SpringBoot系列——i18n国际化
前言
国际化是项目中不可或缺的功能,本文将实现springboot + thymeleaf的HTML页面、js代码、java代码国际化过程记录下来。
代码编写
工程结构
每个文件里面的值(按工程结构循序从上往下)
##################默认值############################# welcome=Welcome
##################英文############################# welcome=Welcome
##################简体中文############################# welcome=欢迎
##################簡體中文############################# welcome=歡迎
yml配置文件
#注意:在yml文件中添加value值时,value前面需要加一个空格 #2.0.0的配置切换为servlet.path而不是"-" server: port: 10086 #端口号 servlet: context-path: /springboot #访问根路径 spring: thymeleaf: cache: false #关闭页面缓存 prefix: classpath:/view/ #thymeleaf访问根路径 mode: LEGACYHTML5 messages: basename: static/i18n/messages #指定国际化文件路径
LocaleConfig.java,
@Configuration @EnableAutoConfiguration @ComponentScan public class LocaleConfig extends WebMvcConfigurerAdapter { @Bean public LocaleResolver localeResolver() { SessionLocaleResolver slr = new SessionLocaleResolver(); // 默认语言 slr.setDefaultLocale(Locale.US); return slr; } @Bean public LocaleChangeInterceptor localeChangeInterceptor() { LocaleChangeInterceptor lci = new LocaleChangeInterceptor(); // 参数名 lci.setParamName("lang"); return lci; } @Override public void addInterceptors(InterceptorRegistry registry) { registry.addInterceptor(localeChangeInterceptor()); } }
controller
单纯的跳转页面即可
@RequestMapping("/i18nTest") public ModelAndView i18nTest(){ ModelAndView mv=new ModelAndView(); mv.setViewName("i18nTest.html"); return mv; }
HTML使用,注意要用 #{} 来取值
<!DOCTYPE html> <!--解决idea thymeleaf 表达式模板报红波浪线--> <!--suppress ALL --> <html xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <title></title> </head> <body> <h3 th:text="#{welcome}"></h3> <a href="?lang=en_US">English(US)</a> <a href="?lang=zh_CN">简体中文</a> <a href="?lang=zh_TW">繁体中文</a> </body> </html>
js代码使用
需要先引入jquery插件,自行百度下载或者使用webjar去拉取,可以封装成一个全局方法,用到时直接调用
<script th:src="@{/js/jquery-1.9.1.min.js}"></script> <script th:src="@{/js/jquery.i18n.properties.js}"></script>
<script th:inline="javascript"> //项目路径 ctx = [[${#request.getContextPath()}]]; //初始化i18n插件 try { $.i18n.properties({ path: ctx + '/i18n/', name: 'messages', language: [[${#locale.language+'_'+#locale.country}]], mode: "both" }); } catch (e) { console.error(e); } //初始化i18n方法 function i18n(labelKey) { try { return $.i18n.prop(labelKey); } catch (e) { console.error(e); return labelKey; } } console.log(i18n("welcome")); </script>
java代码使用
先封装个工具类,直接静态调用
@Component public class I18nUtil { private static MessageSource messageSource; public I18nUtil(MessageSource messageSource) { I18nUtil.messageSource = messageSource; } /** * 获取单个国际化翻译值 */ public static String get(String msgKey) { try { return messageSource.getMessage(msgKey, null, LocaleContextHolder.getLocale()); } catch (Exception e) { return msgKey; } } }
调用
System.out.println(I18nUtil.get("welcome"));
效果展示
默认
英文
中文
繁体
结束语
文章部分参考:玩转spring boot——国际化:https://www.cnblogs.com/GoodHelper/p/6824492.html
代码开源
代码已经开源、托管到我的GitHub、码云:
版权声明
作者:huanzi-qch
若标题中有“转载”字样,则本文版权归原作者所有。若无转载字样,本文版权归作者所有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文链接,否则保留追究法律责任的权利.
捐献、打赏
请注意:相应的资金支持能更好的持续开源和创作,如果喜欢这篇文章,请随意打赏!
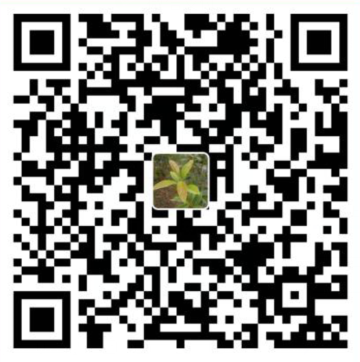
支付宝

微信
QQ群交流群
QQ群交流群
有事请加群,有问题进群大家一起交流!
有事请加群,有问题进群大家一起交流!
