C#使用SQL语句时候的万用密码问题
实际上万用密码就是因为SQL里面的语句--是注释,利用bug添加用户名和密码。
例如,用户名为 adada’ or 1=1--
第一个种写法登录成功了
第二种写法登录失败(正确)
第三种写法登录失败(正确)
测试代码
数据库部分
create database ThreeDb go USE ThreeDb; GO CREATE TABLE classify --分类表 ( id int primary key identity(1,1), name nvarchar(20) not null ) GO CREATE TABLE product --产品表 ( id int primary key identity(1,1), name nvarchar(20) not null, price decimal, number int default 0, c_id int FOREIGN KEY references classify(id) ) GO CREATE TABLE users ( id int primary key identity(1,1), name nvarchar(20) not null, pwd nvarchar(20) not null ) GO --添加分类测试数据 insert into classify(name) values('图书'); insert into classify(name) values('家电'); insert into classify(name) values('服饰'); --添加users的测试数据 insert into users(name,pwd) values('admin','admin'); --添加商品测试数据 insert into product(name,price,number,c_id) values('arduino基础版',168,50,1); insert into product(name,price,number,c_id) values('arduino提高版',268,50,1); insert into product(name,price,number,c_id) values('arduino至尊版',468,50,1); insert into product(name,price,number,c_id) values('比基尼',68,50,3); insert into product(name,price,number,c_id) values('虎皮裙',168,50,3); insert into product(name,price,number,c_id) values('长靴',368,50,3); insert into product(name,price,number,c_id) values('电冰箱',3268,50,2); insert into product(name,price,number,c_id) values('烘干机',2268,50,2); GO select count(*) from users where name='admin' and pwd='admin' go select count(*) from users where name='xxxdadad' or 1=1 --' and pwd='admin' go select * from classify; go select * from product order by c_id; go
C#部分
form1.cs
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; using System.Data.SqlClient; using System.Configuration; namespace AdoTestForm { public partial class Form1 : Form { string constr = "server=QT-201303030913;database=ThreeDb;uid=sa;pwd=daxiang"; public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { comboBox1.Text = "0-->全部分类"; //确定数据库连接字符串 //string constr = "server=QT-201303030913;database=OneDb;uid=sa;pwd=daxiang"; //string constr = ConfigurationManager.ConnectionStrings["str"].ConnectionString; //实例化一个数据库连接的对象 SqlConnection conn = new SqlConnection(constr); //打开数据库连接 conn.Open(); //实例化SqlCommand对象(该对象主要用于执行Sql命令) SqlCommand cmd = new SqlCommand(); //指定要执行的SQL语句或者存储过程名称 cmd.CommandText = "select id,name from classify"; //确定上面为CommandText类型所赋的值是SQL语句还是存储过程名称 cmd.CommandType = CommandType.Text; //指定该命令所用的数据库连接 cmd.Connection = conn; //声明一个SqlDataReader(数据流对象),并将cmd执行后的结果交给它 SqlDataReader sdr = cmd.ExecuteReader(); //循环整个SqlDataReader对象,将里面的值取出来添加到ListBox中 //sdr.Read()的作用是前进到下一条记录,这也说明SqlDataReader中的数据是一行行放置的 while (sdr.Read()) { //将数据流中的第一列的值添加到listBox1的项中 comboBox1.Items.Add(sdr[0]+"-->"+sdr["name"]); //上面这句也可以用下面这句代替,sdr["name"]表示当前sdr的name列的值 //comboBox1.Items.Add(str["name"]); } //关闭数据流 sdr.Close(); //关闭数据库连接 conn.Close(); using (SqlConnection conn2 = new SqlConnection(constr)) { conn2.Open(); SqlCommand cmd2 = new SqlCommand("select count(*) from product", conn2); string sum = cmd2.ExecuteScalar().ToString(); label2.Text = string.Format("共计{0}件商品",sum); } } private void button1_Click(object sender, EventArgs e) { if (comboBox1.Text != "0-->全部分类") { string constr = "server=QT-201303030913;database=ThreeDb;uid=sa;pwd=daxiang"; //实例化一个数据库连接的对象 SqlConnection conn = new SqlConnection(constr); //打开数据库连接 conn.Open(); //实例化SqlCommand对象(该对象主要用于执行Sql命令) SqlCommand cmd = new SqlCommand(); //获取分类的id //int i = comboBox1.Text.IndexOf("-->");//获取字符串中-->所在位置索引 string id = comboBox1.Text.Substring(0, 1);//只获取-->之前的字符 //指定要执行的SQL语句和存储过程名字 cmd.CommandText = "select * from product where c_id=" + id; //去顶上面的CommandText属性所赋值到底是sql还是存储过程名称 cmd.CommandType = CommandType.Text; //指定该命令所用的数据库连接 cmd.Connection = conn; //声明一个SqlDataReader(数据流对象),并将cmd执行后的结果交给它 SqlDataReader sdr = cmd.ExecuteReader(); //清空listBox中的项 listBox1.Items.Clear(); //循环整个SqlDataReader对象,将里面的值取出来添加到ListBox中 //sdr.Read()的作用是前进到下一条记录,这也说明SqlDataReader中的数据是一行行放置的 while (sdr.Read()) { //将数据流中的第一列的值添加到listBox1的项中 listBox1.Items.Add(sdr["id"]+":"+sdr["name"]); } //关闭数据流 sdr.Close(); //关闭数据库连接 conn.Close(); } } private void listBox1_SelectedIndexChanged(object sender, EventArgs e) { button2.Text = "修改"; using (SqlConnection conn = new SqlConnection(constr)) { int index = listBox1.Text.IndexOf(":"); //获取-的位置 string id = listBox1.Text.Substring(0,index);//只获取-之前的字符 string sql = "select * from product where id=" + id; SqlCommand cmd = new SqlCommand(sql, conn); conn.Open(); SqlDataReader sdr = cmd.ExecuteReader(); while(sdr.Read()) { tb_name.Text = sdr["name"].ToString(); tb_price.Text = sdr["price"].ToString(); tb_number.Text = sdr["number"].ToString(); } sdr.Close(); } } private void button2_Click(object sender, EventArgs e) { switch (((Button)sender).Text) { case "添加": using (SqlConnection conn = new SqlConnection(constr)) { int index = comboBox1.Text.IndexOf("-->"); string id = comboBox1.Text.Substring(0, index); if (int.Parse(id) > 0) { string sql = "insert into product(name,price,number,c_id) values('"+ tb_name.Text +"',"+ tb_price.Text+ ","+ tb_number.Text +","+ id + ")"; SqlCommand cmd = new SqlCommand(sql, conn); conn.Open(); int i = cmd.ExecuteNonQuery(); if (i > 0) { MessageBox.Show("添加成功" + i.ToString() + "行数据"); } else { MessageBox.Show("好囧,一行数据都没加上"); } } else { MessageBox.Show("你敢不敢先选个分类,亲!"); } } break; case "修改": using (SqlConnection conn = new SqlConnection(constr)) { //获取当前选择行的id int index = listBox1.Text.IndexOf(":"); string id = listBox1.Text.Substring(0, index); //获取分类的id int index2 = comboBox1.Text.IndexOf("-->"); string c_id = comboBox1.Text.Substring(0, index2); string sql = "UPDATE product SET name = '" + tb_name.Text + "',price =" + tb_price.Text + ",number = " + tb_number.Text + ",c_id = " + c_id + "WHERE id = "+id; SqlCommand cmd = new SqlCommand(sql, conn); conn.Open(); int i = cmd.ExecuteNonQuery(); if (i > 0) { MessageBox.Show("修改成功" + i.ToString() + "行数据"); } else { MessageBox.Show("好囧,一行数据都没修改到"); } } break; } } private void button3_Click(object sender, EventArgs e) { using (SqlConnection conn = new SqlConnection(constr)) { //获取当前选择行的id int index = listBox1.Text.IndexOf(":"); string id = listBox1.Text.Substring(0, index); string sql = "delete from product WHERE id = " + id; SqlCommand cmd = new SqlCommand(sql, conn); conn.Open(); int i = cmd.ExecuteNonQuery(); if (i > 0) { MessageBox.Show("删除成功" + i.ToString() + "行数据"); } else { MessageBox.Show("好囧,一行数据都没删除到"); } } } //当前窗体关闭,则推出当前程序 private void Form1_FormClosing(object sender, FormClosingEventArgs e) { Application.Exit(); } //private void listBox1_SelectedIndexChanged(object sender, EventArgs e) //{ // if (listBox1.SelectedItems.Count > 0) // { // string constr = "server=QT-201303030913;database=ThreeDb;uid=sa;pwd=daxiang"; // //实例化一个数据库连接的对象 // SqlConnection conn = new SqlConnection(constr); // //打开数据库连接 // conn.Open(); // //实例化SqlCommand对象(该对象主要用于执行Sql命令) // SqlCommand cmd = new SqlCommand(); // //获取分类的id // //int i = comboBox1.Text.IndexOf("-->");//获取字符串中-->所在位置索引 // string id = comboBox1.Text.Substring(0, 1);//只获取-->之前的字符 // //指定要执行的SQL语句和存储过程名字 // cmd.CommandText = "select * from product where c_id=" + id; // //去顶上面的CommandText属性所赋值到底是sql还是存储过程名称 // cmd.CommandType = CommandType.Text; // //指定该命令所用的数据库连接 // cmd.Connection = conn; // //声明一个SqlDataReader(数据流对象),并将cmd执行后的结果交给它 // SqlDataReader sdr = cmd.ExecuteReader(); // //循环整个SqlDataReader对象,将里面的值取出来添加到ListBox中 // //sdr.Read()的作用是前进到下一条记录,这也说明SqlDataReader中的数据是一行行放置的 // while (sdr.Read()) // { // if (sdr["name"].ToString() == listBox1.SelectedItem.ToString()) // { // lbl_name.Text = sdr["name"].ToString(); // lbl_price.Text = sdr["price"].ToString(); // lbl_number.Text = sdr["number"].ToString(); // lbl_c_id.Text = comboBox1.Text; // } // } // //关闭数据流 // sdr.Close(); // //关闭数据库连接 // conn.Close(); // } //} } }
form2.cs
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; using System.Data.SqlClient; namespace AdoTestForm { public partial class Form2 : Form { public Form2() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { string constr = "server=QT-201303030913;database=ThreeDb;uid=sa;pwd=daxiang"; SqlConnection conn = new SqlConnection(constr); SqlCommand cmd = new SqlCommand(); try { conn.Open(); cmd.CommandText = "select count(*) from users where name='" + tb_uname.Text + "' and pwd = '" + tb_pwd.Text + "'"; cmd.Connection = conn; int count = Convert.ToInt32(cmd.ExecuteScalar()); if (count > 0) { MessageBox.Show("哈哈,登陆上了哦!"); } else { MessageBox.Show("插,忘记密码了!"); } } catch (System.Exception ex) { MessageBox.Show(ex.Message); return; } finally { conn.Close(); } #region 使用参数 try { conn.Open(); cmd.CommandText = "select count(*) from users where name=@name and pwd=@pwd"; cmd.Connection = conn; //参数 cmd.Parameters.AddWithValue("@name", tb_uname.Text); cmd.Parameters.AddWithValue("@pwd", tb_pwd.Text); int count = Convert.ToInt32(cmd.ExecuteScalar()); if (count > 0) { MessageBox.Show("哈哈,登陆上了哦!"); } else { MessageBox.Show("插,忘记密码了!"); } ; } catch (System.Exception ex) { MessageBox.Show(ex.Message); return; } finally { conn.Close(); } #endregion //参数化类 try { conn.Open(); cmd.CommandText = "select count(*) from users where name=@name1 and pwd=@pwd1"; cmd.Connection = conn; //参数 //方式1 SqlParameter uname = new SqlParameter("@name1", SqlDbType.VarChar, 40, "name"); uname.Value = tb_uname.Text; //方式2 SqlParameter pwd = new SqlParameter("@pwd1", tb_pwd.Text); cmd.Parameters.Add(uname); cmd.Parameters.Add(pwd); int count = Convert.ToInt32(cmd.ExecuteScalar()); if (count > 0) { MessageBox.Show("哈哈,登陆上了哦!"); } else { MessageBox.Show("插,忘记密码了!"); } ; } catch (System.Exception ex) { MessageBox.Show(ex.Message); return; } finally { conn.Close(); } } private void button2_Click(object sender, EventArgs e) { Form1 f1 = new Form1(); f1.Show(); this.Hide(); } } }
form1和form2的设计界面
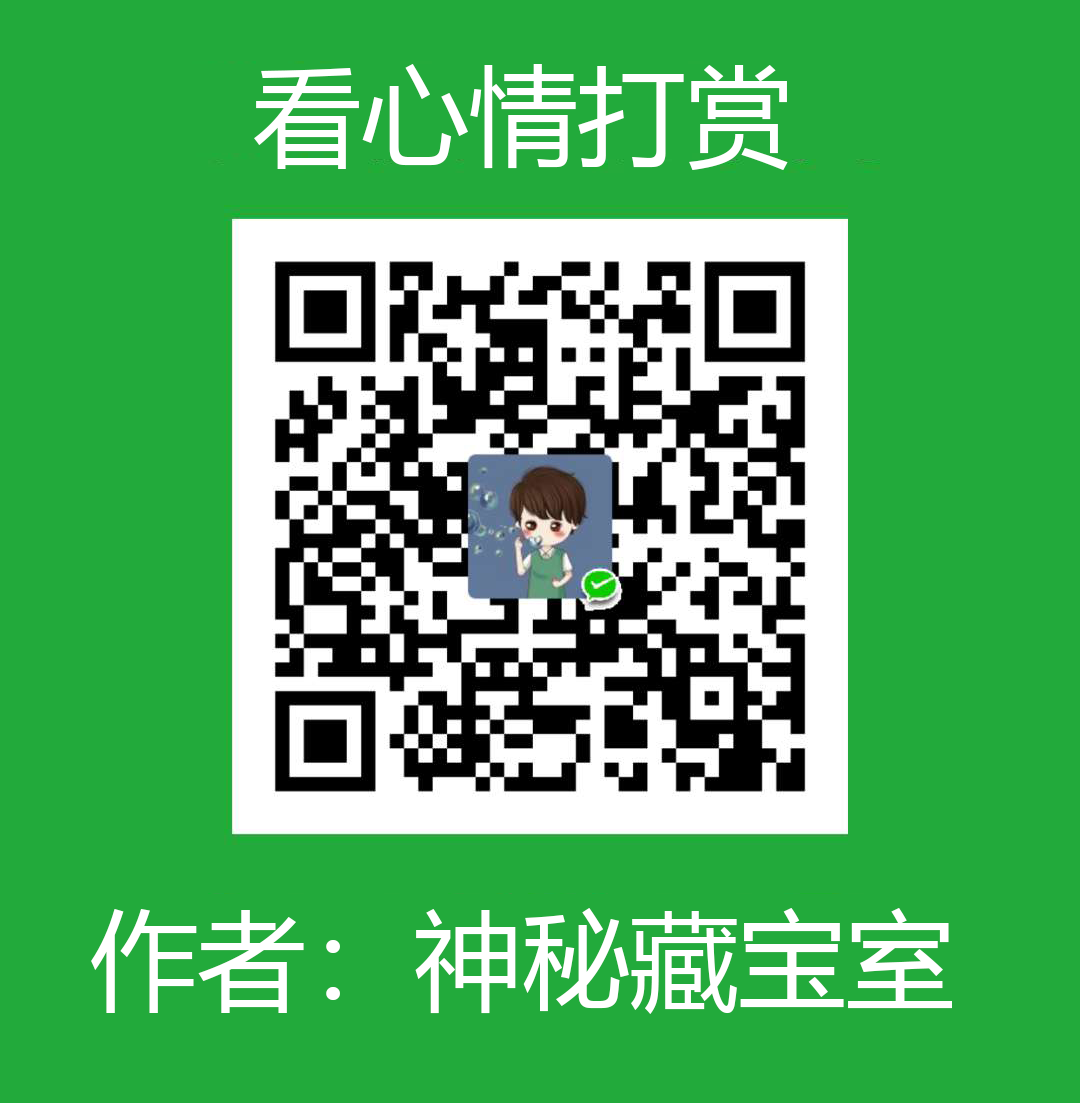
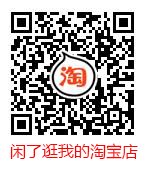
文章对您有帮助,开心可以打赏我,金额随意,欢迎来赏!
需要电子方面开发板/传感器/模块等硬件可以到我的淘宝店逛逛