ESP32 开发环境的搭建与详解
ESP32 开发环境的搭建与详解
ESP-IDF
ESP-IDF (Espressif IoT Development Framework) 是乐鑫科技提供的一站式物联网开发框架,它以C/C++ 为主要的开发语言。
Github:https://github.com/espressif/esp-idf
安装
windows 系统
windows 系统安装 ESP-IDF 开发环境,可以通过 https://dl.espressif.cn/dl/esp-idf 下载在线或者离线安装工具。
TIPS:
离线安装工具本身文件大(500MB 至1.xG),但相较于只有 4MB 的在线安装工具,安装成功几率大,在线安装可能会因为网络问题导致安装失败,故这里推荐使用离线安装包进行安装。
安装工具本身也是开源,可通过访问 https://github.com/espressif/idf-installer 查看源码。
在浏览器中打开下载地址:https://dl.espressif.cn/dl/esp-idf ,下载离线安装包 esp-idf-tools-setup-offline-4.3.4
,如下图所示:
安装过程中,选择的安装目录中不要有空格和中文,这里我们选择的安装路径是:
C:\ProgramFiles\Espressif
安装完成后,会得到两个 ESP_IDF 终端 ,如下图所示:
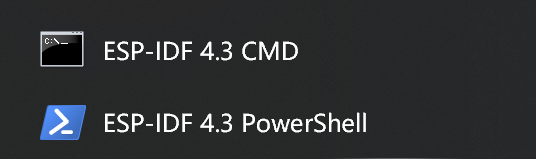
在这两个终端任选其一,打开终端时会自动添加 ESP-IDF 的环境变量,之后就可使用 idf.py 命令进行操作了。如下图所示:
idf.py
使用 idf.py 工具进行开发,参见:
VS Code 代码编辑工具
ESP-IDF SDK默认不附带代码编辑工具(最新的Windows版安装工具可选择安装ESP-IDF Eclipse),读者可使用任何文本编辑工具进行代码的编辑,代码编辑完成后可在终端控制台使用命令进行代码的编译。
VS Code 下载地址 :https://code.visualstudio.com/
vscode-esp-idf-extension
要在 VS Code 中开发 ESP,还得安装插件 vscode-esp-idf-extension,
TIPS:
如何安装插件,可以查看其安装文档:https://github.com/espressif/vscode-esp-idf-extension/blob/HEAD/docs/tutorial/install.md
下面演示安装过程:
在 VS Code 的左侧菜单中选择【扩展(Ctrl + Shift + X)】,在搜索框中输入:
ESP-IDF
在搜索结果中找到 ESP-IDF 插件,如下图所示:
点击【安装】按钮进行安装。
插件安装成功后,如果没有弹出【ESP-IDF 插件】的配置界面,可以安装 F1,在弹出的输入框中输入:
configure esp-idf
如下图所示:
选择【ESP-IDF:配置 ESP-IDF 插件】选项,然后出现配置界面,如下图所示:
因为之前我们已经离线安装了 ESP-IDF 的开发环境,ESP-IDF 插件 已经检测到了,所以这里直接选择第 3 个选择,即:选择已经存在的 ESP-IDF 开发环境,如下图所示:
TIPS
如果在其它选项中通过 ESP-IDF 插件 安装 ESP-IDF 开发环境,有可能因为网络原因而导致安装失败,故推荐的做法是:
先通过 ESP-IDF 离线安装工具进行安装, 在 ESP-IDF 插件 中选择已经存在的ESP-IDF 开发环境。
选择【USE EXISTING SETUP】,然后跳转到配置界面:
接着开始初始化配置:
请耐心等待,过一会,弹出如下界面,表示配置成功:
在 VS Code 的左侧菜单中也会出现一个插件的按钮,如下图所示:
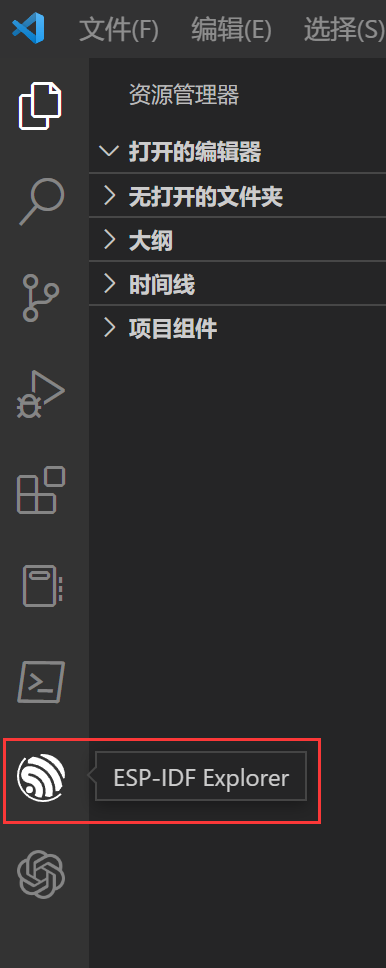
Hello_world 示例
创建项目
打开 VS Code, 按 F1, 在弹框中输入: ESP-IDF,
在弹框中选择【展示项目示例】选项
选择当前的 ESP-IDF 开发环境,最后弹出示例列表,如下图所示:
这里选择【helo_world】示例,使用该示例项目创建新的项目。
在弹框中,选择项目的保存路径,点击确定即可。
特别注意:项目的保存路径中不要出现中文,否则无法项目编译会失败!!!
创建项目成功后,项目的结构如下图所示:
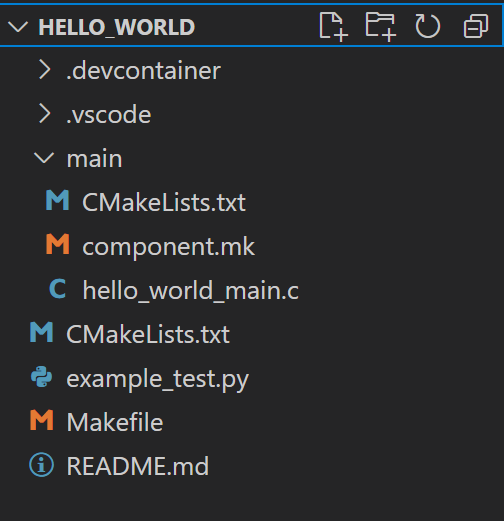
程序入口
程序入口文件,hello_world_main.c
代码清单:main/hello_world_main.c
/* Hello World Example
This example code is in the Public Domain (or CC0 licensed, at your option.)
Unless required by applicable law or agreed to in writing, this
software is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
CONDITIONS OF ANY KIND, either express or implied.
*/
#include <stdio.h>
#include "sdkconfig.h"
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "esp_system.h"
#include "esp_spi_flash.h"
void app_main(void)
{
printf("Hello world!\n");
/* Print chip information */
esp_chip_info_t chip_info;
esp_chip_info(&chip_info);
printf("This is %s chip with %d CPU core(s), WiFi%s%s, ",
CONFIG_IDF_TARGET,
chip_info.cores,
(chip_info.features & CHIP_FEATURE_BT) ? "/BT" : "",
(chip_info.features & CHIP_FEATURE_BLE) ? "/BLE" : "");
printf("silicon revision %d, ", chip_info.revision);
printf("%dMB %s flash\n", spi_flash_get_chip_size() / (1024 * 1024),
(chip_info.features & CHIP_FEATURE_EMB_FLASH) ? "embedded" : "external");
printf("Minimum free heap size: %d bytes\n", esp_get_minimum_free_heap_size());
for (int i = 10; i >= 0; i--) {
printf("Restarting in %d seconds...\n", i);
vTaskDelay(1000 / portTICK_PERIOD_MS);
}
printf("Restarting now.\n");
fflush(stdout);
esp_restart();
}
ESP32 开发板连接 PC
使用 USB 线将 ESP32 开发板连接到电脑,如下图所示:
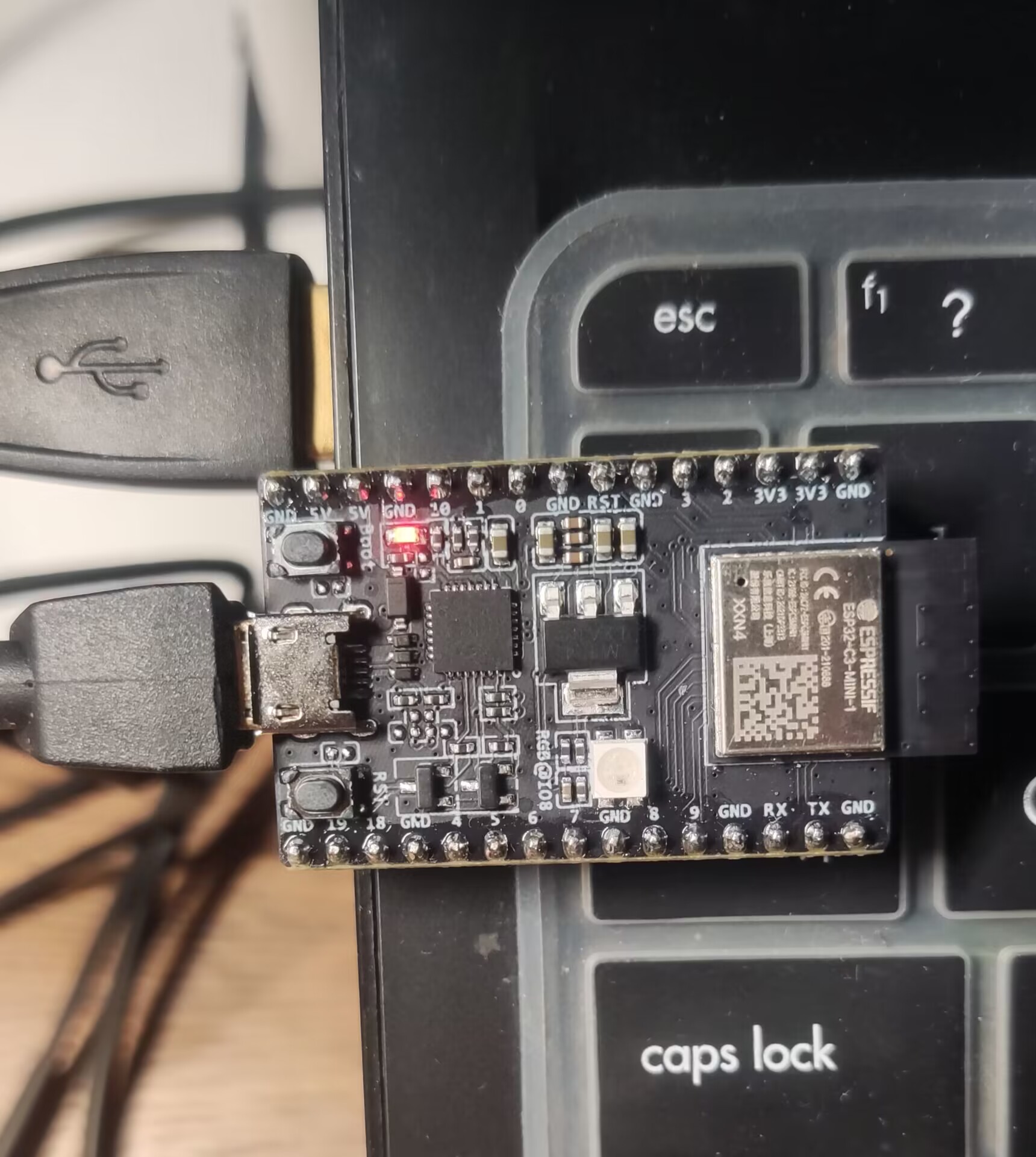
这里使用的开发板是:ESP32-C3-DevKitM-1
选择串口
使用 USB 线将 ESP32 开发板连接到电脑后,然后在 PC 桌面右键单击【此电脑】图标,选择【管理】菜单项,在【计算机管理】中可以查看连接端口,如下图所示:
看到开发板连接的串口,每个人的串口可能不同,我这里是 COM7, 请记住串口名,您会在后续步骤中使用。
回到 VS Code,在下方的工具栏中,点击【COM1】按钮 ,如下图所示:
在弹框框中选择【COM7】:
接着选择项目目录:
这时,串口选择变成了【COM7】,如下图所示:
目标设备
下面开始配置开发板,如下图所示进行操作:
在弹出框中选择【esp32c3】
选择【ESP-PROG】:
选择成功后,工具栏的目标设备位置显示:esp32c3,如下图所示:
配置工程
点击【配置】按钮,如下所示:
出错了!因为项目目录中有中文,把项目拷贝到新的目录下:
F:\05-workspace\dev\01-lab\esp32\book-esp32-c3-guide\src\ch04
使用 VS Code 打开文件夹 hell_world, 点击【配置】按钮,
这时,项目目录下会自动生成一个 名为:sdkconfig 的文件和一个 build 文件夹,如下图所示:
其中,通过如下代码设置目标设备:
CONFIG_IDF_TARGET="esp32c3"
这里可对特定的开发板进行配置,比如:内置的 LED 灯对应的IO脚进行设置,因为该项目没用使用 GPIO, 故这里不用做任何操作,直接关闭即可,如下图所示:
编译工程
点击【build】图标按钮进行构建,如下图所示:
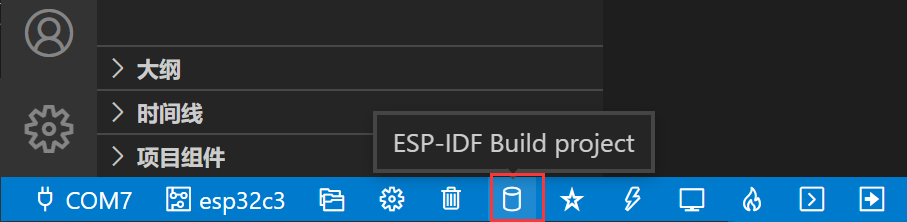
然后开始构建:
过程有点漫长,请耐心等待...
出现如下提示,表示构建成功:
Total sizes:
Used stat D/IRAM: 51602 bytes ( 276078 remain, 15.7% used)
.data size: 7024 bytes
.bss size: 3688 bytes
.text size: 40890 bytes
Used Flash size : 98344 bytes
.text : 71984 bytes
.rodata : 26104 bytes
Total image size: 146258 bytes (.bin may be padded larger)
烧录到设备
点击【flash】按钮,将程序上传到开发板(俗称:烧录),如下图所示:

在弹框中选择【UART】:

然后选择项目目录:

开始烧录:
* 正在执行任务: C:/ProgramFiles/Espressif/python_env/idf4.3_py3.8_env/Scripts/python.exe C:\ProgramFiles\Espressif\frameworks\esp-idf-v4.3.4\components\esptool_py\esptool\esptool.py -p COM7 -b 460800 --before default_reset --after hard_reset --chip esp32c3 write_flash --flash_mode dio --flash_freq 80m --flash_size detect 0x8000 partition_table/partition-table.bin 0x0 bootloader/bootloader.bin 0x10000 hello-world.bin
esptool.py v3.3.2-dev
Serial port COM7
Connecting....
Chip is ESP32-C3 (revision 3)
Features: Wi-Fi
Crystal is 40MHz
MAC: f4:12:fa:03:30:0c
Uploading stub...
Running stub...
Stub running...
Changing baud rate to 460800
Changed.
Configuring flash size...
Auto-detected Flash size: 4MB
Flash will be erased from 0x00008000 to 0x00008fff...
Flash will be erased from 0x00000000 to 0x00004fff...
Flash will be erased from 0x00010000 to 0x00033fff...
Compressed 3072 bytes to 103...
Wrote 3072 bytes (103 compressed) at 0x00008000 in 0.1 seconds (effective 391.1 kbit/s)...
Hash of data verified.
Flash params set to 0x022f
Compressed 20432 bytes to 12167...
Wrote 20432 bytes (12167 compressed) at 0x00000000 in 0.6 seconds (effective 268.2 kbit/s)...
Hash of data verified.
Compressed 146384 bytes to 78299...
Wrote 146384 bytes (78299 compressed) at 0x00010000 in 2.7 seconds (effective 433.9 kbit/s)...
Hash of data verified.
Leaving...
Hard resetting via RTS pin...
当出现如下提示,表示烧录成功。
Leaving...
Hard resetting via RTS pin...
监视输出
点击【监视】按钮,
可以看到输出信息,如下图所示:
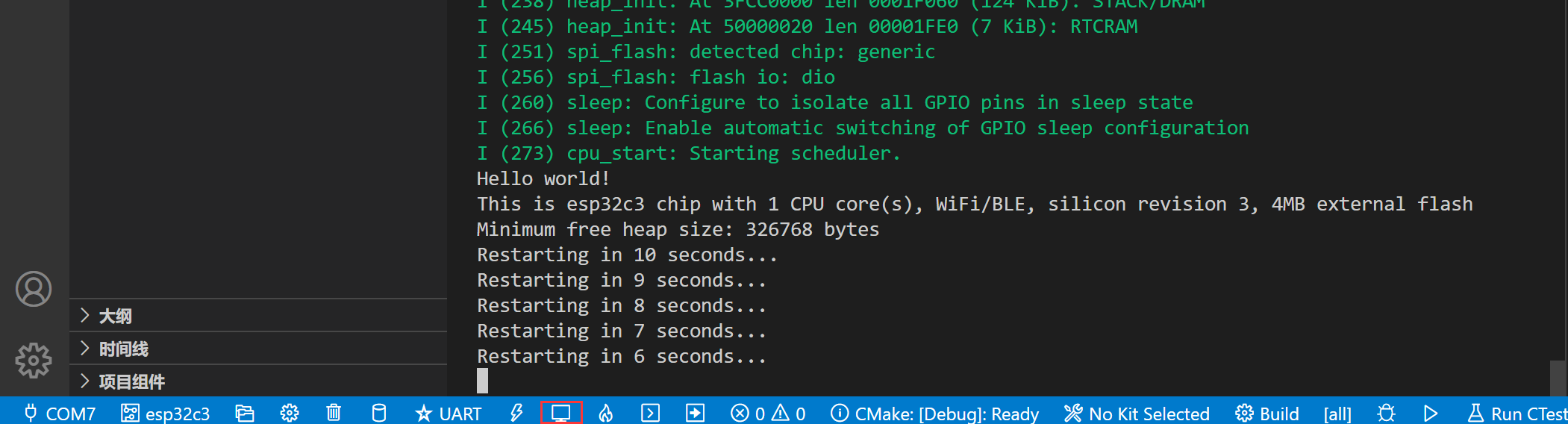
完整的输出信息如下:
F:\05-workspace\dev\01-lab\esp32\book-esp32-c3-guide\src\ch04\hello_world>set IDF_PATH=C:/ProgramFiles/Espressif/frameworks/esp-idf-v4.3.4/
F:\05-workspace\dev\01-lab\esp32\book-esp32-c3-guide\src\ch04\hello_world>C:/ProgramFiles/Espressif/python_env/idf4.3_py3.8_env/Scripts/python.exe C:\ProgramFiles\Espressif\frameworks\esp-idf-v4.3.4\tools\idf_monitor.py -p COM7 -b 115200 --toolchain-prefix riscv32-esp-elf- --target esp32c3 f:\05-workspace\dev\01-lab\esp32\book-esp32-c3-guide\src\ch04\hello_world\build\hello-world.elf
--- WARNING: GDB cannot open serial ports accessed as COMx
--- Using \\.\COM7 instead...
C:\ProgramFiles\Espressif\frameworks\esp-idf-v4.3.4\tools\idf_monitor.py:518: DeprecationWarning: distutils Version classes are deprecated. Use packaging.version instead.
if StrictVersion(serial.VERSION) < StrictVersion('3.3.0'):
--- idf_monitor on \\.\COM7 115200 ---
--- Quit: Ctrl+] | Menu: Ctrl+T | Help: Ctrl+T followed by Ctrl+H ---
ESP-ROM:esp32c3-api1-20210207
Build:Feb 7 2021
rst:0x1 (POWERON),boot:0xc (SPI_FAST_FLASH_BOOT)
SPIWP:0xee
mode:DIO, clock div:1
load:0x3fcd6100,len:0x191c
load:0x403ce000,len:0x8d4
load:0x403d0000,len:0x2d80
SHA-256 comparison failed:
Calculated: cad2e99d2d13fe2764d0287f1986bf5ae3b6c778c7ebb696474c299799b04393
Expected: c4e0839fb749861d811badbc02c51d14ebb0a5e10442504381d759d0270a49c4
Attempting to boot anyway...
entry 0x403ce000
I (48) boot: ESP-IDF v4.3.4 2nd stage bootloader
I (49) boot: compile time 16:45:17
I (49) boot: chip revision: 3
I (50) boot.esp32c3: SPI Speed : 80MHz
I (55) boot.esp32c3: SPI Mode : DIO
I (60) boot.esp32c3: SPI Flash Size : 4MB
I (64) boot: Enabling RNG early entropy source...
I (70) boot: Partition Table:
I (73) boot: ## Label Usage Type ST Offset Length
I (81) boot: 0 nvs WiFi data 01 02 00009000 00006000
I (88) boot: 1 phy_init RF data 01 01 0000f000 00001000
I (96) boot: 2 factory factory app 00 00 00010000 00100000
I (103) boot: End of partition table
I (107) esp_image: segment 0: paddr=00010020 vaddr=3c020020 size=066f8h ( 26360) map
I (120) esp_image: segment 1: paddr=00016720 vaddr=3fc8a000 size=01b70h ( 7024) load
I (126) esp_image: segment 2: paddr=00018298 vaddr=40380000 size=07d80h ( 32128) load
I (139) esp_image: segment 3: paddr=00020020 vaddr=42000020 size=11930h ( 71984) map
I (153) esp_image: segment 4: paddr=00031958 vaddr=40387d80 size=0223ch ( 8764) load
I (155) esp_image: segment 5: paddr=00033b9c vaddr=50000010 size=00010h ( 16) load
I (162) boot: Loaded app from partition at offset 0x10000
I (165) boot: Disabling RNG early entropy source...
I (181) cpu_start: Pro cpu up.
I (190) cpu_start: Pro cpu start user code
I (190) cpu_start: cpu freq: 160000000
I (190) cpu_start: Application information:
I (193) cpu_start: Project name: hello-world
I (198) cpu_start: App version: 1
I (202) cpu_start: Compile time: Feb 15 2023 16:43:03
I (208) cpu_start: ELF file SHA256: 24d1a3f78a5c5519...
I (214) cpu_start: ESP-IDF: v4.3.4
I (219) heap_init: Initializing. RAM available for dynamic allocation:
I (227) heap_init: At 3FC8C9E0 len 00033620 (205 KiB): DRAM
I (233) heap_init: At 3FCC0000 len 0001F060 (124 KiB): STACK/DRAM
I (240) heap_init: At 50000020 len 00001FE0 (7 KiB): RTCRAM
I (246) spi_flash: detected chip: generic
I (251) spi_flash: flash io: dio
I (255) sleep: Configure to isolate all GPIO pins in sleep state
I (261) sleep: Enable automatic switching of GPIO sleep configuration
I (269) cpu_start: Starting scheduler.
Hello world!
This is esp32c3 chip with 1 CPU core(s), WiFi/BLE, silicon revision 3, 4MB external flash
Minimum free heap size: 326768 bytes
Restarting in 10 seconds...
Restarting in 9 seconds...
Restarting in 8 seconds...
Restarting in 7 seconds...
Restarting in 6 seconds...
Restarting in 5 seconds...
Restarting in 4 seconds...
Restarting in 3 seconds...
Restarting in 2 seconds...
Restarting in 1 seconds...
Restarting in 0 seconds...
Restarting now.
ESP-ROM:esp32c3-api1-20210207
Build:Feb 7 2021
rst:0x3 (RTC_SW_SYS_RST),boot:0xc (SPI_FAST_FLASH_BOOT)
Saved PC:0x4038050c
0x4038050c: esp_restart_noos_dig at C:/ProgramFiles/Espressif/frameworks/esp-idf-v4.3.4/components/esp_system/system_api.c:62 (discriminator 1)
SPIWP:0xee
mode:DIO, clock div:1
load:0x3fcd6100,len:0x191c
load:0x403ce000,len:0x8d4
load:0x403d0000,len:0x2d80
SHA-256 comparison failed:
Calculated: cad2e99d2d13fe2764d0287f1986bf5ae3b6c778c7ebb696474c299799b04393
Expected: c4e0839fb749861d811badbc02c51d14ebb0a5e10442504381d759d0270a49c4
Attempting to boot anyway...
entry 0x403ce000
I (53) boot: ESP-IDF v4.3.4 2nd stage bootloader
I (53) boot: compile time 16:45:17
I (54) boot: chip revision: 3
I (55) boot.esp32c3: SPI Speed : 80MHz
I (60) boot.esp32c3: SPI Mode : DIO
I (65) boot.esp32c3: SPI Flash Size : 4MB
I (69) boot: Enabling RNG early entropy source...
I (75) boot: Partition Table:
I (78) boot: ## Label Usage Type ST Offset Length
I (86) boot: 0 nvs WiFi data 01 02 00009000 00006000
I (93) boot: 1 phy_init RF data 01 01 0000f000 00001000
I (101) boot: 2 factory factory app 00 00 00010000 00100000
I (108) boot: End of partition table
I (112) esp_image: segment 0: paddr=00010020 vaddr=3c020020 size=066f8h ( 26360) map
I (125) esp_image: segment 1: paddr=00016720 vaddr=3fc8a000 size=01b70h ( 7024) load
I (131) esp_image: segment 2: paddr=00018298 vaddr=40380000 size=07d80h ( 32128) load
I (144) esp_image: segment 3: paddr=00020020 vaddr=42000020 size=11930h ( 71984) map
I (158) esp_image: segment 4: paddr=00031958 vaddr=40387d80 size=0223ch ( 8764) load
I (160) esp_image: segment 5: paddr=00033b9c vaddr=50000010 size=00010h ( 16) load
I (167) boot: Loaded app from partition at offset 0x10000
I (170) boot: Disabling RNG early entropy source...
I (186) cpu_start: Pro cpu up.
I (195) cpu_start: Pro cpu start user code
I (195) cpu_start: cpu freq: 160000000
I (195) cpu_start: Application information:
I (198) cpu_start: Project name: hello-world
I (203) cpu_start: App version: 1
I (207) cpu_start: Compile time: Feb 15 2023 16:43:03
I (213) cpu_start: ELF file SHA256: 24d1a3f78a5c5519...
I (219) cpu_start: ESP-IDF: v4.3.4
I (224) heap_init: Initializing. RAM available for dynamic allocation:
I (232) heap_init: At 3FC8C9E0 len 00033620 (205 KiB): DRAM
I (238) heap_init: At 3FCC0000 len 0001F060 (124 KiB): STACK/DRAM
I (245) heap_init: At 50000020 len 00001FE0 (7 KiB): RTCRAM
I (251) spi_flash: detected chip: generic
I (256) spi_flash: flash io: dio
I (260) sleep: Configure to isolate all GPIO pins in sleep state
I (266) sleep: Enable automatic switching of GPIO sleep configuration
I (273) cpu_start: Starting scheduler.
......
使用 idf.py 工具
使用 idf.py 工具进行开发,参见:
其实上面在 VS Code 中的所有的操作,底层调用的就是 idf.py 命令,在安装 ESP_IDF 成功后,得到两个 ESP_IDF 终端 ,如下图所示:
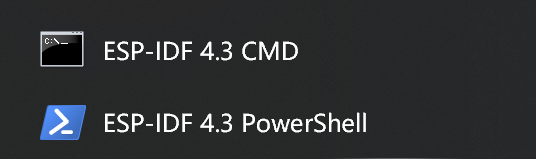
在这两个终端任选其一,也可以在 VS code 中打开,如下图所示:
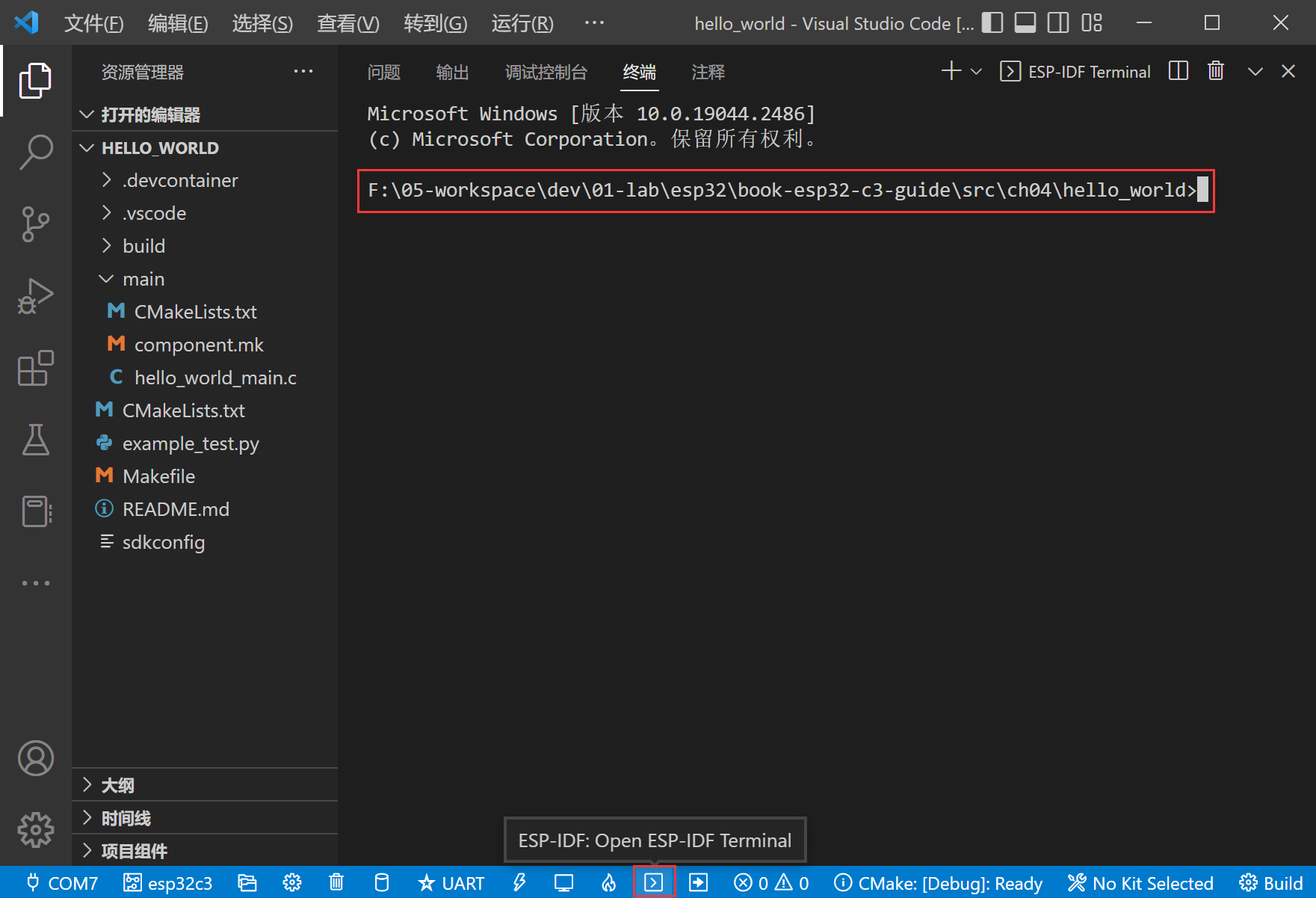
然后就可以使用 idf.py 进行操作了。
这里打开 ESP-IDE PowerShell :
Using Python in C:\ProgramFiles\Espressif\python_env\idf4.3_py3.8_env\Scripts
Python 3.8.7
Using Git in C:\ProgramFiles\Espressif\tools\idf-git\2.34.2\cmd
git version 2.34.1.windows.1
Setting IDF_PATH: C:\ProgramFiles\Espressif\frameworks\esp-idf-v4.3.4
Adding ESP-IDF tools to PATH...
Name Value
---- -----
OPENOCD_SCRIPTS C:\ProgramFiles\Espressif\tools\openocd-esp32\v0.11.0-esp32-20220706\openocd-esp32\sh...
IDF_CCACHE_ENABLE 1
IDF_PYTHON_ENV_PATH C:\ProgramFiles\Espressif\python_env\idf4.3_py3.8_env
Added to PATH
-------------
C:\ProgramFiles\Espressif\frameworks\esp-idf-v4.3.4\components\esptool_py\esptool
C:\ProgramFiles\Espressif\frameworks\esp-idf-v4.3.4\components\app_update
C:\ProgramFiles\Espressif\frameworks\esp-idf-v4.3.4\components\espcoredump
C:\ProgramFiles\Espressif\frameworks\esp-idf-v4.3.4\components\partition_table
C:\ProgramFiles\Espressif\tools\xtensa-esp32-elf\esp-2021r2-patch3-8.4.0\xtensa-esp32-elf\bin
C:\ProgramFiles\Espressif\tools\xtensa-esp32s2-elf\esp-2021r2-patch3-8.4.0\xtensa-esp32s2-elf\bin
C:\ProgramFiles\Espressif\tools\xtensa-esp32s3-elf\esp-2021r2-patch3-8.4.0\xtensa-esp32s3-elf\bin
C:\ProgramFiles\Espressif\tools\riscv32-esp-elf\esp-2021r2-patch3-8.4.0\riscv32-esp-elf\bin
C:\ProgramFiles\Espressif\tools\esp32ulp-elf\2.28.51-esp-20191205\esp32ulp-elf-binutils\bin
C:\ProgramFiles\Espressif\tools\esp32s2ulp-elf\2.28.51-esp-20191205\esp32s2ulp-elf-binutils\bin
C:\ProgramFiles\Espressif\tools\cmake\3.16.4\bin
C:\ProgramFiles\Espressif\tools\openocd-esp32\v0.11.0-esp32-20220706\openocd-esp32\bin
C:\ProgramFiles\Espressif\tools\ninja\1.10.2\
C:\ProgramFiles\Espressif\tools\idf-exe\1.0.1\
C:\ProgramFiles\Espressif\tools\ccache\3.7\
C:\ProgramFiles\Espressif\tools\dfu-util\0.9\dfu-util-0.9-win64
C:\ProgramFiles\Espressif\frameworks\esp-idf-v4.3.4\tools
Checking if Python packages are up to date...
Python requirements from C:\ProgramFiles\Espressif\frameworks\esp-idf-v4.3.4\requirements.txt are satisfied.
Done! You can now compile ESP-IDF projects.
Go to the project directory and run:
idf.py build
打开终端时会自动添加 ESP-IDF 的环境变量。
然后切换到项目的根目录下:
PS C:\ProgramFiles\Espressif\frameworks\esp-idf-v4.3.4> cd F:\05-workspace\dev\01-lab\esp32\book-esp32-c3-guide\src\ch04\hello_world
接着就开始进行命令行操作。
项目的创建
参见:ESP-ID 构建系统: https://docs.espressif.com/projects/esp-idf/zh_CN/latest/esp32/api-guides/build-system.html
选择串口
ESP32 开发板连接 PC 后,在 PC 桌面右键单击【此电脑】图标,选择【管理】菜单项,
看到开发板连接的串口,每个人的串口可能不同,我这里是 COM7, 请记住串口名,您会在后续步骤中使用。
选择设备
idf.py set-target esp32c3
其中,目标设置为:esp32c3
注意,此操作将清除并初始化项目之前的编译和配置(如有) ,
如果执行失败,尝试手动删除在使用 VS Code 编译时产生的 build 文件夹,执行结果如下:
> idf.py set-target esp32c3
Adding "set-target"'s dependency "fullclean" to list of commands with default set of options.
Executing action: fullclean
Build directory 'f:\05-workspace\dev\01-lab\esp32\book-esp32-c3-guide\src\ch04\hello_world\build' not found. Nothing to clean.
Executing action: set-target
Set Target to: esp32c3, new sdkconfig created. Existing sdkconfig renamed to sdkconfig.old.
Running cmake in directory f:\05-workspace\dev\01-lab\esp32\book-esp32-c3-guide\src\ch04\hello_world\build
Executing "cmake -G Ninja -DPYTHON_DEPS_CHECKED=1 -DESP_PLATFORM=1 -DIDF_TARGET=esp32c3 -DCCACHE_ENABLE=1 f:\05-workspace\dev\01-lab\esp32\book-esp32-c3-guide\src\ch04\hello_world"...
-- Found Git: C:/ProgramFiles/Espressif/tools/idf-git/2.34.2/cmd/git.exe (found version "2.34.1.windows.1")
-- ccache will be used for faster recompilation
......
-- Component paths: C:/ProgramFiles/Espressif/frameworks/esp-idf-v4.3.4/components/app_trace C:/ProgramFiles/Espressif/frameworks/esp-idf-v4.3.4/components/app_update ..
......
C:/ProgramFiles/Espressif/frameworks/esp-idf-v4.3.4/components/wpa_supplicant
-- Configuring done
-- Generating done
-- Build files have been written to: F:/05-workspace/dev/01-lab/esp32/book-esp32-c3-guide/src/ch04/hello_world/build
配置工程
使用如下命令进行配置:
idf.py menuconfig
正确操作上述步骤后,系统将显示以下菜单
工程配置 — 主窗口
你可以通过此菜单设置项目的具体变量,包括 Wi-Fi 网络名称、密码和处理器速度等。hello_world
示例项目会以默认配置运行,因此在这一项目中,可以跳过使用 menuconfig
进行项目配置这一步骤
编译工程
请使用以下命令,编译工程:
idf.py build
运行以上命令可以编译应用程序和所有 ESP-IDF 组件,接着生成引导加载程序、分区表和应用程序二进制文件。
> idf.py build
Executing action: all (aliases: build)
Running ninja in directory f:\05-workspace\dev\01-lab\esp32\book-esp32-c3-guide\src\ch04\hello_world\build
Executing "ninja all"...
[0/1] Re-running CMake...
-- ccache will be used for faster recompilation
......
*******************************************************************************
# ESP-IDF Partition Table
# Name, Type, SubType, Offset, Size, Flags
nvs,data,nvs,0x9000,24K,
phy_init,data,phy,0xf000,4K,
factory,app,factory,0x10000,1M,
*******************************************************************************
[27/966] Building C object esp-idf/mbedtls/mbedtls/library/CMakeFiles/mbedcrypto.dir/ecjpake.c.obj
.....
编译需要一段漫长的时间,请耐心等待....
当出现如下提示,表示编译成功
esptool.py v3.3.2-dev
Creating esp32c3 image...
Merged 1 ELF section
Successfully created esp32c3 image.
Generated F:/05-workspace/dev/01-lab/esp32/book-esp32-c3-guide/src/ch04/hello_world/build/bootloader/bootloader.bin
[966/966] Generating binary image from built executable
esptool.py v3.3.2-dev
Creating esp32c3 image...
Merged 1 ELF section
Successfully created esp32c3 image.
Generated F:/05-workspace/dev/01-lab/esp32/book-esp32-c3-guide/src/ch04/hello_world/build/hello-world.bin
Project build complete. To flash, run this command:
C:\ProgramFiles\Espressif\python_env\idf4.3_py3.8_env\Scripts\python.exe C:\ProgramFiles\Espressif\frameworks\esp-idf-v4.3.4\components\esptool_py\esptool\esptool.py -p (PORT) -b 460800 --before default_reset --after hard_reset --chip esp32c3 write_flash --flash_mode dio --flash_size detect --flash_freq 80m 0x0 build\bootloader\bootloader.bin 0x8000 build\partition_table\partition-table.bin 0x10000 build\hello-world.bin
or run 'idf.py -p (PORT) flash'
烧录到设备
请运行以下命令,将刚刚生成的二进制文件烧录至您的 ESP32-C3 开发板:
idf.py -p PORT flash
请将 PORT 替换为 ESP32-C3 开发板的串口名称。如果 PORT
未经定义,idf.py 将尝试使用可用的串口自动连接。
更多有关 idf.py 参数的详情,请见 idf.py。
若在烧录过程中遇到问题,请前往 烧录故障排除 或 与 ESP32 创建串口连接 获取更多详细信息。
在烧录过程中,您会看到类似如下的输出日志 :
> idf.py -p COM7 flash
Executing action: flash
Running ninja in directory f:\05-workspace\dev\01-lab\esp32\book-esp32-c3-guide\src\ch04\hello_world\build
Executing "ninja flash"...
[1/4] Performing build step for 'bootloader'
ninja: no work to do.
[1/2] cmd.exe /C "cd /D C:\ProgramFiles\Espressif\framewor...sp-idf-v4.3.4/components/esptool_py/run_serial_tool.cmake"
esptool.py esp32c3 -p COM7 -b 460800 --before=default_reset --after=hard_reset write_flash --flash_mode dio --flash_freq 80m --flash_size 2MB 0x8000 partition_table/partition-table.bin 0x0 bootloader/bootloader.bin 0x10000 hello-world.bin
esptool.py v3.3.2-dev
Serial port COM7
Connecting....
Chip is ESP32-C3 (revision 3)
Features: Wi-Fi
Crystal is 40MHz
MAC: f4:12:fa:03:30:0c
Uploading stub...
Running stub...
Stub running...
Changing baud rate to 460800
Changed.
Configuring flash size...
Flash will be erased from 0x00008000 to 0x00008fff...
Flash will be erased from 0x00000000 to 0x00004fff...
Flash will be erased from 0x00010000 to 0x00033fff...
Compressed 3072 bytes to 103...
Writing at 0x00008000... (100 %)
Wrote 3072 bytes (103 compressed) at 0x00008000 in 0.1 seconds (effective 391.1 kbit/s)...
Hash of data verified.
Compressed 20432 bytes to 12168...
Writing at 0x00000000... (100 %)
Wrote 20432 bytes (12168 compressed) at 0x00000000 in 0.6 seconds (effective 266.0 kbit/s)...
Hash of data verified.
Compressed 146384 bytes to 78299...
Writing at 0x00010000... (20 %)
Writing at 0x00019c1a... (40 %)
Writing at 0x000203de... (60 %)
Writing at 0x00027729... (80 %)
Writing at 0x0002d8f3... (100 %)
Wrote 146384 bytes (78299 compressed) at 0x00010000 in 2.7 seconds (effective 434.6 kbit/s)...
Hash of data verified.
Leaving...
Hard resetting via RTS pin...
Done
如果一切顺利,烧录完成后,开发板将会复位,应用程序 “hello_world” 开始运行。
监视输出
您可以使用 命令,监视 “hello_world” 工程的运行情况:
idf.py -p PORT monitor
注意,不要忘记将 PORT 替换为您的串口名称。
运行该命令后,IDF 监视器 应用程序将启动
> idf.py -p COM7 monitor
...
Hello world!
This is esp32c3 chip with 1 CPU core(s), WiFi/BLE, silicon revision 3, 2MB external flash
Minimum free heap size: 326768 bytes
Restarting in 10 seconds...
Restarting in 9 seconds...
Restarting in 8 seconds...
Restarting in 7 seconds...
此时,您就可以在启动日志和诊断日志之后,看到打印的 “Hello world!” 了 .
您可使用快捷键 Ctrl+]
,退出 IDF 监视器。
TIPS
您也可以运行以下命令,一次性执行构建、烧录和监视过程:
idf.py -p PORT flash monitor
总结
以上所用到的idf.py
汇总如下:
idf.py set-target esp32c3 # 选择设备(这里的设备是:esp32c3开发板)
idf.py menuconfig # 配置工程
idf.py build # 编译工程
idf.py -p COM7 flash # 烧录到设备
idf.py -p COM7 monitor # 监视输出
idf.py -p COM7 flash monitor # 烧录到设备并监视输出
常用命令详解
在ESP-IDF编译代码的过程中,需要使用CMake项目配置工具、Ninja项目构建工具和esptool烧录工具,每种工具分别在编译、构建、烧录过程中发挥不同作用,同时也支持不同的操作命令。为了便于用户操作,ESP-IDF添加了一个统一前端idf.py,通过idf.py可以快速和连续调用上述的命令。
在使用idf.py之前,需要确保:
● ESP-IDF的环境变量IDF_PATH已经添加到当前终端
● 命令执行目录为工程的根目录,即包含工程编译脚本CMakeLists.txt的目录。
idf.py的常用命令如下:
● idf.py --help:可显示命令列表和使用说明。
● idf.py set-target
● idf.py menuconfig:运行menuconfig终端图像化配置工具,可以选择或修改配置选项,配置结果将保存在sdkconfig文件中。
● idf.py build:开始编译代码,编译产生的中间文件和最终的可执行程序将默认保存在项目的build目录。编译过程是增量式的,如果仅对一个源文件进行修改,在下次编译时将只编译已修改的文件。
● idf.pyclean:清理项目编译产生的中间文件,下次编译时会强制编译整个项目。需要注意,清理时不会删除CMake配置和使用menuconfig修改的配置。
● idf.py fullclean:删除整个build目录下的内容,包括所有CMake的配置输出文件。在下次构建项目时,CMake会从头开始配置项目。请注意,该命令会递归删除构建目录下的所有文件,请谨慎使用,项目配置文件不会被删除。
● idf.py flash:将build生成的可执行程序二进制文件烧录到目标ESP32-C3中。选项-p <port_name> 和-b <baud_rate> 分别用于设置串口的设备名和烧录时的波特率,如果不指定这两个选项,将自动搜索串口并使用默认的波特率。
● idf.py monitor用于显示目标ESP32-C3的串口输出。选项-p可用于设置主机端串口的设备名,在串口打印期间,可按下组合键Ctrl+] 退出监视器。
以上命令也可以组合输入,如命令idf.py build flash monitor将依次进行代码编译、下载、打开串口监视器等操作。
读者可访问 https://docs.espressif.com/projects/esp-idf/zh_CN/v4.3.2/esp32c3/api-guides/build-system.html 阅读ESP-IDF编译系统章节。