[数据结构]数组与字符串
一.1.1 数组和字符串
1.1.1 一维数组的倒置
#include<stdio.h>
#define M 20
void fun(int *x,int n)
{
int *p,m=n/2,*i,*j;
i=x;
j=x+n-1;
p=x+m;
for(;i<p;i++,j--)
{
int t=*i;
*i=*j;
*j=t;
}
}
int main()
{
int i,a[M],n; //n为输入位数
printf("Enter n:\n");
scanf("%d",&n);
printf("The original array:\n"); //输入原始数据到数组中
for(i=0;i<n;i++)
scanf("%d",a+i);
fun(a,i);
printf("\nThe array inverted:\n"); //数组翻转
for(i=0;i<n;i++)
printf("%d ",*(a+i));
}
运行结果
1.1.2 一维数组应用
#include"stdio.h"
void main()
{
int Employee[10]={27000,32000,32500,27500,30000,29000,31000,32500,30000,26000};
int Index;
int NewSalary;
int Selection;
while(1)
{
printf("===================================================\n");
printf("=Simple Employee Salary Management System =\n");
printf("=1.Display employee salary =\n");
printf("=2.Modify employee salary =\n");
printf("=3.Quit =\n");
printf("Please input your choose:");
scanf("%d",&Selection);
if(Selection==1||Selection==2)
{
printf("**Please input the employee number:");
scanf("%d",&Index);
if(Index<10)
{
printf("**Employee Number is %d.",Index);
printf("The Salary is %d\n",Employee[Index]);
}
else
{
printf("##The error employee number!\n");
exit(1);
}
}
switch(Selection)
{
case 1:
break;
case 2:
printf("**Please input new salary:");
scanf("%d",&NewSalary);
Employee[Index]=NewSalary;
break;
case 3:
exit(1);
break;
}
printf("\n");
}
}
运行结果
1.1.3 一维数组的高级应用
#include"stdio.h"
int main()
{
int Data[40];
int Digit;
int i,j,r,k;
int N;
for(i=1;i<41;i++)
Data[i]=0;
Data[0]=1;
Data[1]=1;
Digit=1;
printf("Enter a number what you want to calculus:");
scanf("%d",&N);
for(i=1;i<N+1;i++)
{
for(j=1;j<Digit+1;j++)
Data[j]*=i;
for(j=1;j<Digit+1;j++)
{
if(Data[j]>10)
{
for(r=1;r<Digit+1;r++)
{
if(Data[Digit]>10)
Digit++;
Data[r+1]+=Data[r]/10;
Data[r]=Data[r]%10;
}
}
}
printf("%d!= ",i);
for(k=Digit;k>0;k--)
printf("%d",Data[k]);
printf("\n");
}
}
运行结果
1.1.4 显示杨辉三角
#include<stdio.h>
int c(int x,int y) /*求杨辉三角形中第x行第y列的值*/
{
int z;
if((y==1)||(y==x+1))
return 1; /*若为x行的第1或第x+1列,则输出1*/
z=c(x-1,y-1)+c(x-1,y); /*否则,其值为前一行中第y-1列与第y列值之和*/
return z;
}
int main()
{
int i,j,n=13;
printf("N=");
while(n>12)
scanf("%d",&n); /*控制输入正确的值以保证屏幕显示的图形正确*/
for(i=0;i<=n;i++) /*控制输出N行*/
{
for(j=0;j<24-2*i;j++)
printf(" "); /*控制输出第i行前面的空格*/
for(j=1;j<i+2;j++)
printf("%4d",c(i,j)); /*输出第i行的第j个值*/
printf("\n");
}
return 0;
}
运行结果
1.1.5 魔方阵
#include <stdio.h>
#include <math.h>
int main()
{
int a[16][16],i,j,n,k;
printf("Please input n(1~15,it must be odd.): ");
scanf("%d",&n);
while(!(n>=1&&n<=15)||n%2==0)
{
printf("The number is invalid.Please insert again:");
scanf("%d",&n);
}
for(i=1;i<=n;i++)
for(j=1;j<=n;j++)
a[i][j]=0;
j=n/2+1;
a[1][j]=1;
i=1;
for(k=2;k<=n*n;k++)
{
i=i-1;j=j+1;
if(i==0&&j==n+1)
{
i=i+2;j=j-1;
}
else
{
if(i==0)
{
i=n;
}
if(j==n+1)
{
j=1;
}
}
if(a[i][j]==0)
{
a[i][j]=k;
}
else
{
i=i+2;
j=j-1;
a[i][j]=k;
}
}
for(i=1;i<=n;i++)
{
for(j=1;j<=n;j++)
printf("%3d",a[i][j]);
printf("\n");
}
return 0;
}
运行结果
1.1.6 三维数组的表示
#include<stdio.h> /* EOF(=^Z或F6),NULL */
#include<math.h> /* floor(),ceil(),abs() */
#include<stdarg.h>
#define OK 1
#define ERROR 0
#define MAX_ARRAY_DIM 8
#define OVERFLOW -1
#define UNDERFLOW 4
typedef int ElemType;
typedef int Status; /* Status是函数的类型,其值是函数结果状态代码,如OK等 */
typedef struct
{
ElemType *base; /* 数组元素基址,由InitArray分配 */
int dim; /* 数组维数 */
int *bounds; /* 数组维界基址,由InitArray分配 */
int *constants; /* 数组映象函数常量基址,由InitArray分配 */
}Array;
/* 顺序存储数组的基本操作*/
Status InitArray(Array *A,int dim,...)
{ /* 若维数dim和各维长度合法,则构造相应的数组A,并返回OK */
int elemtotal=1,i; /* elemtotal是元素总值 */
va_list ap;
if(dim<1||dim>MAX_ARRAY_DIM)
return ERROR;
(*A).dim=dim;
(*A).bounds=(int *)malloc(dim*sizeof(int));
if(!(*A).bounds)
exit(OVERFLOW);
va_start(ap,dim);
for(i=0;i<dim;++i)
{
(*A).bounds[i]=va_arg(ap,int);
if((*A).bounds[i]<0)
return UNDERFLOW; /* 在math.h中定义为4 */
elemtotal*=(*A).bounds[i];
}
va_end(ap);
(*A).base=(ElemType *)malloc(elemtotal*sizeof(ElemType));
if(!(*A).base)
exit(OVERFLOW);
(*A).constants=(int *)malloc(dim*sizeof(int));
if(!(*A).constants)
exit(OVERFLOW);
(*A).constants[dim-1]=1;
for(i=dim-2;i>=0;--i)
(*A).constants[i]=(*A).bounds[i+1]*(*A).constants[i+1];
return OK;
}
Status DestroyArray(Array *A)
{ /* 销毁数组A */
if((*A).base)
{
free((*A).base);
(*A).base=NULL;
}
else
return ERROR;
if((*A).bounds)
{
free((*A).bounds);
(*A).bounds=NULL;
}
else
return ERROR;
if((*A).constants)
{
free((*A).constants);
(*A).constants=NULL;
}
else
return ERROR;
return OK;
}
Status Locate(Array A,va_list ap,int *off) /* Value()、Assign()调用此函数 */
{ /* 若ap指示的各下标值合法,则求出该元素在A中的相对地址off */
int i,ind;
*off=0;
for(i=0;i<A.dim;i++)
{
ind=va_arg(ap,int);
if(ind<0||ind>=A.bounds[i])
return OVERFLOW;
*off+=A.constants[i]*ind;
}
return OK;
}
Status Value(ElemType *e,Array A,...)
{ /* ...依次为各维的下标值,若各下标合法,则e被赋值为A的相应的元素值 */
va_list ap;
Status result;
int off;
va_start(ap,A);
if((result=Locate(A,ap,&off))==OVERFLOW) /* 调用Locate() */
return result;
*e=*(A.base+off);
return OK;
}
Status Assign(Array *A,ElemType e,...)
{ /* ...依次为各维的下标值,若各下标合法,则将e的值赋给A的指定的元素 */
va_list ap;
Status result;
int off;
va_start(ap,e);
if((result=Locate(*A,ap,&off))==OVERFLOW) /* 调用Locate() */
return result;
*((*A).base+off)=e;
return OK;
}
int main()
{
Array A;
int i,j,k,*p,dim=3,bound1=3,bound2=4,bound3=2; /* a[3][4][2]数组 */
ElemType e,*p1;
InitArray(&A,dim,bound1,bound2,bound3); /* 构造3*4*2的3维数组A */
p=A.bounds;
printf("A.bounds=");
for(i=0;i<dim;i++) /* 顺序输出A.bounds */
printf("%d ",*(p+i));
p=A.constants;
printf("\nA.constants=");
for(i=0;i<dim;i++) /* 顺序输出A.constants */
printf("%d ",*(p+i));
printf("\n%d页%d行%d列矩阵元素如下:\n",bound1,bound2,bound3);
for(i=0;i<bound1;i++)
{
for(j=0;j<bound2;j++)
{
for(k=0;k<bound3;k++)
{
Assign(&A,i*100+j*10+k,i,j,k); /* 将i*100+j*10+k赋值给A[i][j][k] */
Value(&e,A,i,j,k); /* 将A[i][j][k]的值赋给e */
printf("A[%d][%d][%d]=%2d ",i,j,k,e); /* 输出A[i][j][k] */
}
printf("\n");
}
printf("\n");
}
p1=A.base;
printf("A.base=\n");
for(i=0;i<bound1*bound2*bound3;i++) /* 顺序输出A.base */
{
printf("%4d",*(p1+i));
if(i%(bound2*bound3)==bound2*bound3-1)
printf("\n");
}
DestroyArray(&A);
}
1.1.7 多项式的数组表示
#include "stdio.h"
#define MAX_TERMS 100 /* size of terms array */
typedef struct {
float coef;
int expon;
} polynomial;
polynomial terms[ MAX_TERMS];
int avail = 0;
int COMPARE(int coef1,int coef2)
{
if(coef1<coef2)
return -1;
else if(coef1==coef2)
return 0;
else
return 1;
}
void attach (float coefficient, int exponent)
{ /* 加一个新项到多项式中 */
if (avail > MAX_TERMS) {
printf("Too many terms in the polynomial \n");
}
terms[avail].coef = coefficient;
terms[avail++].expon = exponent;
}
void padd( int starta, int finisha, int startb, int finishb,
int *startd,int *finishd)
{ /* A(x)+B(x)=D (x) */
float coefficient;
*startd = avail;
while ( starta <= finisha && startb <=finishb)
switch (COMPARE(terms[starta].expon, terms[startb].expon)) {
case -1: /* a 指数小于 b指数*/
attach(terms[startb].coef, terms[startb].expon);
startb ++;
break;
case 0: /*两指数相等*/
coefficient = terms[starta].coef + terms[startb].coef;
if (coefficient)
attach(coefficient, terms[starta].expon);
starta++;
startb ++;
break;
case 1: /* a指数大于b指数*/
attach(terms[starta].coef,terms[starta].expon);
starta++;
}
/* 把其余的A(x)相加 */
for (; starta <= finisha; starta++)
attach(terms[starta].coef,terms[starta].expon);
/* 把其余的B(x)相加 */
for (; startb <= finishb; startb++)
attach(terms[startb].coef,terms[startb].expon);
*finishd = avail -1;
}
int main()
{
int startd,finishd;
padd(1,2,3,4,&startd,&finishd);
printf("sssss");
return 0;
}
1.1.8 查找矩阵的马鞍点
#include "stdio.h"
#define n 3
#define m 3
void Get_Saddle(int A[m][n])/*求矩阵A中的马鞍点*/
{
int i,j,flag,min,k;
for(i=0;i<m;i++)
{
for(min=A[i][0],j=0;j<n;j++)
if(A[i][j]<min) min=A[i][j]; /*求一行中的最小值*/
for(j=0;j<n;j++)
if(A[i][j]==min) /*判断这个(些)最小值是否鞍点*/
{
for(flag=1,k=0;k<m;k++)
if(min<A[k][j]) flag=0;
if(flag)
printf("Found a saddle element!\nA[%d][%d]=%d",i,j,A[i][j]);
}
}
printf("\n");
}/*Get_Saddle*/
int main()
{
int A[m][n]={1,2,3,4,5,6,7,8,9};
/*初始化A[n] */
Get_Saddle(A);
return 0;
}
运行结果
1.1.9 对角矩阵建立
#include "stdio.h"
#define n 4
int d[n];
int Store(int x, int i, int j)
{/* 把x存为D ( i , j ) */
if (i<0||j<0||i>=n||j>=n)
{
printf("数组出界!");
return(1);
}
if (i != j && x != 0)
{
printf("非对角线上元素值必须为零");
return(1);
}
if (i == j)
d[i] = x;
}
int main()
{
int i,j;
int D[n][n] ={{2,0,0,0},{0,1,0,0},{0,0,4,0},{0,0,0,6}};
for(i=0;i<n;i++)
for(j=0;j<n;j++)
Store(D[i][j],i,j);
for(i=0;i<n;i++)
printf("%d ",d[i]);
printf("\n");
return 1;
}
运行结果
1.1.10 三对角矩阵的建立
#include "stdio.h"
#define n 4
int t[3*n];
int Store(int x, int i, int j)
{
if ( i < 0 || j <0 ||i >=n || j >=n)
{
printf("数组出界!");
return(1);
}
switch (i - j) {
case 1: /* 低对角线*/
t[i - 1] = x; break;
case 0: /* 主对角线*/
t[n + i - 1] = x; break;
case -1: /* 高对角线*/
t[2 *n + i - 1] = x; break;
default:
if(x != 0)
{
printf("非对角线上元素值必须为零");
return(1);
}
return 1;
}
}
int main()
{
int i,j;
int D[n][n]={{2,1,0,0},{3,1,3,0},{0,5,2,7},{0,0,9,0}};
for(i=0;i<n;i++)
for(j=0;j<n;j++)
Store(D[i][j],i,j);
for(i=0;i<3*n-2;i++)
printf("%d ",t[i]);
printf("\n");
return 0;
}
运行结果
1.1.11 三角矩阵建立
#include "stdio.h"
#define n 4
int t[n*n/2];
int Store(int x, int i, int j)
{/* 把x 存为L ( i , j ) */
if (i<0||j<0||i>=n||j>=n)
{
printf("数组出界!");
return(1);
}
/* 当且仅当i ≥ j 时(i,j) 位于下三角*/
if (i >= j)
t[i*(i+1)/2+j] = x;
else if (x != 0)
{
printf("非对角线上元素值必须为零");
return(1);
}
}
int main()
{
int i,j;
int D[n][n]={2,0,0,0,5,1,0,0,0,3,1,0,4,2,7,0};
for(i=0;i<n;i++)
for(j=0;j<n;j++)
Store(D[i][j],i,j);
for(i=0;i<=n*n/2+1;i++)
printf("%d ",t[i]);
printf("\n");
return 0;
}
运行结果
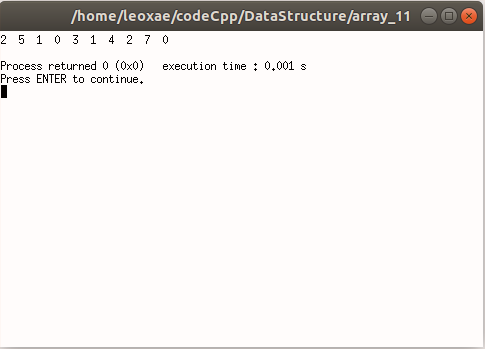
1.1.12 对称矩阵的建立
#include"stdio.h"
#define n 4
int t[n*(n+1)/2];
int Store(int x,int i,int j)
{
if(x<0||j<0||i>=n||j>=n)
{
printf("数组出界!");
return(1);
}
else if(i>=j)
t[i*(i+1)/2+j]=x;
}
int main()
{
int i,j;
int D[n][n]={2,4,6,0,4,1,9,5,6,9,4,7,0,5,7,0};
for(i=0;i<n;i++)
for(j=0;j<n;j++)
Store(D[i][j],i,j);
for(i=0;i<=n*n/2+1;i++)
printf("%d ",t[i]);
printf("\n");
return 1;
}
运行结果
1.1.13 字符串长度的计算
不使用strlen()函数
#include <stdio.h>
int main()
{
char s[1000], i;
printf("\nPlease input string: ");
scanf("%s", s);
for(i = 0; s[i] != '\0'; ++i);
printf("\nThe input string length is %d", i);
return 0;
}
使用strlen()函数
#include <stdio.h>
#include <string.h>
int main()
{
char s[1000];
int len;
printf("\nPlease input string:");
scanf("%s", s);
len = strlen(s);
printf("\nThe input string length is %d", len);
return 0;
}
运行结果
1.1.14 字符串的复制
#include"stdio.h"
char *strcpy(char *s1,char *s2)
{
int i;
for(i=0;s2[i]!='\0';i++)
s1[i]=s2[i];
s1[i]='\0';
return s1;
}
int main()
{
char string[50];
char copystring[50];
printf("\nPlease input string:");
scanf("%s",string);
strcpy(copystring,string);
printf("\nString:%s",string);
printf("\nCopystring:%s",copystring);
return 0;
}
运行结果
1.1.15 字符串的替换
#include"stdio.h"
char *strrep(char *s1,char *s2,int pos)
{
int i,j;
pos--;
i=0;
for(j=pos;s1[j]!='\0';j++)
if(s2[i]!='\0')
{
s1[j]=s2[i];
i++;
}
else
break;
return s1;
}
int main()
{
char string1[100];
char string2[100];
int position;
printf("\nPlease input original string:");
scanf("%s",string1);
printf("\nPlease input substitute string:");
scanf("%s",string2);
printf("\nPlease input substitute position:");
scanf("%d",&position);
strrep(string1,string2,position);
printf("\nThe final string:%s\n",string1);
return 0;
}
运行结果
1.1.16 字符串的删除
#include"stdio.h"
char *strdel(char *s,int pos,int len)
{
int i;
pos--;
for(i=pos+len;s[i]!='\0';i++)
s[i-len]=s[i];
s[i-len]='\0';
return s;
}
int main()
{
char string[50];
int position;
int length;
printf("\nPlease input original string:");
scanf("%s",string);
printf("\nPlease input delete position:");
scanf("%d",&position);
printf("\nPlease input delete length:");
scanf("%d",&length);
strdel(string,position,length);
printf("\nThe final string:%s",string);
return 0;
}
运行结果
1.1.17 字符串的比较
#include"stdio.h"
#include"string.h"
char *strcmp(char *s1,char *s2)
{
int i,j;
for(i=0;s1[i]==s2[i];i++)
if(s1[i]=='\0'&&s2[i]=='\0')
return 0;
if(s1[i]>s2[i])
return 1;
return -1;
}
int main()
{
char s1[50];
char s2[50];
int compare;
printf("\nPlease input string(1):");
fgets(s1,50,stdin);
printf("\nPlease input string(2):");
fgets(s2,50,stdin);
compare=strcmp(s1,s2);
printf("\nString(1):%s",s1);
printf("\nString(2):%s",s2);
printf("\nCompare result:%d",compare);
if (compare == 0) {
printf("\nString(1)=String(2)\n");
} else if (compare > 0) {
printf("\nString(1)>String(2)\n");
} else if (compare < 0) {
printf("\nString(1)<String(2)\n");
}
return 0;
}
运行结果
1.1.18 字符串的抽取
#include"stdio.h"
char *substr(char *s,int pos,int len)
{
char s1[50];
int i,j,endpos;
pos--;
endpos=pos+len-1;
for(i=pos,j=0;i<=endpos;i++,j++)
s1[j]=s[i];
s1[len]='\0';
printf("\nThe substring is '%s'\n",s1);
return s1;
}
int main()
{
char string[100];
char *substring;
int position;
int length;
printf("\nPlease input string:");
fgets(string,100,stdin);
printf("Please input start position:");
scanf("%d",&position);
printf("Please input substring length:");
scanf("%d",&length);
substring=substr(string,position,length);
return 0;
}
运行结果
1.1.19 字符串的分割
#include"stdio.h"
int partition(char *s1,char *s2,int pos)
{
int i,j;
i=pos;
while(s1[i]==' ')
i++;
if(s1[i]!='\0')
{
j=0;
while(s1[i]!='\0'&&s1[i]!=' ')
{
s2[j]=s1[i];
i++;
j++;
}
s2[j]='\0';
return i;
}
else
return -1;
}
int main()
{
char string[50];
char partition_string[20];
int position;
int k;
printf("\nPlease input strng:");
fgets(string,50,stdin);
position=0;
printf("\nPartition result:\n");
k=0;
while((position=partition(string,partition_string,position))!=-1)
{
k++;
printf("Partition %d:%s\n",k,partition_string);
}
}
运行结果
1.1.20 字符串的插入
#include "stdio.h"
#include <string.h>
#define MAX_SIZE 100
int insert (char *s, char *t, int i)
{
char string[MAX_SIZE], *temp =string;
if ( i < 0||i > strlen (s) )
{
printf ("error pos!\n");
return (1);
}
if (!strlen (s))
strcpy (s, t);
else if (strlen (t)) {
strncpy (temp, s, i);
strcat (temp, t) ;
strcat (temp, (s + i ));
strcpy (s, temp );
}
}
int main ()
{
char s[]="Sitplease.";
char t[]=" down ";
insert(s,t,3);
printf("%s",s);
printf ( "\n");
return 0;
}
运行结果
1.1.21 字符串的匹配
#include "stdio.h"
#include <string.h>
int nfind ( char *B, char *A )
{
int i, j, start = 0;
int lasts = strlen (B)-1;
int lastp = strlen (A)-1 ;
int endmatch = lastp;
for(i=0;endmatch<=lasts;endmatch++,start++)
{
if ( B[endmatch] == A[lastp])
for (j=0,i=start;j<lastp&&B[i]==A[j];)
i++,j++;
if ( j == lastp )
return (start+1); /*成功 */
}
/*printf("%d %d %d",lasts,lastp,start); */
if(start==0)
return -1;
}
int main ()
{
char s[]="Sit please";
char t[]="please";
int po=nfind(s,t);
printf("find pos is %d",po);
printf("\n");
return 0;
}
运行结果
1.1.22 字符串的合并
#include "stdio.h"
#include <string.h>
void catstr(char *des,char *sour)
{
int end=strlen(des);
int i,j,num;
num=strlen(sour);
for(i=end,j=0;j<num;i++,j++)
des[i]=sour[j];
for(i=0;i<end+num;i++)
printf("%c",des[i]);
printf("\n");
}
int main ()
{
char s[]="Sit down";
char t[]=" please!";
catstr(s,t);
return 0;
}
运行结果
1.1.23 文本编辑
#include<iostream>
#include<string>
#include<cstdlib>
#include<ctype.h>
#include<cstdio>
#include<fstream>
using namespace std;
int NumberCount=0;//数字个数
int CharCount=0;//字母个数
int PunctuationCount=0;//标点符号个数
int BlankCount=0;//空白符个数
class Node
{
public:
string character;
int cursor;
int offset;
Node* next;
Node(){
cursor=0;//每行的光标初始位置
offset=0;//每行的初始偏移位置
next=NULL;
}
};
class TextEditor
{
private:
Node* head;
string name;
int line;//可更改的行数
int length;//行数
public:
TextEditor();
~TextEditor();
string GetName();
void SetName(string name);
int GetCursor();
int MoveCursor(int offset);
int SetCursor(int line,int offset);
void AddText(const string s);
void InsertText(int seat,string s);
int FindText(string s);
void DeleteText(string s);
int GetLine();
void Count();
friend ostream& operator<<(ostream& out,TextEditor &text);
Node* Gethead(){
return head;
}
//int GetLength()
//{
// return length;
// }
// int FindText(string s);
// void DeleteText(int seat,string s);
};
TextEditor::TextEditor()
{
head=NULL;
name="test";//文件初始名
//tail=NULL;
line=1;
length=0;
}
TextEditor::~TextEditor()
{
Node* p=head;
Node* q;
while(p!=NULL){
q=p->next;
delete p;
p=q;
}
}
int TextEditor::GetLine()
{
return line;
}
string TextEditor::GetName()
{
return name;
}
void TextEditor::SetName(string name)
{
this->name=name;
}
int TextEditor::GetCursor()
{
Node *p=head;
while(p->next!=NULL)
p=p->next;
return p->cursor;
}
int TextEditor::MoveCursor(int offset)
{
Node *p=head;
int i=1;
if(length+1<line){
cout<<"输入错误!"<<endl;
exit(0);
}
else{
while(p->next!=NULL&&i<line){
p=p->next;
i++;
}
}
if(offset>p->character.length()){
cout<<"移动位置太大!"<<endl;
exit(0);
}
else
p->cursor+=offset;
//cout<<"p ->cursor="<<p->cursor<<endl;
return p->cursor;
}
int TextEditor::SetCursor(int line,int offset)
{
this->line=line;
//cout<<"line="<<this->line<<endl;
return MoveCursor(offset);
}
void TextEditor::AddText(const string s)
{
line=length+1;
Node* p=new Node;
Node* q=head;
p->character=s;
p->next=NULL;
if(head==NULL)
head=p;
else{
while(q->next!=NULL)
q=q->next;
q->next=p;
}
length++;
// line++;
}
void TextEditor::InsertText(int seat,string s)
{
Node *p=head;
int i=1;
if(length+1<line){
cout<<"输入错误!"<<endl;
exit(0);
}
else{
while(p->next!=NULL&&i<line){
p=p->next;
i++;
}
}
//MoveCursor(seat);
//cout<<"p->cursor="<<p->cursor<<endl;
string substr;
for(int i=seat;i<s.length()+seat&&i<=p->character.length();i++)
substr+=p->character[i];
p->character.insert(p->cursor,s);
cout<<"substr="<<substr<<endl;
DeleteText(substr);//覆盖子串
p->cursor=0;//光标清零
}
ostream& operator<<(ostream& out,TextEditor &text)
{
int i=1;
Node* p=text.Gethead();
while(p!=NULL){
out<<p->character<<endl;
p=p->next;
}
// cout<<"length="<<text.GetLength()<<endl;
return out;
}
int TextEditor::FindText(string P)
{
Node* q=head;
//int templine=1;
line=1;
int p=0;
int t=0;
int plen=P.length()-1;
//cout<<"P="<<P<<endl;
//cout<<"plen="<<plen<<endl;
int tlen=q->character.length();
while(q!=NULL){
p=0;
t=0;
tlen=q->character.length();
if(tlen<plen){
line++;
q=q->next;
}
while(p<plen&&t<tlen){
if(q->character[t]==P[p]){
t++;
p++;
}
else{
t=t-p+1;
p=0;
}
}
// cout<<"P="<<P<<endl;
// cout<<"p="<<p<<endl;
// cout<<"plen="<<plen<<endl;
if(p>=plen){
return t-plen+1;
}
else{
line++;
q=q->next;
}
}
return -1;
}
void TextEditor::DeleteText(string s)
{
Node *p=head;
int i=1;
int k=FindText(s);
if(k==-1)
cout<<"未出现该字符串!"<<endl;
else{
while(p!=NULL&&i<line){
p=p->next;
// cout<<p->character<<endl;
i++;
}
p->character.erase(k-1,s.length());
cout<<"删除成功!"<<endl;
}
}
void TextEditor::Count()
{
Node *p=head;
NumberCount=0;
CharCount=0;
PunctuationCount=0;
BlankCount=0;
while(p!=NULL){
for(int i=0;i<p->character.length();i++){
if(p->character[i]>='0'&&p->character[i]<='9')
NumberCount++;
else if(p->character[i]>'a'&&p->character[i]<'z'||p->character[i]>'A'&&p->character[i]<'Z')
CharCount++;
else if(ispunct(p->character[i]))
PunctuationCount++;
else if(p->character[i]==' ')
BlankCount++;
}
p=p->next;
}
}
int main()
{
int i,j,k,n=2;
string s,t,name;
TextEditor text;
cout<<"---------------------------------------"<<endl;
cout<<"1.添加字符"<<endl;
cout<<"2.设置文档名字"<<endl;
cout<<"3.获取文档名字"<<endl;
cout<<"4.显示光标位置"<<endl;
cout<<"5.设置光标位置,在光标位置处插入文本"<<endl;
cout<<"6.在文档中查找文本"<<endl;
cout<<"7.在文档中删除文本"<<endl;
cout<<"8.统计字母、数字、标点符号、空白符号及总字符个数"<<endl;
cout<<"9.输入文本"<<endl;
cout<<"0.退出"<<endl;
while(n){
// cout<<endl;
cout<<endl;
cout<<"---------------------------------------"<<endl;
cout<<"请输入:";
cin>>n;
getchar();
switch(n){
case 1: cout<<"请输入字符:"; getline(cin,s,'\n'); text.AddText(s); break;
case 2: cout<<"请输入文档名字:"; cin>>name; text.SetName(name); break;
case 3: cout<<text.GetName()<<endl; break;
case 4: cout<<"光标在第"<<text.GetLine()<<"行,第"<<text.GetCursor()<<"个字符前!"<<endl; break;
case 5:{
cout<<"输入行数:";
cin>>i;
cout<<"光标在第"<<text.GetCursor()<<"个字符前!"<<endl;
cout<<"输入移动位数:";
cin>>j;
cout<<"输入插入字符:";
getchar();
getline(cin,s);
text.InsertText(text.SetCursor(i,j),s); break;
}
case 6: {
cout<<"输入查找的字符串:";
getline(cin,s);
int k=text.FindText(s);
if(k==-1)
cout<<"查找失败!"<<endl;
else
cout<<"所查找文本首次出现在:"<<text.GetLine()<<"行,第"<<k<<"个字符处!"<<endl;
break;
}
case 7: cout<<"输入要删除的字符串:"; getline(cin,s); text.DeleteText(s); break;
case 8: {
text.Count();
cout<<"文档中共有:"<<endl;
cout<<NumberCount<<"个数字"<<endl;
cout<<CharCount<<"个字母"<<endl;
cout<<PunctuationCount<<"个标点符号"<<endl;
cout<<BlankCount<<"个空白字符"<<endl;
cout<<"共有"<<NumberCount+CharCount+PunctuationCount+BlankCount<<"个字符!"<<endl;
break;
}
case 9: cout<<text; break;
case 0:{
string ss=text.GetName();
ss+=".txt";
cout<<ss<<endl;
ofstream outFile(ss.c_str());
Node* p=text.Gethead();
while(p!=NULL){
outFile<<p->character<<endl;
p=p->next;
}
exit(0);
break;
}
default: cout<<"输入错误,请重新输入!"<<endl; break;
}
}
}
运行结果
Talk is cheap. Show me the code