一:字符串的内置方法
1.大小写
res = 'jasOn123 JASon'
res1 = 'jason123'
转全大写
print(res.upper())
#JASON123 JASON
转全小写
print(res.lower())
#jason123 jason
我们在登录网站的时候会出现图片验证码
'实际案例:图片验证码忽略大小写
思路:全部转大写或者小写在比对
可以做到图片验证码忽略大小写了'
# 图片验证码底层原理
old_code = 'JaSon123'
print('这是返回给用户的图片验证码: %s' % old_code)
new_code = input('请输入验证码:').strip()
if old_code.upper() == new_code.upper(): # upper()大小写转换 这里也可以是lower()
print('验证码正确')
else:
print('验证码错误')
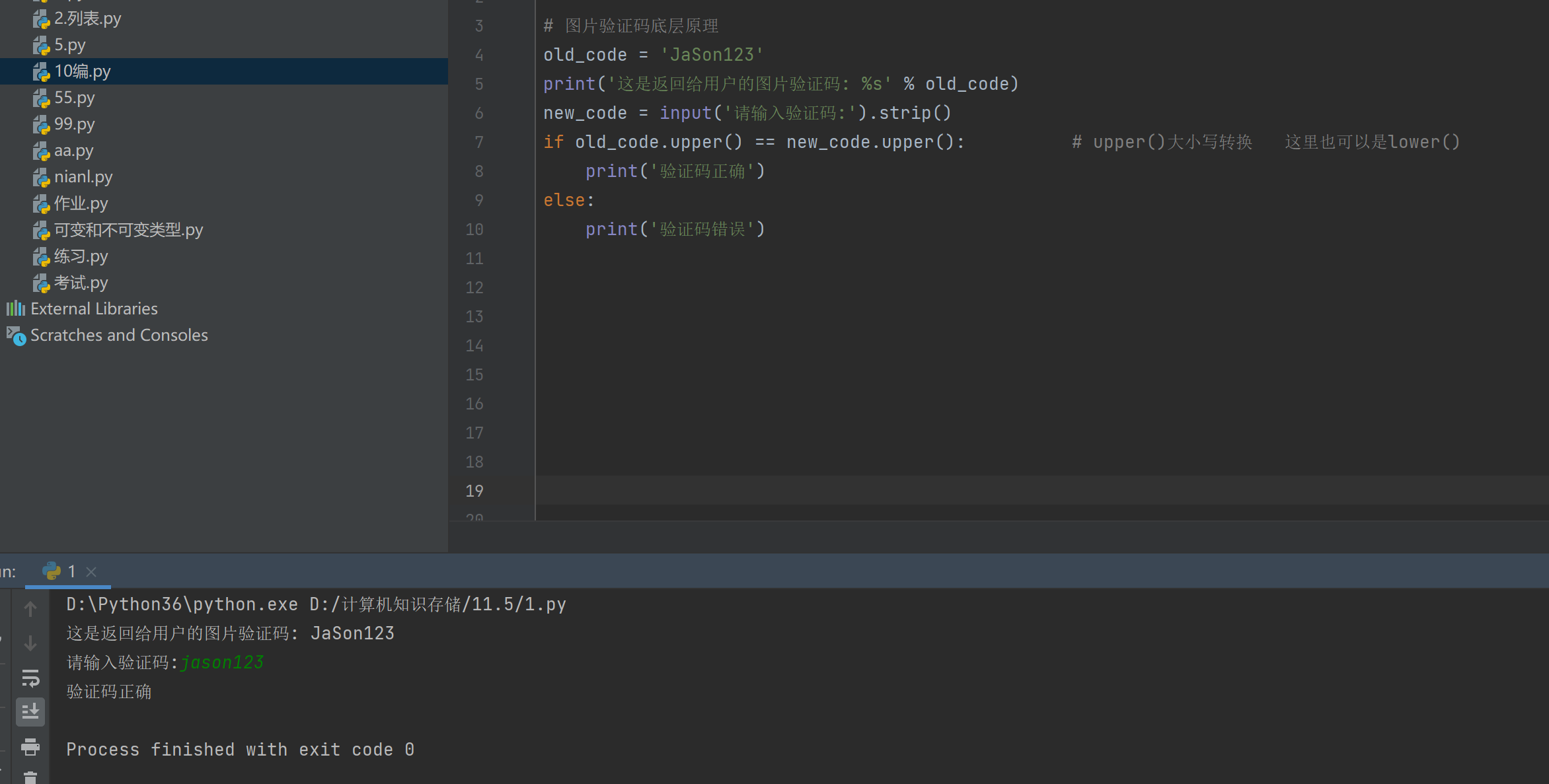
二:字符串内置操作
res = 'JasOn123 JAson'
res1 = 'jason123'
# 判断是否是纯大写 isupper()是否纯大写
print(res.isupper()) # is开头,一个变量名或者一个方法名,用is开头这个变量所指的值,这个方法的结果,肯定是布尔值
# False
print(res1.isupper())
# False
# 只判断英文
# 判断是否是纯小写 islower()是否纯小写
print(res.islower())
# False
print(res1.islower())
# True
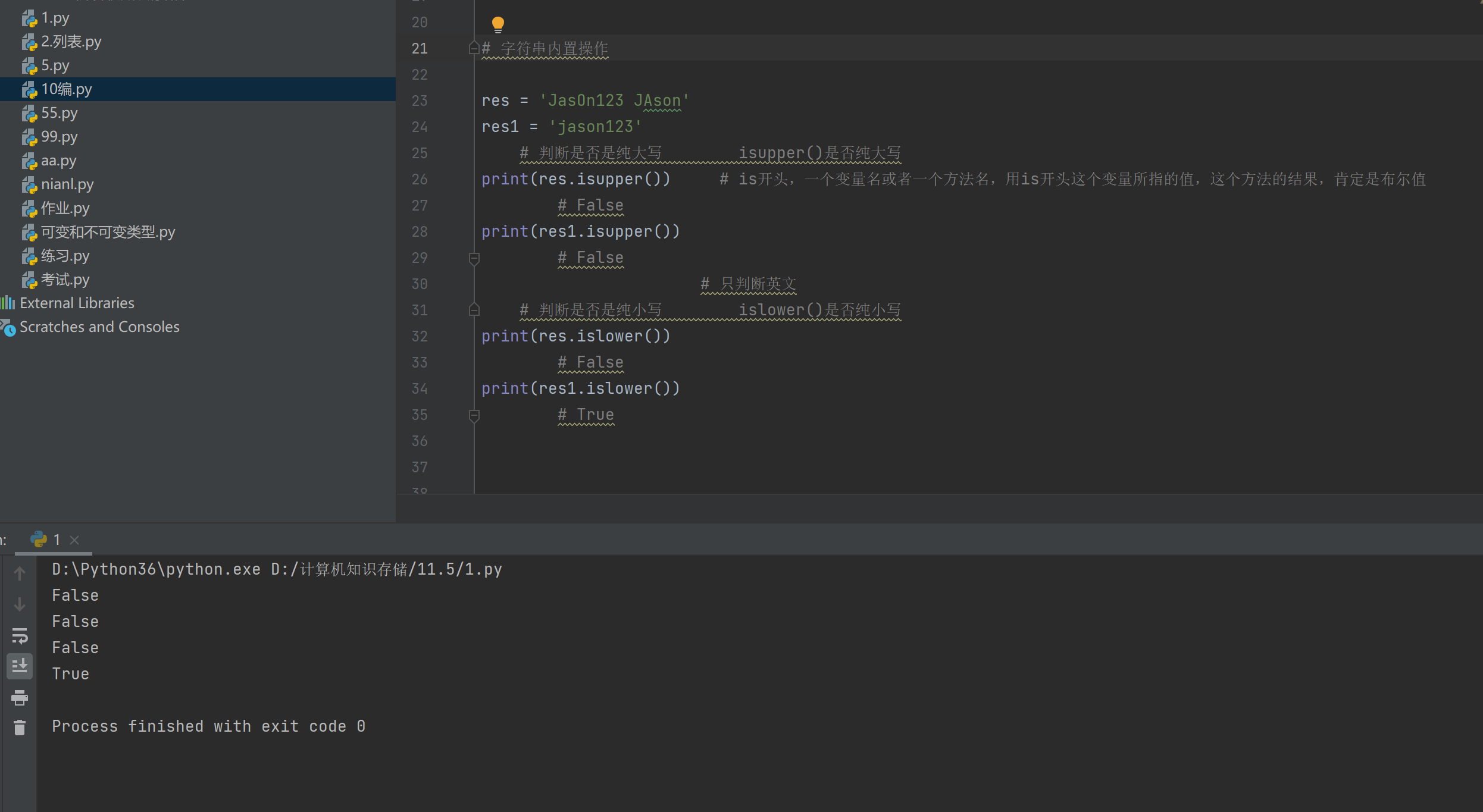
6.替换字符串中指定的字符 replace
s4 = 'my name is tony tony my age is 18'
替换字符串中所有的tony >变 jason
print(s4.replace('tony', 'jason')) # my name is jason jason my age is 18
替换指定个数的文本
print(s4.replace('tony', 'jason', 1)) # 比如说就想替换一个,写个1
my name is jason tony my age is 18
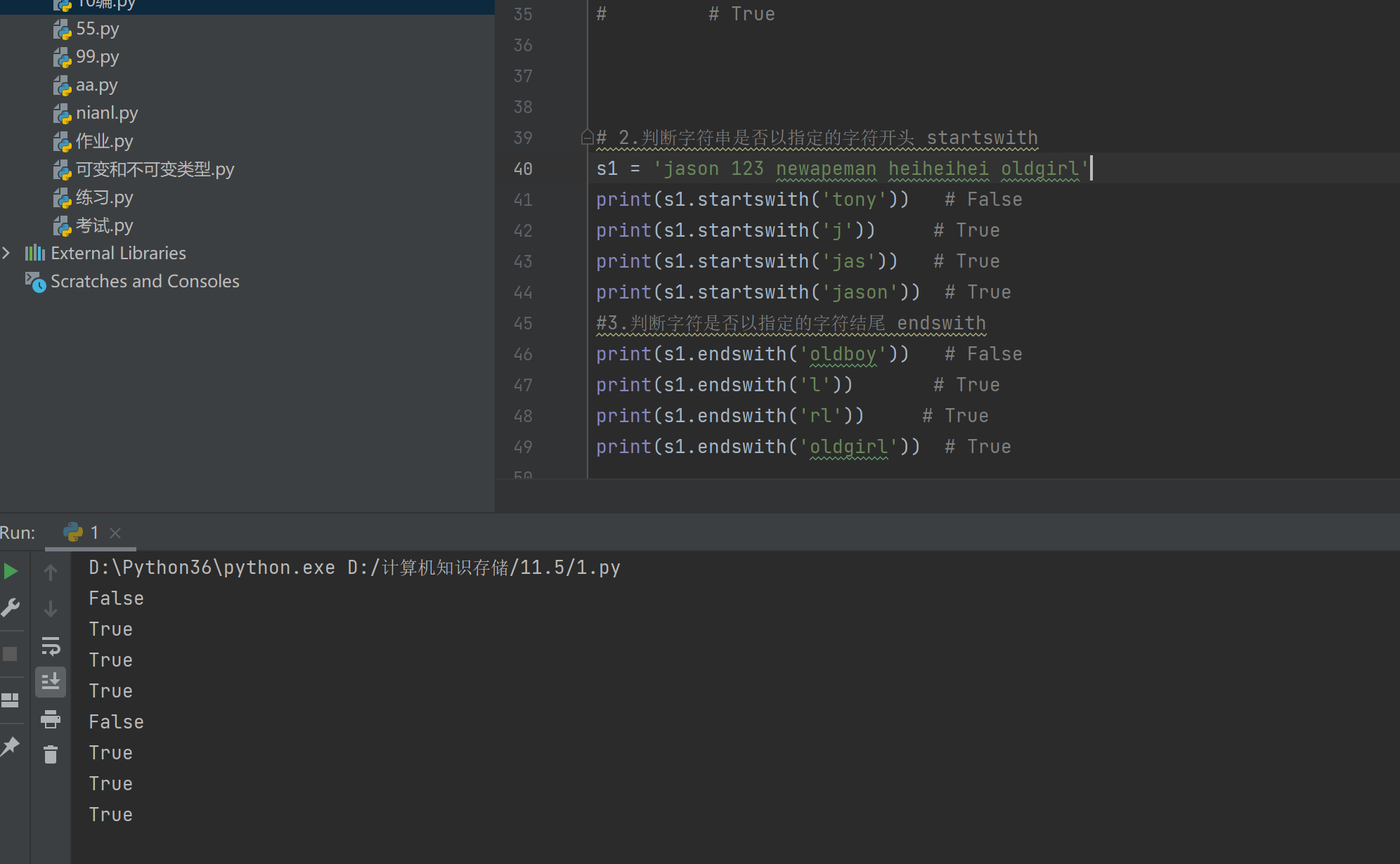
4.格式化输出
4.1 与用户交互 占位符 %s %d # 图快使用推荐
s1 = 'my name is %s my age is %s'
print(s1 % ('tony', 18)) # my name is tony my age is 18
4.2 字符串内置方法 format() # 格式化输出建议使用format() # 重复使用推荐
第一种 相当于%s占位符
s2 = 'my name is {} my age is {}'
print(s2.format('jason', 18)) # my name is jason my age is 18
第二种 # 大括号内索引值可以打破顺序 并且可以反复使用相同位置的数据
s3 = 'my name is {0} my age is {1} {1} {0}' # {}这个里面的数字是 索引取值
print(s3.format('jason', 18)) # my name is jason my age is 18 18 jason
第三种 # 大括号内写变量名
s4 = '{name1} my name is {name1} my age is {age} {name1} {name1}'
print(s4.format(name1='jason', age=18)) # jason my name is jason my age is 18 jason jason
'占位符与format结合集体情况挑选使用'
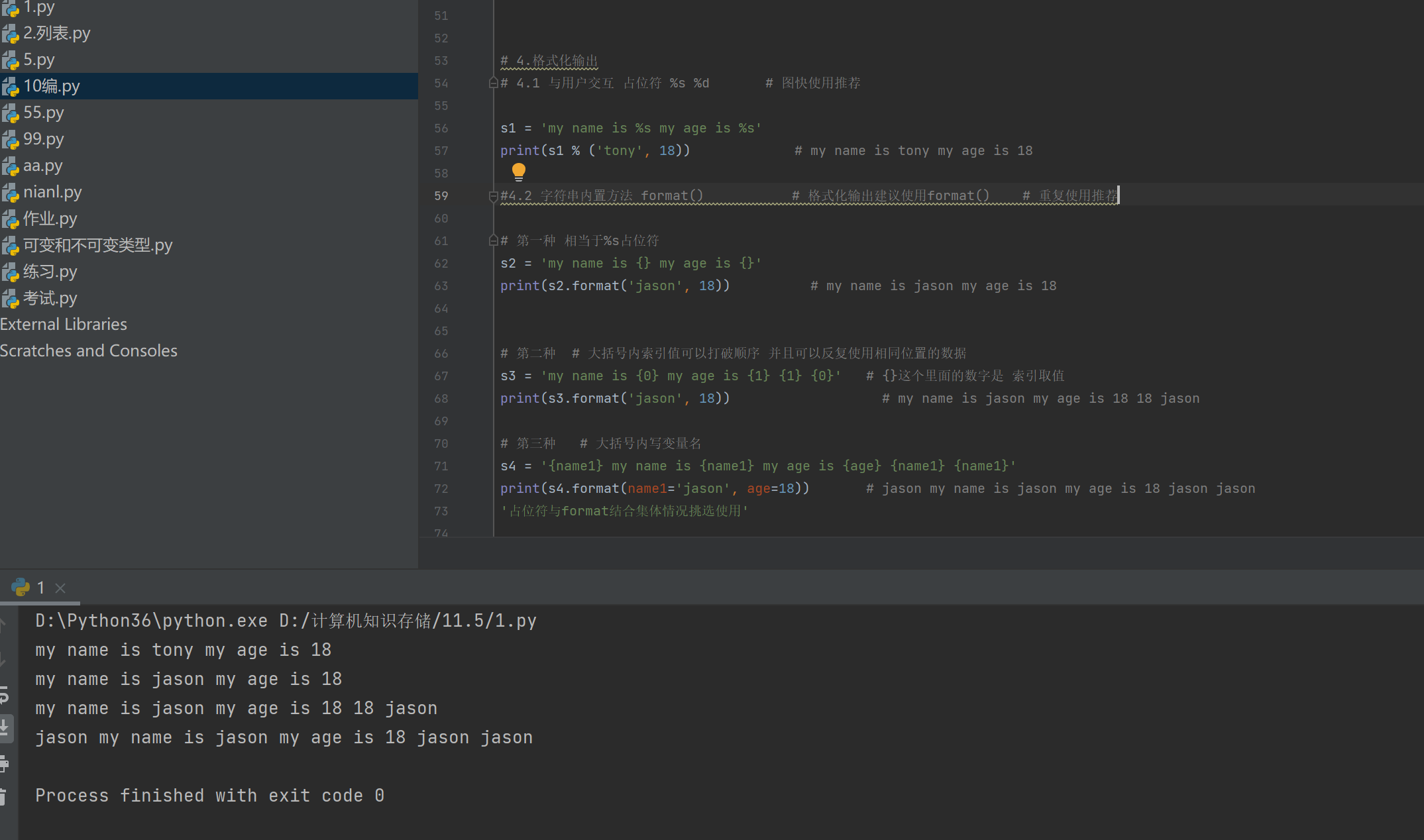
5.字符串的拼接
方式1 字符串相加
print('hello' + 'world') # helloworld
方式2 join方法 更方便
l = ['jason', 'tony', 'kevin', 'tom', 'jack']
print('|'.join(l)) # jason|tony|kevin|tom|jack
字符串类型拼接必须是字符串类型,相同的类型(在python不同数据类型之间无法直接操作)
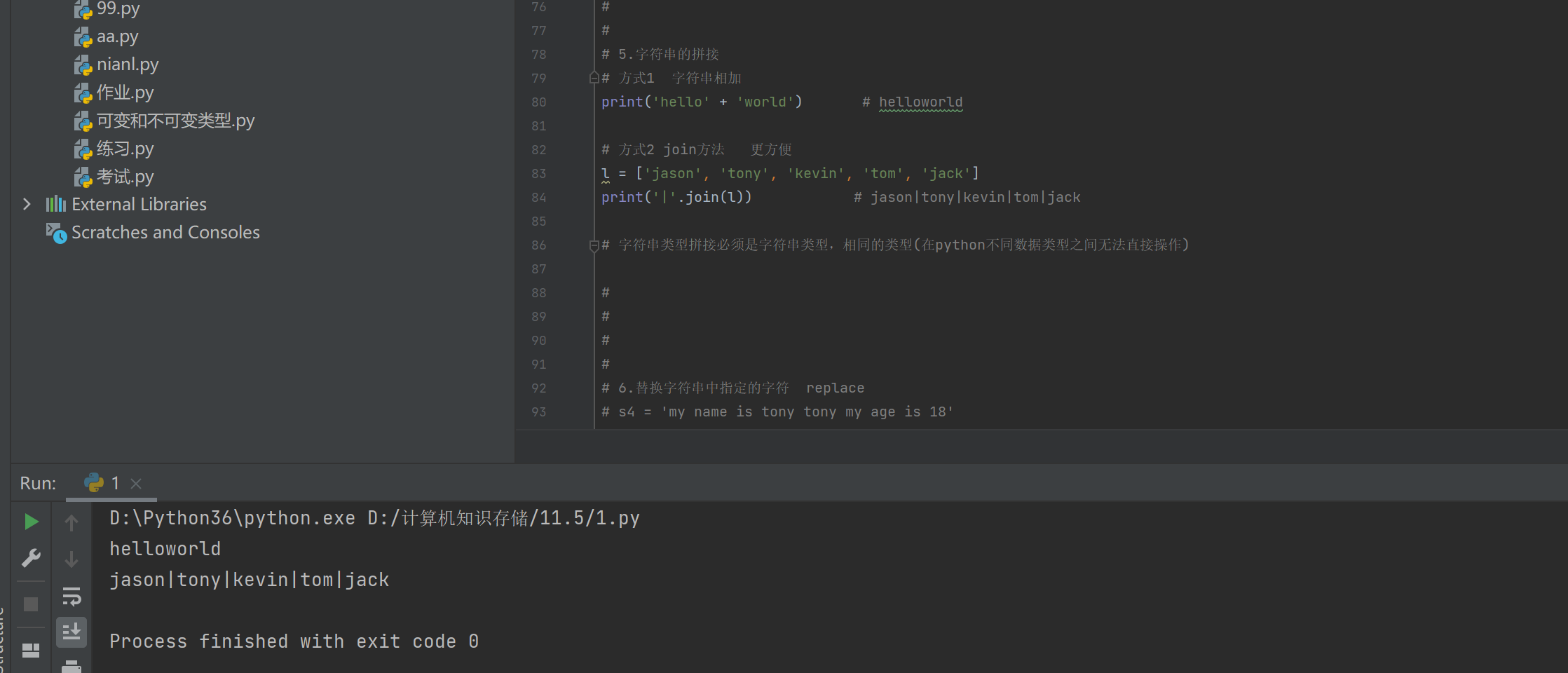
6.替换字符串中指定的字符 replace
s4 = 'my name is tony tony my age is 18'
替换字符串中所有的tony >变 jason
print(s4.replace('tony', 'jason')) # my name is jason jason my age is 18
替换指定个数的文本
print(s4.replace('tony', 'jason', 1)) # 比如说就想替换一个,写个1
# my name is jason tony my age is 18
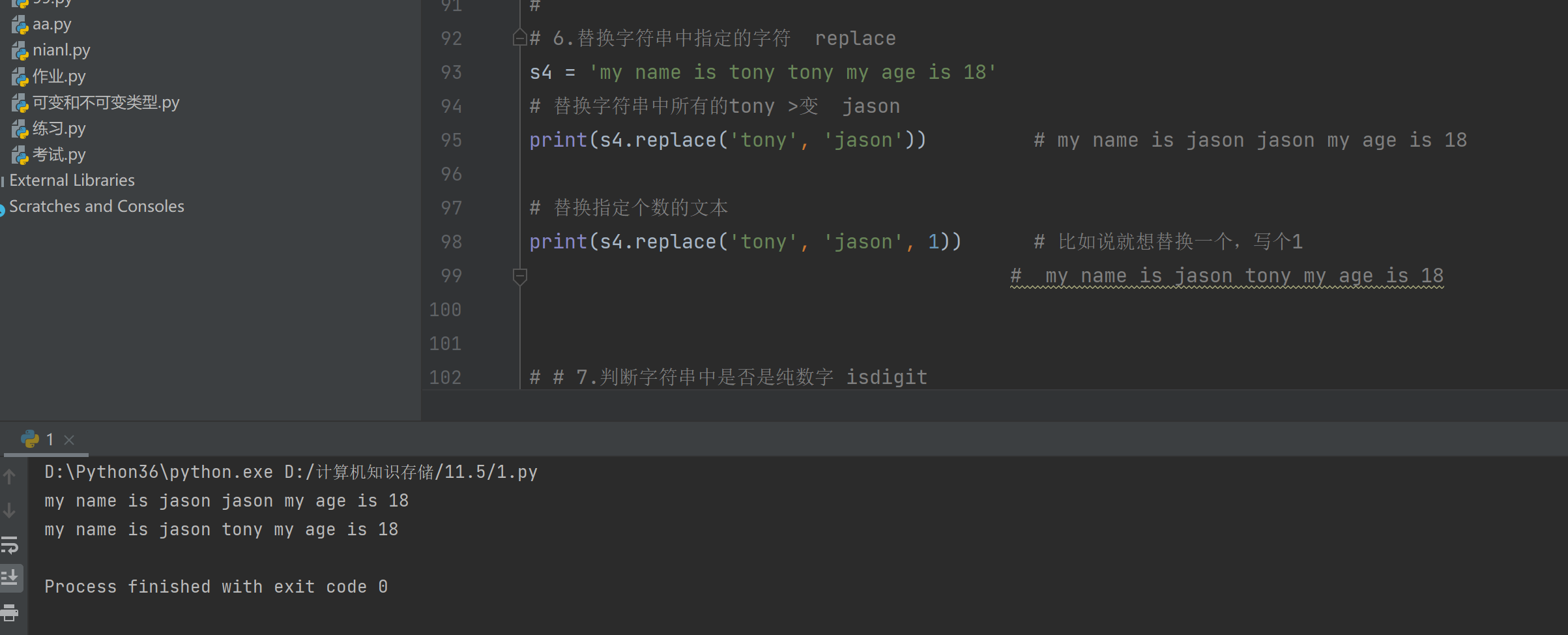
7.判断字符串中是否是纯数字 isdigit
s5 = 'jason123'
print(s5.isdigit()) # False
s6 = '123'
print(s6.isdigit()) # True
猜年龄,判断用户是否输入的是纯数字
guess_age = input('请输入您猜测的年龄:').strip()
if guess_age.isdigit(): # 判断用户输入的是否是数字
guess_age = int(guess_age)
else:
print('您能不能好好输')
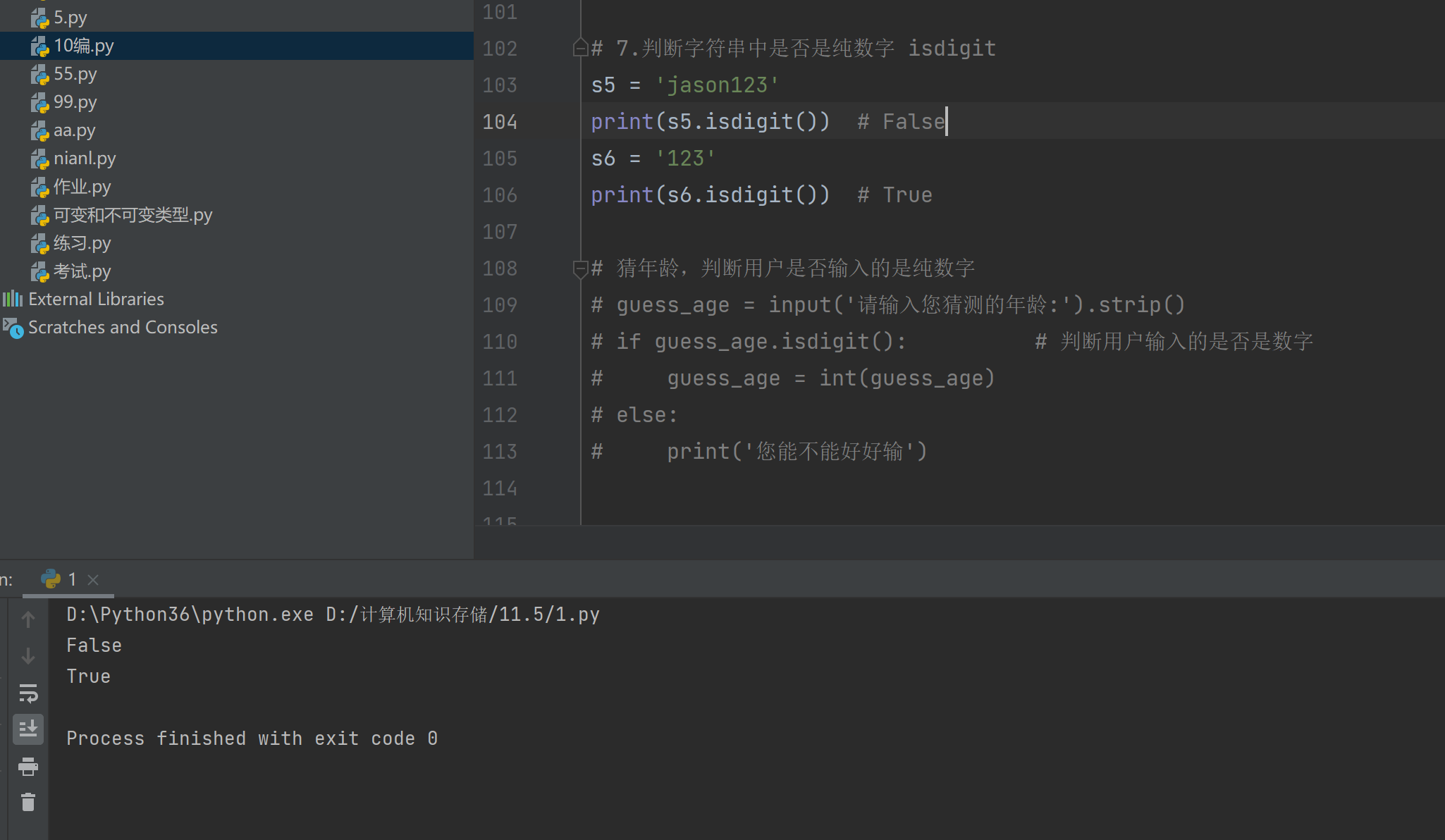
### # 了解
#### # 1.字体格式
s5 = 'my name is jason my age is 18' # My Name Is Jason My Age Is 18
print(s5.title()) # 所有单词首字母大写
print(s5.capitalize()) # My name is jason my age is 18
# 开头单词首字母大写
s6 = 'JaSOn iS Sb'
print(s6.swapcase()) # jAsoN Is sB 大小写互换
s7 = 'my name is jason sb sb sb somebody'
print(s7.find('s')) # 9 # 查找指定字符对应的索引值 从左往右找到一个就结束
print(s7.find('w')) # 找不到返回-1
print(s7.index('w')) # 找不到直接报错
* 统计某个字符出现的次数(记忆)
* print(s7.count('sb')) # 3
s8 = 'jason'
print(s8.center(15, '$')) # 居中展示,将左右两边添上你想要的符号 $$$$$jason$$$$$
print(s8.ljust(15, '&')) # 左对齐 jason&&&&&&&&&&
print(s8.rjust(15, '*')) # 右对齐 **********jason

* is其他
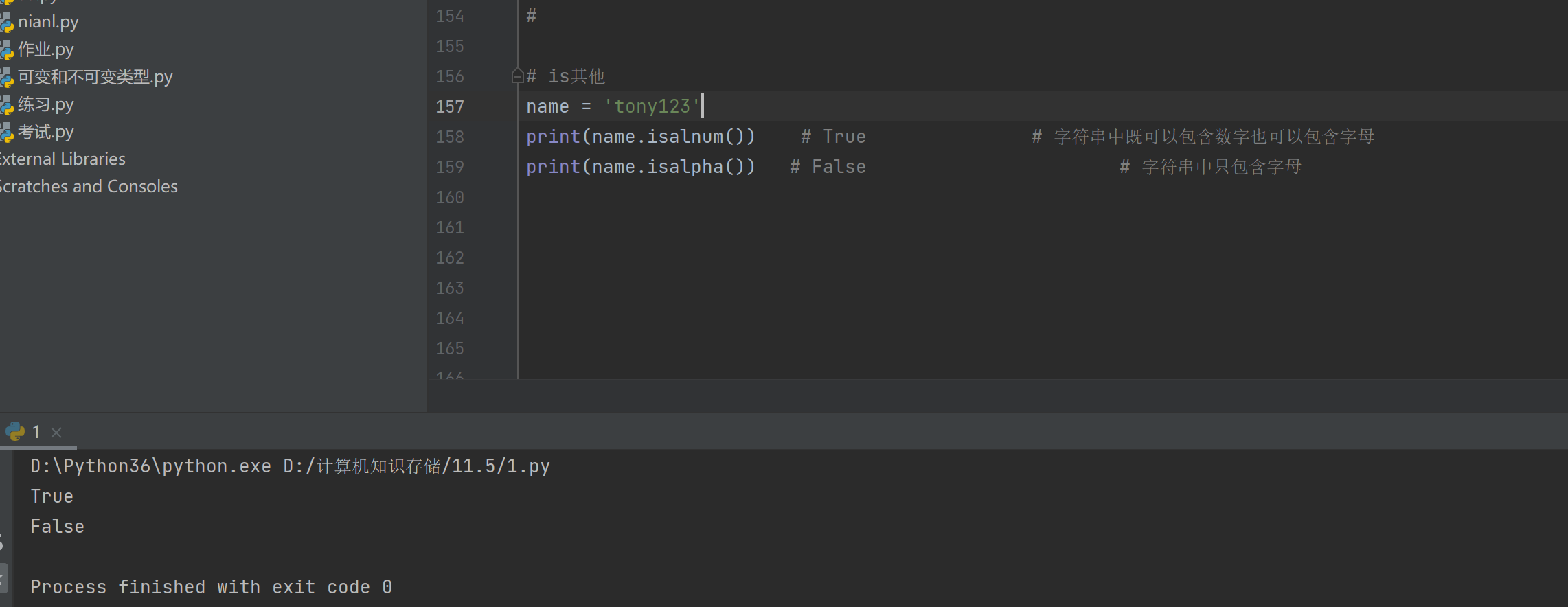
name_list = ['jason', 'kevin', 'tony', 'jack']
l1 = []
* 把列表name_list的值放入列表l1
for name in name_list:
l1.append(name)
* 队列: FIFO
for name1 in l1:
print(name1, end=' ')
print()
* 堆栈: FILO
print(l1[::-1])
for name2 in l1[::-1]:
print(name2, end=' ')
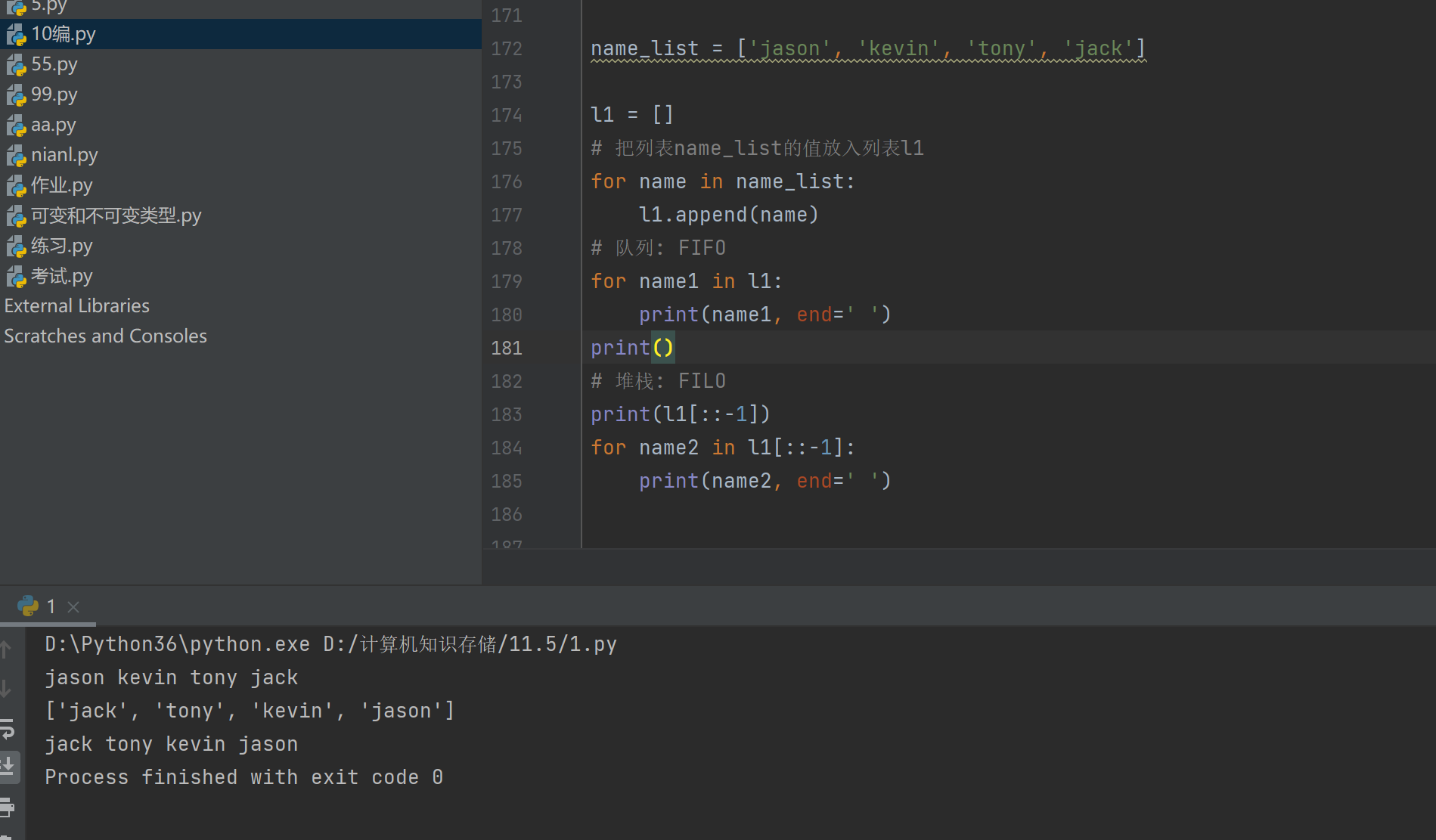
二:列表内置方法
* 列表内一般都会存储相同数据类型的数据
* 类型转换 数据类型关键字(需要转换的数据)
print(list(123)) # 报错
print(list(123.21) # 报错
print(list('hello')) # ['h', 'e', 'l', 'l', 'o']
print(list({'username': 'jason', 'pwd': 123})) # ['username', 'pwd']
print(list((11, 22, 33))) # [11, 22, 33]
print(list({11, 22, 33})) # [33, 11, 22]
* "list关键字可以将支持for循环的数据类型转换成列表"
* 列表的内置方法
name_list = ['jason', 'kevin', 'tony', 'jack']
1.索引取值
print(name_list[0]) # jason
* 2.切片操作 顾头不顾尾
print(name_list[1:3]) # ['kevin', 'tony']
* 2.1减负操作
print(name_list[-1:-4:-1]) # ['jack', 'tony', 'kevin']
print(name_list[-4:-1]) # ['jason', 'kevin', 'tony']
* 3.间隔个数(步长) 自定义取值 跳一个拿一个
print(name_list[0:4:2]) # ['jason', 'tony']
* 4.统计列表中元素的个数 len
print(len(name_list)) # 4
1.修改值
name_list = ['jason', 'kevin', 'tony', 'jack']
1.修改值 append()
name_list[0] = 666
print(name_list) # [666, 'kevin', 'tony', 'jack']
2.添加值 append
方式1 尾部添加
name_list.append(666)
print(name_list) # ['jason', 'kevin', 'tony', 'jack', 666]
name_list.append([666, 777, 888, 999])
print(name_list) # ['jason', 'kevin', 'tony', 'jack', 666, [666, 777, 888, 999]]
方式2 插入元素 insert() (将括号内的数据当成一个整体插入到索引指定位置)
name_list.insert(0, 'heiheihei')
print(name_list) # ['heiheihei', 'jason', 'kevin', 'tony', 'jack']
name_list.insert(2, 'heiheihei')
print(name_list) # ['heiheihei', 'jason', 'heiheihei', 'kevin', 'tony', 'jack']
name_list.insert(0, [11, 22, 33])
print(name_list) # [[11, 22, 33], 'heiheihei', 'jason', 'heiheihei', 'kevin', 'tony', 'jack']
### 三:扩展元素
方式3 扩展元素 (相当于for循环+append操作)
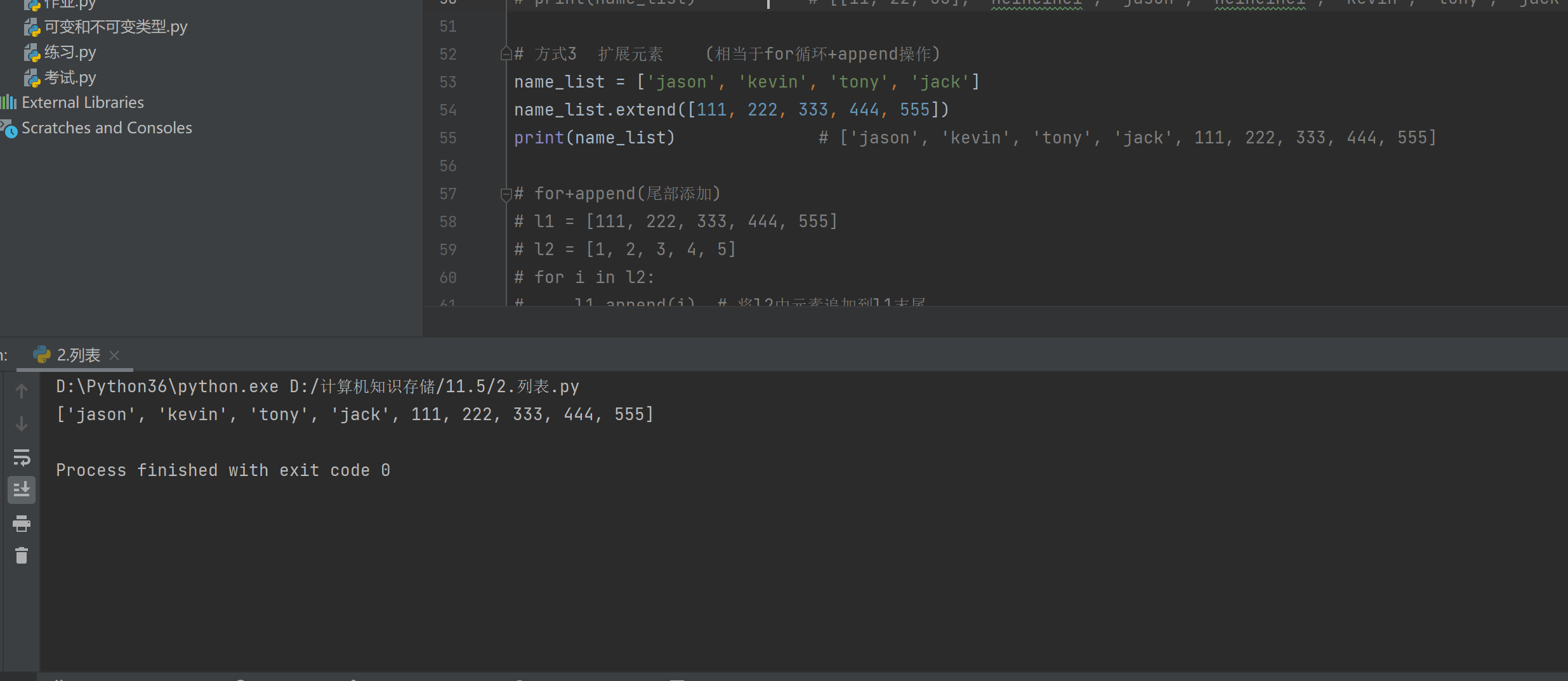
for+append(尾部添加)
l1 = [111, 222, 333, 444, 555]
l2 = [1, 2, 3, 4, 5]
for i in l2:
l1.append(i) # 将l2中元素追加到l1末尾
print(l1) # [111, 222, 333, 444, 555, 1, 2, 3, 4, 5]
* 针对数据的操作就是:增删改查
* 改和增
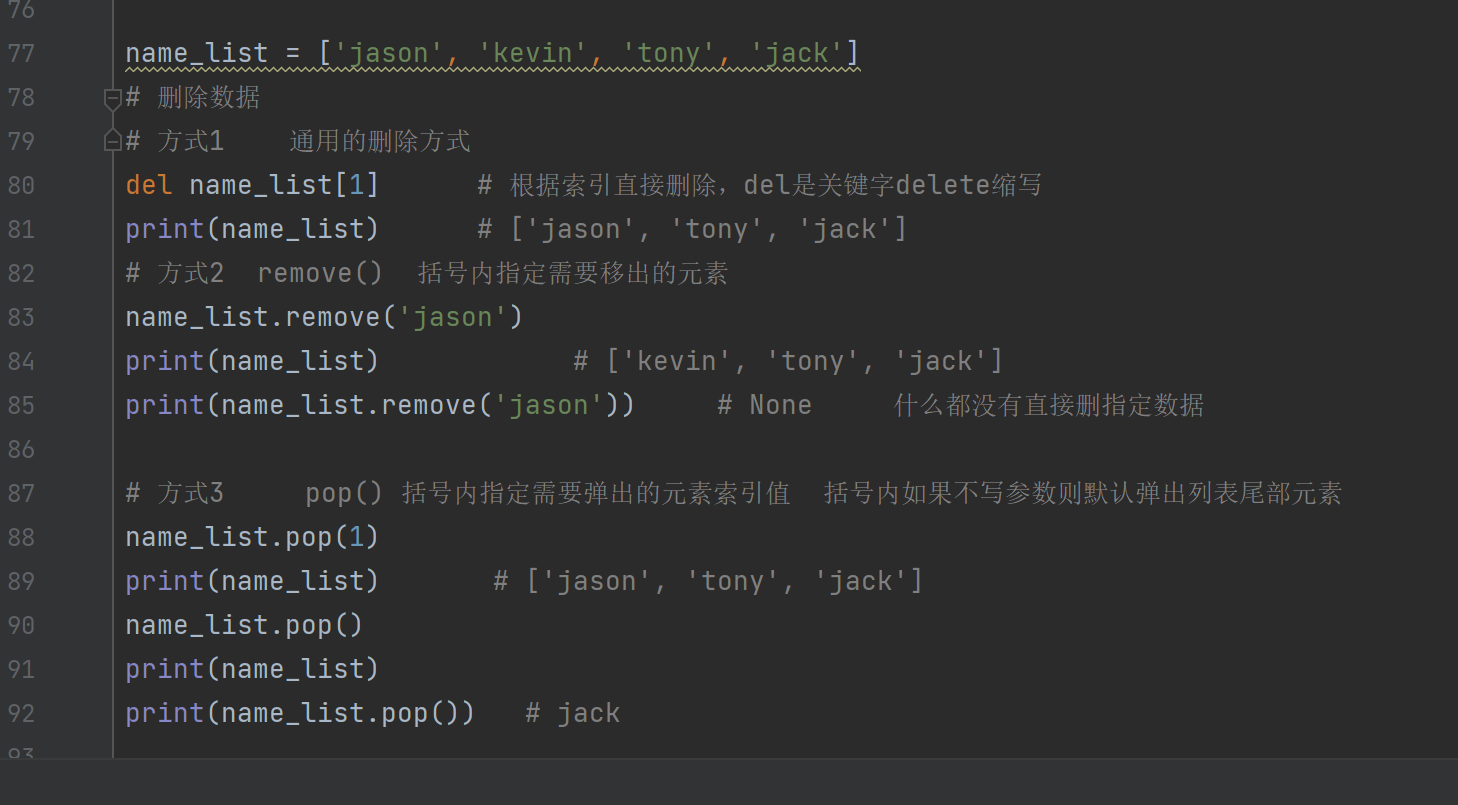
四:可变类型与不可变类型
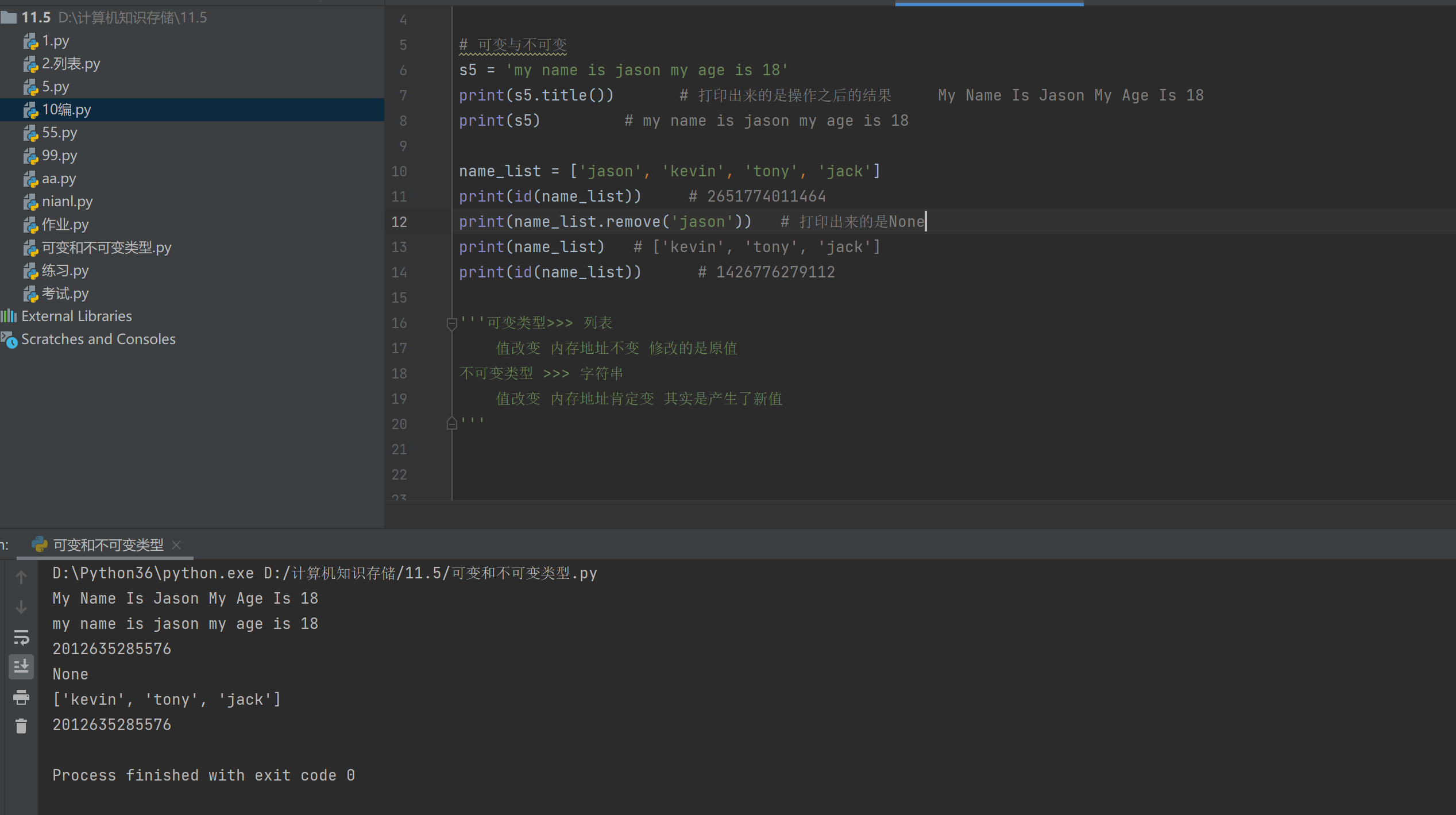