力扣 129. 求根节点到叶节点数字之和
给你一个二叉树的根节点
root
,树中每个节点都存放有一个 0
到 9
之间的数字。
每条从根节点到叶节点的路径都代表一个数字:
- 例如,从根节点到叶节点的路径
1 -> 2 -> 3
表示数字123
。
计算从根节点到叶节点生成的 所有数字之和 。
叶节点 是指没有子节点的节点。
示例 1:
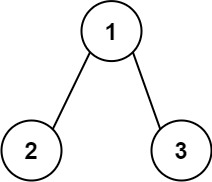
输入:root = [1,2,3]
输出:25
解释:
从根到叶子节点路径 1->2 代表数字 12 从根到叶子节点路径 1->3 代表数字 13 因此,数字总和 = 12 + 13 = 25
示例 2:
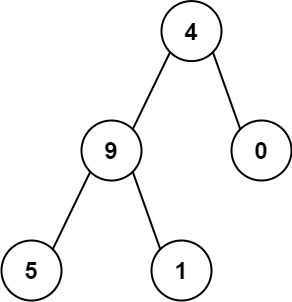
输入:root = [4,9,0,5,1]
输出:1026
解释:
从根到叶子节点路径 4->9->5 代表数字 495 从根到叶子节点路径 4->9->1 代表数字 491 从根到叶子节点路径 4->0 代表数字 40 因此,数字总和 = 495 + 491 + 40 = 1026
提示:
- 树中节点的数目在范围
[1, 1000]
内 0 <= Node.val <= 9
- 树的深度不超过
10
题解
使用递归完成dfs,每次遍历到当前节点时,通过变量curSum
根节点到当前节点的和,在每次遍历加入子节点时,当前和就乘以10+子节点的值。如果是叶子节点就记录并停止递归。
查看代码
class Solution {
public:
int allSum=0;
void work(TreeNode* cur,int curSum){//cur当前节点,curSum根节点到当前节点的和
if(cur->left==nullptr&&cur->right==nullptr){//如果是叶子节点就记录并停止递归
allSum+=curSum;
return;
}
if(cur->left)
work(cur->left,curSum*10+cur->left->val);
if(cur->right)
work(cur->right,curSum*10+cur->right->val);
}
int sumNumbers(TreeNode* root) {
if(root==nullptr)
return 0 ;
work(root,root->val);
return allSum;
}
};
也有不使用遍历记录和的写法。
查看代码
class Solution {
public:
int work(TreeNode* cur,int curSum){
if(cur==nullptr)
return 0;
curSum=curSum*10+cur->val;
if(cur->left==nullptr&&cur->right==nullptr){
return curSum;
}
return work(cur->left,curSum)+work(cur->right,curSum);
}
int sumNumbers(TreeNode* root) {
if(root==nullptr)
return 0 ;
return work(root,0);
}
};