互斥锁
互斥锁总结:将并行变成串行,虽然牺牲了程序的执行效率倒是保证了数据的安全!
常见的一些锁:
行锁,表锁,乐观锁,悲观锁
线程
理论
# 进程其实是资源单位,负责提供线程执行过程中所需的各项资源
# 线程其实是执行单位 真正在进程中干活的人(借助于解释器与CPU交互)
1.一个进程至少含有一个线程
2.多进程与线程的区别:
多进程 : 需要申请内存空间!需要拷贝全部代码,资源消耗大
多线程: 不需要申请内存空间 也不需要拷贝全部代码 资源消耗较小!
3.同一个进程下多个线程之间资源共享
4.开设线程的资源消耗远远小于开设进程
创建线程的两种线程
1.函数创建
from threading import Thread
import time
def Mythred(name):
print('%s正在运行' % name)
time.sleep(3)
print('%s 结束运行' % name)
t = Thread(target=Mythred, args=('summer',))
t.start()
print('主线程')
------------
2.类继承
class MyThread(Thread):
def __init__(self, name):
super().__init__()
self.name = name
def run(self):
print(f'{self.name}正在运行')
time.sleep(4)
print(f'{self.name}结束运行')
t = MyThread('summer')
t.start()
print('主线程')
线程join方法
主线程等到子线程运行结束之后再运行
def task():
def task():
from threading import Thread
import time
class MyThread(Thread):
def __init__(self, name):
super().__init__()
self.name = name
def run(self):
print(f'{self.name}正在运行')
time.sleep(4)
print(f'{self.name}结束运行')
t = MyThread('summer')
t.start()
t.join()
print('主线程')
同一个进程下线程数据共享
from threading import Thread
money = 1000
def res():
global money
money = 999
print(money)
t = Thread(target=res)
t.start()
t.join()
print(money)
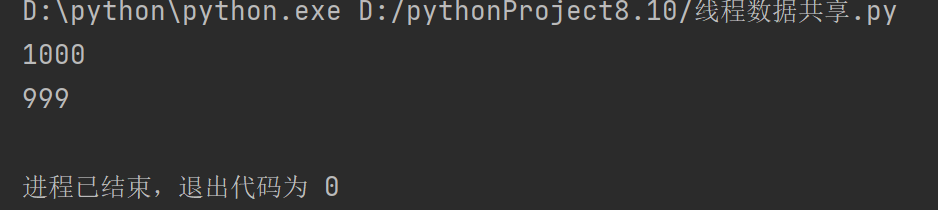
线程对象数据和方法
1.进程号
同一个进程下开设的多个线程拥有相同的进程号
2.线程名
from threading import Thread, current_thread
current_thread().name
主:MainThread 子:Thread-N
from threading import Thread,current_thread
import time
import os
print(current_thread().name) # MainThread主线程
def task():
print('子线程')
time.sleep(5)
print('子线程结束')
print('子线程',os.getpid()) # 子线程 238608
print('子线程名',current_thread().name) # 子线程名 Thread-1
t = Thread(target=task)
print('主线程',os.getpid()) # 主线程 238608
t.start()
3.进程下的线程数
active_count()
t = Thread(target=task)
print('主线程',os.getpid()) # 主线程 238608
t.start()
print('当前进程下活跃的线程数量',active_count()) # 当前进程下活跃的线程数量 2
守护线程
# 主线程需要等待所有的非守护线程结束才能结束
from threading import Thread
import time
def res():
print('子线程')
time.sleep(3)
print('子线程结束')
t = Thread(target=res)
t.daemon = True
t.start()
print('主线程')
GIL全局解释器锁
# 官方文档对GIL的解释
In CPython, the global interpreter lock, or GIL, is a mutex that prevents multiple native threads from executing Python bytecodes at once. This lock is necessary mainly because CPython’s memory management is not thread-safe. (However, since the GIL exists, other features have grown to depend on the guarantees that it enforces.)
1.GIL是Cpython解释器的特点,不是python语言的
2.GIL的存在是为了防止Cpython解释器垃圾回收机制造成数据不安全
3.GIL的本质其实是一把互斥锁,只不过它用于维护解释器级别的数据安全,针对不同 的数据应该加不同的锁!!!
用户自定义的互斥锁是保护业务数据的安全
4.python同一个进程下的多个线程无法利用多核优势