python爬虫获取一个月内地震目录,基于cartopy模块在世界地图上画震中分布图
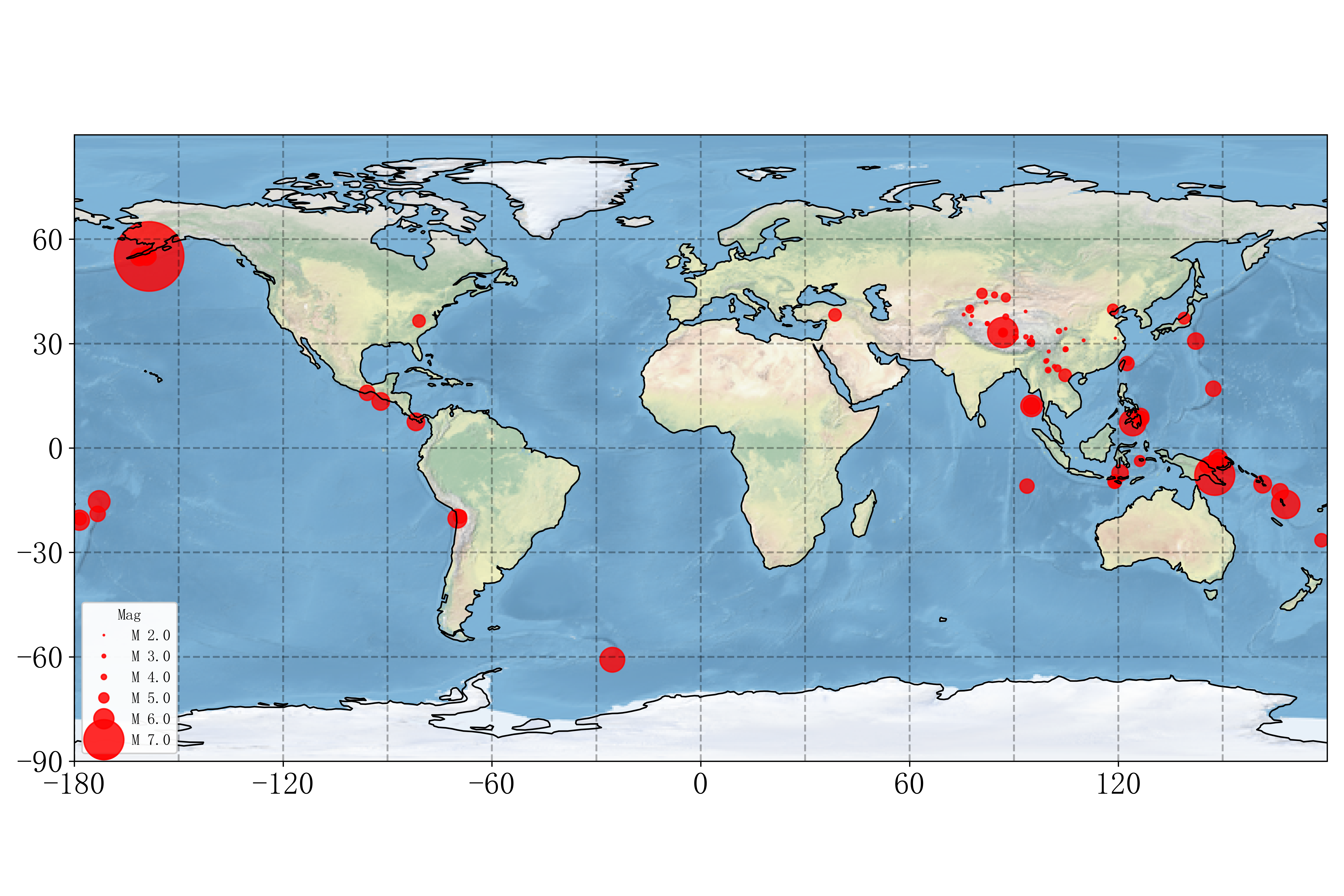
import cartopy.crs as ccrs import matplotlib.pyplot as plt from cartopy.mpl.gridliner import LONGITUDE_FORMATTER, LATITUDE_FORMATTER import matplotlib.ticker as mticker import numpy as np import pandas as pd import requests from bs4 import BeautifulSoup def get_catalog(): url = 'http://news.ceic.ac.cn/index.html' web_data = requests.get(url) web_data.encoding = web_data.apparent_encoding web_data = web_data.text f = open('earthquake.csv', 'w') Date = [] Latitude = [] Longitude = [] Magnitude = [] soup = BeautifulSoup(web_data, 'lxml') rows = soup.find_all('tr') for i in rows: cols = i.find_all('td') if len(cols) != 0: Magnitude.append(cols[0].text) Date.append(cols[1].text.split()[0]) Latitude.append(cols[2].text) Longitude.append(cols[3].text) f.write('Date,Latitude,Longitude,Magnitude\n') for i in range(len(Date)): line = Date[i] + ',' + Latitude[i] + ',' + Longitude[i] + ',' + Magnitude[i] print(Date[i] + ',' + Latitude[i] + ',' + Longitude[i] + ',' + Magnitude[i]) f.write(line + '\n') f.close() def plot_map(): plt.figure(figsize=(12, 8)) plt.rcParams['font.sans-serif'] = 'FangSong' # 设置中文字体为仿宋 plt.rcParams['axes.unicode_minus'] = False # 正常显示坐标轴上的铀号 ax = plt.axes(projection=ccrs.PlateCarree()) ax.coastlines() ax.stock_img() # 将参考底图图像添加到地图,如果没有这条命令,底图是没有背景色的 # 画经纬度网格 gl = ax.gridlines(crs=ccrs.PlateCarree(), draw_labels=False, linewidth=1.2, color='k', alpha=0.3, linestyle='--') gl.xlabels_top = False # 关闭顶端的经纬度标签 gl.ylabels_right = False # 关闭右侧的经纬度标签 gl.xformatter = LONGITUDE_FORMATTER # x轴设为经度的格式 gl.yformatter = LATITUDE_FORMATTER # y轴设为纬度的格式 #设置经纬度网格的间隔 gl.xlocator = mticker.FixedLocator(np.arange(-180, 180, 30)) gl.ylocator = mticker.FixedLocator(np.arange(-90, 90, 30)) # 设置显示范围 ax.set_extent([-180, 180, -90, 90],crs=ccrs.PlateCarree()) #设置坐标标签 ax.set_xticks(list(range(-180,180,60)), crs=ccrs.PlateCarree()) ax.set_yticks(list(range(-90,90,30)), crs=ccrs.PlateCarree()) plt.xticks(fontsize = 20) plt.yticks(fontsize = 20) # 填加大地测量座标系下的线条 # ny_lon, ny_lat = -75, 43 # delhi_lon, delhi_lat = 77.23, 28.61 # plt.plot([ny_lon, delhi_lon], [ny_lat, delhi_lat], # color='blue', linewidth=2, marker='o', # transform=ccrs.Geodetic(), # ) # 填加直角座标系下的线条 # plt.plot([ny_lon, delhi_lon], [ny_lat, delhi_lat], # color='red', linestyle='--', # transform=ccrs.PlateCarree(), # ) # 填加文字 # plt.text(ny_lon - 3, ny_lat - 12, '纽约', # horizontalalignment='right', color = 'red', # transform=ccrs.Geodetic()) # plt.text(delhi_lon + 3, delhi_lat - 12, 'Delhi', # horizontalalignment='left', color = 'red', # transform=ccrs.Geodetic()) # 画震中分布 data = pd.read_csv('earthquake.csv') scatter = ax.scatter(data.Longitude, data.Latitude, s= (0.2* 2 ** data.Magnitude)**2, c='red', alpha=0.8, # c=data.depth / data.depth.max(), alpha=0.8, transform=ccrs.PlateCarree()) # 填加图例 kw = dict(prop="sizes", num=5, color='red', fmt="M {x:.1f}", func=lambda s: np.log2(np.sqrt(s)/0.2)) legend2 = ax.legend(*scatter.legend_elements(**kw), loc="lower left", title="Mag") ax.add_artist(legend2) plt.tight_layout() plt.savefig('world.png',dpi = 600) # plt.show() #################主程序################### get_catalog() plot_map()