[LeetCode]54. Spiral Matrix
54. Spiral Matrix
1. 正常做法
class Solution(object):
def spiralOrder(self, matrix):
"""
:type matrix: List[List[int]]
:rtype: List[int]
"""
if not matrix:
return matrix
start_row, end_row = 0, len(matrix) - 1
start_col, end_col = 0, len(matrix[0]) - 1
ans = []
while start_row <= end_row and start_col <= end_col:
# 向右移动
for j in range(start_col, end_col+1):
ans.append(matrix[start_row][j])
start_row += 1
# 向下移动
for i in range(start_row, end_row+1):
ans.append(matrix[i][end_col])
end_col -= 1
# 向左移动
if start_row <= end_row:
for j in range(end_col, start_col-1, -1):
ans.append(matrix[end_row][j])
end_row -= 1
# 向上移动
if start_col <= end_col:
for i in range(end_row, start_row-1, -1):
ans.append(matrix[i][start_col])
start_col += 1
return ans
2. 数组弹出
def spiralOrder(self, matrix):
ret = []
while matrix:
# 弹出上面
ret += matrix.pop(0)
# 弹出右边
if matrix and matrix[0]:
for row in matrix:
ret.append(row.pop())
# 弹出下面
if matrix:
ret += matrix.pop()[::-1]
# 弹出左边
if matrix and matrix[0]:
for row in matrix[::-1]:
ret.append(row.pop(0))
return ret
3. 递归
class Solution(object):
def spiralOrder(self, matrix):
return matrix and list(matrix.pop(0)) + spiralOrder(list(zip(*matrix))[::-1])
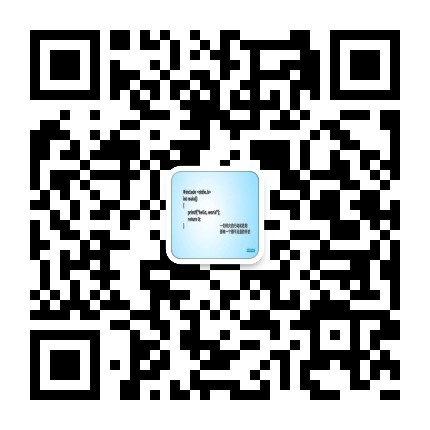
关注公众号:数据结构与算法那些事儿,每天一篇数据结构与算法