复合控件dll: using System; using System.Web.UI; using System.Web.UI.WebControls; using System.ComponentModel; namespace CustomControls { /// <summary> /// WebCustomControl1 的摘要说明。 /// </summary> [DefaultProperty("Text"), ToolboxData("<{0}:Composite runat=server></{0}:Composite>")] public class Composite : Control, INamingContainer //vs2010 只需继承CompositeControl 类 //标识在 Page 对象的控件层次结构内创建新 ID 命名空间的容器控件。这仅是一个标记接口。 { private int number = 100; private Label label; public int Number { get { return number; } set { number = value; } } private int Sum { get { EnsureChildControls(); return Int32.Parse(((TextBox)Controls[1]).Text) + Int32.Parse(((TextBox)Controls[4]).Text); } } public string Text { get { EnsureChildControls(); return label.Text; } set { EnsureChildControls(); label.Text = value; } } public event CheckEventHandler Check; protected virtual void OnCheck(CheckEventArgs ce) { if (Check != null) { Check(this,ce); } } protected override void CreateChildControls() { Controls.Add(new LiteralControl("<h3>第一个数字 : ")); TextBox box1 = new TextBox(); box1.Text = "0"; Controls.Add(box1); Controls.Add(new LiteralControl("</h3>")); Controls.Add(new LiteralControl("<h3>第二个数字 : ")); TextBox box2 = new TextBox(); box2.Text = "0"; Controls.Add(box2); Controls.Add(new LiteralControl("</h3>")); Button button1 = new Button(); button1.Text = "提交"; Controls.Add(new LiteralControl("<br>")); Controls.Add(button1); button1.Click += new EventHandler(this.ButtonClicked); Controls.Add(new LiteralControl("<br><br>")); label = new Label(); label.Height = 50; label.Width = 500; label.Text = "点击提交按钮看是否匹配"; Controls.Add(label); } protected override void OnPreRender(EventArgs e) { ((TextBox)Controls[1]).Text = "0"; ((TextBox)Controls[4]).Text = "0"; } private void ButtonClicked(Object sender, EventArgs e) { OnCheck(new CheckEventArgs(Sum - Number)); } } public class CheckEventArgs : EventArgs { private bool match = false; public CheckEventArgs (int difference) { if (difference == 0) { match = true; } } public bool Match { get { return match; } } } public delegate void CheckEventHandler(object sender, CheckEventArgs ce); } 引用页面: <%@ Register TagPrefix="cc1" Namespace="CustomControls" Assembly="Composite" %> <%@ Page language="c#" Codebehind="01UseComposite.aspx.cs" AutoEventWireup="false" Inherits="CompositeControl.WebForm1" %> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.0 Transitional//EN" > <HTML> <HEAD> <title>WebForm1</title> <meta name="GENERATOR" Content="Microsoft Visual Studio 7.0"> <meta name="CODE_LANGUAGE" Content="C#"> <meta name="vs_defaultClientScript" content="JavaScript"> <meta name="vs_targetSchema" content="http://schemas.microsoft.com/intellisense/ie5"> </HEAD> <body MS_POSITIONING="GridLayout"> <form id="Form1" method="post" runat="server"> <FONT face="宋体"> <cc1:Composite id="Composite1" OnCheck="Sum_Checked" Number="10" runat="server"></cc1:Composite></FONT> </form> </body> </HTML> 页面后台: using System; using System.Collections; using System.ComponentModel; using System.Data; using System.Drawing; using System.Web; using System.Web.SessionState; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.UI.HtmlControls; namespace CompositeControl { /// <summary> /// WebForm1 的摘要说明。 /// </summary> public class WebForm1 : System.Web.UI.Page { protected CustomControls.Composite Composite1; private void Page_Load(object sender, System.EventArgs e) { // 在此处放置用户代码以初始化页面 } public void Sum_Checked (Object sender,CustomControls.CheckEventArgs e) { if (e.Match == true) Composite1.Text = "<h2> 您输入的匹配!</h2>"; else Composite1.Text = "对不起,您输入的数字的和不等于内置数字!"; } #region Web Form Designer generated code override protected void OnInit(EventArgs e) { // // CODEGEN:该调用是 ASP.NET Web 窗体设计器所必需的。 // InitializeComponent(); base.OnInit(e); } /// <summary> /// 设计器支持所需的方法 - 不要使用代码编辑器修改 /// 此方法的内容。 /// </summary> private void InitializeComponent() { this.Load += new System.EventHandler(this.Page_Load); } #endregion } } //事件冒泡 using System; using System.Web.UI; using System.Web.UI.WebControls; using System.ComponentModel; namespace CompositeEvent { /// <summary> /// WebCustomControl1 的摘要说明。 /// </summary> [DefaultProperty("Text"), ToolboxData("<{0}:EventBubbler runat=server></{0}:EventBubbler>")] public class EventBubbler : Control, INamingContainer { private int number = 100; private Label label; private TextBox box1; private TextBox box2; public event EventHandler Click; public event EventHandler Reset; public event EventHandler Submit; public string Label { get { EnsureChildControls(); return label.Text; } set { EnsureChildControls(); label.Text = value; } } public int Number { get { return number; } set { number = value; } } public string Text1 { get { EnsureChildControls(); return box1.Text; } set { EnsureChildControls(); box1.Text = value; } } public string Text2 { get { EnsureChildControls(); return box2.Text; } set { EnsureChildControls(); box2.Text = value; } } protected override void CreateChildControls() { Controls.Add(new LiteralControl("<h3>第一个数字 : ")); box1 = new TextBox(); box1.Text = "0"; Controls.Add(box1); Controls.Add(new LiteralControl("</h3>")); Controls.Add(new LiteralControl("<h3>第二个数字: ")); box2 = new TextBox(); box2.Text = "0"; Controls.Add(box2); Controls.Add(new LiteralControl("</h3>")); Button button1 = new Button(); button1.Text = "点击"; button1.CommandName = "Click"; Controls.Add(button1); Button button2 = new Button(); button2.Text = "重置"; button2.CommandName = "Reset"; Controls.Add(button2); Button button3 = new Button(); button3.Text = "提交"; button3.CommandName = "Submit"; Controls.Add(button3); Controls.Add(new LiteralControl("<br><br>")); label = new Label(); label.Height = 50; label.Width = 500; label.Text = "单击一个按钮"; Controls.Add(label); } protected override bool OnBubbleEvent(object source, EventArgs e) { bool handled = false; if (e is CommandEventArgs) { CommandEventArgs ce = (CommandEventArgs)e; if (ce.CommandName == "Click") { OnClick(ce); handled = true; } else if (ce.CommandName == "Reset") { OnReset(ce); handled = true; } else if (ce.CommandName == "Submit") { OnSubmit(ce); handled = true; } } return handled; } protected virtual void OnClick (EventArgs e) { if (Click != null) { Click(this,e); } } protected virtual void OnReset (EventArgs e) { if (Reset != null) { Reset(this,e); } } protected virtual void OnSubmit (EventArgs e) { if (Submit != null) { Submit(this,e); } } } } 列子: using System; using System.Web.UI; using System.Web.UI.WebControls; using System.ComponentModel; using System.Xml; using System.Collections; namespace CompositeInput { /// <summary> /// WebCustomControl1 的摘要说明。 /// </summary> [DefaultProperty("Text"), ToolboxData("<{0}:ComInput runat=server></{0}:ComInput>")] public class ComInput : Control, INamingContainer //标识在 Page 对象的控件层次结构内创建新 ID 命名空间的容器控件。这仅是一个标记接口。 { private string strFileName = "bad_words.xml"; private Label label; public string FileName { get { return strFileName; } set { strFileName = value; } } //以用户提交的文本内容为输入参数。如果用户提交的内容包含攻击性言辞, //则返回修改后的版本, //否则,直接返回原始的文本。 public string CheckString(string InputString) { ArrayList alWordList = new ArrayList(); string xmlDocPath = this.Page.Server.MapPath("bad_words.xml"); XmlTextReader xmlReader = new XmlTextReader(xmlDocPath); string asterisks = "*************************"; // 将定义攻击性言辞的xml文件内容读入到一个ArrayList while (xmlReader.Read()) { if(xmlReader.NodeType==XmlNodeType.Text) alWordList.Add(xmlReader.Value); } xmlReader.Close(); //检查用户提交的文本内容,将攻击性言辞替换为适当数量的星号 foreach(string element in alWordList) InputString=InputString.Replace(element,asterisks.Substring(1, (element.Length))); return InputString ; } public string Text { get { EnsureChildControls(); return label.Text; } set { EnsureChildControls(); label.Text = value; } } public event CheckEventHandler Check; protected virtual void OnCheck(CheckEventArgs ce) { if (Check != null) { Check(this,ce); } } protected override void CreateChildControls() { Controls.Add(new LiteralControl("<h3>请在下面输入文字内容: ")); TextBox box1 = new TextBox(); box1.Text = ""; Controls.Add(box1); Controls.Add(new LiteralControl("</h3>")); Button button1 = new Button(); button1.Text = "提交"; Controls.Add(new LiteralControl("<br>")); Controls.Add(button1); button1.Click += new EventHandler(this.ButtonClicked); Controls.Add(new LiteralControl("<br><br>")); label = new Label(); label.Height = 50; label.Width = 500; label.Text = ""; Controls.Add(label); } protected override void OnPreRender(EventArgs e) { ((TextBox)Controls[1]).Text = ""; } private void ButtonClicked(Object sender, EventArgs e) { OnCheck(new CheckEventArgs(((TextBox)Controls[1]).Text,CheckString(((TextBox)Controls[1]).Text))); } } public class CheckEventArgs : EventArgs { private bool match = false; public CheckEventArgs (string str1,string str2) { if (str1 == str2) { match = true; } } public bool Match { get { return match; } } } public delegate void CheckEventHandler(object sender, CheckEventArgs ce); } using System; using System.Collections; using System.ComponentModel; using System.Data; using System.Drawing; using System.Web; using System.Web.SessionState; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.UI.HtmlControls; namespace CompositeControl { /// <summary> /// UseInput 的摘要说明。 /// </summary> public class UseInput : System.Web.UI.Page { protected CompositeInput.ComInput ComInput1; private void Page_Load(object sender, System.EventArgs e) { // 在此处放置用户代码以初始化页面 } public void CheckText(object sender,CompositeInput.CheckEventArgs e) { if(e.Match) ComInput1.Text = "你提交的内容已通过检查!"; else ComInput1.Text = "<h2>发布内容请遵守本站规则!不得发布攻击性言辞!</h2>"; } #region Web Form Designer generated code override protected void OnInit(EventArgs e) { // // CODEGEN:该调用是 ASP.NET Web 窗体设计器所必需的。 // InitializeComponent(); base.OnInit(e); } /// <summary> /// 设计器支持所需的方法 - 不要使用代码编辑器修改 /// 此方法的内容。 /// </summary> private void InitializeComponent() { this.Load += new System.EventHandler(this.Page_Load); } #endregion } }
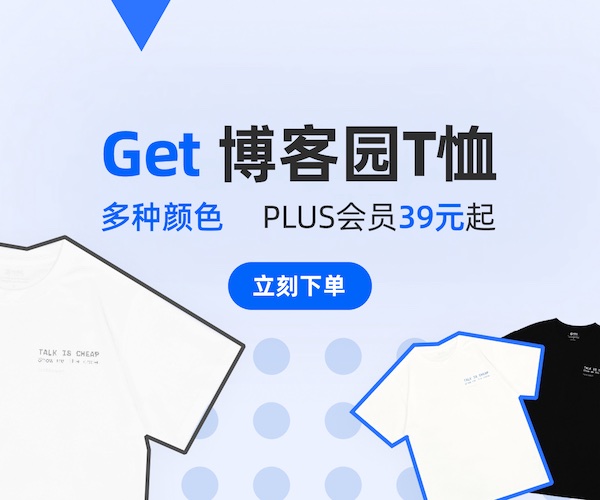