【LeetCode】Maximum Binary Tree 最大值二叉树
Maximum Binary Tree 最大值二叉树
题意
- The root is the maximum number in the array.
- The left subtree is the maximum tree constructed from left part subarray divided by the maximum number.
- The right subtree is the maximum tree constructed from right part subarray divided by the maximum number.
解法
dfs
class Solution(object):
def constructMaximumBinaryTree(self, nums):
"""
:type nums: List[int]
:rtype: TreeNode
"""
if not nums:
return None
root = TreeNode(max(nums))
idx = nums.index(root.val)
root.left = self.constructMaximumBinaryTree(nums[:idx])
root.right = self.constructMaximumBinaryTree(nums[idx+1:])
return root
class Solution(object):
def constructMaximumBinaryTree(self, nums):
"""
:type nums: List[int]
:rtype: TreeNode
"""
if not nums:
return None
node_stack = []
for num in nums:
node = TreeNode(num)
# 判断当前值是否比之前的值大,大则放置在左边,小则放置在右边
while node_stack and node.val > node_stack[-1].val:
node.left = node_stack.pop()
if node_stack:
node_stack[-1].right = node
node_stack.append(node)
return node_stack[0] # 最后剩下的一定是最大的值,也就是跟节点
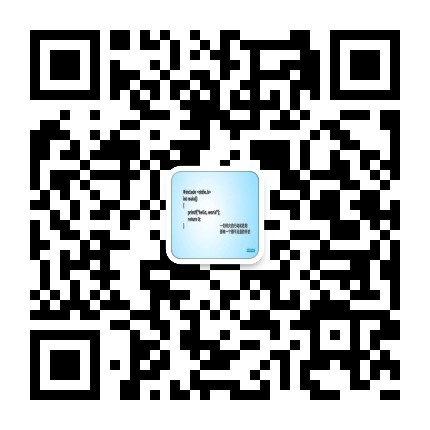
关注公众号:数据结构与算法那些事儿,每天一篇数据结构与算法