driver在不同fixture之间共享
前言:
如果要用到fixture函数的返回值,先return出来,用例传fixture对象就能直接调用了。
fixture参数共享driver
实现场景:
1、用例开始前只打开一次浏览器
2、每个用例开始前,都打开首页,从首页开始运行
3、用例执行完成最后,关闭浏览器
实现方法
1、先定义一个driver函数,设置scope=‘module’,在当前模块全局生效,这样可以做到只启动一次浏览器,就能执行全部用例
2、定义一个start函数,设置scope=‘function’,在每个用例前调用一次
3、addfinalizer终结函数可以在用例全部执行完成后,最后调用driver.quit()退出浏览器
from selenium import webdriver import pytest import time @pytest.fixture(scope='module') def driver(request): driver=webdriver.Chrome(r"D:\chromedriver.exe") print("start chrome浏览器") def end(): driver.quit() request.addfinalizer(end) return driver @pytest.fixture(scope='function') def start(driver): print("open baidu.com") driver.get("https://wwww.baidu.com") time.sleep(1) def test_01(driver,start): print("用例1") driver.find_element_by_id("kw").send_keys("hello") driver.find_element_by_id("su").click() time.sleep(1) print(driver.title) assert 'hello' in driver.title def test_02(driver,start): print("用例2") driver.find_element_by_id("kw").send_keys("hello world!") driver.find_element_by_id("su").click() time.sleep(1) print(driver.title) assert "hello world!" in driver.title if __name__ == '__main__': pytest.main(['-v','test_baidu.py'])
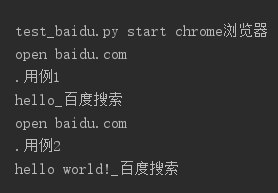
用例用Classs实现
from selenium import webdriver import pytest import time @pytest.fixture(scope='module') def driver(request): driver=webdriver.Chrome(r'D:\chromedriver.exe') print("start chrome浏览器") def end(): driver.quit() request.addfinalizer(end) return driver class TestBaidu(): @pytest.fixture(scope='function',autouse=True)#zutouse=True自动调用fixture功能 def start(self,driver): print("open baidu.com") driver.get("https://www.baidu.com") time.sleep(1) def test_01(self,driver): print("用例01") driver.find_element_by_id('kw').send_keys("hello") driver.find_element_by_id('su').click() time.sleep(1) print(driver.title) assert 'hello' in driver.title def test_02(self,driver): print("用例02") driver.find_element_by_id("kw").send_keys("hello world!") driver.find_element_by_id("su").click() time.sleep(1) print(driver.title) assert 'hello world!' in driver.title if __name__ == '__main__': pytest.main(['-v','test_baiduClass.py'])
posted on 2020-01-19 16:18 crystal1126 阅读(564) 评论(0) 编辑 收藏 举报