微信公众号
前期准备
准备如下
个人只能申请订阅号,而且不能进行微信认证,但是可以申请公众平台测试账号,拥有全部的权限,(首页—>开发者工具—>公众平台测试账号)
接入
在公网部署好一个web项目,一定是80或者443端口。接入指南
pom.xml
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.wx.aeolian</groupId> <artifactId>com.wx.aeolian</artifactId> <version>1.0-SNAPSHOT</version> <dependencies> <!--servlet--> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>3.1.0</version> <scope>provided</scope> </dependency> <!--jsp--> <dependency> <groupId>javax.servlet.jsp</groupId> <artifactId>jsp-api</artifactId> <version>2.2</version> <scope>provided</scope> </dependency> <!--jstl--> <dependency> <groupId>javax.servlet</groupId> <artifactId>jstl</artifactId> <version>1.2</version> <scope>runtime</scope> </dependency> <!--加密工具--> <dependency> <groupId>commons-codec</groupId> <artifactId>commons-codec</artifactId> <version>1.12</version> </dependency> </dependencies> <!--编码方式UTF-8--> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.basedir>D:\ProgramFiles_QY\IdeaProjects\WxAeolian</project.basedir> </properties> <build> <plugins> <!--编译Compile,使用JDK1.7开发--> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.3</version> <configuration> <source>1.7</source> <target>1.7</target> </configuration> </plugin> <!--打包时跳过测试--> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-surefire-plugin</artifactId> <version>2.18.1</version> <configuration> <skipTests>true</skipTests> </configuration> </plugin> <!--Tomcat--> <plugin> <groupId>org.apache.tomcat.maven</groupId> <artifactId>tomcat7-maven-plugin</artifactId> <version>2.2</version> <configuration> <path>/${project.artifactId}</path> </configuration> </plugin> </plugins> </build> </project>
核心Servlet
package com.aeolian.core; import org.apache.commons.codec.digest.DigestUtils; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; import java.util.*; @WebServlet("/wxCore") public class WxServlet extends HttpServlet{ @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws IOException { try { doPost(req,resp); } catch (ServletException e) { e.printStackTrace(); } } /*1)将token、timestamp、nonce三个参数进行字典序排序 *2)将三个参数字符串拼接成一个字符串进行sha1加密 *3)开发者获得加密后的字符串可与signature对比,标识该请求来源于微信*/ @Override protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { String signature = req.getParameter("signature"); String timestamp = req.getParameter("timestamp"); String nonce = req.getParameter("nonce"); String echostr = req.getParameter("echostr"); String token = "微信公众平台中设置的token"; //微信公众平台中配置 /*1)将token、timestamp、nonce三个参数进行字典序排序*/ String[] str = { token, timestamp, nonce }; Arrays.sort(str); // 字典序排序 String sortStr = str[0] + str[1] + str[2]; //排序后拼接 /*2)将三个参数字符串拼接成一个字符串进行sha1加密*/ String digest = DigestUtils.sha1Hex(sortStr); /*System.out.println("signature: "+signature); System.out.println("timestamp: "+timestamp); System.out.println("nonce: "+nonce); System.out.println("echostr: "+echostr); System.out.println("nosha1: "+sortStr); System.out.println("digest: "+digest);*/ /*3)校验*/ String result = ""; if (digest.equals(signature)){ //与signature对比,标识该请求来源于微信 result = echostr; //如果是,返回echostr } resp.resetBuffer(); resp.setContentType("text/html;charset=utf-8"); resp.getOutputStream().write(result.getBytes("utf-8")); resp.getOutputStream().flush(); } }
打成war包是注意事项,在Artifacts中右击Available Elements-》put into /WEB-INF/lib,否则打成的包中没有pom引入的jar
如果这篇文章对你有用,可以关注本人微信公众号获取更多ヽ(^ω^)ノ ~
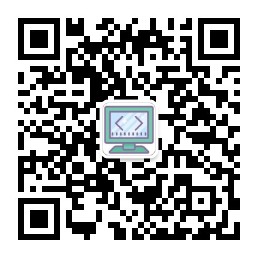