PHP常用算法整理
1.排序-冒泡
function mp($arr = []){
$len = count($arr);
if($len == 0) return [];
if($len == 1) return $arr;
//第一层控制冒泡轮数 $len-1轮
for($i=1;$i<$len;$i++){
//相邻两个比较,交换位置 每个for结束最大的都排到最后for循环-1 比较次数len-$i
for($j=0;$j<$len - $i;$j++){
if($arr[$j] > $arr[$j+1]){
$temp = $arr[$j];
$arr[$j] = $arr[$j+1];
$arr[$j+1] = $temp;
}
}
}
return $arr;
}
2.排序-快排
function quickly_order($arr=[]){
if(count($arr) == 0 || !is_array($arr)){
return [];
}
if(count($arr) == 1){
return $arr;
}
$mid = $arr[0];
$left = $right = [];
foreach($arr as $val){
if($val > $mid){
$right[] = $val;
}elseif($val < $mid){
$left[] = $val;
}
}
$left = quickly_order($left);
$right = quickly_order($right);
return array_merge($left,[$mid],$right);
}
3.usort()排序
function order_array($arr = []){
if(count($arr) == 0) return [];
usort($arr,function ($a,$b){
return $a == $b ? 0:($a>$b ? 1:-1);
});
return $arr;
}
4.二叉树遍历
建立如下二叉树
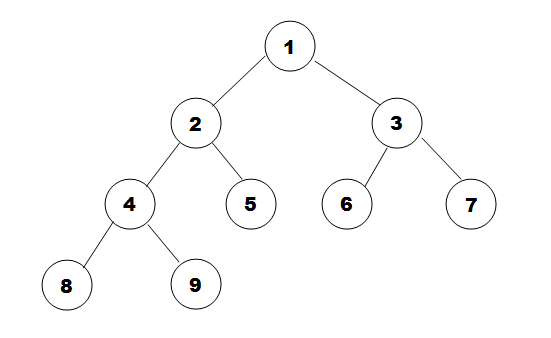
class Node{
public $data=null;
public $left=null;
public $right=null;
}
$node1 = new Node();
$node2 = new Node();
$node3 = new Node();
$node4 = new Node();
$node5 = new Node();
$node6 = new Node();
$node7 = new Node();
$node8 = new Node();
$node9 = new Node();
$node1->data = 1;
$node2->data = 2;
$node3->data = 3;
$node4->data = 4;
$node5->data = 5;
$node6->data = 6;
$node7->data = 7;
$node8->data = 8;
$node9->data = 9;
$node1->left = $node2;
$node1->right = $node3;
$node2->left = $node4;
$node2->right = $node5;
$node3->left = $node6;
$node3->right = $node7;
$node4->left = $node8;
$node4->right = $node9;
//深度优先
function binary_tree($node){
$return = []; //返回
$data = [];
array_push($data,$node); //入栈
while(count($data) > 0){
$_node = array_pop($data); //尾出
$return[] = $_node->data;
//先进后出 先把右边节点入栈
if(!empty($_node->right)){
array_push($data,$_node->right);
}
if(!empty($_node->left)){
array_push($data,$_node->left);
}
}
return $return;
}
echo "<pre>";
print_r(binary_tree($node1));
运行结果:
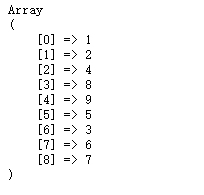
//广度优先
function binary_tree2($node){
$return = []; //返回
$data = [];
array_push($data,$node); //入栈
while(count($data) > 0){
$_node = array_shift($data); //首出
$return[] = $_node->data;
//先进先出 左先进
if(!empty($_node->left)){
array_push($data,$_node->left);
}
if(!empty($_node->right)){
array_push($data,$_node->right);
}
}
return $return;
}
echo "<pre>";
print_r(binary_tree2($node1));
运行结果:
5.全排列
function sort_full($arr,$str="",&$return=[]){
if(count($arr) == 0 || !is_array($arr)) return [];
if(count($arr) == 1){
$return[] = $str.$arr[0];
}else{
for($i=0;$i<count($arr);$i++){
$temp = $arr[0];
$arr[0] = $arr[$i];
$arr[$i] = $temp;
sort_full(array_slice($arr,1),$str.$arr[0],$return);
}
}
return $return;
}
echo "<pre>";
print_r(sort_full(['a','b','c','d']));
运行结果:
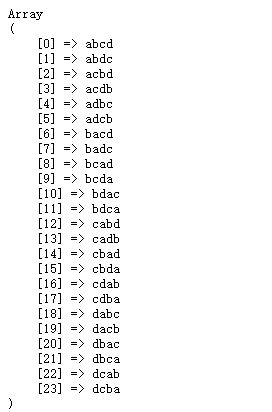