SQLite DBHelper 单例模式实现
[mw_shl_code=java,true]package
com.tre.android.app.ui.trial.database;
import
java.util.ArrayList;
import java.util.List;
import
com.tre.android.framework.Configuration;
import
android.content.ContentValues;
import android.content.Context;
import
android.database.Cursor;
import
android.database.sqlite.SQLiteDatabase;
import
android.database.sqlite.SQLiteException;
import
android.database.sqlite.SQLiteOpenHelper;
import
android.util.Log;
/**
* DB 帮助类 继承 SQLiteOpenHelper
*
* @author
yangkaikai
*
*/
public class DBHelper extends SQLiteOpenHelper
{
public static DBHelper mInstance = null;
/** DB对象
**/
SQLiteDatabase mDb = null;
static Context mContext
= null;
public static final String DB_NAME =
"PacerPlayer.db";
public static final int DB_VERSION = 1;
public static final String TB_SERVER = "serverinfo";
public static
final String TB_LOGIN = "logininfo";
public static final String
TB_RECARD = "recardinfo";
public static final String TB_OFFLINEDATA =
"offlinedata";
public static final String TB_APPRECORD =
"applicationList";
public static final String MODULE_TYPE_LOCAL =
"0";
// 清空 logininfo 表
public static final String
DROP_TABLE_LOGIN = "DROP TABLE IF EXISTS "
+
TB_LOGIN;
// 清空 serverinfo 表
public static final String
DROP_TABLE_SERVER = "DROP TABLE IF EXISTS "
+
TB_SERVER;
public static final String DROP_TABLE_APPRECORD =
"DROP TABLE IF EXISTS "
+ TB_APPRECORD;
public static final String CREATE_TABLE_SERVER = "CREATE TABLE IF NOT EXISTS
"
+ TB_SERVER
+ "("
+ ServerModel.storeIP
+ "
VARCHAR ,"
+ ServerModel.serverIP
+ " VARCHAR , "
+ ServerModel.storeCD
+ " VARCHAR , "
+
ServerModel.storeName
+ " VARCHAR , "
+ ServerModel.sipIP + " VARCHAR )";
public static final
String CREATE_TABLE_LOGIN = "CREATE TABLE IF NOT EXISTS "
+ TB_LOGIN
+ "("
+
LoginModel.storeIP
+ " VARCHAR NOT NULL,"
+ LoginModel.empCD
+ " VARCHAR ,
"
+ LoginModel.empName
+ "
VARCHAR )";
public static final String CREATE_TABLE_RECARD = "CREATE
TABLE IF NOT EXISTS "
+ TB_RECARD
+ " ( "
+ LoginModel.storeIP
+ " VARCHAR NOT NULL ,"
+
LoginModel.empCD
+ " VARCHAR , "
+ LoginModel.empName
+ " VARCHAR , "
+ LoginModel.pwd
+ " VARCHAR ,
"
+ LoginModel.time
+ "
default(datetime('now','localtime')))";
public static final String
CREATE_TABLE_OFFLINEDATA = "Create TABLE IF NOT EXISTS "
+ TB_OFFLINEDATA
+ "("
+ OffLineMode.RowNo
+ " INTEGER PRIMARY KEY
AUTOINCREMENT , "
+ OffLineMode.SysName
+ " text ,"
+ OffLineMode.FunName
+ " text , "
+
OffLineMode._URL
+ " text , "
+ OffLineMode.CreateTime
+ "
DEFAULT(datetime('now','localtime')) , "
+
OffLineMode.State
+ " int DEFAULT 0 )";
public static final String CREATE_TABLE_APPRECORD = "CREATE TABLE IF NOT
EXISTS "
+ TB_APPRECORD
+
"("
+ ApplicationRecordModel.systemID
+ " INTEGER ,"
+
ApplicationRecordModel.systemName
+ " VARCHAR ,
"
+ ApplicationRecordModel.systemType
+ " VARCHAR , "
+
ApplicationRecordModel.systemURL
+ " VARCHAR , "
+ ApplicationRecordModel.systemRecordCount + ")";
public DBHelper(Context context) throws SQLiteException
{
super(context, DB_NAME, null, DB_VERSION);
// 得到数据库对象
try {
mDb =
getWritableDatabase();
} catch (Exception e) {
mDb=getReadableDatabase();
}
mContext = context;
}
/**
* 单例模式
*/
public static synchronized DBHelper getInstance(Context
context) {
if (mInstance == null) {
mInstance = new DBHelper(context);
}
return mInstance;
}
@Override
public
void onCreate(SQLiteDatabase db) {
db.execSQL(CREATE_TABLE_SERVER);
db.execSQL(CREATE_TABLE_LOGIN);
db.execSQL(CREATE_TABLE_RECARD);
db.execSQL(CREATE_TABLE_OFFLINEDATA);
db.execSQL(CREATE_TABLE_APPRECORD);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion)
{
db.execSQL(DROP_TABLE_LOGIN);
db.execSQL(DROP_TABLE_SERVER);
db.execSQL(DROP_TABLE_APPRECORD);
}
/*
*
public void insert(String tablename, String key[], String date[]) {
* ContentValues values = new ContentValues(); for (int i = 0; i <
* key.length; i++) { values.put(key, date); } mDb.insert(tablename,
* null, values); }
*/
/**
* 插入一条数据
*
* @return
*/
public long insert(
String table, ContentValues values) {
return mDb.insert(table, null,
values);
}
/**
* 查询
*
* @return
*/
public Cursor query(String table,
String[] columns, String selection,
String[]
selectionArgs, String orderBy) {
Log.i("************", "this
is query");
return mDb.query(table,
columns, selection, selectionArgs, null, null,
orderBy);
}
/**
* 删除
*
* @return
*/
public long delete(String
table, String whereClause, String[] whereArgs) {
return mDb.delete(table, whereClause, whereArgs);
}
/**
* 更新某条数据
*
* @return
*/
public long update(String table, ContentValues values, String
whereClause,
String[] whereArgs) {
return mDb.update(table, values, whereClause, whereArgs);
}
/**
* 删除所有数据
*
*
@return
*/
public long deleteAllData(String tablename)
{
return mDb.delete(tablename, null,
null);
}
}[/mw_shl_code]
2.调用方法:
dbHelper =
DBHelper.getInstance(this);// 实例化数据库对象
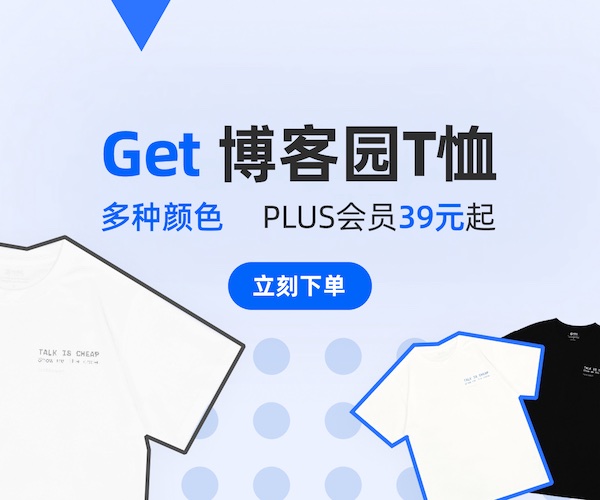