传智播客实战taotao项目页面菜单栏Tree的Java实现方法
1.controller查询方法
package com.taotao.manage.controller.api; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.http.HttpStatus; import org.springframework.http.ResponseEntity; import org.springframework.stereotype.Controller; import org.springframework.util.StringUtils; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import com.taotao.common.vo.ItemCatResult; import com.taotao.manage.service.ItemCatService; @Controller @RequestMapping("api/item/cat") public class ApiItemCatController { @Autowired private ItemCatService itemCatService; /** * lr 获取商品类目的树形结构 * @return */ @RequestMapping(method = RequestMethod.GET) public ResponseEntity<ItemCatResult> queryItemCatList(){ try { ItemCatResult itemCatResult = this.itemCatService.queryAllToTree(); if(StringUtils.isEmpty(itemCatResult)) { return ResponseEntity.status(HttpStatus.NOT_FOUND).body(null); } return ResponseEntity.ok(itemCatResult); } catch (Exception e) { e.printStackTrace(); } return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body(null); } }
2.service实现方法
package com.taotao.manage.service; import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map; import org.springframework.stereotype.Service; import com.taotao.common.vo.EasyUIResult; import com.taotao.common.vo.ItemCatData; import com.taotao.common.vo.ItemCatResult; import com.taotao.manage.pojo.ItemCat; @Service public class ItemCatService extends BaseService<ItemCat> { /** * 全部查询,并且生成树状结构 * * @return */ public ItemCatResult queryAllToTree() { ItemCatResult result = new ItemCatResult(); // 全部查出,并且在内存中生成树形结构 List<ItemCat> cats = super.queryAll(); // 转为map存储,key为父节点ID,value为数据集合 Map<Long, List<ItemCat>> itemCatMap = new HashMap<Long, List<ItemCat>>(); for (ItemCat itemCat : cats) { if (!itemCatMap.containsKey(itemCat.getParentId())) { itemCatMap.put(itemCat.getParentId(), new ArrayList<ItemCat>()); } itemCatMap.get(itemCat.getParentId()).add(itemCat); } // 封装一级对象 List<ItemCat> itemCatList1 = itemCatMap.get(0L); for (ItemCat itemCat : itemCatList1) { ItemCatData itemCatData = new ItemCatData(); itemCatData.setUrl("/products/" + itemCat.getId() + ".html"); itemCatData.setName("<a href='" + itemCatData.getUrl() + "'>" + itemCat.getName() + "</a>"); result.getItemCats().add(itemCatData); if (!itemCat.getIsParent()) { continue; } // 封装二级对象 List<ItemCat> itemCatList2 = itemCatMap.get(itemCat.getId()); List<ItemCatData> itemCatData2 = new ArrayList<ItemCatData>(); itemCatData.setItems(itemCatData2); for (ItemCat itemCat2 : itemCatList2) { ItemCatData id2 = new ItemCatData(); id2.setName(itemCat2.getName()); id2.setUrl("/products/" + itemCat2.getId() + ".html"); itemCatData2.add(id2); if (itemCat2.getIsParent()) { // 封装三级对象 List<ItemCat> itemCatList3 = itemCatMap.get(itemCat2.getId()); List<String> itemCatData3 = new ArrayList<String>(); id2.setItems(itemCatData3); for (ItemCat itemCat3 : itemCatList3) { itemCatData3.add("/products/" + itemCat3.getId() + ".html|" + itemCat3.getName()); } } } if (result.getItemCats().size() >= 14) { break; } } return result; } }
3.实体类vo
ItemCatResult.java
package com.taotao.common.vo; import java.util.ArrayList; import java.util.List; import com.fasterxml.jackson.annotation.JsonProperty; public class ItemCatResult { @JsonProperty("data") private List<ItemCatData> itemCats = new ArrayList<ItemCatData>(); public List<ItemCatData> getItemCats() { return itemCats; } public void setItemCats(List<ItemCatData> itemCats) { this.itemCats = itemCats; } }
ItemCatData.java
package com.taotao.common.vo; import java.util.List; import com.fasterxml.jackson.annotation.JsonProperty; public class ItemCatData { @JsonProperty("u") // 序列化成json数据时为 u private String url; @JsonProperty("n") private String name; @JsonProperty("i") private List<?> items; public String getUrl() { return url; } public void setUrl(String url) { this.url = url; } public String getName() { return name; } public void setName(String name) { this.name = name; } public List<?> getItems() { return items; } public void setItems(List<?> items) { this.items = items; } }
4.需要导入的包
<!-- Jackson Json处理工具包 --> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-web</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>servlet-api</artifactId> <scope>provided</scope> </dependency> <!-- Apache工具组件 --> <dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-lang3</artifactId> </dependency> <dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-io</artifactId> </dependency>
5.自定义的spring的消息转换器
<!-- 注解驱动 --> <mvc:annotation-driven> <mvc:message-converters> <bean class="com.taotao.common.spring.exetend.converter.json.CallbackMappingJackson2HttpMessageConverter"> <property name="callbackName" value="callback"></property> </bean> </mvc:message-converters> </mvc:annotation-driven>
6.CallbackMappingJackson2HttpMessageConverter.java 自定义方法实现
package com.taotao.common.spring.exetend.converter.json; import java.io.IOException; import javax.servlet.http.HttpServletRequest; import org.apache.commons.io.IOUtils; import org.apache.commons.lang3.StringUtils; import org.springframework.http.HttpOutputMessage; import org.springframework.http.converter.HttpMessageNotWritableException; import org.springframework.http.converter.json.MappingJackson2HttpMessageConverter; import org.springframework.web.context.request.RequestContextHolder; import org.springframework.web.context.request.ServletRequestAttributes; import com.fasterxml.jackson.core.JsonEncoding; import com.fasterxml.jackson.core.JsonProcessingException; public class CallbackMappingJackson2HttpMessageConverter extends MappingJackson2HttpMessageConverter { // 做jsonp的支持的标识,在请求参数中加该参数 private String callbackName; @Override protected void writeInternal(Object object, HttpOutputMessage outputMessage) throws IOException, HttpMessageNotWritableException { // 从threadLocal中获取当前的Request对象 HttpServletRequest request = ((ServletRequestAttributes) RequestContextHolder.currentRequestAttributes()).getRequest(); String callbackParam = request.getParameter(callbackName); if(StringUtils.isEmpty(callbackParam)){ // 没有找到callback参数,直接返回json数据 super.writeInternal(object, outputMessage); }else{ JsonEncoding encoding = getJsonEncoding(outputMessage.getHeaders().getContentType()); try { String result =callbackParam+"("+super.getObjectMapper().writeValueAsString(object)+");"; IOUtils.write(result, outputMessage.getBody(),encoding.getJavaName()); } catch (JsonProcessingException ex) { throw new HttpMessageNotWritableException("Could not write JSON: " + ex.getMessage(), ex); } } } public String getCallbackName() { return callbackName; } public void setCallbackName(String callbackName) { this.callbackName = callbackName; } }
最后能获取数据的接口如下:
http://manage.taotao.com/rest/api/item/cat
获取的数据如下:

{"data":[{"u":"/products/1.html","n":"<a href='/products/1.html'>图书、音像、电子书刊</a>","i":[{"u":"/products/2.html","n":"电子书刊","i":["/products/3.html|电子书","/products/4.html|网络原创","/products/5.html|数字杂志","/products/6.html|多媒体图书"]},{"u":"/products/7.html","n":"音像","i":["/products/8.html|音乐","/products/9.html|影视","/products/10.html|教育音像"]},{"u":"/products/11.html","n":"英文原版","i":["/products/12.html|少儿","/products/13.html|商务投资","/products/14.html|英语学习与考试","/products/15.html|小说","/products/16.html|传记","/products/17.html|励志"]},{"u":"/products/18.html","n":"文艺","i":["/products/19.html|小说","/products/20.html|文学","/products/21.html|青春文学","/products/22.html|传记","/products/23.html|艺术"]},{"u":"/products/24.html","n":"少儿","i":["/products/25.html|少儿","/products/26.html|0-2岁","/products/27.html|3-6岁","/products/28.html|7-10岁","/products/29.html|11-14岁"]},{"u":"/products/30.html","n":"人文社科","i":["/products/31.html|历史","/products/32.html|哲学","/products/33.html|国学","/products/34.html|政治/军事","/products/35.html|法律","/products/36.html|宗教","/products/37.html|心理学","/products/38.html|文化","/products/39.html|社会科学"]},{"u":"/products/40.html","n":"经管励志","i":["/products/41.html|经济","/products/42.html|金融与投资","/products/43.html|管理","/products/44.html|励志与成功"]},{"u":"/products/45.html","n":"生活","i":["/products/46.html|生活","/products/47.html|健身与保健","/products/48.html|家庭与育儿","/products/49.html|旅游","/products/50.html|动漫/幽默"]},{"u":"/products/51.html","n":"科技","i":["/products/52.html|科技","/products/53.html|工程","/products/54.html|建筑","/products/55.html|医学","/products/56.html|科学与自然","/products/57.html|计算机与互联网","/products/58.html|体育/运动"]},{"u":"/products/59.html","n":"教育","i":["/products/60.html|教材教辅","/products/61.html|教育与考试","/products/62.html|外语学习","/products/63.html|新闻出版","/products/64.html|语言文字"]},{"u":"/products/65.html","n":"港台图书","i":["/products/66.html|艺术/设计/收藏","/products/67.html|经济管理","/products/68.html|文化/学术","/products/69.html|少儿文学/国学"]},{"u":"/products/70.html","n":"其它","i":["/products/71.html|工具书","/products/72.html|影印版","/products/73.html|套装书"]}]},{"u":"/products/74.html","n":"<a href='/products/74.html'>家用电器</a>","i":[{"u":"/products/75.html","n":"大 家 电","i":["/products/76.html|平板电视","/products/77.html|空调","/products/78.html|冰箱","/products/79.html|洗衣机","/products/80.html|家庭影院","/products/81.html|DVD播放机","/products/82.html|迷你音响","/products/83.html|烟机/灶具","/products/84.html|热水器","/products/85.html|消毒柜/洗碗机","/products/86.html|酒柜/冰吧/冷柜","/products/87.html|家电配件","/products/88.html|家电下乡"]},{"u":"/products/89.html","n":"生活电器","i":["/products/90.html|电风扇","/products/91.html|冷风扇","/products/92.html|净化器","/products/93.html|饮水机","/products/94.html|净水设备","/products/95.html|挂烫机/熨斗","/products/96.html|吸尘器","/products/97.html|电话机","/products/98.html|插座","/products/99.html|收录/音机","/products/100.html|清洁机","/products/101.html|加湿器","/products/102.html|除湿机","/products/103.html|取暖电器","/products/104.html|其它生活电器","/products/105.html|扫地机器人","/products/106.html|干衣机","/products/107.html|生活电器配件"]},{"u":"/products/108.html","n":"厨房电器","i":["/products/109.html|料理/榨汁机","/products/110.html|豆浆机","/products/111.html|电饭煲","/products/112.html|电压力锅","/products/113.html|面包机","/products/114.html|咖啡机","/products/115.html|微波炉","/products/116.html|电烤箱","/products/117.html|电磁炉","/products/118.html|电饼铛/烧烤盘","/products/119.html|煮蛋器","/products/120.html|酸奶机","/products/121.html|电炖锅","/products/122.html|电水壶/热水瓶","/products/123.html|多用途锅","/products/124.html|果蔬解毒机","/products/125.html|其它厨房电器"]},{"u":"/products/126.html","n":"个护健康","i":["/products/127.html|剃须刀","/products/128.html|剃/脱毛器","/products/129.html|口腔护理","/products/130.html|电吹风","/products/131.html|美容器","/products/132.html|美发器","/products/133.html|按摩椅","/products/134.html|按摩器","/products/135.html|足浴盆","/products/136.html|血压计","/products/137.html|健康秤/厨房秤","/products/138.html|血糖仪","/products/139.html|体温计","/products/140.html|计步器/脂肪检测仪","/products/141.html|其它健康电器"]},{"u":"/products/142.html","n":"五金家装","i":["/products/143.html|电动工具","/products/144.html|手动工具","/products/145.html|仪器仪表","/products/146.html|浴霸/排气扇","/products/147.html|灯具","/products/148.html|LED灯","/products/149.html|洁身器","/products/150.html|水槽","/products/151.html|龙头","/products/152.html|淋浴花洒","/products/153.html|厨卫五金","/products/154.html|家具五金","/products/155.html|门铃","/products/156.html|电气开关","/products/157.html|插座","/products/158.html|电工电料","/products/159.html|监控安防","/products/160.html|电线/线缆"]}]},{"u":"/products/161.html","n":"<a href='/products/161.html'>电脑、办公</a>","i":[{"u":"/products/162.html","n":"电脑整机","i":["/products/163.html|笔记本","/products/164.html|超极本","/products/165.html|游戏本","/products/166.html|平板电脑","/products/167.html|平板电脑配件","/products/168.html|台式机","/products/169.html|服务器/工作站","/products/170.html|笔记本配件"]},{"u":"/products/171.html","n":"电脑配件","i":["/products/172.html|CPU","/products/173.html|主板","/products/174.html|显卡","/products/175.html|硬盘","/products/176.html|SSD固态硬盘","/products/177.html|内存","/products/178.html|机箱","/products/179.html|电源","/products/180.html|显示器","/products/181.html|刻录机/光驱","/products/182.html|散热器","/products/183.html|声卡/扩展卡","/products/184.html|装机配件","/products/185.html|组装电脑"]},{"u":"/products/186.html","n":"外设产品","i":["/products/187.html|移动硬盘","/products/188.html|U盘","/products/189.html|鼠标","/products/190.html|键盘","/products/191.html|鼠标垫","/products/192.html|摄像头","/products/193.html|手写板","/products/194.html|外置盒","/products/195.html|插座","/products/196.html|线缆","/products/197.html|UPS电源","/products/198.html|电脑工具","/products/199.html|游戏设备","/products/200.html|电玩","/products/201.html|电脑清洁"]},{"u":"/products/202.html","n":"网络产品","i":["/products/203.html|路由器","/products/204.html|网卡","/products/205.html|交换机","/products/206.html|网络存储","/products/207.html|4G/3G上网","/products/208.html|网络盒子","/products/209.html|网络配件"]},{"u":"/products/210.html","n":"办公设备","i":["/products/211.html|投影机","/products/212.html|投影配件","/products/213.html|多功能一体机","/products/214.html|打印机","/products/215.html|传真设备","/products/216.html|验钞/点钞机","/products/217.html|扫描设备","/products/218.html|复合机","/products/219.html|碎纸机","/products/220.html|考勤机","/products/221.html|墨粉","/products/222.html|收款/POS机","/products/223.html|会议音频视频","/products/224.html|保险柜","/products/225.html|装订/封装机","/products/226.html|安防监控","/products/227.html|办公家具","/products/228.html|白板"]},{"u":"/products/229.html","n":"文具/耗材","i":["/products/230.html|硒鼓/墨粉","/products/231.html|墨盒","/products/232.html|色带","/products/233.html|纸类","/products/234.html|办公文具","/products/235.html|学生文具","/products/236.html|文件管理","/products/237.html|财会用品","/products/238.html|本册/便签","/products/239.html|计算器","/products/240.html|激光笔","/products/241.html|笔类","/products/242.html|画具画材","/products/243.html|刻录碟片/附件"]},{"u":"/products/244.html","n":"服务产品","i":["/products/245.html|上门服务","/products/246.html|远程服务","/products/247.html|电脑软件","/products/248.html|京东服务"]}]},{"u":"/products/249.html","n":"<a href='/products/249.html'>个护化妆</a>","i":[{"u":"/products/250.html","n":"面部护肤","i":["/products/251.html|清洁","/products/252.html|护肤","/products/253.html|面膜","/products/254.html|剃须","/products/255.html|套装"]},{"u":"/products/256.html","n":"身体护肤","i":["/products/257.html|沐浴","/products/258.html|润肤","/products/259.html|颈部","/products/260.html|手足","/products/261.html|纤体塑形","/products/262.html|美胸","/products/263.html|套装"]},{"u":"/products/264.html","n":"口腔护理","i":["/products/265.html|牙膏/牙粉","/products/266.html|牙刷/牙线","/products/267.html|漱口水","/products/268.html|套装"]},{"u":"/products/269.html","n":"女性护理","i":["/products/270.html|卫生巾","/products/271.html|卫生护垫","/products/272.html|私密护理","/products/273.html|脱毛膏"]},{"u":"/products/274.html","n":"洗发护发","i":["/products/275.html|洗发","/products/276.html|护发","/products/277.html|染发","/products/278.html|造型","/products/279.html|假发","/products/280.html|套装"]},{"u":"/products/281.html","n":"香水彩妆","i":["/products/282.html|香水","/products/283.html|底妆","/products/284.html|腮红","/products/285.html|眼部","/products/286.html|唇部","/products/287.html|美甲","/products/288.html|美容工具","/products/289.html|套装"]}]},{"u":"/products/290.html","n":"<a href='/products/290.html'>钟表</a>","i":[{"u":"/products/291.html","n":"钟表","i":["/products/292.html|男表","/products/293.html|女表","/products/294.html|儿童手表","/products/295.html|座钟挂钟"]}]},{"u":"/products/296.html","n":"<a href='/products/296.html'>母婴</a>","i":[{"u":"/products/297.html","n":"奶粉","i":["/products/298.html|婴幼奶粉","/products/299.html|成人奶粉"]},{"u":"/products/300.html","n":"营养辅食","i":["/products/301.html|益生菌/初乳","/products/302.html|米粉/菜粉","/products/303.html|果泥/果汁","/products/304.html|DHA","/products/305.html|宝宝零食","/products/306.html|钙铁锌/维生素","/products/307.html|清火/开胃","/products/308.html|面条/粥"]},{"u":"/products/309.html","n":"尿裤湿巾","i":["/products/310.html|婴儿尿裤","/products/311.html|拉拉裤","/products/312.html|湿巾","/products/313.html|成人尿裤"]},{"u":"/products/314.html","n":"喂养用品","i":["/products/315.html|奶瓶奶嘴","/products/316.html|吸奶器","/products/317.html|暖奶消毒","/products/318.html|碗盘叉勺","/products/319.html|水壶/水杯","/products/320.html|牙胶安抚","/products/321.html|辅食料理机"]},{"u":"/products/322.html","n":"洗护用品","i":["/products/323.html|宝宝护肤","/products/324.html|宝宝洗浴","/products/325.html|奶瓶清洗","/products/326.html|驱蚊防蚊","/products/327.html|理发器","/products/328.html|洗衣液/皂","/products/329.html|日常护理","/products/330.html|座便器"]},{"u":"/products/331.html","n":"童车童床","i":["/products/332.html|婴儿推车","/products/333.html|餐椅摇椅","/products/334.html|婴儿床","/products/335.html|学步车","/products/336.html|三轮车","/products/337.html|自行车","/products/338.html|电动车","/products/339.html|扭扭车","/products/340.html|滑板车"]},{"u":"/products/341.html","n":"寝居服饰","i":["/products/342.html|婴儿外出服","/products/343.html|婴儿内衣","/products/344.html|婴儿礼盒","/products/345.html|婴儿鞋帽袜","/products/346.html|安全防护","/products/347.html|家居床品"]},{"u":"/products/348.html","n":"妈妈专区","i":["/products/349.html|妈咪包/背婴带","/products/350.html|产后塑身","/products/351.html|文胸/内裤","/products/352.html|防辐射服","/products/353.html|孕妇装","/products/354.html|孕期营养","/products/355.html|孕妈美容","/products/356.html|待产/新生","/products/357.html|月子装"]},{"u":"/products/358.html","n":"童装童鞋","i":["/products/359.html|套装","/products/360.html|上衣","/products/361.html|裤子","/products/362.html|裙子","/products/363.html|内衣/家居服","/products/364.html|羽绒服/棉服","/products/365.html|亲子装","/products/366.html|儿童配饰","/products/367.html|礼服/演出服","/products/368.html|运动鞋","/products/369.html|皮鞋/帆布鞋","/products/370.html|靴子","/products/371.html|凉鞋","/products/372.html|功能鞋","/products/373.html|户外/运动服"]},{"u":"/products/374.html","n":"安全座椅","i":["/products/375.html|提篮式","/products/376.html|安全座椅","/products/377.html|增高垫"]}]},{"u":"/products/378.html","n":"<a href='/products/378.html'>食品饮料、保健食品</a>","i":[{"u":"/products/379.html","n":"进口食品","i":["/products/380.html|饼干蛋糕","/products/381.html|糖果/巧克力","/products/382.html|休闲零食","/products/383.html|冲调饮品","/products/384.html|粮油调味","/products/385.html|牛奶"]},{"u":"/products/386.html","n":"地方特产","i":["/products/387.html|其他特产","/products/388.html|新疆","/products/389.html|北京","/products/390.html|山西","/products/391.html|内蒙古","/products/392.html|福建","/products/393.html|湖南","/products/394.html|四川","/products/395.html|云南","/products/396.html|东北"]},{"u":"/products/397.html","n":"休闲食品","i":["/products/398.html|休闲零食","/products/399.html|坚果炒货","/products/400.html|肉干肉脯","/products/401.html|蜜饯果干","/products/402.html|糖果/巧克力","/products/403.html|饼干蛋糕","/products/404.html|无糖食品"]},{"u":"/products/405.html","n":"粮油调味","i":["/products/406.html|米面杂粮","/products/407.html|食用油","/products/408.html|调味品","/products/409.html|南北干货","/products/410.html|方便食品","/products/411.html|有机食品"]},{"u":"/products/412.html","n":"饮料冲调","i":["/products/413.html|饮用水","/products/414.html|饮料","/products/415.html|牛奶乳品","/products/416.html|咖啡/奶茶","/products/417.html|冲饮谷物","/products/418.html|蜂蜜/柚子茶","/products/419.html|成人奶粉"]},{"u":"/products/420.html","n":"食品礼券","i":["/products/421.html|月饼","/products/422.html|大闸蟹","/products/423.html|粽子","/products/424.html|卡券"]},{"u":"/products/425.html","n":"茗茶","i":["/products/426.html|铁观音","/products/427.html|普洱","/products/428.html|龙井","/products/429.html|绿茶","/products/430.html|红茶","/products/431.html|乌龙茶","/products/432.html|花草茶","/products/433.html|花果茶","/products/434.html|养生茶","/products/435.html|黑茶","/products/436.html|白茶","/products/437.html|其它茶"]}]},{"u":"/products/438.html","n":"<a href='/products/438.html'>汽车用品</a>","i":[{"u":"/products/439.html","n":"维修保养","i":["/products/440.html|润滑油","/products/441.html|添加剂","/products/442.html|防冻液","/products/443.html|滤清器","/products/444.html|火花塞","/products/445.html|雨刷","/products/446.html|车灯","/products/447.html|后视镜","/products/448.html|轮胎","/products/449.html|轮毂","/products/450.html|刹车片/盘","/products/451.html|喇叭/皮带","/products/452.html|蓄电池","/products/453.html|底盘装甲/护板","/products/454.html|贴膜","/products/455.html|汽修工具"]},{"u":"/products/456.html","n":"车载电器","i":["/products/457.html|导航仪","/products/458.html|安全预警仪","/products/459.html|行车记录仪","/products/460.html|倒车雷达","/products/461.html|蓝牙设备","/products/462.html|时尚影音","/products/463.html|净化器","/products/464.html|电源","/products/465.html|冰箱","/products/466.html|吸尘器"]},{"u":"/products/467.html","n":"美容清洗","i":["/products/468.html|车蜡","/products/469.html|补漆笔","/products/470.html|玻璃水","/products/471.html|清洁剂","/products/472.html|洗车工具","/products/473.html|洗车配件"]},{"u":"/products/474.html","n":"汽车装饰","i":["/products/475.html|脚垫","/products/476.html|座垫","/products/477.html|座套","/products/478.html|后备箱垫","/products/479.html|头枕腰靠","/products/480.html|香水","/products/481.html|空气净化","/products/482.html|车内饰品","/products/483.html|功能小件","/products/484.html|车身装饰件","/products/485.html|车衣"]},{"u":"/products/486.html","n":"安全自驾","i":["/products/487.html|安全座椅","/products/488.html|胎压充气","/products/489.html|防盗设备","/products/490.html|应急救援","/products/491.html|保温箱","/products/492.html|储物箱","/products/493.html|自驾野营","/products/494.html|摩托车装备"]}]},{"u":"/products/495.html","n":"<a href='/products/495.html'>玩具乐器</a>","i":[{"u":"/products/496.html","n":"适用年龄","i":["/products/497.html|0-6个月","/products/498.html|6-12个月","/products/499.html|1-3岁","/products/500.html|3-6岁","/products/501.html|6-14岁","/products/502.html|14岁以上"]},{"u":"/products/503.html","n":"遥控/电动","i":["/products/504.html|遥控车","/products/505.html|遥控飞机","/products/506.html|遥控船","/products/507.html|机器人/电动","/products/508.html|轨道/助力"]},{"u":"/products/509.html","n":"毛绒布艺","i":["/products/510.html|毛绒/布艺","/products/511.html|靠垫/抱枕"]},{"u":"/products/512.html","n":"娃娃玩具","i":["/products/513.html|芭比娃娃","/products/514.html|卡通娃娃","/products/515.html|智能娃娃"]},{"u":"/products/516.html","n":"模型玩具","i":["/products/517.html|仿真模型","/products/518.html|拼插模型","/products/519.html|收藏爱好"]},{"u":"/products/520.html","n":"健身玩具","i":["/products/521.html|炫舞毯","/products/522.html|爬行垫/毯","/products/523.html|户外玩具","/products/524.html|戏水玩具"]},{"u":"/products/525.html","n":"动漫玩具","i":["/products/526.html|电影周边","/products/527.html|卡通周边","/products/528.html|网游周边"]},{"u":"/products/529.html","n":"益智玩具","i":["/products/530.html|摇铃/床铃","/products/531.html|健身架","/products/532.html|早教启智","/products/533.html|拖拉玩具"]},{"u":"/products/534.html","n":"积木拼插","i":["/products/535.html|积木","/products/536.html|拼图","/products/537.html|磁力棒","/products/538.html|立体拼插"]},{"u":"/products/539.html","n":"DIY玩具","i":["/products/540.html|手工彩泥","/products/541.html|绘画工具","/products/542.html|情景玩具"]},{"u":"/products/543.html","n":"创意减压","i":["/products/544.html|减压玩具","/products/545.html|创意玩具"]},{"u":"/products/546.html","n":"乐器相关","i":["/products/547.html|钢琴","/products/548.html|电子琴","/products/549.html|手风琴","/products/550.html|吉他/贝斯","/products/551.html|民族管弦乐器","/products/552.html|西洋管弦乐","/products/553.html|口琴/口风琴/竖笛","/products/554.html|西洋打击乐器","/products/555.html|各式乐器配件","/products/556.html|电脑音乐","/products/557.html|工艺礼品乐器"]}]},{"u":"/products/558.html","n":"<a href='/products/558.html'>手机</a>","i":[{"u":"/products/559.html","n":"手机通讯","i":["/products/560.html|手机","/products/561.html|对讲机"]},{"u":"/products/562.html","n":"运营商","i":["/products/563.html|购机送费","/products/564.html|“0”元购机","/products/565.html|选号中心","/products/566.html|选号入网"]},{"u":"/products/567.html","n":"手机配件","i":["/products/568.html|手机电池","/products/569.html|蓝牙耳机","/products/570.html|充电器/数据线","/products/571.html|手机耳机","/products/572.html|手机贴膜","/products/573.html|手机存储卡","/products/574.html|手机保护套","/products/575.html|车载配件","/products/576.html|iPhone 配件","/products/577.html|创意配件","/products/578.html|便携/无线音响","/products/579.html|手机饰品"]}]},{"u":"/products/580.html","n":"<a href='/products/580.html'>数码</a>","i":[{"u":"/products/581.html","n":"摄影摄像","i":["/products/582.html|数码相机","/products/583.html|单电/微单相机","/products/584.html|单反相机","/products/585.html|摄像机","/products/586.html|拍立得","/products/587.html|运动相机","/products/588.html|镜头","/products/589.html|户外器材","/products/590.html|影棚器材"]},{"u":"/products/591.html","n":"数码配件","i":["/products/592.html|存储卡","/products/593.html|读卡器","/products/594.html|滤镜","/products/595.html|闪光灯/手柄","/products/596.html|相机包","/products/597.html|三脚架/云台","/products/598.html|相机清洁","/products/599.html|相机贴膜","/products/600.html|机身附件","/products/601.html|镜头附件","/products/602.html|电池/充电器","/products/603.html|移动电源"]},{"u":"/products/604.html","n":"智能设备","i":["/products/605.html|智能手环","/products/606.html|智能手表","/products/607.html|智能眼镜","/products/608.html|运动跟踪器","/products/609.html|健康监测","/products/610.html|智能配饰","/products/611.html|智能家居","/products/612.html|体感车","/products/613.html|其他配件"]},{"u":"/products/614.html","n":"时尚影音","i":["/products/615.html|MP3/MP4","/products/616.html|智能设备","/products/617.html|耳机/耳麦","/products/618.html|音箱","/products/619.html|高清播放器","/products/620.html|MP3/MP4配件","/products/621.html|麦克风","/products/622.html|专业音频","/products/623.html|数码相框","/products/624.html|苹果配件"]},{"u":"/products/625.html","n":"电子教育","i":["/products/626.html|电子词典","/products/627.html|电纸书","/products/628.html|录音笔","/products/629.html|复读机","/products/630.html|点读机/笔","/products/631.html|学生平板","/products/632.html|早教机"]}]},{"u":"/products/633.html","n":"<a href='/products/633.html'>家居家装</a>","i":[{"u":"/products/634.html","n":"家纺","i":["/products/635.html|床品套件","/products/636.html|被子","/products/637.html|枕芯","/products/638.html|床单被罩","/products/639.html|毯子","/products/640.html|床垫/床褥","/products/641.html|蚊帐","/products/642.html|抱枕靠垫","/products/643.html|毛巾浴巾","/products/644.html|电热毯","/products/645.html|窗帘/窗纱","/products/646.html|布艺软饰","/products/647.html|凉席"]},{"u":"/products/648.html","n":"灯具","i":["/products/649.html|台灯","/products/650.html|节能灯","/products/651.html|装饰灯","/products/652.html|落地灯","/products/653.html|应急灯/手电","/products/654.html|LED灯","/products/655.html|吸顶灯","/products/656.html|五金电器","/products/657.html|筒灯射灯","/products/658.html|吊灯","/products/659.html|氛围照明"]},{"u":"/products/660.html","n":"生活日用","i":["/products/661.html|收纳用品","/products/662.html|雨伞雨具","/products/663.html|浴室用品","/products/664.html|缝纫/针织用品","/products/665.html|洗晒/熨烫","/products/666.html|净化除味"]},{"u":"/products/667.html","n":"家装软饰","i":["/products/668.html|桌布/罩件","/products/669.html|地毯地垫","/products/670.html|沙发垫套/椅垫","/products/671.html|相框/照片墙","/products/672.html|装饰字画","/products/673.html|节庆饰品","/products/674.html|手工/十字绣","/products/675.html|装饰摆件","/products/676.html|保暖防护","/products/677.html|帘艺隔断","/products/678.html|墙贴/装饰贴","/products/679.html|钟饰","/products/680.html|花瓶花艺","/products/681.html|香薰蜡烛","/products/682.html|创意家居"]},{"u":"/products/683.html","n":"清洁用品","i":["/products/684.html|纸品湿巾","/products/685.html|衣物清洁","/products/686.html|清洁工具","/products/687.html|驱虫用品","/products/688.html|家庭清洁","/products/689.html|皮具护理","/products/690.html|一次性用品"]},{"u":"/products/691.html","n":"宠物生活","i":["/products/692.html|宠物主粮","/products/693.html|宠物零食","/products/694.html|医疗保健","/products/695.html|家居日用","/products/696.html|宠物玩具","/products/697.html|出行装备","/products/698.html|洗护美容"]}]},{"u":"/products/699.html","n":"<a href='/products/699.html'>厨具</a>","i":[{"u":"/products/700.html","n":"烹饪锅具","i":["/products/701.html|炒锅","/products/702.html|煎锅","/products/703.html|压力锅","/products/704.html|蒸锅","/products/705.html|汤锅","/products/706.html|奶锅","/products/707.html|锅具套装","/products/708.html|煲类","/products/709.html|水壶","/products/710.html|火锅"]},{"u":"/products/711.html","n":"刀剪菜板","i":["/products/712.html|菜刀","/products/713.html|剪刀","/products/714.html|刀具套装","/products/715.html|砧板","/products/716.html|瓜果刀/刨","/products/717.html|多功能刀"]},{"u":"/products/718.html","n":"厨房配件","i":["/products/719.html|保鲜盒","/products/720.html|烘焙/烧烤","/products/721.html|饭盒/提锅","/products/722.html|储物/置物架","/products/723.html|厨房DIY/小工具"]},{"u":"/products/724.html","n":"水具酒具","i":["/products/725.html|塑料杯","/products/726.html|运动水壶","/products/727.html|玻璃杯","/products/728.html|陶瓷/马克杯","/products/729.html|保温杯","/products/730.html|保温壶","/products/731.html|酒杯/酒具","/products/732.html|杯具套装"]},{"u":"/products/733.html","n":"餐具","i":["/products/734.html|餐具套装","/products/735.html|碗/碟/盘","/products/736.html|筷勺/刀叉","/products/737.html|一次性用品","/products/738.html|果盘/果篮"]},{"u":"/products/739.html","n":"茶具/咖啡具","i":["/products/740.html|整套茶具","/products/741.html|茶杯","/products/742.html|茶壶","/products/743.html|茶盘茶托","/products/744.html|茶叶罐","/products/745.html|茶具配件","/products/746.html|茶宠摆件","/products/747.html|咖啡具","/products/748.html|其他"]}]},{"u":"/products/749.html","n":"<a href='/products/749.html'>服饰内衣</a>","i":[{"u":"/products/750.html","n":"女装","i":["/products/751.html|T恤","/products/752.html|衬衫","/products/753.html|针织衫","/products/754.html|雪纺衫","/products/755.html|卫衣","/products/756.html|马甲","/products/757.html|连衣裙","/products/758.html|半身裙","/products/759.html|牛仔裤","/products/760.html|休闲裤","/products/761.html|打底裤","/products/762.html|正装裤","/products/763.html|小西装","/products/764.html|短外套","/products/765.html|风衣","/products/766.html|毛呢大衣","/products/767.html|真皮皮衣","/products/768.html|棉服","/products/769.html|羽绒服","/products/770.html|大码女装","/products/771.html|中老年女装","/products/772.html|婚纱","/products/773.html|打底衫","/products/774.html|旗袍/唐装","/products/775.html|加绒裤","/products/776.html|吊带/背心","/products/777.html|羊绒衫","/products/778.html|短裤","/products/779.html|皮草","/products/780.html|礼服","/products/781.html|仿皮皮衣","/products/782.html|羊毛衫","/products/783.html|设计师/潮牌"]},{"u":"/products/784.html","n":"男装","i":["/products/785.html|衬衫","/products/786.html|T恤","/products/787.html|POLO衫","/products/788.html|针织衫","/products/789.html|羊绒衫","/products/790.html|卫衣","/products/791.html|马甲/背心","/products/792.html|夹克","/products/793.html|风衣","/products/794.html|毛呢大衣","/products/795.html|仿皮皮衣","/products/796.html|西服","/products/797.html|棉服","/products/798.html|羽绒服","/products/799.html|牛仔裤","/products/800.html|休闲裤","/products/801.html|西裤","/products/802.html|西服套装","/products/803.html|大码男装","/products/804.html|中老年男装","/products/805.html|唐装/中山装","/products/806.html|工装","/products/807.html|真皮皮衣","/products/808.html|加绒裤","/products/809.html|卫裤/运动裤","/products/810.html|短裤","/products/811.html|设计师/潮牌","/products/812.html|羊毛衫"]},{"u":"/products/813.html","n":"内衣","i":["/products/814.html|文胸","/products/815.html|女式内裤","/products/816.html|男式内裤","/products/817.html|睡衣/家居服","/products/818.html|塑身美体","/products/819.html|泳衣","/products/820.html|吊带/背心","/products/821.html|抹胸","/products/822.html|连裤袜/丝袜","/products/823.html|美腿袜","/products/824.html|商务男袜","/products/825.html|保暖内衣","/products/826.html|情侣睡衣","/products/827.html|文胸套装","/products/828.html|少女文胸","/products/829.html|休闲棉袜","/products/830.html|大码内衣","/products/831.html|内衣配件","/products/832.html|打底裤袜","/products/833.html|打底衫","/products/834.html|秋衣秋裤","/products/835.html|情趣内衣"]},{"u":"/products/836.html","n":"服饰配件","i":["/products/837.html|太阳镜","/products/838.html|光学镜架/镜片","/products/839.html|围巾/手套/帽子套装","/products/840.html|袖扣","/products/841.html|棒球帽","/products/842.html|毛线帽","/products/843.html|遮阳帽","/products/844.html|老花镜","/products/845.html|装饰眼镜","/products/846.html|防辐射眼镜","/products/847.html|游泳镜","/products/848.html|女士丝巾/围巾/披肩","/products/849.html|男士丝巾/围巾","/products/850.html|鸭舌帽","/products/851.html|贝雷帽","/products/852.html|礼帽","/products/853.html|真皮手套","/products/854.html|毛线手套","/products/855.html|防晒手套","/products/856.html|男士腰带/礼盒","/products/857.html|女士腰带/礼盒","/products/858.html|钥匙扣","/products/859.html|遮阳伞/雨伞","/products/860.html|口罩","/products/861.html|耳罩/耳包","/products/862.html|假领","/products/863.html|毛线/布面料","/products/864.html|领带/领结/领带夹"]}]}]}
http://manage.taotao.com/rest/api/item/cat?callback=category.getDataService

category.getDataService({"data":[{"u":"/products/1.html","n":"<a href='/products/1.html'>图书、音像、电子书刊</a>","i":[{"u":"/products/2.html","n":"电子书刊","i":["/products/3.html|电子书","/products/4.html|网络原创","/products/5.html|数字杂志","/products/6.html|多媒体图书"]},{"u":"/products/7.html","n":"音像","i":["/products/8.html|音乐","/products/9.html|影视","/products/10.html|教育音像"]},{"u":"/products/11.html","n":"英文原版","i":["/products/12.html|少儿","/products/13.html|商务投资","/products/14.html|英语学习与考试","/products/15.html|小说","/products/16.html|传记","/products/17.html|励志"]},{"u":"/products/18.html","n":"文艺","i":["/products/19.html|小说","/products/20.html|文学","/products/21.html|青春文学","/products/22.html|传记","/products/23.html|艺术"]},{"u":"/products/24.html","n":"少儿","i":["/products/25.html|少儿","/products/26.html|0-2岁","/products/27.html|3-6岁","/products/28.html|7-10岁","/products/29.html|11-14岁"]},{"u":"/products/30.html","n":"人文社科","i":["/products/31.html|历史","/products/32.html|哲学","/products/33.html|国学","/products/34.html|政治/军事","/products/35.html|法律","/products/36.html|宗教","/products/37.html|心理学","/products/38.html|文化","/products/39.html|社会科学"]},{"u":"/products/40.html","n":"经管励志","i":["/products/41.html|经济","/products/42.html|金融与投资","/products/43.html|管理","/products/44.html|励志与成功"]},{"u":"/products/45.html","n":"生活","i":["/products/46.html|生活","/products/47.html|健身与保健","/products/48.html|家庭与育儿","/products/49.html|旅游","/products/50.html|动漫/幽默"]},{"u":"/products/51.html","n":"科技","i":["/products/52.html|科技","/products/53.html|工程","/products/54.html|建筑","/products/55.html|医学","/products/56.html|科学与自然","/products/57.html|计算机与互联网","/products/58.html|体育/运动"]},{"u":"/products/59.html","n":"教育","i":["/products/60.html|教材教辅","/products/61.html|教育与考试","/products/62.html|外语学习","/products/63.html|新闻出版","/products/64.html|语言文字"]},{"u":"/products/65.html","n":"港台图书","i":["/products/66.html|艺术/设计/收藏","/products/67.html|经济管理","/products/68.html|文化/学术","/products/69.html|少儿文学/国学"]},{"u":"/products/70.html","n":"其它","i":["/products/71.html|工具书","/products/72.html|影印版","/products/73.html|套装书"]}]},{"u":"/products/74.html","n":"<a href='/products/74.html'>家用电器</a>","i":[{"u":"/products/75.html","n":"大 家 电","i":["/products/76.html|平板电视","/products/77.html|空调","/products/78.html|冰箱","/products/79.html|洗衣机","/products/80.html|家庭影院","/products/81.html|DVD播放机","/products/82.html|迷你音响","/products/83.html|烟机/灶具","/products/84.html|热水器","/products/85.html|消毒柜/洗碗机","/products/86.html|酒柜/冰吧/冷柜","/products/87.html|家电配件","/products/88.html|家电下乡"]},{"u":"/products/89.html","n":"生活电器","i":["/products/90.html|电风扇","/products/91.html|冷风扇","/products/92.html|净化器","/products/93.html|饮水机","/products/94.html|净水设备","/products/95.html|挂烫机/熨斗","/products/96.html|吸尘器","/products/97.html|电话机","/products/98.html|插座","/products/99.html|收录/音机","/products/100.html|清洁机","/products/101.html|加湿器","/products/102.html|除湿机","/products/103.html|取暖电器","/products/104.html|其它生活电器","/products/105.html|扫地机器人","/products/106.html|干衣机","/products/107.html|生活电器配件"]},{"u":"/products/108.html","n":"厨房电器","i":["/products/109.html|料理/榨汁机","/products/110.html|豆浆机","/products/111.html|电饭煲","/products/112.html|电压力锅","/products/113.html|面包机","/products/114.html|咖啡机","/products/115.html|微波炉","/products/116.html|电烤箱","/products/117.html|电磁炉","/products/118.html|电饼铛/烧烤盘","/products/119.html|煮蛋器","/products/120.html|酸奶机","/products/121.html|电炖锅","/products/122.html|电水壶/热水瓶","/products/123.html|多用途锅","/products/124.html|果蔬解毒机","/products/125.html|其它厨房电器"]},{"u":"/products/126.html","n":"个护健康","i":["/products/127.html|剃须刀","/products/128.html|剃/脱毛器","/products/129.html|口腔护理","/products/130.html|电吹风","/products/131.html|美容器","/products/132.html|美发器","/products/133.html|按摩椅","/products/134.html|按摩器","/products/135.html|足浴盆","/products/136.html|血压计","/products/137.html|健康秤/厨房秤","/products/138.html|血糖仪","/products/139.html|体温计","/products/140.html|计步器/脂肪检测仪","/products/141.html|其它健康电器"]},{"u":"/products/142.html","n":"五金家装","i":["/products/143.html|电动工具","/products/144.html|手动工具","/products/145.html|仪器仪表","/products/146.html|浴霸/排气扇","/products/147.html|灯具","/products/148.html|LED灯","/products/149.html|洁身器","/products/150.html|水槽","/products/151.html|龙头","/products/152.html|淋浴花洒","/products/153.html|厨卫五金","/products/154.html|家具五金","/products/155.html|门铃","/products/156.html|电气开关","/products/157.html|插座","/products/158.html|电工电料","/products/159.html|监控安防","/products/160.html|电线/线缆"]}]},{"u":"/products/161.html","n":"<a href='/products/161.html'>电脑、办公</a>","i":[{"u":"/products/162.html","n":"电脑整机","i":["/products/163.html|笔记本","/products/164.html|超极本","/products/165.html|游戏本","/products/166.html|平板电脑","/products/167.html|平板电脑配件","/products/168.html|台式机","/products/169.html|服务器/工作站","/products/170.html|笔记本配件"]},{"u":"/products/171.html","n":"电脑配件","i":["/products/172.html|CPU","/products/173.html|主板","/products/174.html|显卡","/products/175.html|硬盘","/products/176.html|SSD固态硬盘","/products/177.html|内存","/products/178.html|机箱","/products/179.html|电源","/products/180.html|显示器","/products/181.html|刻录机/光驱","/products/182.html|散热器","/products/183.html|声卡/扩展卡","/products/184.html|装机配件","/products/185.html|组装电脑"]},{"u":"/products/186.html","n":"外设产品","i":["/products/187.html|移动硬盘","/products/188.html|U盘","/products/189.html|鼠标","/products/190.html|键盘","/products/191.html|鼠标垫","/products/192.html|摄像头","/products/193.html|手写板","/products/194.html|外置盒","/products/195.html|插座","/products/196.html|线缆","/products/197.html|UPS电源","/products/198.html|电脑工具","/products/199.html|游戏设备","/products/200.html|电玩","/products/201.html|电脑清洁"]},{"u":"/products/202.html","n":"网络产品","i":["/products/203.html|路由器","/products/204.html|网卡","/products/205.html|交换机","/products/206.html|网络存储","/products/207.html|4G/3G上网","/products/208.html|网络盒子","/products/209.html|网络配件"]},{"u":"/products/210.html","n":"办公设备","i":["/products/211.html|投影机","/products/212.html|投影配件","/products/213.html|多功能一体机","/products/214.html|打印机","/products/215.html|传真设备","/products/216.html|验钞/点钞机","/products/217.html|扫描设备","/products/218.html|复合机","/products/219.html|碎纸机","/products/220.html|考勤机","/products/221.html|墨粉","/products/222.html|收款/POS机","/products/223.html|会议音频视频","/products/224.html|保险柜","/products/225.html|装订/封装机","/products/226.html|安防监控","/products/227.html|办公家具","/products/228.html|白板"]},{"u":"/products/229.html","n":"文具/耗材","i":["/products/230.html|硒鼓/墨粉","/products/231.html|墨盒","/products/232.html|色带","/products/233.html|纸类","/products/234.html|办公文具","/products/235.html|学生文具","/products/236.html|文件管理","/products/237.html|财会用品","/products/238.html|本册/便签","/products/239.html|计算器","/products/240.html|激光笔","/products/241.html|笔类","/products/242.html|画具画材","/products/243.html|刻录碟片/附件"]},{"u":"/products/244.html","n":"服务产品","i":["/products/245.html|上门服务","/products/246.html|远程服务","/products/247.html|电脑软件","/products/248.html|京东服务"]}]},{"u":"/products/249.html","n":"<a href='/products/249.html'>个护化妆</a>","i":[{"u":"/products/250.html","n":"面部护肤","i":["/products/251.html|清洁","/products/252.html|护肤","/products/253.html|面膜","/products/254.html|剃须","/products/255.html|套装"]},{"u":"/products/256.html","n":"身体护肤","i":["/products/257.html|沐浴","/products/258.html|润肤","/products/259.html|颈部","/products/260.html|手足","/products/261.html|纤体塑形","/products/262.html|美胸","/products/263.html|套装"]},{"u":"/products/264.html","n":"口腔护理","i":["/products/265.html|牙膏/牙粉","/products/266.html|牙刷/牙线","/products/267.html|漱口水","/products/268.html|套装"]},{"u":"/products/269.html","n":"女性护理","i":["/products/270.html|卫生巾","/products/271.html|卫生护垫","/products/272.html|私密护理","/products/273.html|脱毛膏"]},{"u":"/products/274.html","n":"洗发护发","i":["/products/275.html|洗发","/products/276.html|护发","/products/277.html|染发","/products/278.html|造型","/products/279.html|假发","/products/280.html|套装"]},{"u":"/products/281.html","n":"香水彩妆","i":["/products/282.html|香水","/products/283.html|底妆","/products/284.html|腮红","/products/285.html|眼部","/products/286.html|唇部","/products/287.html|美甲","/products/288.html|美容工具","/products/289.html|套装"]}]},{"u":"/products/290.html","n":"<a href='/products/290.html'>钟表</a>","i":[{"u":"/products/291.html","n":"钟表","i":["/products/292.html|男表","/products/293.html|女表","/products/294.html|儿童手表","/products/295.html|座钟挂钟"]}]},{"u":"/products/296.html","n":"<a href='/products/296.html'>母婴</a>","i":[{"u":"/products/297.html","n":"奶粉","i":["/products/298.html|婴幼奶粉","/products/299.html|成人奶粉"]},{"u":"/products/300.html","n":"营养辅食","i":["/products/301.html|益生菌/初乳","/products/302.html|米粉/菜粉","/products/303.html|果泥/果汁","/products/304.html|DHA","/products/305.html|宝宝零食","/products/306.html|钙铁锌/维生素","/products/307.html|清火/开胃","/products/308.html|面条/粥"]},{"u":"/products/309.html","n":"尿裤湿巾","i":["/products/310.html|婴儿尿裤","/products/311.html|拉拉裤","/products/312.html|湿巾","/products/313.html|成人尿裤"]},{"u":"/products/314.html","n":"喂养用品","i":["/products/315.html|奶瓶奶嘴","/products/316.html|吸奶器","/products/317.html|暖奶消毒","/products/318.html|碗盘叉勺","/products/319.html|水壶/水杯","/products/320.html|牙胶安抚","/products/321.html|辅食料理机"]},{"u":"/products/322.html","n":"洗护用品","i":["/products/323.html|宝宝护肤","/products/324.html|宝宝洗浴","/products/325.html|奶瓶清洗","/products/326.html|驱蚊防蚊","/products/327.html|理发器","/products/328.html|洗衣液/皂","/products/329.html|日常护理","/products/330.html|座便器"]},{"u":"/products/331.html","n":"童车童床","i":["/products/332.html|婴儿推车","/products/333.html|餐椅摇椅","/products/334.html|婴儿床","/products/335.html|学步车","/products/336.html|三轮车","/products/337.html|自行车","/products/338.html|电动车","/products/339.html|扭扭车","/products/340.html|滑板车"]},{"u":"/products/341.html","n":"寝居服饰","i":["/products/342.html|婴儿外出服","/products/343.html|婴儿内衣","/products/344.html|婴儿礼盒","/products/345.html|婴儿鞋帽袜","/products/346.html|安全防护","/products/347.html|家居床品"]},{"u":"/products/348.html","n":"妈妈专区","i":["/products/349.html|妈咪包/背婴带","/products/350.html|产后塑身","/products/351.html|文胸/内裤","/products/352.html|防辐射服","/products/353.html|孕妇装","/products/354.html|孕期营养","/products/355.html|孕妈美容","/products/356.html|待产/新生","/products/357.html|月子装"]},{"u":"/products/358.html","n":"童装童鞋","i":["/products/359.html|套装","/products/360.html|上衣","/products/361.html|裤子","/products/362.html|裙子","/products/363.html|内衣/家居服","/products/364.html|羽绒服/棉服","/products/365.html|亲子装","/products/366.html|儿童配饰","/products/367.html|礼服/演出服","/products/368.html|运动鞋","/products/369.html|皮鞋/帆布鞋","/products/370.html|靴子","/products/371.html|凉鞋","/products/372.html|功能鞋","/products/373.html|户外/运动服"]},{"u":"/products/374.html","n":"安全座椅","i":["/products/375.html|提篮式","/products/376.html|安全座椅","/products/377.html|增高垫"]}]},{"u":"/products/378.html","n":"<a href='/products/378.html'>食品饮料、保健食品</a>","i":[{"u":"/products/379.html","n":"进口食品","i":["/products/380.html|饼干蛋糕","/products/381.html|糖果/巧克力","/products/382.html|休闲零食","/products/383.html|冲调饮品","/products/384.html|粮油调味","/products/385.html|牛奶"]},{"u":"/products/386.html","n":"地方特产","i":["/products/387.html|其他特产","/products/388.html|新疆","/products/389.html|北京","/products/390.html|山西","/products/391.html|内蒙古","/products/392.html|福建","/products/393.html|湖南","/products/394.html|四川","/products/395.html|云南","/products/396.html|东北"]},{"u":"/products/397.html","n":"休闲食品","i":["/products/398.html|休闲零食","/products/399.html|坚果炒货","/products/400.html|肉干肉脯","/products/401.html|蜜饯果干","/products/402.html|糖果/巧克力","/products/403.html|饼干蛋糕","/products/404.html|无糖食品"]},{"u":"/products/405.html","n":"粮油调味","i":["/products/406.html|米面杂粮","/products/407.html|食用油","/products/408.html|调味品","/products/409.html|南北干货","/products/410.html|方便食品","/products/411.html|有机食品"]},{"u":"/products/412.html","n":"饮料冲调","i":["/products/413.html|饮用水","/products/414.html|饮料","/products/415.html|牛奶乳品","/products/416.html|咖啡/奶茶","/products/417.html|冲饮谷物","/products/418.html|蜂蜜/柚子茶","/products/419.html|成人奶粉"]},{"u":"/products/420.html","n":"食品礼券","i":["/products/421.html|月饼","/products/422.html|大闸蟹","/products/423.html|粽子","/products/424.html|卡券"]},{"u":"/products/425.html","n":"茗茶","i":["/products/426.html|铁观音","/products/427.html|普洱","/products/428.html|龙井","/products/429.html|绿茶","/products/430.html|红茶","/products/431.html|乌龙茶","/products/432.html|花草茶","/products/433.html|花果茶","/products/434.html|养生茶","/products/435.html|黑茶","/products/436.html|白茶","/products/437.html|其它茶"]}]},{"u":"/products/438.html","n":"<a href='/products/438.html'>汽车用品</a>","i":[{"u":"/products/439.html","n":"维修保养","i":["/products/440.html|润滑油","/products/441.html|添加剂","/products/442.html|防冻液","/products/443.html|滤清器","/products/444.html|火花塞","/products/445.html|雨刷","/products/446.html|车灯","/products/447.html|后视镜","/products/448.html|轮胎","/products/449.html|轮毂","/products/450.html|刹车片/盘","/products/451.html|喇叭/皮带","/products/452.html|蓄电池","/products/453.html|底盘装甲/护板","/products/454.html|贴膜","/products/455.html|汽修工具"]},{"u":"/products/456.html","n":"车载电器","i":["/products/457.html|导航仪","/products/458.html|安全预警仪","/products/459.html|行车记录仪","/products/460.html|倒车雷达","/products/461.html|蓝牙设备","/products/462.html|时尚影音","/products/463.html|净化器","/products/464.html|电源","/products/465.html|冰箱","/products/466.html|吸尘器"]},{"u":"/products/467.html","n":"美容清洗","i":["/products/468.html|车蜡","/products/469.html|补漆笔","/products/470.html|玻璃水","/products/471.html|清洁剂","/products/472.html|洗车工具","/products/473.html|洗车配件"]},{"u":"/products/474.html","n":"汽车装饰","i":["/products/475.html|脚垫","/products/476.html|座垫","/products/477.html|座套","/products/478.html|后备箱垫","/products/479.html|头枕腰靠","/products/480.html|香水","/products/481.html|空气净化","/products/482.html|车内饰品","/products/483.html|功能小件","/products/484.html|车身装饰件","/products/485.html|车衣"]},{"u":"/products/486.html","n":"安全自驾","i":["/products/487.html|安全座椅","/products/488.html|胎压充气","/products/489.html|防盗设备","/products/490.html|应急救援","/products/491.html|保温箱","/products/492.html|储物箱","/products/493.html|自驾野营","/products/494.html|摩托车装备"]}]},{"u":"/products/495.html","n":"<a href='/products/495.html'>玩具乐器</a>","i":[{"u":"/products/496.html","n":"适用年龄","i":["/products/497.html|0-6个月","/products/498.html|6-12个月","/products/499.html|1-3岁","/products/500.html|3-6岁","/products/501.html|6-14岁","/products/502.html|14岁以上"]},{"u":"/products/503.html","n":"遥控/电动","i":["/products/504.html|遥控车","/products/505.html|遥控飞机","/products/506.html|遥控船","/products/507.html|机器人/电动","/products/508.html|轨道/助力"]},{"u":"/products/509.html","n":"毛绒布艺","i":["/products/510.html|毛绒/布艺","/products/511.html|靠垫/抱枕"]},{"u":"/products/512.html","n":"娃娃玩具","i":["/products/513.html|芭比娃娃","/products/514.html|卡通娃娃","/products/515.html|智能娃娃"]},{"u":"/products/516.html","n":"模型玩具","i":["/products/517.html|仿真模型","/products/518.html|拼插模型","/products/519.html|收藏爱好"]},{"u":"/products/520.html","n":"健身玩具","i":["/products/521.html|炫舞毯","/products/522.html|爬行垫/毯","/products/523.html|户外玩具","/products/524.html|戏水玩具"]},{"u":"/products/525.html","n":"动漫玩具","i":["/products/526.html|电影周边","/products/527.html|卡通周边","/products/528.html|网游周边"]},{"u":"/products/529.html","n":"益智玩具","i":["/products/530.html|摇铃/床铃","/products/531.html|健身架","/products/532.html|早教启智","/products/533.html|拖拉玩具"]},{"u":"/products/534.html","n":"积木拼插","i":["/products/535.html|积木","/products/536.html|拼图","/products/537.html|磁力棒","/products/538.html|立体拼插"]},{"u":"/products/539.html","n":"DIY玩具","i":["/products/540.html|手工彩泥","/products/541.html|绘画工具","/products/542.html|情景玩具"]},{"u":"/products/543.html","n":"创意减压","i":["/products/544.html|减压玩具","/products/545.html|创意玩具"]},{"u":"/products/546.html","n":"乐器相关","i":["/products/547.html|钢琴","/products/548.html|电子琴","/products/549.html|手风琴","/products/550.html|吉他/贝斯","/products/551.html|民族管弦乐器","/products/552.html|西洋管弦乐","/products/553.html|口琴/口风琴/竖笛","/products/554.html|西洋打击乐器","/products/555.html|各式乐器配件","/products/556.html|电脑音乐","/products/557.html|工艺礼品乐器"]}]},{"u":"/products/558.html","n":"<a href='/products/558.html'>手机</a>","i":[{"u":"/products/559.html","n":"手机通讯","i":["/products/560.html|手机","/products/561.html|对讲机"]},{"u":"/products/562.html","n":"运营商","i":["/products/563.html|购机送费","/products/564.html|“0”元购机","/products/565.html|选号中心","/products/566.html|选号入网"]},{"u":"/products/567.html","n":"手机配件","i":["/products/568.html|手机电池","/products/569.html|蓝牙耳机","/products/570.html|充电器/数据线","/products/571.html|手机耳机","/products/572.html|手机贴膜","/products/573.html|手机存储卡","/products/574.html|手机保护套","/products/575.html|车载配件","/products/576.html|iPhone 配件","/products/577.html|创意配件","/products/578.html|便携/无线音响","/products/579.html|手机饰品"]}]},{"u":"/products/580.html","n":"<a href='/products/580.html'>数码</a>","i":[{"u":"/products/581.html","n":"摄影摄像","i":["/products/582.html|数码相机","/products/583.html|单电/微单相机","/products/584.html|单反相机","/products/585.html|摄像机","/products/586.html|拍立得","/products/587.html|运动相机","/products/588.html|镜头","/products/589.html|户外器材","/products/590.html|影棚器材"]},{"u":"/products/591.html","n":"数码配件","i":["/products/592.html|存储卡","/products/593.html|读卡器","/products/594.html|滤镜","/products/595.html|闪光灯/手柄","/products/596.html|相机包","/products/597.html|三脚架/云台","/products/598.html|相机清洁","/products/599.html|相机贴膜","/products/600.html|机身附件","/products/601.html|镜头附件","/products/602.html|电池/充电器","/products/603.html|移动电源"]},{"u":"/products/604.html","n":"智能设备","i":["/products/605.html|智能手环","/products/606.html|智能手表","/products/607.html|智能眼镜","/products/608.html|运动跟踪器","/products/609.html|健康监测","/products/610.html|智能配饰","/products/611.html|智能家居","/products/612.html|体感车","/products/613.html|其他配件"]},{"u":"/products/614.html","n":"时尚影音","i":["/products/615.html|MP3/MP4","/products/616.html|智能设备","/products/617.html|耳机/耳麦","/products/618.html|音箱","/products/619.html|高清播放器","/products/620.html|MP3/MP4配件","/products/621.html|麦克风","/products/622.html|专业音频","/products/623.html|数码相框","/products/624.html|苹果配件"]},{"u":"/products/625.html","n":"电子教育","i":["/products/626.html|电子词典","/products/627.html|电纸书","/products/628.html|录音笔","/products/629.html|复读机","/products/630.html|点读机/笔","/products/631.html|学生平板","/products/632.html|早教机"]}]},{"u":"/products/633.html","n":"<a href='/products/633.html'>家居家装</a>","i":[{"u":"/products/634.html","n":"家纺","i":["/products/635.html|床品套件","/products/636.html|被子","/products/637.html|枕芯","/products/638.html|床单被罩","/products/639.html|毯子","/products/640.html|床垫/床褥","/products/641.html|蚊帐","/products/642.html|抱枕靠垫","/products/643.html|毛巾浴巾","/products/644.html|电热毯","/products/645.html|窗帘/窗纱","/products/646.html|布艺软饰","/products/647.html|凉席"]},{"u":"/products/648.html","n":"灯具","i":["/products/649.html|台灯","/products/650.html|节能灯","/products/651.html|装饰灯","/products/652.html|落地灯","/products/653.html|应急灯/手电","/products/654.html|LED灯","/products/655.html|吸顶灯","/products/656.html|五金电器","/products/657.html|筒灯射灯","/products/658.html|吊灯","/products/659.html|氛围照明"]},{"u":"/products/660.html","n":"生活日用","i":["/products/661.html|收纳用品","/products/662.html|雨伞雨具","/products/663.html|浴室用品","/products/664.html|缝纫/针织用品","/products/665.html|洗晒/熨烫","/products/666.html|净化除味"]},{"u":"/products/667.html","n":"家装软饰","i":["/products/668.html|桌布/罩件","/products/669.html|地毯地垫","/products/670.html|沙发垫套/椅垫","/products/671.html|相框/照片墙","/products/672.html|装饰字画","/products/673.html|节庆饰品","/products/674.html|手工/十字绣","/products/675.html|装饰摆件","/products/676.html|保暖防护","/products/677.html|帘艺隔断","/products/678.html|墙贴/装饰贴","/products/679.html|钟饰","/products/680.html|花瓶花艺","/products/681.html|香薰蜡烛","/products/682.html|创意家居"]},{"u":"/products/683.html","n":"清洁用品","i":["/products/684.html|纸品湿巾","/products/685.html|衣物清洁","/products/686.html|清洁工具","/products/687.html|驱虫用品","/products/688.html|家庭清洁","/products/689.html|皮具护理","/products/690.html|一次性用品"]},{"u":"/products/691.html","n":"宠物生活","i":["/products/692.html|宠物主粮","/products/693.html|宠物零食","/products/694.html|医疗保健","/products/695.html|家居日用","/products/696.html|宠物玩具","/products/697.html|出行装备","/products/698.html|洗护美容"]}]},{"u":"/products/699.html","n":"<a href='/products/699.html'>厨具</a>","i":[{"u":"/products/700.html","n":"烹饪锅具","i":["/products/701.html|炒锅","/products/702.html|煎锅","/products/703.html|压力锅","/products/704.html|蒸锅","/products/705.html|汤锅","/products/706.html|奶锅","/products/707.html|锅具套装","/products/708.html|煲类","/products/709.html|水壶","/products/710.html|火锅"]},{"u":"/products/711.html","n":"刀剪菜板","i":["/products/712.html|菜刀","/products/713.html|剪刀","/products/714.html|刀具套装","/products/715.html|砧板","/products/716.html|瓜果刀/刨","/products/717.html|多功能刀"]},{"u":"/products/718.html","n":"厨房配件","i":["/products/719.html|保鲜盒","/products/720.html|烘焙/烧烤","/products/721.html|饭盒/提锅","/products/722.html|储物/置物架","/products/723.html|厨房DIY/小工具"]},{"u":"/products/724.html","n":"水具酒具","i":["/products/725.html|塑料杯","/products/726.html|运动水壶","/products/727.html|玻璃杯","/products/728.html|陶瓷/马克杯","/products/729.html|保温杯","/products/730.html|保温壶","/products/731.html|酒杯/酒具","/products/732.html|杯具套装"]},{"u":"/products/733.html","n":"餐具","i":["/products/734.html|餐具套装","/products/735.html|碗/碟/盘","/products/736.html|筷勺/刀叉","/products/737.html|一次性用品","/products/738.html|果盘/果篮"]},{"u":"/products/739.html","n":"茶具/咖啡具","i":["/products/740.html|整套茶具","/products/741.html|茶杯","/products/742.html|茶壶","/products/743.html|茶盘茶托","/products/744.html|茶叶罐","/products/745.html|茶具配件","/products/746.html|茶宠摆件","/products/747.html|咖啡具","/products/748.html|其他"]}]},{"u":"/products/749.html","n":"<a href='/products/749.html'>服饰内衣</a>","i":[{"u":"/products/750.html","n":"女装","i":["/products/751.html|T恤","/products/752.html|衬衫","/products/753.html|针织衫","/products/754.html|雪纺衫","/products/755.html|卫衣","/products/756.html|马甲","/products/757.html|连衣裙","/products/758.html|半身裙","/products/759.html|牛仔裤","/products/760.html|休闲裤","/products/761.html|打底裤","/products/762.html|正装裤","/products/763.html|小西装","/products/764.html|短外套","/products/765.html|风衣","/products/766.html|毛呢大衣","/products/767.html|真皮皮衣","/products/768.html|棉服","/products/769.html|羽绒服","/products/770.html|大码女装","/products/771.html|中老年女装","/products/772.html|婚纱","/products/773.html|打底衫","/products/774.html|旗袍/唐装","/products/775.html|加绒裤","/products/776.html|吊带/背心","/products/777.html|羊绒衫","/products/778.html|短裤","/products/779.html|皮草","/products/780.html|礼服","/products/781.html|仿皮皮衣","/products/782.html|羊毛衫","/products/783.html|设计师/潮牌"]},{"u":"/products/784.html","n":"男装","i":["/products/785.html|衬衫","/products/786.html|T恤","/products/787.html|POLO衫","/products/788.html|针织衫","/products/789.html|羊绒衫","/products/790.html|卫衣","/products/791.html|马甲/背心","/products/792.html|夹克","/products/793.html|风衣","/products/794.html|毛呢大衣","/products/795.html|仿皮皮衣","/products/796.html|西服","/products/797.html|棉服","/products/798.html|羽绒服","/products/799.html|牛仔裤","/products/800.html|休闲裤","/products/801.html|西裤","/products/802.html|西服套装","/products/803.html|大码男装","/products/804.html|中老年男装","/products/805.html|唐装/中山装","/products/806.html|工装","/products/807.html|真皮皮衣","/products/808.html|加绒裤","/products/809.html|卫裤/运动裤","/products/810.html|短裤","/products/811.html|设计师/潮牌","/products/812.html|羊毛衫"]},{"u":"/products/813.html","n":"内衣","i":["/products/814.html|文胸","/products/815.html|女式内裤","/products/816.html|男式内裤","/products/817.html|睡衣/家居服","/products/818.html|塑身美体","/products/819.html|泳衣","/products/820.html|吊带/背心","/products/821.html|抹胸","/products/822.html|连裤袜/丝袜","/products/823.html|美腿袜","/products/824.html|商务男袜","/products/825.html|保暖内衣","/products/826.html|情侣睡衣","/products/827.html|文胸套装","/products/828.html|少女文胸","/products/829.html|休闲棉袜","/products/830.html|大码内衣","/products/831.html|内衣配件","/products/832.html|打底裤袜","/products/833.html|打底衫","/products/834.html|秋衣秋裤","/products/835.html|情趣内衣"]},{"u":"/products/836.html","n":"服饰配件","i":["/products/837.html|太阳镜","/products/838.html|光学镜架/镜片","/products/839.html|围巾/手套/帽子套装","/products/840.html|袖扣","/products/841.html|棒球帽","/products/842.html|毛线帽","/products/843.html|遮阳帽","/products/844.html|老花镜","/products/845.html|装饰眼镜","/products/846.html|防辐射眼镜","/products/847.html|游泳镜","/products/848.html|女士丝巾/围巾/披肩","/products/849.html|男士丝巾/围巾","/products/850.html|鸭舌帽","/products/851.html|贝雷帽","/products/852.html|礼帽","/products/853.html|真皮手套","/products/854.html|毛线手套","/products/855.html|防晒手套","/products/856.html|男士腰带/礼盒","/products/857.html|女士腰带/礼盒","/products/858.html|钥匙扣","/products/859.html|遮阳伞/雨伞","/products/860.html|口罩","/products/861.html|耳罩/耳包","/products/862.html|假领","/products/863.html|毛线/布面料","/products/864.html|领带/领结/领带夹"]}]}]});
根据调用方法不同,获取的数据最终有细微差异。
最后是数据库信息
SET FOREIGN_KEY_CHECKS=0; -- ---------------------------- -- Table structure for tb_item_cat -- ---------------------------- DROP TABLE IF EXISTS `tb_item_cat`; CREATE TABLE `tb_item_cat` ( `id` bigint(20) NOT NULL AUTO_INCREMENT COMMENT '类目ID', `parent_id` bigint(20) DEFAULT NULL COMMENT '父类目ID=0时,代表的是一级的类目', `name` varchar(50) DEFAULT NULL COMMENT '类目名称', `status` int(1) DEFAULT '1' COMMENT '状态。可选值:1(正常),2(删除)', `sort_order` int(4) DEFAULT NULL COMMENT '排列序号,表示同级类目的展现次序,如数值相等则按名称次序排列。取值范围:大于零的整数', `is_parent` tinyint(1) DEFAULT '1' COMMENT '该类目是否为父类目,1为true,0为false', `created` datetime DEFAULT NULL COMMENT '创建时间', `updated` datetime DEFAULT NULL COMMENT '创建时间', PRIMARY KEY (`id`), KEY `parent_id` (`parent_id`,`status`) USING BTREE, KEY `sort_order` (`sort_order`) ) ENGINE=InnoDB AUTO_INCREMENT=1183 DEFAULT CHARSET=utf8 COMMENT='商品类目'; -- ---------------------------- -- Records of tb_item_cat -- ---------------------------- INSERT INTO `tb_item_cat` VALUES ('1', '0', '图书、音像、电子书刊', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('2', '1', '电子书刊', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('3', '2', '电子书', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('4', '2', '网络原创', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('5', '2', '数字杂志', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('6', '2', '多媒体图书', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('7', '1', '音像', '1', '2', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('8', '7', '音乐', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('9', '7', '影视', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('10', '7', '教育音像', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('11', '1', '英文原版', '1', '3', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('12', '11', '少儿', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('13', '11', '商务投资', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('14', '11', '英语学习与考试', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('15', '11', '小说', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('16', '11', '传记', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('17', '11', '励志', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('18', '1', '文艺', '1', '4', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('19', '18', '小说', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('20', '18', '文学', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('21', '18', '青春文学', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('22', '18', '传记', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('23', '18', '艺术', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('24', '1', '少儿', '1', '5', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('25', '24', '少儿', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('26', '24', '0-2岁', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('27', '24', '3-6岁', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('28', '24', '7-10岁', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('29', '24', '11-14岁', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('30', '1', '人文社科', '1', '6', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('31', '30', '历史', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('32', '30', '哲学', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('33', '30', '国学', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('34', '30', '政治/军事', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('35', '30', '法律', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('36', '30', '宗教', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('37', '30', '心理学', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('38', '30', '文化', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('39', '30', '社会科学', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('40', '1', '经管励志', '1', '7', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('41', '40', '经济', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('42', '40', '金融与投资', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('43', '40', '管理', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('44', '40', '励志与成功', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('45', '1', '生活', '1', '8', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('46', '45', '生活', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('47', '45', '健身与保健', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('48', '45', '家庭与育儿', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('49', '45', '旅游', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('50', '45', '动漫/幽默', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('51', '1', '科技', '1', '9', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('52', '51', '科技', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('53', '51', '工程', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('54', '51', '建筑', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('55', '51', '医学', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('56', '51', '科学与自然', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('57', '51', '计算机与互联网', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('58', '51', '体育/运动', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('59', '1', '教育', '1', '10', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('60', '59', '教材教辅', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('61', '59', '教育与考试', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('62', '59', '外语学习', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('63', '59', '新闻出版', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('64', '59', '语言文字', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('65', '1', '港台图书', '1', '11', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('66', '65', '艺术/设计/收藏', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('67', '65', '经济管理', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('68', '65', '文化/学术', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('69', '65', '少儿文学/国学', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('70', '1', '其它', '1', '12', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('71', '70', '工具书', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('72', '70', '影印版', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('73', '70', '套装书', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('74', '0', '家用电器', '1', '2', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('75', '74', '大 家 电', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('76', '75', '平板电视', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('77', '75', '空调', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('78', '75', '冰箱', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('79', '75', '洗衣机', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('80', '75', '家庭影院', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('81', '75', 'DVD播放机', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('82', '75', '迷你音响', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('83', '75', '烟机/灶具', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('84', '75', '热水器', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('85', '75', '消毒柜/洗碗机', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('86', '75', '酒柜/冰吧/冷柜', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('87', '75', '家电配件', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('88', '75', '家电下乡', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('89', '74', '生活电器', '1', '2', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('90', '89', '电风扇', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('91', '89', '冷风扇', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('92', '89', '净化器', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('93', '89', '饮水机', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('94', '89', '净水设备', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('95', '89', '挂烫机/熨斗', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('96', '89', '吸尘器', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('97', '89', '电话机', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('98', '89', '插座', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('99', '89', '收录/音机', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('100', '89', '清洁机', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('101', '89', '加湿器', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('102', '89', '除湿机', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('103', '89', '取暖电器', '1', '14', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('104', '89', '其它生活电器', '1', '15', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('105', '89', '扫地机器人', '1', '16', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('106', '89', '干衣机', '1', '17', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('107', '89', '生活电器配件', '1', '18', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('108', '74', '厨房电器', '1', '3', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('109', '108', '料理/榨汁机', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('110', '108', '豆浆机', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('111', '108', '电饭煲', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('112', '108', '电压力锅', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('113', '108', '面包机', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('114', '108', '咖啡机', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('115', '108', '微波炉', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('116', '108', '电烤箱', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('117', '108', '电磁炉', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('118', '108', '电饼铛/烧烤盘', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('119', '108', '煮蛋器', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('120', '108', '酸奶机', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('121', '108', '电炖锅', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('122', '108', '电水壶/热水瓶', '1', '14', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('123', '108', '多用途锅', '1', '15', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('124', '108', '果蔬解毒机', '1', '16', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('125', '108', '其它厨房电器', '1', '17', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('126', '74', '个护健康', '1', '4', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('127', '126', '剃须刀', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('128', '126', '剃/脱毛器', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('129', '126', '口腔护理', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('130', '126', '电吹风', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('131', '126', '美容器', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('132', '126', '美发器', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('133', '126', '按摩椅', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('134', '126', '按摩器', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('135', '126', '足浴盆', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('136', '126', '血压计', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('137', '126', '健康秤/厨房秤', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('138', '126', '血糖仪', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('139', '126', '体温计', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('140', '126', '计步器/脂肪检测仪', '1', '14', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('141', '126', '其它健康电器', '1', '15', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('142', '74', '五金家装', '1', '5', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('143', '142', '电动工具', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('144', '142', '手动工具', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('145', '142', '仪器仪表', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('146', '142', '浴霸/排气扇', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('147', '142', '灯具', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('148', '142', 'LED灯', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('149', '142', '洁身器', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('150', '142', '水槽', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('151', '142', '龙头', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('152', '142', '淋浴花洒', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('153', '142', '厨卫五金', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('154', '142', '家具五金', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('155', '142', '门铃', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('156', '142', '电气开关', '1', '14', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('157', '142', '插座', '1', '15', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('158', '142', '电工电料', '1', '16', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('159', '142', '监控安防', '1', '17', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('160', '142', '电线/线缆', '1', '18', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('161', '0', '电脑、办公', '1', '3', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('162', '161', '电脑整机', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('163', '162', '笔记本', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('164', '162', '超极本', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('165', '162', '游戏本', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('166', '162', '平板电脑', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('167', '162', '平板电脑配件', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('168', '162', '台式机', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('169', '162', '服务器/工作站', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('170', '162', '笔记本配件', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('171', '161', '电脑配件', '1', '2', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('172', '171', 'CPU', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('173', '171', '主板', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('174', '171', '显卡', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('175', '171', '硬盘', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('176', '171', 'SSD固态硬盘', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('177', '171', '内存', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('178', '171', '机箱', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('179', '171', '电源', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('180', '171', '显示器', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('181', '171', '刻录机/光驱', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('182', '171', '散热器', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('183', '171', '声卡/扩展卡', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('184', '171', '装机配件', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('185', '171', '组装电脑', '1', '14', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('186', '161', '外设产品', '1', '3', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('187', '186', '移动硬盘', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('188', '186', 'U盘', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('189', '186', '鼠标', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('190', '186', '键盘', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('191', '186', '鼠标垫', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('192', '186', '摄像头', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('193', '186', '手写板', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('194', '186', '外置盒', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('195', '186', '插座', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('196', '186', '线缆', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('197', '186', 'UPS电源', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('198', '186', '电脑工具', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('199', '186', '游戏设备', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('200', '186', '电玩', '1', '14', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('201', '186', '电脑清洁', '1', '15', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('202', '161', '网络产品', '1', '4', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('203', '202', '路由器', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('204', '202', '网卡', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('205', '202', '交换机', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('206', '202', '网络存储', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('207', '202', '4G/3G上网', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('208', '202', '网络盒子', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('209', '202', '网络配件', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('210', '161', '办公设备', '1', '5', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('211', '210', '投影机', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('212', '210', '投影配件', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('213', '210', '多功能一体机', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('214', '210', '打印机', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('215', '210', '传真设备', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('216', '210', '验钞/点钞机', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('217', '210', '扫描设备', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('218', '210', '复合机', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('219', '210', '碎纸机', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('220', '210', '考勤机', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('221', '210', '墨粉', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('222', '210', '收款/POS机', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('223', '210', '会议音频视频', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('224', '210', '保险柜', '1', '14', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('225', '210', '装订/封装机', '1', '15', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('226', '210', '安防监控', '1', '16', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('227', '210', '办公家具', '1', '17', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('228', '210', '白板', '1', '18', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('229', '161', '文具/耗材', '1', '6', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('230', '229', '硒鼓/墨粉', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('231', '229', '墨盒', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('232', '229', '色带', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('233', '229', '纸类', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('234', '229', '办公文具', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('235', '229', '学生文具', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('236', '229', '文件管理', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('237', '229', '财会用品', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('238', '229', '本册/便签', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('239', '229', '计算器', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('240', '229', '激光笔', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('241', '229', '笔类', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('242', '229', '画具画材', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('243', '229', '刻录碟片/附件', '1', '14', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('244', '161', '服务产品', '1', '7', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('245', '244', '上门服务', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('246', '244', '远程服务', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('247', '244', '电脑软件', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('248', '244', '京东服务', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('249', '0', '个护化妆', '1', '4', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('250', '249', '面部护肤', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('251', '250', '清洁', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('252', '250', '护肤', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('253', '250', '面膜', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('254', '250', '剃须', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('255', '250', '套装', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('256', '249', '身体护肤', '1', '2', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('257', '256', '沐浴', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('258', '256', '润肤', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('259', '256', '颈部', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('260', '256', '手足', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('261', '256', '纤体塑形', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('262', '256', '美胸', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('263', '256', '套装', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('264', '249', '口腔护理', '1', '3', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('265', '264', '牙膏/牙粉', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('266', '264', '牙刷/牙线', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('267', '264', '漱口水', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('268', '264', '套装', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('269', '249', '女性护理', '1', '4', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('270', '269', '卫生巾', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('271', '269', '卫生护垫', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('272', '269', '私密护理', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('273', '269', '脱毛膏', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('274', '249', '洗发护发', '1', '5', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('275', '274', '洗发', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('276', '274', '护发', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('277', '274', '染发', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('278', '274', '造型', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('279', '274', '假发', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('280', '274', '套装', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('281', '249', '香水彩妆', '1', '6', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('282', '281', '香水', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('283', '281', '底妆', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('284', '281', '腮红', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('285', '281', '眼部', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('286', '281', '唇部', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('287', '281', '美甲', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('288', '281', '美容工具', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('289', '281', '套装', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('290', '0', '钟表', '1', '5', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('291', '290', '钟表', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('292', '291', '男表', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('293', '291', '女表', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('294', '291', '儿童手表', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('295', '291', '座钟挂钟', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('296', '0', '母婴', '1', '6', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('297', '296', '奶粉', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('298', '297', '婴幼奶粉', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('299', '297', '成人奶粉', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('300', '296', '营养辅食', '1', '2', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('301', '300', '益生菌/初乳', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('302', '300', '米粉/菜粉', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('303', '300', '果泥/果汁', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('304', '300', 'DHA', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('305', '300', '宝宝零食', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('306', '300', '钙铁锌/维生素', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('307', '300', '清火/开胃', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('308', '300', '面条/粥', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('309', '296', '尿裤湿巾', '1', '3', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('310', '309', '婴儿尿裤', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('311', '309', '拉拉裤', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('312', '309', '湿巾', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('313', '309', '成人尿裤', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('314', '296', '喂养用品', '1', '4', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('315', '314', '奶瓶奶嘴', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('316', '314', '吸奶器', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('317', '314', '暖奶消毒', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('318', '314', '碗盘叉勺', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('319', '314', '水壶/水杯', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('320', '314', '牙胶安抚', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('321', '314', '辅食料理机', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('322', '296', '洗护用品', '1', '5', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('323', '322', '宝宝护肤', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('324', '322', '宝宝洗浴', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('325', '322', '奶瓶清洗', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('326', '322', '驱蚊防蚊', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('327', '322', '理发器', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('328', '322', '洗衣液/皂', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('329', '322', '日常护理', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('330', '322', '座便器', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('331', '296', '童车童床', '1', '6', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('332', '331', '婴儿推车', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('333', '331', '餐椅摇椅', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('334', '331', '婴儿床', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('335', '331', '学步车', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('336', '331', '三轮车', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('337', '331', '自行车', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('338', '331', '电动车', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('339', '331', '扭扭车', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('340', '331', '滑板车', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('341', '296', '寝居服饰', '1', '7', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('342', '341', '婴儿外出服', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('343', '341', '婴儿内衣', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('344', '341', '婴儿礼盒', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('345', '341', '婴儿鞋帽袜', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('346', '341', '安全防护', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('347', '341', '家居床品', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('348', '296', '妈妈专区', '1', '8', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('349', '348', '妈咪包/背婴带', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('350', '348', '产后塑身', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('351', '348', '文胸/内裤', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('352', '348', '防辐射服', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('353', '348', '孕妇装', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('354', '348', '孕期营养', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('355', '348', '孕妈美容', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('356', '348', '待产/新生', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('357', '348', '月子装', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('358', '296', '童装童鞋', '1', '9', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('359', '358', '套装', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('360', '358', '上衣', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('361', '358', '裤子', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('362', '358', '裙子', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('363', '358', '内衣/家居服', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('364', '358', '羽绒服/棉服', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('365', '358', '亲子装', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('366', '358', '儿童配饰', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('367', '358', '礼服/演出服', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('368', '358', '运动鞋', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('369', '358', '皮鞋/帆布鞋', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('370', '358', '靴子', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('371', '358', '凉鞋', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('372', '358', '功能鞋', '1', '14', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('373', '358', '户外/运动服', '1', '15', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('374', '296', '安全座椅', '1', '10', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('375', '374', '提篮式', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('376', '374', '安全座椅', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('377', '374', '增高垫', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('378', '0', '食品饮料、保健食品', '1', '7', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('379', '378', '进口食品', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('380', '379', '饼干蛋糕', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('381', '379', '糖果/巧克力', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('382', '379', '休闲零食', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('383', '379', '冲调饮品', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('384', '379', '粮油调味', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('385', '379', '牛奶', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('386', '378', '地方特产', '1', '2', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('387', '386', '其他特产', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('388', '386', '新疆', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('389', '386', '北京', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('390', '386', '山西', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('391', '386', '内蒙古', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('392', '386', '福建', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('393', '386', '湖南', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('394', '386', '四川', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('395', '386', '云南', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('396', '386', '东北', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('397', '378', '休闲食品', '1', '3', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('398', '397', '休闲零食', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('399', '397', '坚果炒货', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('400', '397', '肉干肉脯', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('401', '397', '蜜饯果干', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('402', '397', '糖果/巧克力', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('403', '397', '饼干蛋糕', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('404', '397', '无糖食品', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('405', '378', '粮油调味', '1', '4', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('406', '405', '米面杂粮', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('407', '405', '食用油', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('408', '405', '调味品', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('409', '405', '南北干货', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('410', '405', '方便食品', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('411', '405', '有机食品', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('412', '378', '饮料冲调', '1', '5', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('413', '412', '饮用水', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('414', '412', '饮料', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('415', '412', '牛奶乳品', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('416', '412', '咖啡/奶茶', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('417', '412', '冲饮谷物', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('418', '412', '蜂蜜/柚子茶', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('419', '412', '成人奶粉', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('420', '378', '食品礼券', '1', '6', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('421', '420', '月饼', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('422', '420', '大闸蟹', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('423', '420', '粽子', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('424', '420', '卡券', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('425', '378', '茗茶', '1', '7', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('426', '425', '铁观音', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('427', '425', '普洱', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('428', '425', '龙井', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('429', '425', '绿茶', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('430', '425', '红茶', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('431', '425', '乌龙茶', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('432', '425', '花草茶', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('433', '425', '花果茶', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('434', '425', '养生茶', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('435', '425', '黑茶', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('436', '425', '白茶', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('437', '425', '其它茶', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('438', '0', '汽车用品', '1', '8', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('439', '438', '维修保养', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('440', '439', '润滑油', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('441', '439', '添加剂', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('442', '439', '防冻液', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('443', '439', '滤清器', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('444', '439', '火花塞', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('445', '439', '雨刷', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('446', '439', '车灯', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('447', '439', '后视镜', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('448', '439', '轮胎', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('449', '439', '轮毂', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('450', '439', '刹车片/盘', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('451', '439', '喇叭/皮带', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('452', '439', '蓄电池', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('453', '439', '底盘装甲/护板', '1', '14', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('454', '439', '贴膜', '1', '15', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('455', '439', '汽修工具', '1', '16', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('456', '438', '车载电器', '1', '2', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('457', '456', '导航仪', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('458', '456', '安全预警仪', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('459', '456', '行车记录仪', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('460', '456', '倒车雷达', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('461', '456', '蓝牙设备', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('462', '456', '时尚影音', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('463', '456', '净化器', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('464', '456', '电源', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('465', '456', '冰箱', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('466', '456', '吸尘器', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('467', '438', '美容清洗', '1', '3', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('468', '467', '车蜡', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('469', '467', '补漆笔', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('470', '467', '玻璃水', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('471', '467', '清洁剂', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('472', '467', '洗车工具', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('473', '467', '洗车配件', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('474', '438', '汽车装饰', '1', '4', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('475', '474', '脚垫', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('476', '474', '座垫', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('477', '474', '座套', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('478', '474', '后备箱垫', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('479', '474', '头枕腰靠', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('480', '474', '香水', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('481', '474', '空气净化', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('482', '474', '车内饰品', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('483', '474', '功能小件', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('484', '474', '车身装饰件', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('485', '474', '车衣', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('486', '438', '安全自驾', '1', '5', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('487', '486', '安全座椅', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('488', '486', '胎压充气', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('489', '486', '防盗设备', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('490', '486', '应急救援', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('491', '486', '保温箱', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('492', '486', '储物箱', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('493', '486', '自驾野营', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('494', '486', '摩托车装备', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('495', '0', '玩具乐器', '1', '9', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('496', '495', '适用年龄', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('497', '496', '0-6个月', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('498', '496', '6-12个月', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('499', '496', '1-3岁', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('500', '496', '3-6岁', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('501', '496', '6-14岁', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('502', '496', '14岁以上', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('503', '495', '遥控/电动', '1', '2', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('504', '503', '遥控车', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('505', '503', '遥控飞机', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('506', '503', '遥控船', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('507', '503', '机器人/电动', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('508', '503', '轨道/助力', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('509', '495', '毛绒布艺', '1', '3', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('510', '509', '毛绒/布艺', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('511', '509', '靠垫/抱枕', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('512', '495', '娃娃玩具', '1', '4', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('513', '512', '芭比娃娃', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('514', '512', '卡通娃娃', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('515', '512', '智能娃娃', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('516', '495', '模型玩具', '1', '5', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('517', '516', '仿真模型', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('518', '516', '拼插模型', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('519', '516', '收藏爱好', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('520', '495', '健身玩具', '1', '6', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('521', '520', '炫舞毯', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('522', '520', '爬行垫/毯', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('523', '520', '户外玩具', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('524', '520', '戏水玩具', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('525', '495', '动漫玩具', '1', '7', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('526', '525', '电影周边', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('527', '525', '卡通周边', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('528', '525', '网游周边', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('529', '495', '益智玩具', '1', '8', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('530', '529', '摇铃/床铃', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('531', '529', '健身架', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('532', '529', '早教启智', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('533', '529', '拖拉玩具', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('534', '495', '积木拼插', '1', '9', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('535', '534', '积木', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('536', '534', '拼图', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('537', '534', '磁力棒', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('538', '534', '立体拼插', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('539', '495', 'DIY玩具', '1', '10', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('540', '539', '手工彩泥', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('541', '539', '绘画工具', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('542', '539', '情景玩具', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('543', '495', '创意减压', '1', '11', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('544', '543', '减压玩具', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('545', '543', '创意玩具', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('546', '495', '乐器相关', '1', '12', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('547', '546', '钢琴', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('548', '546', '电子琴', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('549', '546', '手风琴', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('550', '546', '吉他/贝斯', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('551', '546', '民族管弦乐器', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('552', '546', '西洋管弦乐', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('553', '546', '口琴/口风琴/竖笛', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('554', '546', '西洋打击乐器', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('555', '546', '各式乐器配件', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('556', '546', '电脑音乐', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('557', '546', '工艺礼品乐器', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('558', '0', '手机', '1', '10', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('559', '558', '手机通讯', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('560', '559', '手机', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('561', '559', '对讲机', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('562', '558', '运营商', '1', '2', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('563', '562', '购机送费', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('564', '562', '“0”元购机', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('565', '562', '选号中心', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('566', '562', '选号入网', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('567', '558', '手机配件', '1', '3', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('568', '567', '手机电池', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('569', '567', '蓝牙耳机', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('570', '567', '充电器/数据线', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('571', '567', '手机耳机', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('572', '567', '手机贴膜', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('573', '567', '手机存储卡', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('574', '567', '手机保护套', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('575', '567', '车载配件', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('576', '567', 'iPhone 配件', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('577', '567', '创意配件', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('578', '567', '便携/无线音响', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('579', '567', '手机饰品', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('580', '0', '数码', '1', '11', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('581', '580', '摄影摄像', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('582', '581', '数码相机', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('583', '581', '单电/微单相机', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('584', '581', '单反相机', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('585', '581', '摄像机', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('586', '581', '拍立得', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('587', '581', '运动相机', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('588', '581', '镜头', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('589', '581', '户外器材', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('590', '581', '影棚器材', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('591', '580', '数码配件', '1', '2', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('592', '591', '存储卡', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('593', '591', '读卡器', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('594', '591', '滤镜', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('595', '591', '闪光灯/手柄', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('596', '591', '相机包', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('597', '591', '三脚架/云台', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('598', '591', '相机清洁', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('599', '591', '相机贴膜', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('600', '591', '机身附件', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('601', '591', '镜头附件', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('602', '591', '电池/充电器', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('603', '591', '移动电源', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('604', '580', '智能设备', '1', '3', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('605', '604', '智能手环', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('606', '604', '智能手表', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('607', '604', '智能眼镜', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('608', '604', '运动跟踪器', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('609', '604', '健康监测', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('610', '604', '智能配饰', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('611', '604', '智能家居', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('612', '604', '体感车', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('613', '604', '其他配件', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('614', '580', '时尚影音', '1', '4', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('615', '614', 'MP3/MP4', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('616', '614', '智能设备', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('617', '614', '耳机/耳麦', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('618', '614', '音箱', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('619', '614', '高清播放器', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('620', '614', 'MP3/MP4配件', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('621', '614', '麦克风', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('622', '614', '专业音频', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('623', '614', '数码相框', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('624', '614', '苹果配件', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('625', '580', '电子教育', '1', '5', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('626', '625', '电子词典', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('627', '625', '电纸书', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('628', '625', '录音笔', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('629', '625', '复读机', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('630', '625', '点读机/笔', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('631', '625', '学生平板', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('632', '625', '早教机', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('633', '0', '家居家装', '1', '12', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('634', '633', '家纺', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('635', '634', '床品套件', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('636', '634', '被子', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('637', '634', '枕芯', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('638', '634', '床单被罩', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('639', '634', '毯子', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('640', '634', '床垫/床褥', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('641', '634', '蚊帐', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('642', '634', '抱枕靠垫', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('643', '634', '毛巾浴巾', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('644', '634', '电热毯', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('645', '634', '窗帘/窗纱', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('646', '634', '布艺软饰', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('647', '634', '凉席', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('648', '633', '灯具', '1', '2', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('649', '648', '台灯', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('650', '648', '节能灯', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('651', '648', '装饰灯', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('652', '648', '落地灯', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('653', '648', '应急灯/手电', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('654', '648', 'LED灯', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('655', '648', '吸顶灯', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('656', '648', '五金电器', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('657', '648', '筒灯射灯', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('658', '648', '吊灯', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('659', '648', '氛围照明', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('660', '633', '生活日用', '1', '3', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('661', '660', '收纳用品', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('662', '660', '雨伞雨具', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('663', '660', '浴室用品', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('664', '660', '缝纫/针织用品', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('665', '660', '洗晒/熨烫', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('666', '660', '净化除味', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('667', '633', '家装软饰', '1', '4', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('668', '667', '桌布/罩件', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('669', '667', '地毯地垫', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('670', '667', '沙发垫套/椅垫', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('671', '667', '相框/照片墙', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('672', '667', '装饰字画', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('673', '667', '节庆饰品', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('674', '667', '手工/十字绣', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('675', '667', '装饰摆件', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('676', '667', '保暖防护', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('677', '667', '帘艺隔断', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('678', '667', '墙贴/装饰贴', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('679', '667', '钟饰', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('680', '667', '花瓶花艺', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('681', '667', '香薰蜡烛', '1', '14', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('682', '667', '创意家居', '1', '15', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('683', '633', '清洁用品', '1', '5', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('684', '683', '纸品湿巾', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('685', '683', '衣物清洁', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('686', '683', '清洁工具', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('687', '683', '驱虫用品', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('688', '683', '家庭清洁', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('689', '683', '皮具护理', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('690', '683', '一次性用品', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('691', '633', '宠物生活', '1', '6', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('692', '691', '宠物主粮', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('693', '691', '宠物零食', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('694', '691', '医疗保健', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('695', '691', '家居日用', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('696', '691', '宠物玩具', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('697', '691', '出行装备', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('698', '691', '洗护美容', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('699', '0', '厨具', '1', '13', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('700', '699', '烹饪锅具', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('701', '700', '炒锅', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('702', '700', '煎锅', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('703', '700', '压力锅', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('704', '700', '蒸锅', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('705', '700', '汤锅', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('706', '700', '奶锅', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('707', '700', '锅具套装', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('708', '700', '煲类', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('709', '700', '水壶', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('710', '700', '火锅', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('711', '699', '刀剪菜板', '1', '2', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('712', '711', '菜刀', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('713', '711', '剪刀', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('714', '711', '刀具套装', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('715', '711', '砧板', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('716', '711', '瓜果刀/刨', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('717', '711', '多功能刀', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('718', '699', '厨房配件', '1', '3', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('719', '718', '保鲜盒', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('720', '718', '烘焙/烧烤', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('721', '718', '饭盒/提锅', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('722', '718', '储物/置物架', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('723', '718', '厨房DIY/小工具', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('724', '699', '水具酒具', '1', '4', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('725', '724', '塑料杯', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('726', '724', '运动水壶', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('727', '724', '玻璃杯', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('728', '724', '陶瓷/马克杯', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('729', '724', '保温杯', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('730', '724', '保温壶', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('731', '724', '酒杯/酒具', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('732', '724', '杯具套装', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('733', '699', '餐具', '1', '5', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('734', '733', '餐具套装', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('735', '733', '碗/碟/盘', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('736', '733', '筷勺/刀叉', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('737', '733', '一次性用品', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('738', '733', '果盘/果篮', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('739', '699', '茶具/咖啡具', '1', '6', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('740', '739', '整套茶具', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('741', '739', '茶杯', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('742', '739', '茶壶', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('743', '739', '茶盘茶托', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('744', '739', '茶叶罐', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('745', '739', '茶具配件', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('746', '739', '茶宠摆件', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('747', '739', '咖啡具', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('748', '739', '其他', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('749', '0', '服饰内衣', '1', '14', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('750', '749', '女装', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('751', '750', 'T恤', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('752', '750', '衬衫', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('753', '750', '针织衫', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('754', '750', '雪纺衫', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('755', '750', '卫衣', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('756', '750', '马甲', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('757', '750', '连衣裙', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('758', '750', '半身裙', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('759', '750', '牛仔裤', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('760', '750', '休闲裤', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('761', '750', '打底裤', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('762', '750', '正装裤', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('763', '750', '小西装', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('764', '750', '短外套', '1', '14', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('765', '750', '风衣', '1', '15', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('766', '750', '毛呢大衣', '1', '16', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('767', '750', '真皮皮衣', '1', '17', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('768', '750', '棉服', '1', '18', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('769', '750', '羽绒服', '1', '19', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('770', '750', '大码女装', '1', '20', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('771', '750', '中老年女装', '1', '21', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('772', '750', '婚纱', '1', '22', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('773', '750', '打底衫', '1', '23', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('774', '750', '旗袍/唐装', '1', '24', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('775', '750', '加绒裤', '1', '25', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('776', '750', '吊带/背心', '1', '26', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('777', '750', '羊绒衫', '1', '27', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('778', '750', '短裤', '1', '28', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('779', '750', '皮草', '1', '29', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('780', '750', '礼服', '1', '30', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('781', '750', '仿皮皮衣', '1', '31', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('782', '750', '羊毛衫', '1', '32', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('783', '750', '设计师/潮牌', '1', '33', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('784', '749', '男装', '1', '2', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('785', '784', '衬衫', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('786', '784', 'T恤', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('787', '784', 'POLO衫', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('788', '784', '针织衫', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('789', '784', '羊绒衫', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('790', '784', '卫衣', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('791', '784', '马甲/背心', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('792', '784', '夹克', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('793', '784', '风衣', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('794', '784', '毛呢大衣', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('795', '784', '仿皮皮衣', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('796', '784', '西服', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('797', '784', '棉服', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('798', '784', '羽绒服', '1', '14', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('799', '784', '牛仔裤', '1', '15', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('800', '784', '休闲裤', '1', '16', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('801', '784', '西裤', '1', '17', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('802', '784', '西服套装', '1', '18', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('803', '784', '大码男装', '1', '19', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('804', '784', '中老年男装', '1', '20', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('805', '784', '唐装/中山装', '1', '21', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('806', '784', '工装', '1', '22', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('807', '784', '真皮皮衣', '1', '23', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('808', '784', '加绒裤', '1', '24', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('809', '784', '卫裤/运动裤', '1', '25', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('810', '784', '短裤', '1', '26', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('811', '784', '设计师/潮牌', '1', '27', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('812', '784', '羊毛衫', '1', '28', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('813', '749', '内衣', '1', '3', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('814', '813', '文胸', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('815', '813', '女式内裤', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('816', '813', '男式内裤', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('817', '813', '睡衣/家居服', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('818', '813', '塑身美体', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('819', '813', '泳衣', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('820', '813', '吊带/背心', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('821', '813', '抹胸', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('822', '813', '连裤袜/丝袜', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('823', '813', '美腿袜', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('824', '813', '商务男袜', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('825', '813', '保暖内衣', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('826', '813', '情侣睡衣', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('827', '813', '文胸套装', '1', '14', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('828', '813', '少女文胸', '1', '15', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('829', '813', '休闲棉袜', '1', '16', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('830', '813', '大码内衣', '1', '17', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('831', '813', '内衣配件', '1', '18', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('832', '813', '打底裤袜', '1', '19', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('833', '813', '打底衫', '1', '20', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('834', '813', '秋衣秋裤', '1', '21', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('835', '813', '情趣内衣', '1', '22', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('836', '749', '服饰配件', '1', '4', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('837', '836', '太阳镜', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('838', '836', '光学镜架/镜片', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('839', '836', '围巾/手套/帽子套装', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('840', '836', '袖扣', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('841', '836', '棒球帽', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('842', '836', '毛线帽', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('843', '836', '遮阳帽', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('844', '836', '老花镜', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('845', '836', '装饰眼镜', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('846', '836', '防辐射眼镜', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('847', '836', '游泳镜', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('848', '836', '女士丝巾/围巾/披肩', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('849', '836', '男士丝巾/围巾', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('850', '836', '鸭舌帽', '1', '14', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('851', '836', '贝雷帽', '1', '15', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('852', '836', '礼帽', '1', '16', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('853', '836', '真皮手套', '1', '17', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('854', '836', '毛线手套', '1', '18', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('855', '836', '防晒手套', '1', '19', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('856', '836', '男士腰带/礼盒', '1', '20', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('857', '836', '女士腰带/礼盒', '1', '21', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('858', '836', '钥匙扣', '1', '22', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('859', '836', '遮阳伞/雨伞', '1', '23', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('860', '836', '口罩', '1', '24', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('861', '836', '耳罩/耳包', '1', '25', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('862', '836', '假领', '1', '26', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('863', '836', '毛线/布面料', '1', '27', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('864', '836', '领带/领结/领带夹', '1', '28', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('865', '0', '鞋靴', '1', '15', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('866', '865', '流行男鞋', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('867', '866', '商务休闲鞋', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('868', '866', '正装鞋', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('869', '866', '休闲鞋', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('870', '866', '凉鞋/沙滩鞋', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('871', '866', '男靴', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('872', '866', '功能鞋', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('873', '866', '拖鞋/人字拖', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('874', '866', '雨鞋/雨靴', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('875', '866', '传统布鞋', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('876', '866', '鞋配件', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('877', '866', '帆布鞋', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('878', '866', '增高鞋', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('879', '866', '工装鞋', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('880', '866', '定制鞋', '1', '14', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('881', '865', '时尚女鞋', '1', '2', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('882', '881', '高跟鞋', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('883', '881', '单鞋', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('884', '881', '休闲鞋', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('885', '881', '凉鞋', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('886', '881', '女靴', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('887', '881', '雪地靴', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('888', '881', '拖鞋/人字拖', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('889', '881', '踝靴', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('890', '881', '筒靴', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('891', '881', '帆布鞋', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('892', '881', '雨鞋/雨靴', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('893', '881', '妈妈鞋', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('894', '881', '鞋配件', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('895', '881', '特色鞋', '1', '14', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('896', '881', '鱼嘴鞋', '1', '15', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('897', '881', '布鞋/绣花鞋', '1', '16', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('898', '881', '马丁靴', '1', '17', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('899', '881', '坡跟鞋', '1', '18', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('900', '881', '松糕鞋', '1', '19', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('901', '881', '内增高', '1', '20', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('902', '881', '防水台', '1', '21', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('903', '0', '礼品箱包', '1', '16', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('904', '903', '潮流女包', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('905', '904', '钱包', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('906', '904', '手拿包/晚宴包', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('907', '904', '单肩包', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('908', '904', '双肩包', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('909', '904', '手提包', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('910', '904', '斜挎包', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('911', '904', '钥匙包', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('912', '904', '卡包/零钱包', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('913', '903', '精品男包', '1', '2', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('914', '913', '钱包/卡包', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('915', '913', '男士手包', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('916', '913', '商务公文包', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('917', '913', '双肩包', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('918', '913', '单肩/斜挎包', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('919', '913', '钥匙包', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('920', '903', '功能箱包', '1', '3', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('921', '920', '电脑包', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('922', '920', '拉杆箱/拉杆包', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('923', '920', '旅行包', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('924', '920', '旅行配件', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('925', '920', '休闲运动包', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('926', '920', '登山包', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('927', '920', '妈咪包', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('928', '920', '书包', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('929', '920', '相机包', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('930', '920', '腰包/胸包', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('931', '903', '礼品', '1', '4', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('932', '931', '火机烟具', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('933', '931', '礼品文具', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('934', '931', '军刀军具', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('935', '931', '收藏品', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('936', '931', '工艺礼品', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('937', '931', '创意礼品', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('938', '931', '礼盒礼券', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('939', '931', '鲜花绿植', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('940', '931', '婚庆用品', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('941', '931', '京东卡', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('942', '931', '美妆礼品', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('943', '931', '地方礼品', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('944', '931', '古董把玩', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('945', '903', '奢侈品', '1', '5', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('946', '945', '箱包', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('947', '945', '钱包', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('948', '945', '服饰', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('949', '945', '腰带', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('950', '945', '太阳镜/眼镜框', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('951', '945', '配件', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('952', '945', '鞋靴', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('953', '945', '饰品', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('954', '945', '名品腕表', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('955', '945', '高档化妆品', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('956', '903', '婚庆', '1', '6', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('957', '956', '婚嫁首饰', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('958', '956', '婚纱摄影', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('959', '956', '婚纱礼服', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('960', '956', '婚庆服务', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('961', '956', '婚庆礼品/用品', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('962', '956', '婚宴', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('963', '0', '珠宝', '1', '17', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('964', '963', '时尚饰品', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('965', '964', '项链', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('966', '964', '手链/脚链', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('967', '964', '戒指', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('968', '964', '耳饰', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('969', '964', '头饰', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('970', '964', '胸针', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('971', '964', '婚庆饰品', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('972', '964', '饰品配件', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('973', '963', '纯金K金饰品', '1', '2', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('974', '973', '吊坠/项链', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('975', '973', '手镯/手链/脚链', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('976', '973', '戒指', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('977', '973', '耳饰', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('978', '963', '金银投资', '1', '3', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('979', '978', '工艺金', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('980', '978', '工艺银', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('981', '963', '银饰', '1', '4', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('982', '981', '吊坠/项链', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('983', '981', '手镯/手链/脚链', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('984', '981', '戒指/耳饰', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('985', '981', '宝宝银饰', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('986', '981', '千足银手镯', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('987', '963', '钻石', '1', '5', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('988', '987', '裸钻', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('989', '987', '戒指', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('990', '987', '项链/吊坠', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('991', '987', '耳饰', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('992', '987', '手镯/手链', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('993', '963', '翡翠玉石', '1', '6', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('994', '993', '项链/吊坠', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('995', '993', '手镯/手串', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('996', '993', '戒指', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('997', '993', '耳饰', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('998', '993', '挂件/摆件/把件', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('999', '993', '高值收藏', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1000', '963', '水晶玛瑙', '1', '7', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1001', '1000', '项链/吊坠', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1002', '1000', '耳饰', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1003', '1000', '手镯/手链/脚链', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1004', '1000', '戒指', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1005', '1000', '头饰/胸针', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1006', '1000', '摆件/挂件', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1007', '963', '彩宝', '1', '8', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1008', '1007', '项链/吊坠', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1009', '1007', '耳饰', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1010', '1007', '手镯/手链', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1011', '1007', '戒指', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1012', '963', '铂金', '1', '9', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1013', '1012', '项链/吊坠', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1014', '1012', '手镯/手链/脚链', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1015', '1012', '戒指', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1016', '1012', '耳饰', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1017', '963', '天然木饰', '1', '10', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1018', '1017', '小叶紫檀', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1019', '1017', '黄花梨', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1020', '1017', '沉香', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1021', '1017', '金丝楠', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1022', '1017', '菩提', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1023', '1017', '其他', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1024', '963', '珍珠', '1', '11', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1025', '1024', '项链', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1026', '1024', '吊坠', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1027', '1024', '耳饰', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1028', '1024', '手链', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1029', '1024', '戒指', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1030', '1024', '胸针', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1031', '0', '运动健康', '1', '18', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1032', '1031', '运动鞋包', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1033', '1032', '休闲鞋', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1034', '1032', '板鞋', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1035', '1032', '帆布鞋', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1036', '1032', '专项运动鞋', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1037', '1032', '跑步鞋', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1038', '1032', '篮球鞋', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1039', '1032', '足球鞋', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1040', '1032', '训练鞋', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1041', '1032', '乒羽网鞋', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1042', '1032', '拖鞋', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1043', '1032', '运动包', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1044', '1031', '运动服饰', '1', '2', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1045', '1044', '运动配饰', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1046', '1044', '羽绒服', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1047', '1044', '毛衫/线衫', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1048', '1044', '乒羽网服', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1049', '1044', '训练服', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1050', '1044', '运动背心', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1051', '1044', '卫衣/套头衫', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1052', '1044', '夹克/风衣', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1053', '1044', 'T恤', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1054', '1044', '棉服', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1055', '1044', '运动裤', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1056', '1044', '套装', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1057', '1031', '骑行运动', '1', '3', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1058', '1057', '折叠车', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1059', '1057', '山地车/公路车', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1060', '1057', '电动车', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1061', '1057', '其他整车', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1062', '1057', '骑行服', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1063', '1057', '骑行装备', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1064', '1031', '垂钓用品', '1', '4', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1065', '1064', '鱼竿鱼线', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1066', '1064', '浮漂鱼饵', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1067', '1064', '钓鱼桌椅', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1068', '1064', '钓鱼配件', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1069', '1064', '钓箱鱼包', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1070', '1064', '其它', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1071', '1031', '游泳用品', '1', '5', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1072', '1071', '泳镜', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1073', '1071', '泳帽', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1074', '1071', '游泳包防水包', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1075', '1071', '女士泳衣', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1076', '1071', '男士泳衣', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1077', '1071', '比基尼', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1078', '1071', '其它', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1079', '1031', '户外鞋服', '1', '6', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1080', '1079', '冲锋衣裤', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1081', '1079', '速干衣裤', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1082', '1079', '滑雪服', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1083', '1079', '羽绒服/棉服', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1084', '1079', '休闲衣裤', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1085', '1079', '抓绒衣裤', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1086', '1079', '软壳衣裤', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1087', '1079', 'T恤', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1088', '1079', '户外风衣', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1089', '1079', '功能内衣', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1090', '1079', '军迷服饰', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1091', '1079', '登山鞋', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1092', '1079', '雪地靴', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1093', '1079', '徒步鞋', '1', '14', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1094', '1079', '越野跑鞋', '1', '15', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1095', '1079', '休闲鞋', '1', '16', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1096', '1079', '工装鞋', '1', '17', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1097', '1079', '溯溪鞋', '1', '18', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1098', '1079', '沙滩/凉拖', '1', '19', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1099', '1079', '户外袜', '1', '20', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1100', '1031', '户外装备', '1', '7', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1101', '1100', '帐篷/垫子', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1102', '1100', '睡袋/吊床', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1103', '1100', '登山攀岩', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1104', '1100', '背包', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1105', '1100', '户外配饰', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1106', '1100', '户外照明', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1107', '1100', '户外仪表', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1108', '1100', '户外工具', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1109', '1100', '望远镜', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1110', '1100', '旅游用品', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1111', '1100', '便携桌椅床', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1112', '1100', '野餐烧烤', '1', '12', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1113', '1100', '军迷用品', '1', '13', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1114', '1100', '救援装备', '1', '14', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1115', '1100', '滑雪装备', '1', '15', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1116', '1100', '极限户外', '1', '16', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1117', '1100', '冲浪潜水', '1', '17', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1118', '1031', '健身训练', '1', '8', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1119', '1118', '综合训练器', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1120', '1118', '其他大型器械', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1121', '1118', '哑铃', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1122', '1118', '仰卧板/收腹机', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1123', '1118', '其他中小型器材', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1124', '1118', '瑜伽舞蹈', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1125', '1118', '武术搏击', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1126', '1118', '健身车/动感单车', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1127', '1118', '跑步机', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1128', '1118', '运动护具', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1129', '1031', '纤体瑜伽', '1', '9', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1130', '1129', '瑜伽垫', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1131', '1129', '瑜伽服', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1132', '1129', '瑜伽配件', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1133', '1129', '瑜伽套装', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1134', '1129', '舞蹈鞋服', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1135', '1031', '体育用品', '1', '10', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1136', '1135', '羽毛球', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1137', '1135', '乒乓球', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1138', '1135', '篮球', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1139', '1135', '足球', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1140', '1135', '网球', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1141', '1135', '排球', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1142', '1135', '高尔夫', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1143', '1135', '台球', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1144', '1135', '棋牌麻将', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1145', '1135', '轮滑滑板', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1146', '1135', '其他', '1', '11', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1147', '0', '彩票、旅行、充值、票务', '1', '19', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1148', '1147', '彩票', '1', '1', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1149', '1148', '双色球', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1150', '1148', '大乐透', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1151', '1148', '福彩3D', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1152', '1148', '排列三', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1153', '1148', '排列五', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1154', '1148', '七星彩', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1155', '1148', '七乐彩', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1156', '1148', '竞彩足球', '1', '8', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1157', '1148', '竞彩篮球', '1', '9', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1158', '1148', '新时时彩', '1', '10', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1159', '1147', '机票', '1', '2', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1160', '1159', '国内机票', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1161', '1147', '酒店', '1', '3', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1162', '1161', '国内酒店', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1163', '1161', '酒店团购', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1164', '1147', '旅行', '1', '4', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1165', '1164', '度假', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1166', '1164', '景点', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1167', '1164', '租车', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1168', '1164', '火车票', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1169', '1164', '旅游团购', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1170', '1147', '充值', '1', '5', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1171', '1170', '手机充值', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1172', '1147', '游戏', '1', '6', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1173', '1172', '游戏点卡', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1174', '1172', 'QQ充值', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1175', '1147', '票务', '1', '7', '1', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1176', '1175', '电影票', '1', '1', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1177', '1175', '演唱会', '1', '2', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1178', '1175', '话剧歌剧', '1', '3', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1179', '1175', '音乐会', '1', '4', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1180', '1175', '体育赛事', '1', '5', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1181', '1175', '舞蹈芭蕾', '1', '6', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55'); INSERT INTO `tb_item_cat` VALUES ('1182', '1175', '戏曲综艺', '1', '7', '0', '2014-10-15 18:31:55', '2014-10-15 18:31:55');
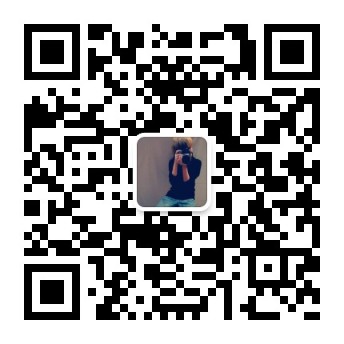