使用java调用fastDFS客户端进行静态资源文件上传
一、背景
上篇博客我介绍了FastDFS的概念、原理以及安装步骤,这篇文章我们来聊一聊如何在java中使用FastDFSClient进行静态资源的上传。
二、使用步骤
1.开发环境
spring+springmvc+maven
2.首先在maven的pom.xml中引入依赖fastdfs-client的依赖
1 <dependency> 2 <groupId>org.csource</groupId> 3 <artifactId>fastdfs-client-java</artifactId> 4 <version>5.0.4</version> 5 </dependency>
3.接着我们来指定一个fastdfs-client.conf配置文件,里面内容如下:
tracker_server=host:port(这里指trackerServer服务器的ip和端口)
4.然后写一个单元测试类来测试服务
package com.hafiz.fastdfs; import java.io.FileNotFoundException; import java.io.IOException; import org.csource.common.MyException; import org.csource.fastdfs.ClientGlobal; import org.csource.fastdfs.StorageClient; import org.csource.fastdfs.StorageServer; import org.csource.fastdfs.TrackerClient; import org.csource.fastdfs.TrackerServer; import org.junit.Test; import com.taotao.common.utils.FastDFSClient; public class FastdfsTest { private static final String CONFIGLOCATION = "D:\\fastdfs_client.conf"; @Test public void testUploadImg () { try { // 初始化全局配置。加载client配置文件 ClientGlobal.init(CONFIGLOCATION); // 创建一个TrackerClient对象 TrackerClient trackerClient = new TrackerClient(); // 创建一个TrackerServer对象 TrackerServer trackerServer = trackerClient.getConnection(); // 声明一个StorageServer对象并初始为null StorageServer storageServer = null; // 获得StorageClient对象 StorageClient storageClient = new StorageClient(trackerServer, storageServer); // 直接调用StorageClient对象方法上传文件即可 String[] result = storageClient.upload_file("D:\\Documents\\Downloads\\高圆圆2.jpg", "jpg", null); for(String item : result) { System.out.println(item); } trackerServer.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } catch (MyException e) { e.printStackTrace(); } } @Test public void fastDfsClientTest() { try { FastDFSClient client = new FastDFSClient(CONFIGLOCATION); String imgUrl = client.uploadFile("D:\\Documents\\Downloads\\高圆圆1.jpg", "jpg", null); System.out.println(imgUrl); } catch (Exception e) { e.printStackTrace(); } } }
5.为了以后在项目中使用方便,我们不能每次都写这么一大串东西,所以我们来对该客户端进行以下封装:
package com.hafiz.common.utils; import org.csource.common.NameValuePair; import org.csource.fastdfs.ClientGlobal; import org.csource.fastdfs.StorageClient1; import org.csource.fastdfs.StorageServer; import org.csource.fastdfs.TrackerClient; import org.csource.fastdfs.TrackerServer; public class FastDFSClient { private TrackerClient trackerClient = null; private TrackerServer trackerServer = null; private StorageServer storageServer = null; private StorageClient1 storageClient = null; public FastDFSClient(String conf) throws Exception { if (conf.contains("classpath:")) { String url = this.getClass().getResource("/").getPath(); url = url.substring(1); conf = conf.replace("classpath:", url); } ClientGlobal.init(conf); trackerClient = new TrackerClient(); trackerServer = trackerClient.getConnection(); storageServer = null; storageClient = new StorageClient1(trackerServer, storageServer); } public String uploadFile(String fileName, String extName, NameValuePair[] metas) throws Exception { return storageClient.upload_file1(fileName, extName, metas); } public String uploadFile(String fileName, String extName) throws Exception { return storageClient.upload_file1(fileName, extName, null); } public String uploadFile(String fileName) throws Exception { return storageClient.upload_file1(fileName, null, null); } public String uploadFile(byte[] fileContent, String extName, NameValuePair[] metas) throws Exception { return storageClient.upload_file1(fileContent, extName, metas); } public String uploadFile(byte[] fileContent, String extName) throws Exception { return storageClient.upload_file1(fileContent, extName, null); } public String uploadFile(byte[] fileContent) throws Exception { return storageClient.upload_file1(fileContent, null, null); } }
三、总结
通过以上的步骤,我们就完成在java中使用fastdfs客户端进行静态资源上传的功能,这里面我们得到一个最重要的思想就是:DRY(Don't Repeat Yourself!),要有封装的思想。
感谢您花时间阅读此篇文章,如果您觉得这篇文章你学到了东西也是为了犒劳下博主的码字不易不妨打赏一下吧,让博主能喝上一杯咖啡,在此谢过了!
如果您觉得阅读本文对您有帮助,请点一下左下角“推荐”按钮,您的将是我最大的写作动力!另外您也可以选择【关注我】,可以很方便找到我!
本文版权归作者和博客园共有,来源网址:http://www.cnblogs.com/hafiz 欢迎各位转载,但是未经作者本人同意,转载文章之后必须在文章页面明显位置给出作者和原文连接,否则保留追究法律责任的权利!
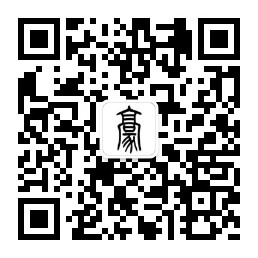
