Java学习-017-EXCEL 文件读取实例源代码
众所周知,EXCEL 也是软件测试开发过程中,常用的数据文件导入导出时的类型文件之一,此文主要讲述如何通过 EXCEL 文件中 Sheet 的索引(index)或者 Sheet 名称获取文件中对应 Sheet 页中的数据。敬请各位小主参阅,若有不足之处,敬请大神指正,不胜感激!
不多言,小二上码咯。。。
通过 sheet_index 读取 EXCEL 数据源代码如下所示,敬请参阅!

1 /** 2 * @function 文件读取: EXCEL文件 3 * @description 通过EXCEL文件sheet的索引index读取 4 * 5 * @author Aaron.ffp 6 * @version V1.0.0: autoUISelenium main.java.aaron.java.tools FileUtils.java excelRead, 2014-11-25 16:11:03 Exp $ 7 * 8 * @param filename : EXCEL文件路径 9 * @param sheetIndex : EXCEL文件sheet索引 10 * 11 * @return ArrayList<String[]> --> EXCEL文件内容的二维数组 12 */ 13 public ArrayList<String[]> excelRead(String filename, int sheetIndex){ 14 /* Excel 数据 */ 15 ArrayList<String[]> excelData = new ArrayList<String[]>(); 16 17 Workbook workbook = null; // 工作薄 18 Cell cell = null; // 单元格 19 20 /* 参数校验: 为null或空字符串时, 抛出参数非法异常 */ 21 if (filename == null || "".equals(filename) || !assertFileType(filename, "EXCEL")) { 22 throw new IllegalArgumentException(); 23 } 24 25 try{ 26 /* 创建输入流 */ 27 FileInputStream fis = new FileInputStream(filename); 28 29 /* 获取 Excel 文件对象 */ 30 workbook = Workbook.getWorkbook(fis); 31 32 /* 获取 Excel 文件的第一个工作表 */ 33 Sheet sheet = workbook.getSheet(sheetIndex); 34 35 /* Excel 文件行数 */ 36 for (int i = 0; i < sheet.getRows(); i++) { 37 /* 声明数组存储行数据 */ 38 String[] rowData = new String[sheet.getColumns()]; 39 40 /* Excel文件列数 */ 41 for (int j = 0; j < sheet.getColumns(); j++) { 42 /* 获取单元格 cell[i][j] */ 43 cell = sheet.getCell(j, i); 44 45 rowData[j] = cell.getContents().toString().trim(); 46 } 47 48 // 若当前行均为null或者空, 则退出循环, 返回非空行数据 49 if (this.stringutil.assertStringListIsNull(rowData)) { 50 return excelData; 51 } 52 53 /* 将获取的行数据存到 excelData */ 54 excelData.add(rowData); 55 } 56 } catch (FileNotFoundException fnfe){ 57 this.message = "文件 {" + filename + "} 不存在!"; 58 this.logger.error(this.message, fnfe); 59 60 return null; 61 } catch (BiffException be){ 62 this.message = "文件 {" + filename + "} 读取失败!"; 63 this.logger.error(this.message, be); 64 65 return null; 66 } catch (IOException ioe){ 67 this.message = "文件 {" + filename + "} 读取失败!"; 68 this.logger.error(this.message, ioe); 69 70 return null; 71 } 72 73 return excelData; 74 }
对应的测试源码如下所示:

1 /** 2 * Test : get data from excel by sheet index 3 * 4 * @author Aaron.ffp 5 * @version V1.0.0: autoUISelenium test.java.aaron.java.tools FileUtilsTest.java test_excelRead_by_index, 2014-11-25 16:19:13 Exp $ 6 * 7 */ 8 @Test 9 public void test_excelRead_by_index(){ 10 this.message = "\n\n\nTEST:FileUtils.csvWrite(String filename, char delimiter, String encoding, ArrayList<String[]> csvdata)"; 11 this.logger.debug(this.message); 12 13 this.fu = new FileUtils(); 14 String filename = this.constantslist.PROJECTHOME + this.constantslist.FILESEPARATOR + 15 "testng-temp" + this.constantslist.FILESEPARATOR + "excelRead.xls"; 16 17 ArrayList<String[]> exceldata = this.fu.excelRead(filename, 0); 18 19 Assert.assertEquals(exceldata.get(5)[2], "5 = 2", "Test case failed."); 20 }
通过 sheet_name 读取 EXCEL 数据源代码如下所示,敬请参阅!

1 /** 2 * @function 文件读取: EXCEL文件(通过EXCEL的sheet名称) 3 * 4 * @author Aaron.ffp 5 * @version V1.0.0: autoUISelenium main.java.aaron.java.tools FileUtils.java excelReadByName, 2014-11-25 16:08:19 Exp $ 6 * 7 * @param filename : EXCEL文件路径 8 * @param sheetname : EXCEL文件sheet名称 9 * @param ignorerows : 忽略的初始行数 10 * 11 * @return ArrayList<String[]> --> EXCEL文件内容的二维数组 12 */ 13 public ArrayList<String[]> excelReadByName(String filename, String sheetname, int ignorerows){ 14 /* Excel 数据 */ 15 ArrayList<String[]> excelData = new ArrayList<String[]>(); 16 17 Workbook workbook = null; // 工作薄 18 Cell cell = null; // 单元格 19 20 /* 参数校验: 为null或空字符串时, 抛出参数非法异常 */ 21 if (filename == null || "".equals(filename) || !this.assertFileType(filename, "EXCEL")) { 22 throw new IllegalArgumentException(); 23 } 24 25 try{ 26 /* 创建输入流 */ 27 FileInputStream fis = new FileInputStream(filename); 28 29 /* 获取 Excel 文件对象 */ 30 workbook = Workbook.getWorkbook(fis); 31 32 /* 获取 Excel 文件的第一个工作表 */ 33 Sheet sheet = workbook.getSheet(sheetname); 34 35 /* Excel 文件遍历 */ 36 for (int i = 0; i < sheet.getRows(); i++) { // Excel 文件行数 37 if (i < ignorerows) { 38 this.message = "忽略参数文件的初始行数为:" + ignorerows; 39 this.logger.info(this.message); 40 41 continue; 42 } 43 44 /* 声明数组存储行数据 */ 45 String[] rowData = new String[sheet.getColumns()]; 46 47 for (int j = 0; j < sheet.getColumns(); j++) { // Excel 文件列数 48 /* 获取单元格 cell[col][row] */ 49 cell = sheet.getCell(j, i); 50 51 rowData[j] = cell.getContents().toString().trim(); 52 } 53 54 // 若当前行均为null或者空, 则退出循环, 返回非空行数据 55 if (this.stringutil.assertStringListIsNull(rowData)) { 56 return excelData; 57 } 58 59 /* 将获取的行数据存到 excelData */ 60 excelData.add(rowData); 61 62 fis.close(); 63 } 64 } catch (FileNotFoundException fnfe){ 65 this.message = "文件 {" + filename + "} 不存在!"; 66 this.logger.error(this.message, fnfe); 67 68 return null; 69 } catch (BiffException be){ 70 this.message = "文件 {" + filename + "} 读取失败!"; 71 this.logger.error(this.message, be); 72 73 return null; 74 } catch (IOException ioe){ 75 this.message = "文件 {" + filename + "} 读取失败!"; 76 this.logger.error(this.message, ioe); 77 78 return null; 79 } catch (NullPointerException npe){ 80 this.message = "文件 { " + filename + " } 不存在 Sheet 页面{ " + sheetname + " }"; 81 this.logger.error(this.message, npe); 82 83 return null; 84 } 85 86 return excelData; 87 }
对应的测试源码如下所示:

1 /** 2 * Test : get data from excel by sheet name 3 * 4 * @author Aaron.ffp 5 * @version V1.0.0: autoUISelenium test.java.aaron.java.tools FileUtilsTest.java test_excelRead_by_sheetname, 2014-11-25 16:20:03 Exp $ 6 * 7 */ 8 @Test 9 public void test_excelRead_by_sheetname(){ 10 this.message = "\n\n\nTEST:FileUtils.csvWrite(String filename, char delimiter, String encoding, ArrayList<String[]> csvdata)"; 11 this.logger.debug(this.message); 12 13 this.fu = new FileUtils(); 14 String filename = this.constantslist.PROJECTHOME + this.constantslist.FILESEPARATOR + 15 "testng-temp" + this.constantslist.FILESEPARATOR + "excelRead.xls"; 16 17 ArrayList<String[]> exceldata = this.fu.excelReadByName(filename, "Sheet2", 0); 18 19 if (exceldata != null) { 20 Assert.assertEquals(exceldata.get(9)[5], "9 = sheet 5", "Test case failed."); 21 } else { 22 this.message = "文件 { " + filename + " } 读取失败"; 23 Assert.fail(this.message); 24 } 25 }
至此, Java学习-017-EXCEL 文件读取实例源代码 顺利完结,希望此文能够给初学 Java 的您一份参考。
最后,非常感谢亲的驻足,希望此文能对亲有所帮助。热烈欢迎亲一起探讨,共同进步。非常感谢! ^_^
欢迎 【 留言 || 关注 || 打赏 】 。您的每一份心意都是对我的鼓励和支持!非常感谢!欢迎互加,相互交流学习!
作者:范丰平,本文链接:https://www.cnblogs.com/fengpingfan/p/4602726.html
Copyright @范丰平 版权所有,如需转载请标明本文原始链接出处,严禁商业用途! 我的个人博客链接地址:http://www.cnblogs.com/fengpingfan
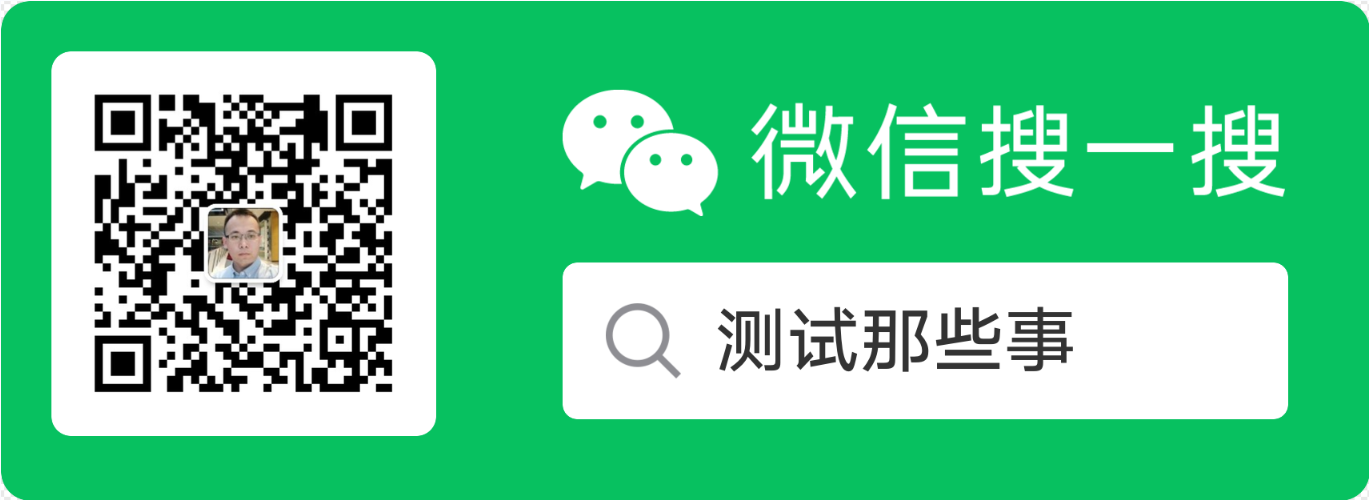