, Verdana, sans-serif;
14
font-size: 12px;
15
color: #000;
16
background: #fff;
17
}
18
li
19
{
20
padding-top: 10px;
21
padding-left: 6px;
22
}
23
.horizontal
24
{
25
float: left;
26
list-style: none;
27
margin: 10px;
28
}
29
.sub-level
30
{
31
background: #ffc;
32
}
33
a
34
{
35
color: #00f;
36
}
37
a.mailto
38
{
39
color: #f00;
40
}
41
a.pdflink
42
{
43
color: #090;
44
}
45
a.mysite
46
{
47
text-decoration: none;
48
border-bottom: 1px dotted #00f;
49
}
50
.table-heading
51
{
52
background-color: #ff0;
53
}
54
th
55
{
56
text-align: left;
57
}
58
.odd
59
{
60
background-color: #ffc;
61
}
62
.even
63
{
64
background-color: #cef;
65
}
66
.highlight
67
{
68
color: #f00;
69
font-weight: bold;
70
}
71
td
72
{
73
color:#fff;
74
}
75
.table_header
76
{
77
background:red;
78
color:Blue;
79
font-size:14px;
80
}
81
.table_odd
82
{
83
background:#333;
84
}
85
.table_even
86
{
87
background:#666;
88
89
}
90
.high
91
{
92
color:Red;
93
font-weight:bold;
94
}
95
</style>
96
97
<script type="text/javascript" src="Scripts/jQuery.1.2.6.zh-cn-vsdoc.js"></script>
98
99
<script type="text/javascript">
100
$(document).ready(function() {
101
$('#selected-plays>li').addClass('horizontal'); //这里>号是jQuery的子选择符,#selected-plays>li 的意思就是id为selected-plays的直接子代(而二代以下不会算进来)
102
$('#selected-plays li:not(.horizontal)').addClass('sub-level'); //#selected-plays li给#selected-plays底下的所有li(包括二代,三代等)添加样式,但不包括li样式为horizontal的,在这里也就是为非直接子代添加样式,因为上面已经为直接子代添加了horizontal样式
103
104
});
105
$(document).ready(function(event) {
106
//^相当于正则表达式中的开始,$则相当于结尾
107
$('a[@href^="mailto:"]').addClass('mailto');//给a标签中包含href属性的,并且href以maito:开头的添加样式
108
$('a[@href$=".pdf"]').addClass('pdflink'); //给a标签中包含href属性的,并且href以.pdf结尾的添加样式
109
$('a[@href*="mysite"]').addClass('mysite'); //给a标签中包含href属性的,并且href中包含mysite的添加样式
110
});
111
$(document).ready(function() {
112
$('th').parent().addClass('table-heading');//包含th的tr添加样式
113
$('tr:not([th]):odd').addClass('table_odd');//冒号相当于CSS中的伪类给不包含th的tr的偶数行添加样式
114
$('tr:not([th]):even').addClass('table_even'); //给不包含th的tr的奇数行添加样式
115
$('td:contains("Henry")').siblings().addClass('high'); //给td中包含Henry字符的兄弟元素添加样式
116
});
117
</script>
118
119
</head>
120
<body>
121
<ul id="selected-plays">
122
<li>Comedies
123
<ul>
124
<li><a href="http://www.mysite.com/asyoulikeit/">As You Like It</a></li>
125
<li>All's Well That Ends Well</li>
126
<li>A Midsummer Night's Dream</li>
127
<li>Twelfth Night</li>
128
</ul>
129
</li>
130
<li>Tragedies
131
<ul>
132
<li><a href="hamlet.pdf">Hamlet</a></li>
133
<li>Macbeth</li>
134
<li>Romeo and Juliet</li>
135
</ul>
136
</li>
137
<li>Histories
138
<ul>
139
<li>Henry IV (<a href="mailto:henryiv@king.co.uk">email</a>)
140
<ul>
141
<li>Part I</li>
142
<li>Part II</li>
143
</ul>
144
<li><a href="http://www.shakespeare.co.uk/henryv.htm">Henry V</a></li>
145
<li>Richard II</li>
146
</ul>
147
</li>
148
</ul>
149
<table>
150
<tr>
151
<th>
152
Like
153
</th>
154
<th>
155
Name
156
</th>
157
</tr>
158
<tr>
159
<td>
160
All's Well that Ends Well
161
</td>
162
<td>
163
Comedy
164
</td>
165
</tr>
166
<tr>
167
<td>
168
Hamlet
169
</td>
170
<td>
171
Tragedy
172
</td>
173
</tr>
174
<tr>
175
<td>
176
Macbeth
177
</td>
178
<td>
179
Tragedy
180
</td>
181
</tr>
182
<tr>
183
<td>
184
Romeo and Juliet
185
</td>
186
<td>
187
Tragedy
188
</td>
189
</tr>
190
<tr>
191
<td>
192
Henry IV, Part I
193
</td>
194
<td>
195
History
196
</td>
197
</tr>
198
<tr>
199
<td>
200
Henry V
201
</td>
202
<td>
203
History
204
</td>
205
</tr>
206
</table>
207
</body>
208
</html>
209

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

53

54

55

56

57

58

59

60

61

62

63

64

65

66

67

68

69

70

71

72

73

74

75

76

77

78

79

80

81

82

83

84

85

86

87

88

89

90

91

92

93

94

95

96

97

98

99

100

101

102

103

104

105

106

107

108

109

110

111

112

113

114

115

116

117

118

119

120

121

122

123

124

125

126

127

128

129

130

131

132

133

134

135

136

137

138

139

140

141

142

143

144

145

146

147

148

149

150

151

152

153

154

155

156

157

158

159

160

161

162

163

164

165

166

167

168

169

170

171

172

173

174

175

176

177

178

179

180

181

182

183

184

185

186

187

188

189

190

191

192

193

194

195

196

197

198

199

200

201

202

203

204

205

206

207

208

209

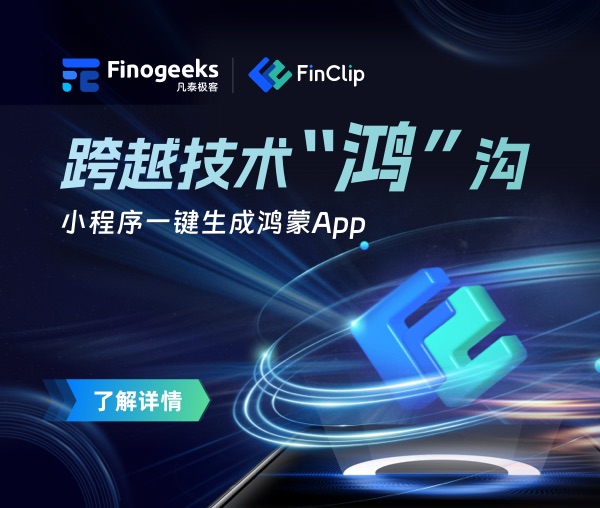