leetcode 797. All Paths From Source to Target
Given a directed, acyclic graph of N nodes. Find all possible paths from node 0 to node N-1, and return them in any order.
The graph is given as follows: the nodes are 0, 1, ..., graph.length - 1. graph[i] is a list of all nodes j for which the edge (i, j) exists.
Example:
Input: [[1,2], [3], [3], []]
Output: [[0,1,3],[0,2,3]]
Explanation: The graph looks like this:
0--->1
| |
v v
2--->3
There are two paths: 0 -> 1 -> 3 and 0 -> 2 -> 3.
思路:建树,然后深度优先遍历一遍就行了。
class Solution {
public:
vector<int> edge[20];
vector<vector<int> > ans;
int n;
void dfs(int u, vector<int>& t) {
t.push_back(u);
if (u == n-1) {
ans.push_back(t);
return ;
}
for (int i = 0; i < edge[u].size(); ++i) {
dfs(edge[u][i], t);
t.pop_back();
}
}
vector<vector<int>> allPathsSourceTarget(vector<vector<int>>& graph) {
n = graph.size();
for (int i = 0; i < graph.size(); ++i) {
for (int j = 0; j < graph[i].size(); ++j) {
edge[i].push_back(graph[i][j]);
}
}
vector<int> t;
dfs(0, t);
return ans;
}
};
原文地址:http://www.cnblogs.com/pk28/
与有肝胆人共事,从无字句处读书。
欢迎关注公众号:
欢迎关注公众号:
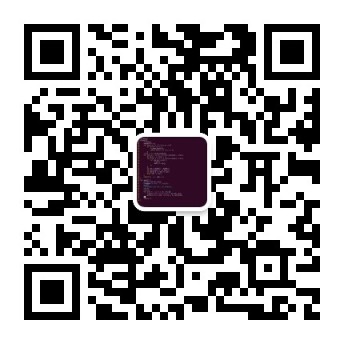