Qt使用cos、sin绘制圆
实例:
.pro

1 QT += core gui 2 3 greaterThan(QT_MAJOR_VERSION, 4): QT += widgets 4 5 CONFIG += c++17 6 7 # The following define makes your compiler emit warnings if you use 8 # any Qt feature that has been marked deprecated (the exact warnings 9 # depend on your compiler). Please consult the documentation of the 10 # deprecated API in order to know how to port your code away from it. 11 DEFINES += QT_DEPRECATED_WARNINGS 12 13 # You can also make your code fail to compile if it uses deprecated APIs. 14 # In order to do so, uncomment the following line. 15 # You can also select to disable deprecated APIs only up to a certain version of Qt. 16 #DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0 17 18 SOURCES += \ 19 main.cpp \ 20 mainwindow.cpp 21 22 HEADERS += \ 23 mainwindow.h 24 25 FORMS += \ 26 mainwindow.ui 27 28 # Default rules for deployment. 29 qnx: target.path = /tmp/$${TARGET}/bin 30 else: unix:!android: target.path = /opt/$${TARGET}/bin 31 !isEmpty(target.path): INSTALLS += target 32 33 RESOURCES += \ 34 resource.qrc
main.cpp

1 #include "mainwindow.h" 2 3 #include <QApplication> 4 5 int main(int argc, char *argv[]) 6 { 7 QApplication a(argc, argv); 8 MainWindow w; 9 w.show(); 10 return a.exec(); 11 }
mainwindow.h

1 #ifndef MAINWINDOW_H 2 #define MAINWINDOW_H 3 4 #include <QMainWindow> 5 #include <QPainter> 6 #include <QDebug> 7 #include <QtWidgets/QLabel> 8 #include <QtWidgets/QLineEdit> 9 #include <QtWidgets/QMainWindow> 10 #include <QtWidgets/QMenuBar> 11 #include <QtWidgets/QSlider> 12 #include <QtWidgets/QStatusBar> 13 #include <QtWidgets/QWidget> 14 #include <QtMath> 15 16 QT_BEGIN_NAMESPACE 17 namespace Ui { class MainWindow; } 18 QT_END_NAMESPACE 19 20 class MainWindow : public QMainWindow 21 { 22 Q_OBJECT 23 24 public: 25 MainWindow(QWidget *parent = nullptr); 26 ~MainWindow(); 27 void paintEvent(QPaintEvent *e); 28 private slots: 29 void on_horizontalSlider_valueChanged(int value); 30 31 void on_horizontalSlider_2_valueChanged(int value); 32 33 void on_pushButton_clicked(); 34 35 void on_pushButton_2_clicked(); 36 37 private: 38 39 double getAngle(double lat_a, double lng_a, double lat_b, double lng_b); //lat_a表示上个点纬度, lat_b表示当前点的纬度 40 41 Ui::MainWindow *ui; 42 int m_y = 0;// 43 int m_l = 100;// 44 int m_nSize = 200; 45 QPoint m_oCentre; 46 int m_nCentreWidth = 6;// 中心点宽度 47 }; 48 #endif // MAINWINDOW_H
mainwindow.cpp

1 #include "mainwindow.h" 2 #include "ui_mainwindow.h" 3 4 MainWindow::MainWindow(QWidget *parent) 5 : QMainWindow(parent) 6 , ui(new Ui::MainWindow) 7 { 8 ui->setupUi(this); 9 setWindowTitle(QStringLiteral("Qt使用cos、sin绘制圆")); 10 } 11 12 MainWindow::~MainWindow() 13 { 14 delete ui; 15 } 16 17 void MainWindow::paintEvent(QPaintEvent *e) 18 { 19 m_oCentre.setX((m_nSize/2)); 20 m_oCentre.setY((m_nSize/2)); 21 22 int nDeviation = 0; 23 // 生成绘制 24 QPainter oLinePainter(this); 25 oLinePainter.setWindow(nDeviation, nDeviation, this->width(), this->height()); 26 // 背景 27 QBrush objBrush(Qt::yellow, Qt::SolidPattern); 28 oLinePainter.fillRect(0, 0, m_nSize, m_nSize, objBrush); 29 // ---------------------画中心点-------------------------- 30 QPainter oCentrePainter(this); 31 oCentrePainter.setWindow(nDeviation, nDeviation, this->width(), this->height()); 32 QPen oCentrePen; 33 oCentrePen.setColor(Qt::red); 34 oCentrePen.setWidth(1); 35 oCentrePen.setStyle(Qt::SolidLine); 36 oCentrePainter.setPen(oCentrePen); 37 38 QBrush oCentreBrush(QColor(255,0,0), Qt::SolidPattern); 39 oCentrePainter.setBrush(oCentreBrush); 40 41 QPainterPath oCentreDrawPath; 42 43 oCentreDrawPath.addRect(m_oCentre.x(), 44 m_oCentre.y(), 45 m_nCentreWidth, 46 m_nCentreWidth); 47 // 48 oCentrePainter.drawPath(oCentreDrawPath); 49 // ---------------------画中心点-------------------------- 50 float x = 0; 51 float y = 0; 52 float fPI = 3.14159265358979323; 53 x = sin(m_y*fPI/180)*m_l + m_oCentre.x(); 54 y = -cos(m_y*fPI/180)*m_l + m_oCentre.x(); 55 56 57 QPainter oPainter(this); 58 oCentrePainter.setWindow(nDeviation, nDeviation, this->width(), this->height()); 59 QPen oPen; 60 oPen.setColor(Qt::blue); 61 oPen.setWidth(1); 62 oPen.setStyle(Qt::SolidLine); 63 oPainter.setPen(oPen); 64 65 QBrush oBrush(QColor(255,0,0), Qt::SolidPattern); 66 oPainter.setBrush(oBrush); 67 68 QPainterPath oDrawPath; 69 70 oDrawPath.addRect(x, 71 y, 72 m_nCentreWidth, 73 m_nCentreWidth); 74 // 75 oPainter.drawPath(oDrawPath); 76 } 77 78 void MainWindow::on_horizontalSlider_valueChanged(int value) 79 { 80 ui->label->setText(QString::number(value)); 81 m_y = value; 82 this->repaint(); 83 } 84 85 void MainWindow::on_horizontalSlider_2_valueChanged(int value) 86 { 87 ui->label_2->setText(QString::number(value)); 88 m_l = value; 89 this->repaint(); 90 } 91 92 void MainWindow::on_pushButton_clicked() 93 { 94 qreal long1 = ui->lineEdit->text().toDouble();//得到第一个点的经度 95 qreal lat1 = ui->lineEdit_2->text().toDouble();//得到第一个点的纬度 96 97 qreal long2 = ui->lineEdit_3->text().toDouble();//得到第二个点的经度 98 qreal lat2 = ui->lineEdit_4->text().toDouble();//得到第二个点的纬度 99 100 qreal a, b, R; 101 R = 6378137; // 地球半径 102 lat1 = lat1 * M_PI / 180.0; 103 lat2 = lat2 * M_PI / 180.0; 104 a = lat1 - lat2; 105 b = (long1 - long2) * M_PI / 180.0; 106 qreal sa2, sb2; 107 sa2 = sin(a / 2.0); 108 sb2 = sin(b / 2.0); 109 qreal distance //计算出距离 110 = 2 * R * asin(sqrt(sa2 * sa2 + cos(lat1) * cos(lat2) * sb2 * sb2)); 111 ui->lineEdit_5->setText(QString::number(distance,'f',3)); //同步到控件上并保留三位小数 112 } 113 114 void MainWindow::on_pushButton_2_clicked() 115 { 116 ui->lineEdit_6->setText(QString::number( 117 getAngle( 118 ui->lineEdit_2->text().toDouble(), 119 ui->lineEdit->text().toDouble(), 120 ui->lineEdit_4->text().toDouble(), 121 ui->lineEdit_3->text().toDouble() 122 ) 123 )); 124 125 } 126 127 double MainWindow::getAngle(double lat_a, double lng_a, double lat_b, double lng_b) 128 { 129 #define PI 3.14 130 131 double lat1 = lat_a* PI / 180.0; 132 double lat2 = lat_b* PI / 180.0; 133 double lon1 = lng_a* PI / 180.0; 134 double lon2 = lng_b* PI / 180.0; 135 double azimuth = sin(lat1) * sin(lat2) + cos(lat1) 136 * cos(lat2) * cos(lon2 - lon1); 137 azimuth = sqrt(1 - azimuth * azimuth); 138 azimuth = cos(lat2) * sin(lon2 - lon1) / azimuth; 139 azimuth = asin(azimuth) * 180 / PI; 140 if (azimuth == NAN) { 141 if (lon1 < lon2) { 142 azimuth = 90.0; 143 return azimuth; 144 } else { 145 azimuth = 270.0; 146 return azimuth; 147 } 148 } 149 150 double x = lat_b - lat_a; 151 double y = lng_b - lng_a; 152 if(x > 0 && y > 0){ 153 return azimuth; 154 } 155 else if(x < 0 && y > 0){ 156 return 180 - azimuth; 157 } 158 else if(x < 0 && y < 0){ 159 return 180 - azimuth; 160 } 161 else if(x > 0 && y < 0){ 162 return 360 + azimuth; 163 } 164 else{ 165 return NAN; 166 } 167 }
mainwindow.ui

1 <?xml version="1.0" encoding="UTF-8"?> 2 <ui version="4.0"> 3 <class>MainWindow</class> 4 <widget class="QMainWindow" name="MainWindow"> 5 <property name="geometry"> 6 <rect> 7 <x>0</x> 8 <y>0</y> 9 <width>678</width> 10 <height>343</height> 11 </rect> 12 </property> 13 <property name="windowTitle"> 14 <string>MainWindow</string> 15 </property> 16 <widget class="QWidget" name="centralwidget"> 17 <widget class="QSlider" name="horizontalSlider"> 18 <property name="geometry"> 19 <rect> 20 <x>260</x> 21 <y>20</y> 22 <width>341</width> 23 <height>22</height> 24 </rect> 25 </property> 26 <property name="maximum"> 27 <number>360</number> 28 </property> 29 <property name="orientation"> 30 <enum>Qt::Horizontal</enum> 31 </property> 32 </widget> 33 <widget class="QLabel" name="label"> 34 <property name="geometry"> 35 <rect> 36 <x>620</x> 37 <y>20</y> 38 <width>54</width> 39 <height>12</height> 40 </rect> 41 </property> 42 <property name="text"> 43 <string>TextLabel</string> 44 </property> 45 </widget> 46 <widget class="QLabel" name="label_2"> 47 <property name="geometry"> 48 <rect> 49 <x>620</x> 50 <y>60</y> 51 <width>54</width> 52 <height>12</height> 53 </rect> 54 </property> 55 <property name="text"> 56 <string>TextLabel</string> 57 </property> 58 </widget> 59 <widget class="QSlider" name="horizontalSlider_2"> 60 <property name="geometry"> 61 <rect> 62 <x>260</x> 63 <y>60</y> 64 <width>341</width> 65 <height>22</height> 66 </rect> 67 </property> 68 <property name="maximum"> 69 <number>150</number> 70 </property> 71 <property name="value"> 72 <number>100</number> 73 </property> 74 <property name="orientation"> 75 <enum>Qt::Horizontal</enum> 76 </property> 77 </widget> 78 <widget class="QPushButton" name="pushButton"> 79 <property name="geometry"> 80 <rect> 81 <x>390</x> 82 <y>230</y> 83 <width>89</width> 84 <height>24</height> 85 </rect> 86 </property> 87 <property name="text"> 88 <string>两点距离</string> 89 </property> 90 </widget> 91 <widget class="QLineEdit" name="lineEdit"> 92 <property name="geometry"> 93 <rect> 94 <x>70</x> 95 <y>230</y> 96 <width>113</width> 97 <height>23</height> 98 </rect> 99 </property> 100 <property name="text"> 101 <string>116.313468</string> 102 </property> 103 </widget> 104 <widget class="QLineEdit" name="lineEdit_2"> 105 <property name="geometry"> 106 <rect> 107 <x>200</x> 108 <y>230</y> 109 <width>113</width> 110 <height>23</height> 111 </rect> 112 </property> 113 <property name="text"> 114 <string>40.074599</string> 115 </property> 116 </widget> 117 <widget class="QLineEdit" name="lineEdit_3"> 118 <property name="geometry"> 119 <rect> 120 <x>70</x> 121 <y>280</y> 122 <width>113</width> 123 <height>23</height> 124 </rect> 125 </property> 126 <property name="text"> 127 <string>116.224182</string> 128 </property> 129 </widget> 130 <widget class="QLineEdit" name="lineEdit_4"> 131 <property name="geometry"> 132 <rect> 133 <x>200</x> 134 <y>280</y> 135 <width>113</width> 136 <height>23</height> 137 </rect> 138 </property> 139 <property name="text"> 140 <string>37.475040</string> 141 </property> 142 </widget> 143 <widget class="QLineEdit" name="lineEdit_5"> 144 <property name="geometry"> 145 <rect> 146 <x>500</x> 147 <y>230</y> 148 <width>113</width> 149 <height>23</height> 150 </rect> 151 </property> 152 </widget> 153 <widget class="QPushButton" name="pushButton_2"> 154 <property name="geometry"> 155 <rect> 156 <x>390</x> 157 <y>280</y> 158 <width>89</width> 159 <height>24</height> 160 </rect> 161 </property> 162 <property name="text"> 163 <string>两点角度</string> 164 </property> 165 </widget> 166 <widget class="QLineEdit" name="lineEdit_6"> 167 <property name="geometry"> 168 <rect> 169 <x>500</x> 170 <y>280</y> 171 <width>113</width> 172 <height>23</height> 173 </rect> 174 </property> 175 </widget> 176 <widget class="QLabel" name="label_3"> 177 <property name="geometry"> 178 <rect> 179 <x>620</x> 180 <y>233</y> 181 <width>72</width> 182 <height>15</height> 183 </rect> 184 </property> 185 <property name="text"> 186 <string>米</string> 187 </property> 188 </widget> 189 </widget> 190 </widget> 191 <resources/> 192 <connections/> 193 </ui>
ps:代码中计算方位的代码可能有问题,以下是另一个算法(弧度 返回弧度 正北为零,这点和osgEarth不同,osgEarth是正东为0)。
来源:https://blog.csdn.net/yangdaocheng/article/details/43195037
double MainWindow::getAngle(double lat_a, double lng_a, double lat_b, double lng_b) { #define PI 3.14 double lat1 = lat_a* PI / 180.0; double lat2 = lat_b* PI / 180.0; double lon1 = lng_a* PI / 180.0; double lon2 = lng_b* PI / 180.0; double dLon = (lon2-lon1); double y = sin(dLon) * cos(lat2); double x = cos(lat1)*sin(lat2) - sin(lat1)*cos(lat2)*cos(dLon); double brng = atan2(y, x); return brng * 180 / PI; }
作者:疯狂Delphi
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利.
欢迎关注我,一起进步!扫描下方二维码即可加我
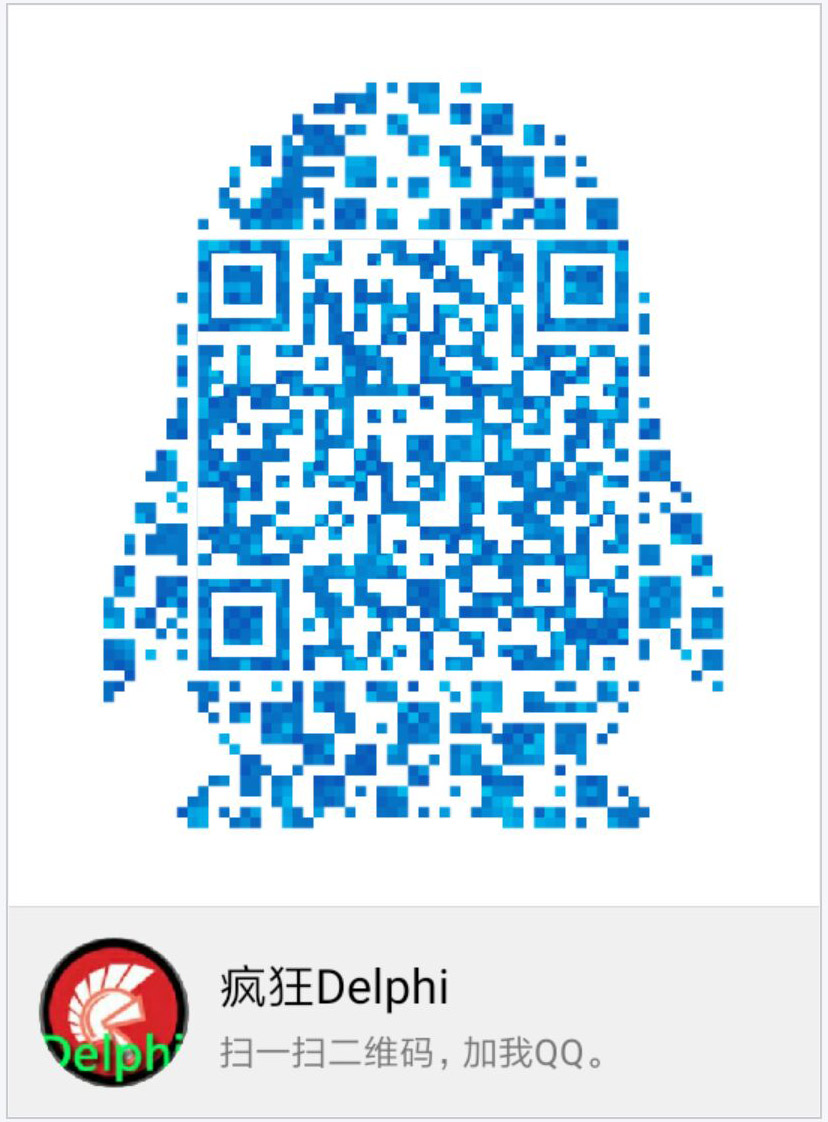
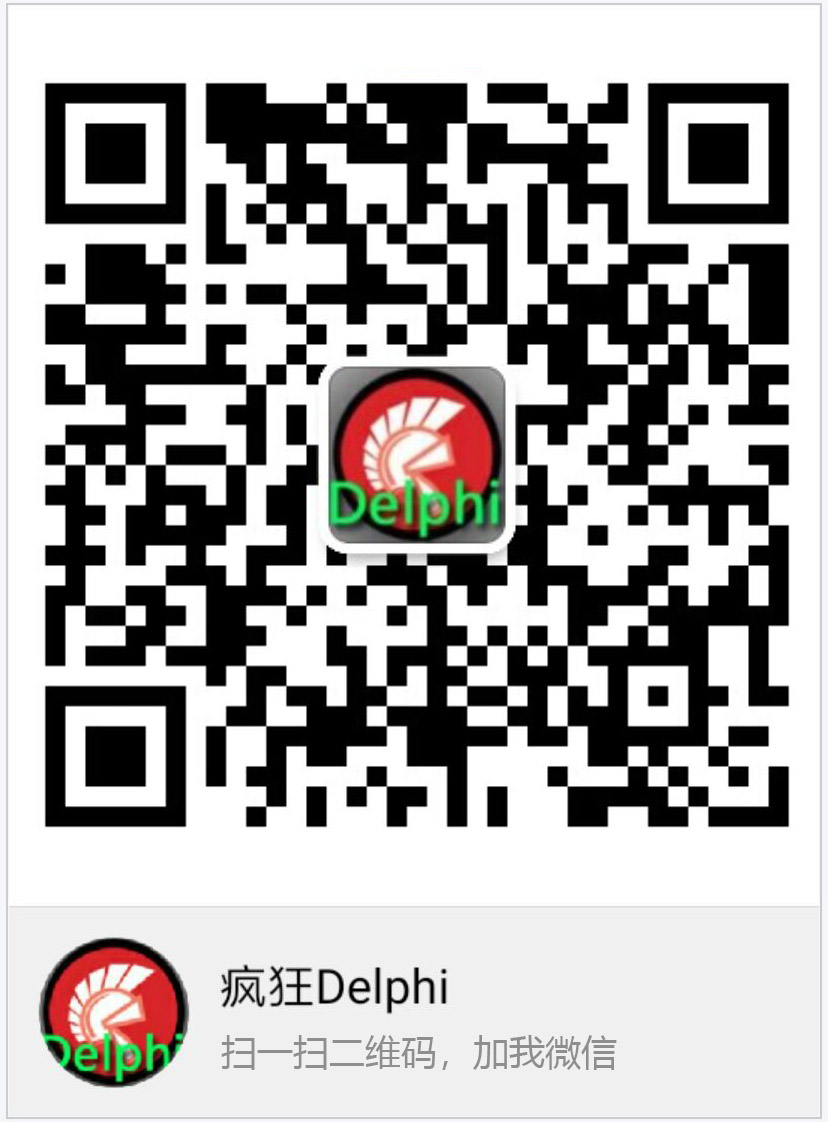