vue2 中的实现
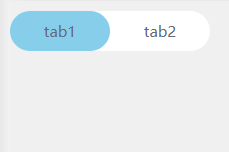
<template>
<div>
<div class="switch-box" :style="{ '--translatex': translatex }">
<div
v-for="(item, index) in type"
:key="index"
@click="switchType(index)"
>
{{ item.name }}
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
translatex: "0px",
type: [{ name: "tab1" }, { name: "tab2" }],
};
},
methods: {
switchType(index) {
if (index === 0) {
this.translatex = `0px`;
} else if (index === 1) {
this.translatex = `100px`;
}
},
},
};
</script>
<style lang="less" scoped>
.switch-box {
width: 200px;
height: 40px;
border-radius: 20px;
display: flex;
background-color: white;
> div {
width: 100px;
height: 40px;
line-height: 40px;
border-radius: 20px;
text-align: center;
cursor: pointer;
z-index: 1;
}
position: relative;
}
.switch-box::after {
content: "";
position: absolute;
background-color: skyblue;
border-radius: 20px;
width: 100px;
height: 100%;
transform: translateX(var(--translatex));
transition: all 0.3s cubic-bezier(0.645, 0.045, 0.355, 1);
z-index: 0;
}
</style>
vue3 组件式(composition)中的实现
<script setup>
import Home from './Home.vue'
import Posts from './Posts.vue'
import Archive from './Archive.vue'
import { ref } from 'vue'
const currentTab = ref('Home')
const tabs = {
Home,
Posts,
Archive
}
</script>
<template>
<div class="demo">
<button
v-for="(_, tab) in tabs"
:key="tab"
:class="['tab-button', { active: currentTab === tab }]"
@click="currentTab = tab"
>
{{ tab }}
</button>
<component :is="tabs[currentTab]" class="tab"></component>
</div>
</template>
<style>
.demo {
font-family: sans-serif;
border: 1px solid #eee;
border-radius: 2px;
padding: 20px 30px;
margin-top: 1em;
margin-bottom: 40px;
user-select: none;
overflow-x: auto;
}
.tab-button {
padding: 6px 10px;
border-top-left-radius: 3px;
border-top-right-radius: 3px;
border: 1px solid #ccc;
cursor: pointer;
background: #f0f0f0;
margin-bottom: -1px;
margin-right: -1px;
}
.tab-button:hover {
background: #e0e0e0;
}
.tab-button.active {
background: #e0e0e0;
}
.tab {
border: 1px solid #ccc;
padding: 10px;
}
</style>
https://cn.vuejs.org/guide/essentials/component-basics.html#dynamic-components:~:text=中查看示例-,上面的例子是通过 Vue 的,-<component> 元素