队列数据结构
队列遵循先进先出原则的一组有序的项。对可在尾部添加新元素并从顶部移除元素。最新添加的元素必须排在队列的末尾
队列类似栈的例子
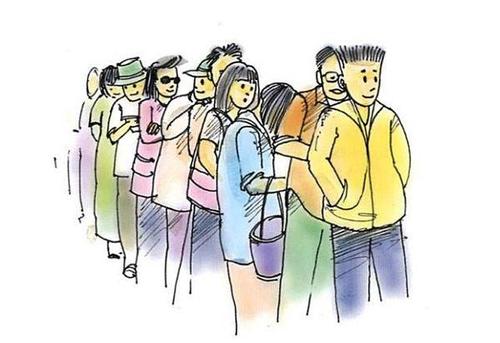
创建队列
- 创建一个类表示队列
- 队列内应该有一些方法
- 添加一个新元素到队列尾部
- 删除队列第一项元素,同时返回这个被删除的元素
- 返回队列第一个元素元素
- 判断栈内是否为空
- 移出栈内所有元素
- 返回栈内元素个数
创建一个类表示队列
class Queue{
constructor(){
// count记录队列最后一项的索引
this.count = 0;
this.items = {};
// lowestCount记录队列第一项的索引
this.lowestCount = 0
}
}
添加一个新元素到队列尾部
inqueue(el){
this.items[this.count] = el;
this.count++;
}
删除队列第一项元素,同时返回这个被删除的元素
requeue(el){
if(this.isEmpty()){
return undefined
}
const result = this.items[this.lowestCount]
delete this.items[this.lowestCount]
this.lowestCount++;
return result;
}
查看队列最后元素
peek(){
if(this.isEmpty()){
return '';
}
return this.items[this.lowestCount]
}
判断队列是否为空
isEmpty(){
return this.size() === 0
}
清空队列元素
clear(){
this.items = {};
this.count = 0
this.lowestCount = 0
}
返回队列元素个数
size(){
return this.count - this.lowestCount;
}
创建双端队列
除以上方法,应该队列的双端的都可以添加删除,同时可以查看 当前队列的第一个元素和最后一个元素
双端队列的前端添加新元素
addFront(el){
if(this.isEmpty()){
this.addBack()
}else if(this.lowestCount > 0){
this.lowestCount--;
this.items[this.lowestCount] = el
}else{
for (let i = this.count; i > 0; i--) {
this.items[i] = this.items[i - 1];
}
this.count++;
this.lowestCount = 0;
this.items[0] = el;
}
}
双端队列的前端删除新元素
removeFront() {
if (this.isEmpty()) {
return undefined;
} else {
delete this.items[this.lowestCount];
this.lowestCount++;
}
}
双端队列的前端第一个元素
peekFront() {
return this.items[this.lowestCount];
}
双端队列的后端添加新元素
addBack(el) {
this.items[this.count] = el;
this.count++;
}
双端队列的后端删除最后一个元素
removeBack() {
if (this.isEmpty()) {
return "0";
} else {
delete this.items[this.count - 1];
this.count--;
}
}
双端队列的后端最后一个元素
peekBack() {
return this.items[this.count - this.lowestCount];
}
判断队列是否为空
isEmpty(){
return this.size() === 0
}
清空队列元素
clear(){
this.items = {};
this.count = 0
this.lowestCount = 0
}
返回队列元素个数
size(){
return this.count - this.lowestCount;
}