一:绑定方法
是一个函数就应该有一个参数,方便对象只要一调就能够传进来
定义类
class Student:
定义一个属性
school = "SH"
def __init__(self, name, age, gender, courses=None):
if courses is None:
courses = []
self.name = name
self.age = age
self.gender = gender
self.courses = courses
定义一个技能
def choose_course(self, course):
self.courses.append(course)
print('学生 %s 选课成功 %s' % (self.name, self.courses))
stu1_obj = Student('egon', 18, 'male') # 产生一个空字典指向类
产生对象调用类
stu2_obj = Student('tom', 38, 'female')
调用类产生对象,默认产生的就是一个空字典
类中有两种属性
1.数据属性
print(Student.school) SH
2.函数属性
print(Student.choose_course) <function Student.choose_course at 0x0000025300E51510>
Student.choose_course(stu1_obj, 'python开发') 学生 egon 选课成功 ['python开发']
但是类中的属性其实是为对象准备
1.类的数据属性是直接共享给所以对象用的
print(Student.school,id(Student.school)) SH 2024079274704
print(stu1_obj.school,id(Student.school)) SH 2024079274704
print(stu2_obj.school,id(Student.school)) SH 2024079274704
2.改属性
Student.school = 'xxx'
print(stu1_obj.school) xxx
print(stu2_obj.school) xxx
stu1_obj.school = 'xxx'
print(stu1_obj.school) xxx
print(stu2_obj.school) SH
print(Student.school) SH
类的属性值改了 所以对象都跟着改
2.类的函数属性是绑定给对象用的
print(Student.choose_course) 类去访问 就是一个函数 内存地址
结果:<function Student.choose_course at 0x000002359E751510>
print(stu1_obj.choose_course) 绑定方法 内存地址
结果:<bound method Student.choose_course of <__main__.Student object at 0x000002359E73BCF8>>
print(stu2_obj.choose_course) 绑定方法 内存地址
结果:<bound method Student.choose_course of <__main__.Student object at 0x000002359E607EB8>>
绑定方法会把自己传进去
stu1_obj.choose_course('python') 学生 egon 选课成功 ['python']
绑定方法: Student.choose_course(stu_obj, 'python')
stu2_obj.choose_course('linux') 学生 tom 选课成功 ['linux']
python3中统一了类与类型的概率 数据类型就是类,一个类也是一个数据类型
l1 = [11,22,33]
list:类
l1 = list[11,22,33]
l1.append(44)
list.append(l1,44)
print(type(l1)) <class 'list'>
一切皆类对象
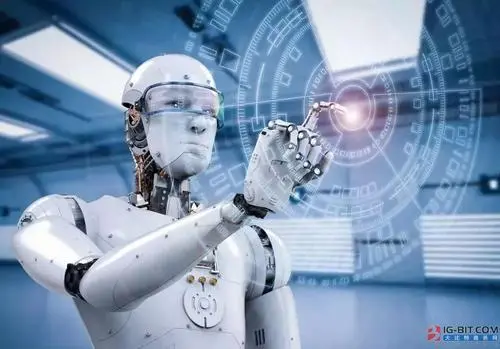
二:属性的查找顺序
class Student():
# 定义一个属性
school = 'SH'
def __init__(self, name, age, gender, course=None):
if course is None:
course = []
self.name = name # stu1.__dict__['name'] = 'jason'
self.age = age # stu1.__dict__['age'] = 18
self.gender = gender # stu1.__dict__['gender'] = 'male'
self.course = course
def choose_course(self, course):
# stu_dict => stu
self.course.append(course)
print('%s选课成功 %s' % (self.name, self.course))
def func(self, name, age):
pass
属性的查找:
1. 类属性: 在类中写的属性就称为类属性
2. 对象属性:在对象自己的名称空间中的属性就是对象属性
类属性的查找
1. 查
print(Student.school)
2. 增加
Student.country = 'China'
print(Student.country)
stue = Student('jaon', 18, 'ses')
print(stue.country)
3. 改
Student.school = 'BJ'
4. 删除
del Student.school
print(Student.__dict__)
{}
stu = Student('ly', 18, 'male') # 实例化对象, stu就是一个实例
对象属性
1. 查
print(stu.name)
print(stu.age)
print(stu.gender)
2. 增
stu.aaa = 'aaa'
3. 改
stu.name = 'bbb'
4. 删除
del stu.name
print(stu.__dict__)
类中的方法,类可以调用,对象也可以调用
类调用方法
Student.choose_course(stu, 'python') # 类来调用,函数的参数有几个就要传几个
print(Student.choose_course)
对象调用方法
类中的方法,类可以调用,对象也可以调用, 但是,推荐对象来调用,因为对象来调用,会把自己当成第一个参数传递给函数
stu.choose_course('python') # stu.choose_course(stu, 'python')
class Teacher():
pass
print(isinstance(123, int))
print(isinstance(stu, Teacher))