C#编写图书列表winform
Book.cs文件
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Collections; namespace Booklist { public class Book { /// <summary> /// 作者 /// </summary> public string Author { get; set; } /// <summary> /// ISBN /// </summary> public string Isbn { get; set; } /// <summary> /// 书名 /// </summary> public string Name { get; set; } /// <summary> /// 价格 /// </summary> public double Price { get; set; } /// <summary> /// 重载构造函数 /// </summary> public Book() { } /// <summary> /// 带参数的构造函数 /// </summary> /// <param name="n">名称</param> /// <param name="s">ISDN</param> /// <param name="p">价格</param> /// <param name="a">作者</param> public Book(string n, string s, double p, string a) { Name = n; Isbn = s; Price = p; Author = a; } } public class Store { private ArrayList booklist; /// <summary> /// 图书列表 /// </summary> public Store() { booklist = new ArrayList(); } /// <summary> /// 获取图书列表 /// </summary> public ArrayList Booklist { get { return booklist; } set { booklist = value; } } /// <summary> /// 从仓库中获取指定书名的书籍 /// </summary> /// <param name="name">名称</param> /// <returns>书的信息</returns> public Book GetBook(string name) { Book book = new Book(); foreach(Book b in booklist) { if (b.Name == name) { book = b; } } return book; } /// <summary> /// 判断指定书名的书籍是否存在 /// </summary> /// <param name="n">书名</param> /// <returns>存在返回true</returns> public bool Exists(string n) { bool s = false; foreach (Book b in booklist) { if (b.Name == n) { s = true; } } return s; } /// <summary> /// 从列表中增加一个图书 /// </summary> /// <param name="b">书的对象</param> /// <returns>列表</returns> public ArrayList AddBook(Book b) { booklist.Add(b); return booklist; } /// <summary> /// 移除指定名称的图书 /// </summary> /// <param name="b">要移除的图书名称</param> /// <returns>处理的结果</returns> public ArrayList Remove(Book b) { if (booklist.Contains(b)) booklist.Remove(b); return booklist; } /// <summary> /// 移除指定书名的图书 /// </summary> /// <param name="n">书名</param> /// <returns>处理后的结果</returns> public ArrayList Remove(string n) { ArrayList temp = (ArrayList)booklist.Clone(); foreach (Book b in temp) { if (b.Name == n) { booklist.Remove(b); } } return booklist; } } }
form1.cs
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; namespace Booklist { public partial class Form1 : Form { Store st; //声明一个仓库的全局变量,作为数据存储的变量 public Form1() { InitializeComponent(); Book b = new Book("神秘藏宝室开发板套件基础版","1122-334",134d,"小白"); Book b1 = new Book("神秘藏宝室开发板套件提高版", "1122-335", 134d, "小白"); Book b2 = new Book("神秘藏宝室开发板套件至尊版", "1122-336", 134d, "小白"); st = new Store(); st.AddBook(b); st.AddBook(b1); st.AddBook(b2); Bind(); } /// <summary> /// 填充数据 /// </summary> private void Bind() { cb_list.Items.Clear(); //清空下拉列表 cb_list.Text = "请选择一本书"; //显示一个默认值 foreach(Book b in st.Booklist) { cb_list.Items.Add(b.Name); } lb_output.Text = "当前仓库中共计" + st.Booklist.Count.ToString() + "本书"; } private void button1_Click(object sender, EventArgs e) { //出库 if (st.Exists(cb_list.Text)) { st.Remove(cb_list.Text); cb_list.Text = "删除成功"; tb_name.Text = ""; tb_isdn.Text = ""; tb_price.Text = ""; tb_author.Text = ""; } Bind(); //重新填充数据 } private void button2_Click(object sender, EventArgs e) { if (st.Exists(cb_list.Text)) { Book b = st.GetBook(cb_list.Text); lb_output.Text = string.Format("书名:{0},\n作者:{1},\n价格:{2},\nISBN:{3}", b.Name, b.Author, b.Price, b.Isbn); } else { lb_output.Text = "不存在的书籍"; } } private void button3_Click(object sender, EventArgs e) { if (tb_name.Text != "" && tb_author.Text != "" && tb_price.Text != "" && tb_isdn.Text != "") { Book b = new Book( tb_name.Text, tb_isdn.Text, double.Parse(tb_price.Text), tb_author.Text ); st.AddBook(b); Bind(); } } private void cb_list_SelectedIndexChanged(object sender, EventArgs e) { Book b = st.GetBook(cb_list.Text); tb_name.Text = b.Name; tb_isdn.Text = b.Isbn; tb_price.Text = b.Price.ToString(); tb_author.Text = b.Author; } } }
winform界面
最终运行界面:
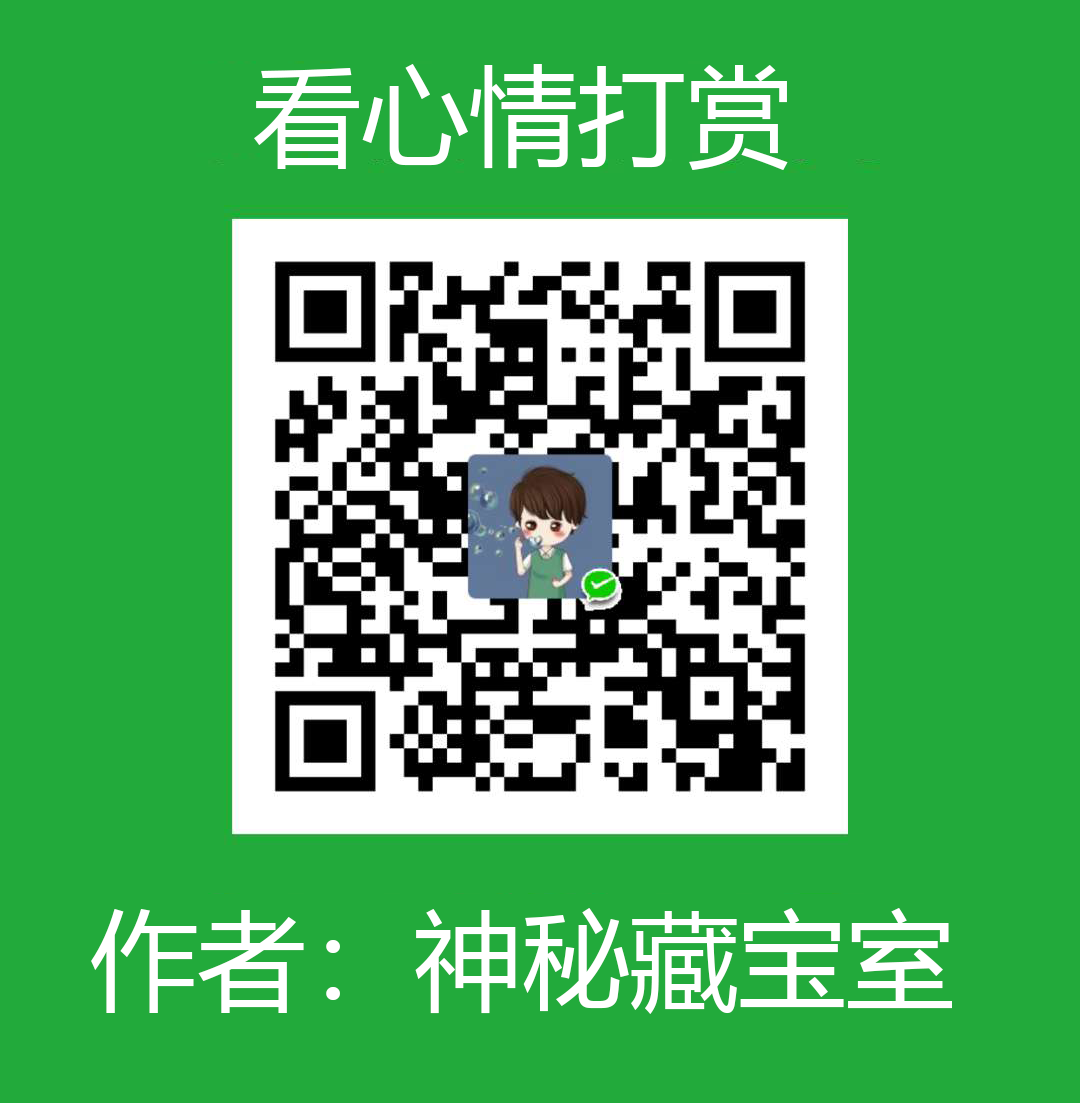
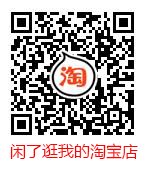
文章对您有帮助,开心可以打赏我,金额随意,欢迎来赏!
需要电子方面开发板/传感器/模块等硬件可以到我的淘宝店逛逛