java 竞赛常用方法
1.int,float.double等转换为字符串用 String.valueOf方法
eg:double转换为String
Double dtDouble=12.31354; String string=String.valueOf(dtDouble); System.out.println(string);
String string="1.254849"; Double dt1=Double.parseDouble(string); System.out.println(dt1);
double d = 32.1415926; //0.00就是两位,0.000就是三位,依此类推 DecimalFormat decimalFormat=new DecimalFormat("0.00"); String d1=decimalFormat.format(d); System.out.println(d1);//32.14
如果直接使用double计算,可能会出现以下情况
double t=1.0-2.2*1.0; System.out.println(t);//-1.2000000000000002
所以double做精确计算时推荐使用BigDecimal
BigDecimal使用教程
import java.math.BigDecimal; public class testDecimal { public static void main(String[] args) { double d1=0.01; double d2=0.02; BigDecimal d1BigDec=new BigDecimal(Double.toString(d1)); BigDecimal d2BigDec=new BigDecimal(Double.toString(d2)); //加法 BigDecimal addB=d1BigDec.add(d2BigDec); Double add=addB.doubleValue(); System.out.println(add);//0.03 //减法 BigDecimal subB=d1BigDec.subtract(d2BigDec); Double sub=subB.doubleValue(); System.out.println(sub);//-0.01 //乘法 BigDecimal mulB=d1BigDec.multiply(d2BigDec); Double mul=mulB.doubleValue(); System.out.println(mul);//2.0E-4 //除法一 BigDecimal divB=d1BigDec.divide(d2BigDec); Double div=divB.doubleValue(); System.out.println(div);//0.5 //除法二(如果有除不尽的,会报异常,需要自己指定保留几位小数) //注意这个divide方法有两个重载的方法,一个是传两个参数的,一个是传三个参数的: //两个参数的方法: //@param divisor value by which this {@code BigDecimal} is to be divided. 传入除数 //@param roundingMode rounding mode to apply. 传入round的模式 //三个参数的方法: //@param divisor value by which this {@code BigDecimal} is to be divided. 传入除数 //@param scale scale of the {@code BigDecimal} quotient to be returned. 传入精度 //@param roundingMode rounding mode to apply. 传入round的模式 double dt1=2.0; double dt2=3.0; BigDecimal dt1BigDec=new BigDecimal(Double.toString(dt1)); BigDecimal dt2BigDec=new BigDecimal(Double.toString(dt2)); //保留六位小数 BigDecimal divideB = dt1BigDec.divide(dt2BigDec, 6, BigDecimal.ROUND_HALF_UP); double divide = divideB.doubleValue(); System.out.println(divide);//0.666667 } }
除并求余运算
import java.math.BigDecimal; public class Main { public static void main(String[] args) { BigDecimal amt = new BigDecimal(1001); BigDecimal[] results = amt.divideAndRemainder(new BigDecimal(50)); //商 20 System.out.println(results[0]); //余数1 System.out.println(results[1]); } }
1.数组自带的方法
2.操纵数组类Arrays(java.util.Arrays)
sort
升序
int scores[] = new int[]{1,2,3,89,4}; Arrays.sort(scores);
降序
-
Collections为java.util.Collections
-
不能使用基本类型(int,double, char),如果是int型需要改成Integer,float要改成Float
Integer scores[] = {1,2,3,89,4};
Arrays.sort(scores, Collections.reverseOrder());
自定义规则排序
-
Comparator为java.util.Comparator
Integer[] arr = {5,4,7,9,2,12,54,21,1}; //降序 Arrays.sort(arr, new Comparator<Integer>() { public int compare(Integer a, Integer b) { return b-a; } }); System.out.println(Arrays.toString(arr));
fill
作用:把数组所有元素都赋值为指定数
int [] scores={12,21,13,24}; Arrays.fill(scores,22);//将数组中所有元素都赋值为22
copyOfRange
作用:将数组指定的范围复制到一个新数组中
int [] scores1={12,21,13,24}; int[]scores= Arrays.copyOfRange(scores1,1,3); //21,13 for(int i=0;i<scores.length;i++){ System.out.println(scores[i]); }
作用:将数组内容转换称为字符串
int[] arr = {1,3,5,6}; String s = Arrays.toString(arr); //打印字符串,输出内容 System.out.println(s);//[1,3,5,6]
作用:将数组转换为集合
-
只有是引用类型的才能使用泛型
Integer[] a = {1,2,3,4};
List<Integer> list= Arrays.asList(a);
四,集合
1.集合自带的方法
2.操作集合的类Collections(java.util.Collections)
sort
自定义规则排序
package shuZu; import java.util.ArrayList; import java.util.Collections; import java.util.Comparator; import java.util.List; public class sort { public static void main(String[] args) { List<Student> stus = new ArrayList<>(); Student stu1 = new Student(); Student stu2 = new Student(); Student stu3 = new Student(); Student stu4 = new Student(); Student stu5 = new Student(); Student stu6 = new Student(); stu1.setAge(30); stu2.setAge(20); stu3.setAge(40); stu4.setAge(30); stu5.setAge(40); stu6.setAge(20); stu1.setNum(1); stu2.setNum(2); stu3.setNum(3); stu4.setNum(4); stu5.setNum(5); stu6.setNum(6); stu1.setName("张三"); stu2.setName("李四"); stu3.setName("王五"); stu4.setName("赵六"); stu5.setName("陈七"); stu6.setName("周八"); stus.add(stu1); stus.add(stu2); stus.add(stu3); stus.add(stu4); stus.add(stu5); stus.add(stu6); Collections.sort(stus, new Comparator<Student>() { @Override public int compare(Student s1, Student s2) { int flag; // 首选按年龄升序排序 flag = s1.getAge() - s2.getAge(); if (flag == 0) { // 再按学号升序排序 flag = s1.getNum() - s2.getNum(); } return flag; } }); System.out.println("年龄 学号 姓名 "); for (Student s : stus) { System.out.println(s.getAge() + " " + s.getNum() + " " + s.getName()); } } } class Student{ int age; int num; String name; public int getAge() { return age; } public void setAge(int age) { this.age = age; } public int getNum() { return num; } public void setNum(int num) { this.num = num; } public String getName() { return name; } public void setName(String name) { this.name = name; } }
//将tem的每位数字加入集合list,将list倒序,将list全添加到result集合里面 private void tc(int tem, List<Integer> result) { // TODO Auto-generated method stub List<Integer>list=new ArrayList<>(); while(tem!=0) { int t=tem%10; tem/=10; list.add(t); } Collections.reverse(list); result.addAll(list); }
输入
第一行 输入n个字符串
其余n行 :n个字符串
输出每个字符串从大到小出现次数
格式 出现次数 - 字符串
eg:
5 2 -1 -1 22 1 11 66 0 1 28 74 35 3 35 28 7 2 -1 -1 22
实现代码
import java.util.ArrayList; import java.util.Collections; import java.util.Comparator; import java.util.HashMap; import java.util.List; import java.util.Map; import java.util.Map.Entry; import java.util.Scanner; public class Main { public static void main(String[] args) { Map<String, Integer>map=new HashMap<>(); Scanner sca=new Scanner(System.in); int n=sca.nextInt(); sca.nextLine(); for(int i=0;i<n;i++) { String str=sca.nextLine(); int num=map.getOrDefault(str, 0)+1; map.put(str, num); } List<Map.Entry<String, Integer>>list=new ArrayList<>(); for(Map.Entry<String, Integer>mv:map.entrySet()) { list.add(mv); } Collections.sort(list,new Comparator<Map.Entry<String, Integer>>() { @Override public int compare(Entry<String, Integer> o1, Entry<String, Integer> o2) { // TODO Auto-generated method stub return o2.getValue()-o1.getValue(); } }); System.out.println(); for(int i=0;i<list.size();i++) { Map.Entry<String, Integer> mvEntry=list.get(i); String key=mvEntry.getKey(); Integer value=mvEntry.getValue(); System.out.println(value +" - "+ key); } } }
输出
2 - 2 -1 -1 22 1 - 1 28 74 35 1 - 1 11 66 0 1 - 3 35 28 7
import java.util.HashSet; import java.util.Iterator; import java.util.Set; public class Main { public static void main(String[] args) { //HashSet没有get方法,所以只能通过加强for循环或迭代器实现遍历 Set<Integer>set=new HashSet<>(); set.add(1); set.add(2); set.add(9); set.add(2); set.add(7); //加强for循环 for(Integer s:set) { System.out.println(s); } //迭代器 Iterator<Integer> itor=set.iterator(); while(itor.hasNext()) { System.out.println(itor.next()); } } }
五,字符串
toCharArray
String str="Hello"; char[] chars = str.toCharArray();
其它方法
创建实例:
String str = new String(); str = "String";
1、char charAt(int index):返回指定索引位置的字符
System.out.println(str.charAt(0)); //return "S";
System.out.println(str.substring(1)); //return "tring"
System.out.println(str.substring(1, 3)); //return "tr";
-
String的split方法支持正则表达式;
-
正则表达式
\s
表示匹配任何空白字符,+
表示匹配一次或多次。
String [] arr = str.split("\\s+"); for(String ss : arr){ System.out.println(ss); }
System.out.println(str.indexOf("i")); //return "3"; System.out.println(str.indexOf("ing")); //return "3";
System.out.println(str.indexOf("ing", 2)); //return "3";
System.out.println(str.replace("g", "gs"));
//return "Strings";
String str1 = new String(); str1 = " "+"string"+" "; System.out.println(str1.length()); //return "8" str1 = str1.trim(); System.out.println(str.length()); //return "6"
String str2 = new String(); str2 = "A/B/C"; String s[] = str2.split("/"); System.out.println("s[0] = "+s[0]); //return"A" for(String ss: s) { System.out.print(ss+" "); } //return"A B C"
String str2 = new String(); str2 = "A/B/C"; String s[] = str2.split("/", 2); for(String ss: s) { System.out.print(ss+" "); } //return"A B/C"
注意: . 、 | 和 * 等转义字符,必须得加 \
注意:多个分隔符,可以用 | 作为连字符
8、*String toLowerCase():转换为小写字母* *String toUpperCase():转换为大写字母*
9、boolean startsWith(String prefix):如果字符串以prefix开头返回true,否则返回false boolean endsWith(String suffix):如果字符串以suffix结尾返回true,否则返回false
10、boolean equals(Object other):如果字符串与other相等返回true,否则返回false boolean equalsIgnoreCase(String other):如果字符串与other相等(忽略大小写)返回true,否则返回false
字符串查找 indexOf
补充:字符判断方法
public class Main { public static void main(String[] args) { char c = 'a'; Boolean arr[]=new Boolean[6]; arr[0]=Character.isDigit(c);//判断字符是否数字 arr[1]=Character.isLetter(c);//判断字符是否字母 arr[2]=Character.isLetterOrDigit(c);//判断字符是否字母或数字 arr[3]=Character.isLowerCase(c);//判断字符是否小写字母 arr[4]=Character.isUpperCase(c);//判断字符是否大写字母 arr[5]=Character.isWhitespace(c);//判断字符是否空格[/size] for(int i=0;i<arr.length;i++) { System.out.println(arr[i]); } }
import java.util.LinkedList; import java.util.Queue; public class Main { public static void main(String[] args) { //add()和remove()方法在失败的时候会抛出异常(不推荐) Queue<String> queue = new LinkedList<String>(); //添加元素 queue.offer("a"); queue.offer("b"); queue.offer("c"); queue.offer("d"); queue.offer("e"); for(String q : queue){ System.out.println(q); } System.out.println("==="); System.out.println("poll="+queue.poll()); //返回第一个元素,并在队列中删除 for(String q : queue){ System.out.println(q); } System.out.println("==="); System.out.println("element="+queue.element()); //返回第一个元素 for(String q : queue){ System.out.println(q); } System.out.println("==="); System.out.println("peek="+queue.peek()); //返回第一个元素 for(String q : queue){ System.out.println(q); } }
Queue 中 element() 和 peek()都是用来返回队列的头元素,不删除。
在队列元素为空的情况下,element() 方法会抛出NoSuchElementException异常,peek() 方法只会返回 null
add()和offer()方法的区别是
区别:两者都是往队列尾部插入元素,不同的时候,当超出队列界限的时候,add()方法是抛出异常让你处理,而offer()方法是直接返回false
所以
添加元素推荐用offer(),返回并删除头元素用 poll(),只返回头元素用peek()
补充:优先级队列
以leecode题目连接所有点的最小费用为例
题目描述
给你一个points 数组,表示 2D 平面上的一些点,其中 points[i] = [xi, yi] 。
连接点 [xi, yi] 和点 [xj, yj] 的费用为它们之间的 曼哈顿距离 :|xi - xj| + |yi - yj| ,其中 |val| 表示 val 的绝对值。
请你返回将所有点连接的最小总费用。只有任意两点之间 有且仅有 一条简单路径时,才认为所有点都已连接。
来源:力扣(LeetCode) 链接:https://leetcode-cn.com/problems/min-cost-to-connect-all-points 著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
import java.util.Comparator; import java.util.PriorityQueue; import java.util.Queue; public class Solution { public Queue<EDGE> priorityQueue = new PriorityQueue<EDGE>(new Comparator<EDGE>() { @Override public int compare(EDGE o1, EDGE o2) { // TODO Auto-generated method stub return o1.quanZhi - o2.quanZhi; } }); public int getQuanZhi(int xi, int yi, int xj, int yj) { // |xi - xj| + |yi - yj| return Math.abs(xi - xj) + Math.abs(yi - yj); } public int vic[]; public int minCostConnectPoints(int[][] points) { vic = new int[points.length + 1]; // 以节点0为起点开始搜索 vic[0] = 1; int size = 1; int result = 0; // 将与节点0相连的节点加入优先级队列 for (int i = 1; i < points.length; i++) { priorityQueue.offer(new EDGE(0, i, getQuanZhi(points[0][0], points[0][1], points[i][0], points[i][1]))); } while (!priorityQueue.isEmpty()) { EDGE edge = priorityQueue.poll(); int end = edge.end; // 如果该节点以及访问过,则continue if (vic[end] == 1) { continue; } result += edge.quanZhi; // 节点个数加一 size++; if (size == points.length) { break; } vic[end] = 1; // 以该节点来将其周围节点加入进去 for (int i = 0; i < points.length; i++) { priorityQueue.offer(new EDGE(end, i, getQuanZhi(points[end][0], points[end][1], points[i][0], points[i][1]))); } } return result; } } class EDGE { public int start; public int end; public int quanZhi; public EDGE(int start, int end, int quanZhi) { this.start = start; this.end = end; this.quanZhi = quanZhi; } }
import java.util.Stack; public class Main { public static void main(String[] args) { Stack<Integer>stack=new Stack<>(); //入栈 stack.push(3); stack.push(5); stack.push(2); //出栈 while(!stack.isEmpty()) { Integer tInteger=stack.pop(); System.out.print(tInteger); }; } }
以蓝桥杯任意年月输出日历为例
题目描述
已知2007年1月1日为星期一。 设计一函数按照下述格式打印2007年以后(含)某年某月的日历,2007年以前的拒绝打印。 为完成此函数,设计必要的辅助函数可能也是必要的。其中输入为年分和月份。
注意:短线“-”个数要与题目中一致,否则系统会判为错误。
样例输入
2010 9
样例输出
---------------------
Su Mo Tu We Th Fr Sa
---------------------
1 2 3 4
5 6 7 8 9 10 11
12 13 14 15 16 17 18
19 20 21 22 23 24 25
26 27 28 29 30
---------------------
代码实现
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.util.Calendar; import java.util.GregorianCalendar; import java.util.StringTokenizer; public class Main { static BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(System.in)); static StringTokenizer stringTokenizer = new StringTokenizer(""); static String nextLine() throws IOException { return bufferedReader.readLine(); } static String next() throws IOException { while (!stringTokenizer.hasMoreTokens()) { stringTokenizer = new StringTokenizer(bufferedReader.readLine()); } return stringTokenizer.nextToken(); } static int nextInt() throws NumberFormatException, IOException { return Integer.parseInt(next()); } public static void main(String[] args) throws NumberFormatException, IOException { int yera = nextInt(); int mouth = nextInt(); //mouth是从0到11的 //创建日期对象,将年月日(1)放进去 GregorianCalendar gregorianCalendar = new GregorianCalendar(yera,mouth-1,1); //获取该月天数 int days=gregorianCalendar.getActualMaximum(Calendar.DAY_OF_MONTH); //获取该月第一天是星期几,需要减一 int xq=gregorianCalendar.get(Calendar.DAY_OF_WEEK)-1; System.out.println("---------------------"); System.out.println(" Su Mo Tu We Th Fr Sa"); System.out.println("---------------------"); //输入前面空格 int t=0; for(int i=0;i<xq;i++) { System.out.print(" "); t++; } for(int i=1;i<=days;i++) { System.out.printf("%3d", i); t++; if(t%7==0) { System.out.println(); } } if(t%7!=0) { System.out.println(); } System.out.println("---------------------"); } }
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.util.StringTokenizer; public class Main { static BufferedReader reader = new BufferedReader(new InputStreamReader(System.in)); static StringTokenizer tokenizer = new StringTokenizer(""); static String nextLine() throws IOException {// 读取下一行字符串 return reader.readLine(); } static String next() throws IOException {// 读取下一个字符串 while (!tokenizer.hasMoreTokens()) { tokenizer = new StringTokenizer(reader.readLine()); } return tokenizer.nextToken(); } static int nextInt() throws IOException {// 读取下一个int型数值 return Integer.parseInt(next()); } static double nextDouble() throws IOException {// 读取下一个double型数值 return Double.parseDouble(next()); } public static void main(String[] args) throws IOException { String str= next(); String str1= next(); String str2= next(); String str3= next(); String str4= next(); System.out.println(tokenizer.toString()); } }
本文来自博客园,作者:lzstar-A2,转载请注明原文链接:https://www.cnblogs.com/lzstar/p/15399235.html
作 者:lzstar-A2
出 处:https://www.cnblogs.com/lzstar/
关于作者:一名java转安全的在校大学生
版权声明:本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文链接。
特此声明:所有评论和私信都会在第一时间回复。也欢迎园子的大大们指正错误,共同进步。或者直接私信我
声援博主:如果您觉得文章对您有帮助,可以点击文章右下角【推荐】一下。您的鼓励是作者坚持原创和持续写作的最大动力!
欢迎大家关注安全学习交流群菜鸟联盟(IThonest),如果您觉得文章对您有很大的帮助,您可以考虑赏博主一杯可乐以资鼓励,您的肯定将是我最大的动力。thx.
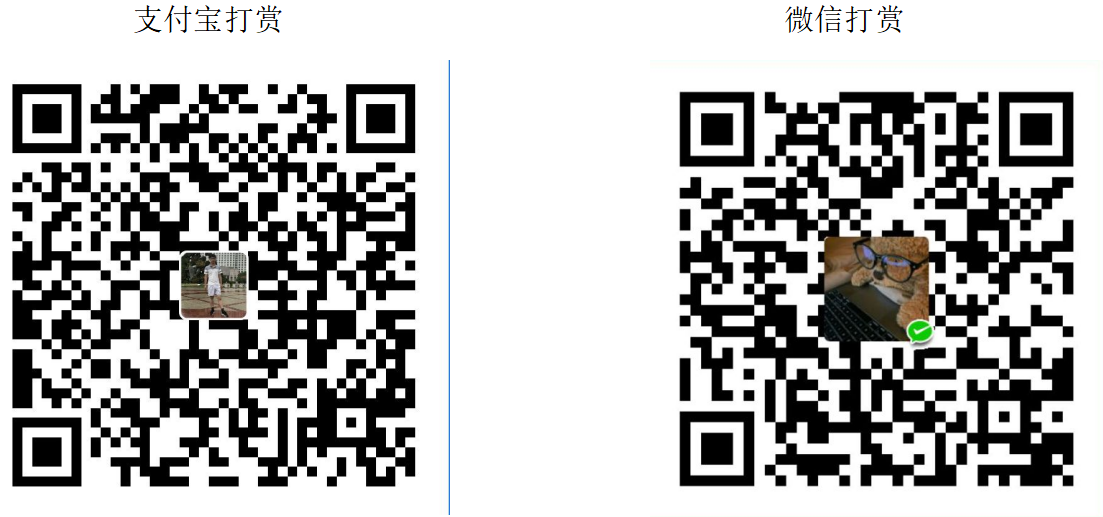
菜鸟联盟(IThonest),一个可能会有故事的qq群,欢迎大家来这里讨论,共同进步,不断学习才能不断进步。扫下面的二维码或者收藏下面的二维码关注吧(长按下面的二维码图片、并选择识别图中的二维码),个人QQ和微信的二维码也已给出,扫描下面👇的二维码一起来讨论吧!!!
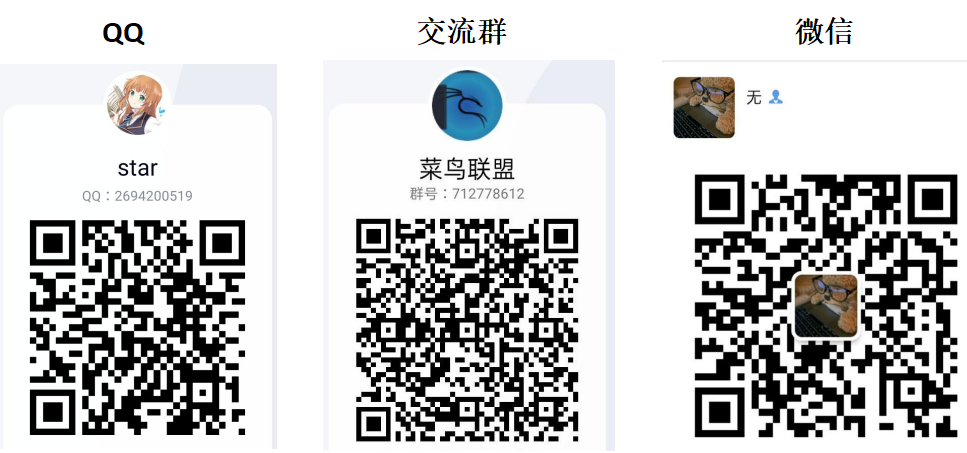