题目
类图
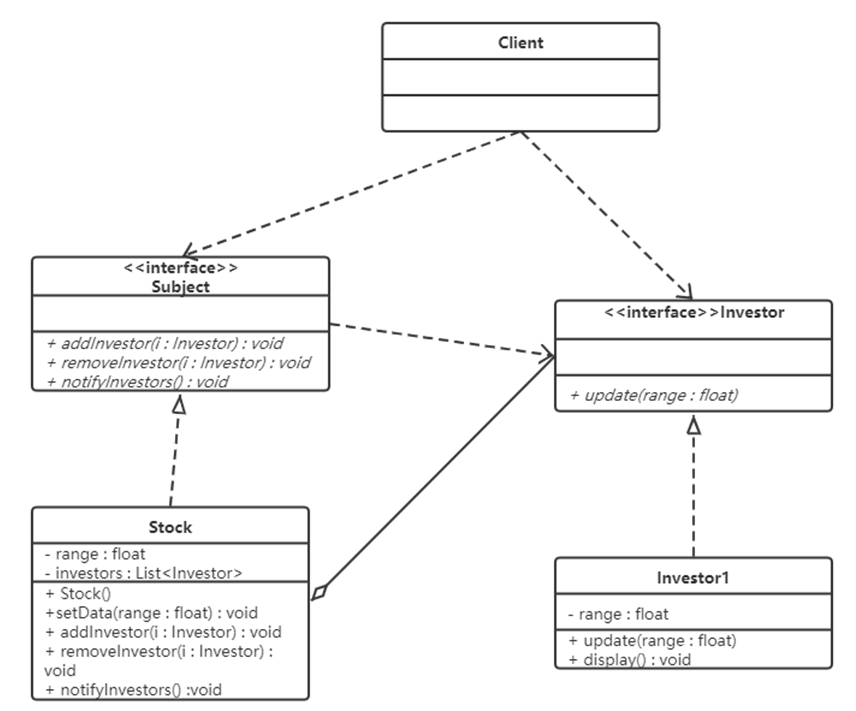
Java
Investor接口
package com.gazikel;
public interface Investor {
void update(float range);
}
Subject接口
package com.gazikel;
public interface Subject {
/**
* 添加一个股民
*/
void addInvestor(Investor investor);
/**
* 移除一个股民
*/
void removeInvestor(Investor investor);
/**
* 通知所有的股民
*/
void notifyInvestors();
}
Investor1实现Investor接口
package com.gazikel;
public class Investor1 implements Investor {
private float range;
@Override
public void update(float range) {
this.range = range;
display();
}
public void display() {
if (this.range > 0) {
// 上涨消息
System.out.println("买股票");
} else if(this.range < 0) {
// 下降消息
System.out.println("大哭一场");
}
}
}
Stock实现Subject接口
package com.gazikel;
public class Investor1 implements Investor {
private float range;
@Override
public void update(float range) {
this.range = range;
display();
}
public void display() {
if (this.range > 0) {
// 上涨消息
System.out.println("买股票");
} else if(this.range < 0) {
// 下降消息
System.out.println("大哭一场");
}
}
}
Client
package com.gazikel;
public class Client {
public static void main(String[] args) {
Investor investor1 = new Investor1();
Stock stock = new Stock();
// 添加股民
stock.addInvestor(investor1);
// 上涨不足5%,不会通知股民
stock.setData(0.03f);
System.out.println("------------------------");
// 上涨超过5%,通知股民
stock.setData(0.06f);
System.out.println("------------------------");
// 下降超过5%,通知股民
stock.setData(-0.07f);
System.out.println("------------------------");
}
}
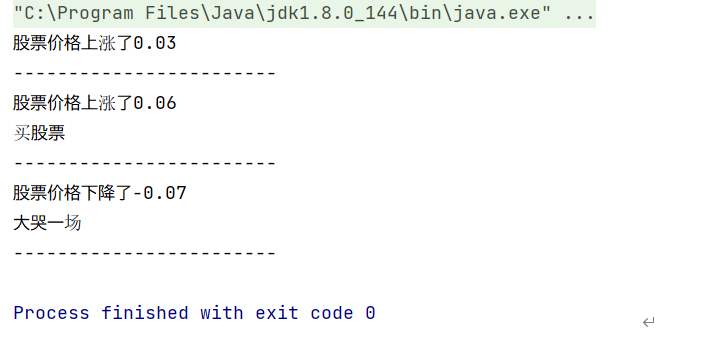
C++
#include<iostream>
#include<list>
using namespace std;
class Investor {
public:
virtual void update(double range) = 0;
};
class Investor1 :public Investor {
private:
double range;
public:
void update(double range) {
this->range = range;
display();
}
void display() {
if (this->range > 0) {
cout << "买股票" << endl;
}
else if (this->range < 0) {
cout << "大哭一场" << endl;
}
}
};
class Subject {
public:
virtual void AddInvestor(Investor* investor) = 0;
virtual void NotifyInvestors() = 0;
};
class Stock :public Subject {
private:
double range;
list<Investor*> investors;
public:
void SetData(double range) {
this->range = range;
if (range > 0) {
cout << "股票价格上涨了" << endl;
}
else if (this->range < 0) {
cout << "股票价格下降了" << endl;
}
else {
cout << "股票价格保持平稳" << endl;
}
if (this->range >= 0.05 || this->range <= -0.05) {
NotifyInvestors();
}
}
void AddInvestor(Investor *investor) {
investors.push_back(investor);
}
void NotifyInvestors() {
list<Investor*>::iterator it;
for (it = investors.begin(); it != investors.end(); it++) {
(*it)->update(this->range);
}
}
};
int main() {
Investor* investor1 = new Investor1();
Stock* stock = new Stock();
// 添加股民
stock->AddInvestor(investor1);
// 上涨不足5%,不会通知股民
stock->SetData(0.03);
cout << "-------------------------------" << endl;
// 上涨超过5%,通知股民
stock->SetData(0.06f);
cout << "-------------------------------" << endl;
// 下降超过5%,通知股民
stock->SetData(-0.07f);
cout << "-------------------------------" << endl;
}
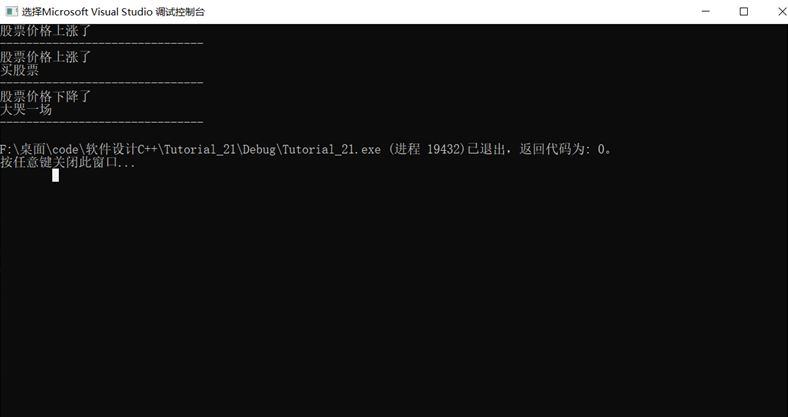