Design 组合模式
基本介绍
组合模式(Composite Pattern),又叫部分整体模式,是用于把一组相似的对象当作一个单一的对象。组合模式依据树形结构来组合对象,用来表示部分以及整体层次。这种类型的设计模式属于结构型模式,它创建了对象组的树形结构。
特点:多个小的构成一个大的,根据不同粒度可以无限制的进行组合
案例图示
举个例子,一个人由躯干、四肢、头部组成。
其实根据不同的粒度,可以有很多种组成的方式,如四肢又包括手脚,头部又包括五官等等。
可以先组成1个小的,再由小的组合成1个大的。
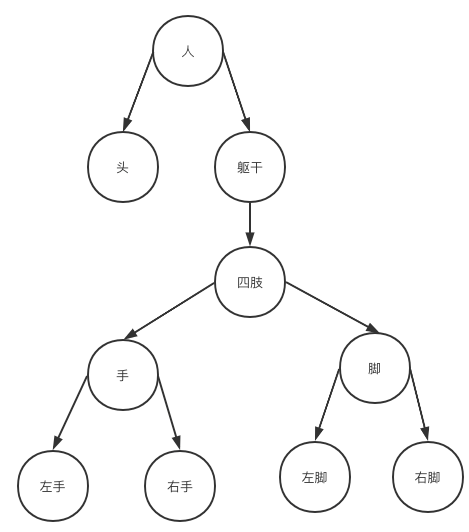
优缺点
优点:
- 高层模块调用简单
- 节点自由增加
缺点:
- 在使用组合模式时,其叶子和树枝的声明都是实现类,而不是接口,违反了依赖倒置原则
Python实现
用Python实现组合模式:
#! /usr/local/bin/python3
# -*- coding:utf-8 -*-
class Base(object):
def __init__(self):
self.composition = []
def add(self, item):
self.composition.extend(item)
def remove(self, item):
self.composition.remove(item)
class Person(Base):
def __init__(self, name):
self.name = name
super(Person, self).__init__()
@property
def desc(self):
desc_info = "%s拥有:\n" % self.name
for obj in self.composition:
desc_info += obj.desc
return desc_info
class Head(Base):
def __init__(self):
super(Head, self).__init__()
@property
def desc(self):
return "\t脑袋\n"
class Body(Base):
def __init__(self):
super(Body, self).__init__()
@property
def desc(self):
desc_info = "\t躯干:"
for obj in self.composition:
desc_info += obj.desc
return desc_info
class Limbs(Base):
def __init__(self, name):
self.name = name
super(Limbs, self).__init__()
@property
def desc(self):
return " %s" % self.name
if __name__ == '__main__':
body = Body()
left_hand = Limbs("左手")
right_hand = Limbs("右手")
left_leg = Limbs("左腿")
right_leg = Limbs("右腿")
body.add(
[
left_hand,
right_hand,
left_leg,
right_leg,
]
)
head = Head()
person = Person("小明")
person.add(
[
head,
body
]
)
print(person.desc)
结果如下:
小明拥有:
脑袋
躯干: 左手 右手 左腿 右腿
Golang实现
用Golang实现组合模式:
...