C++ 算法竞赛、01 周赛篇 | AcWing 第1场周赛
AcWing 第1场周赛
3577 选择数字
朴素
暴力两层循环
#include <cstdio>
#include <iostream>
#include <unordered_set>
using namespace std;
const int N = 101;
int a[N], b[N];
int main() {
int n, m;
cin >> n;
unordered_set<int> s;
for (int i = 0; i < n; i++) {
cin >> a[i];
s.insert(a[i]);
}
cin >> m;
for (int i = 0; i < m; i++) {
cin >> b[i];
s.insert(b[i]);
}
// ^ 两层遍历
for (int i = 0; i < n; i++)
for (int k = 0; k < m; k++) {
if (!s.count(a[i] + b[k])) {
cout << a[i] << " " << b[k] << endl;
return 0;
}
}
return 0;
}
优美
两个数组的最大值相加一定是新数
#include <algorithm>
#include <cstdio>
#include <iostream>
using namespace std;
const int N = 101;
int a[N], b[N];
int main() {
int n, m, a_max = 0, b_max = 0;
cin >> n;
for (int i = 0; i < n; i++) {
cin >> a[i];
a_max = max(a[i], a_max);
}
cin >> m;
for (int i = 0; i < m; i++) {
cin >> b[i];
b_max = max(b[i], b_max);
}
cout << a_max << " " << b_max;
return 0;
}
3578 ⭐最大中位数
整数二分问题。求中位数,并依次递增,计算所需的操作次数。求最后一个操作次数总和 <= k 的中位数值
- 如果 mid - a[i] < 0 ,意味着该数比中位数大,不需要操作
- int 范围 2.174e9,二分过程中计算 (l+r) 可能超过 2.174e9,改用 long 存储
#include <algorithm>
#include <iostream>
using namespace std;
const int N = 2e5 + 10;
int a[N], n;
long k;
long check(long mid) {
long res = 0;
for (int i = n >> 1; i < n; i++) {
res += (mid - a[i] > 0 ? mid - a[i] : 0);
}
return res;
}
int main() {
cin >> n >> k;
for (int i = 0; i < n; i++) scanf("%d", &a[i]);
sort(a, a + n);
long l = a[n >> 1], r = 2e9;
while (l < r) {
long mid = l + r + 1 >> 1;
if (check(mid) <= k)
l = mid;
else
r = mid - 1;
}
cout << l;
return 0;
}
2579 ⭐⭐数字移动
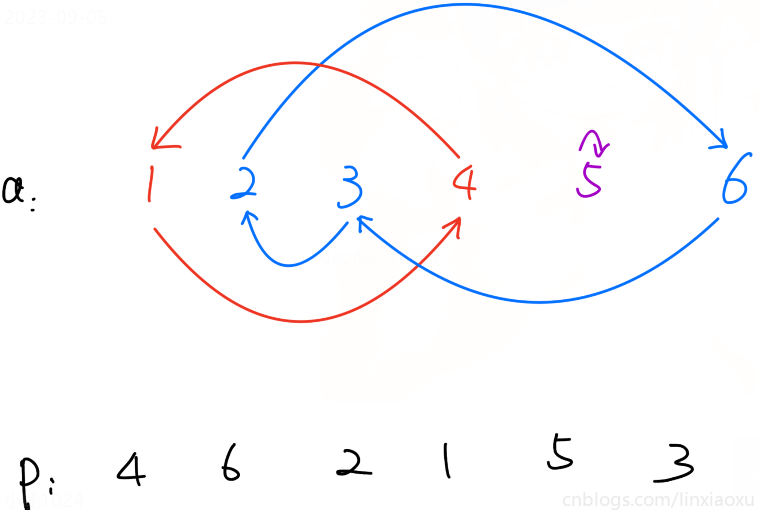
每个点的出度和入度都为 1,每组交换都会形成一个闭环。每个环的节点数就是每个元素回到原来位置需要经过的交换次数
采用并查集维护各个连通块(环)的连通性,然后在并查集的根节点额外维护该并查集的节点个数
难点
- 合并两个根节点的时候,把其中一个根节点维护的并查集元素数量s[pu]累加到另一个并查集的根节点中
- 最后输出包含 i 的连通块数量,也就是 s[find(i)]
#include <algorithm>
#include <cstring>
#include <iostream>
using namespace std;
const int N = 2e5 + 10;
int p[N], s[N]; // s 记录每个连通块的数量
int t, n;
int find(int u) {
if (p[u] != u) p[u] = find(p[u]);
return p[u];
}
int main() {
cin.tie(0);
cin >> t;
while (t--) {
cin >> n;
for (int i = 1; i <= n; i++) {
p[i] = i;
s[i] = 1;
}
for (int u = 1; u <= n; u++) {
int x;
cin >> x;
int px = find(x), pu = find(u);
if (px != pu) { // 使px跟pu在同一个连通块
s[px] += s[pu];
p[pu] = px;
}
}
for (int i = 1; i <= n; i++) {
cout << s[find(i)] << " ";
}
cout << endl;
}
return 0;
}