leetcode 334. Increasing Triplet Subsequence
Given an unsorted array return whether an increasing subsequence of length 3 exists or not in the array.
Formally the function should:
Return true if there exists i, j, k
such that arr[i] < arr[j] < arr[k] given 0 ≤ i < j < k ≤ n-1 else return false.
Your algorithm should run in O(n) time complexity and O(1) space complexity.
Examples:
Given [1, 2, 3, 4, 5],
return true.
Given [5, 4, 3, 2, 1],
return false.
思路:先在左边找一个最小值l(如果遇到小的就更新),如果当前值没用来更新l那么试着更新m,如果没更新m那么就存在一个符合条件的Triplet
class Solution {
public:
bool increasingTriplet(vector<int>& nums) {
if (nums.size() < 3) return false;
int l = nums[0];
int m = INT_MAX;
for (int i = 1; i < nums.size(); ++i) {
if (nums[i] <= l) l = nums[i];
else if (nums[i] < m) m = nums[i];
else if (nums[i] > m) return true;
}
return false;
}
};
原文地址:http://www.cnblogs.com/pk28/
与有肝胆人共事,从无字句处读书。
欢迎关注公众号:
欢迎关注公众号:
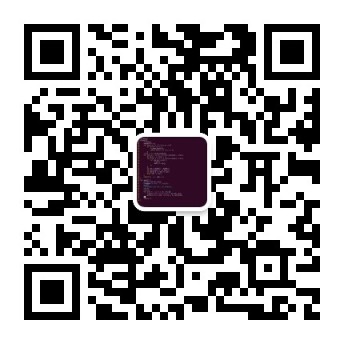