Qt使用QSound播放声音(支持WAV,不支持MP3)
相关资料:
https://www.ebaina.com/articles/140000004830 qt 用QSound播放音频文件
https://download.csdn.net/download/zhujianqiangqq/19518919 代码包下载
.pro

1 QT += core gui 2 QT += multimedia 3 4 greaterThan(QT_MAJOR_VERSION, 4): QT += widgets 5 6 CONFIG += c++11 7 8 # The following define makes your compiler emit warnings if you use 9 # any Qt feature that has been marked deprecated (the exact warnings 10 # depend on your compiler). Please consult the documentation of the 11 # deprecated API in order to know how to port your code away from it. 12 DEFINES += QT_DEPRECATED_WARNINGS 13 14 # You can also make your code fail to compile if it uses deprecated APIs. 15 # In order to do so, uncomment the following line. 16 # You can also select to disable deprecated APIs only up to a certain version of Qt. 17 #DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0 18 19 SOURCES += \ 20 main.cpp \ 21 mainwindow.cpp 22 23 HEADERS += \ 24 mainwindow.h 25 26 FORMS += \ 27 mainwindow.ui 28 29 # Default rules for deployment. 30 qnx: target.path = /tmp/$${TARGET}/bin 31 else: unix:!android: target.path = /opt/$${TARGET}/bin 32 !isEmpty(target.path): INSTALLS += target
main.cpp

1 #include "mainwindow.h" 2 3 #include <QApplication> 4 5 int main(int argc, char *argv[]) 6 { 7 QApplication a(argc, argv); 8 MainWindow w; 9 w.show(); 10 return a.exec(); 11 }
mainwindow.h

1 #ifndef MAINWINDOW_H 2 #define MAINWINDOW_H 3 4 #include <QMainWindow> 5 #include <QSound> 6 7 QT_BEGIN_NAMESPACE 8 namespace Ui { class MainWindow; } 9 QT_END_NAMESPACE 10 11 class MainWindow : public QMainWindow 12 { 13 Q_OBJECT 14 15 public: 16 MainWindow(QWidget *parent = nullptr); 17 ~MainWindow(); 18 19 private slots: 20 void on_pushButton_clicked(); 21 22 void on_pushButton_2_clicked(); 23 24 void on_pushButton_4_clicked(); 25 26 private: 27 Ui::MainWindow *ui; 28 QSound *m_pSound; 29 }; 30 #endif // MAINWINDOW_H
mainwindow.cpp

1 #include "mainwindow.h" 2 #include "ui_mainwindow.h" 3 4 MainWindow::MainWindow(QWidget *parent) 5 : QMainWindow(parent) 6 , ui(new Ui::MainWindow) 7 { 8 ui->setupUi(this); 9 m_pSound = new QSound("D:/QtDemo/QtQSound/QtQSound/Vishnu.wav", this); //构建对象 10 } 11 12 MainWindow::~MainWindow() 13 { 14 delete ui; 15 } 16 17 18 void MainWindow::on_pushButton_clicked() 19 { 20 m_pSound->play();//播放 21 } 22 23 void MainWindow::on_pushButton_2_clicked() 24 { 25 m_pSound->stop();//停止 26 } 27 28 void MainWindow::on_pushButton_4_clicked() 29 { 30 m_pSound->setLoops(-1);//设置循环次数 31 }
mainwindow.ui

1 <?xml version="1.0" encoding="UTF-8"?> 2 <ui version="4.0"> 3 <class>MainWindow</class> 4 <widget class="QMainWindow" name="MainWindow"> 5 <property name="geometry"> 6 <rect> 7 <x>0</x> 8 <y>0</y> 9 <width>578</width> 10 <height>213</height> 11 </rect> 12 </property> 13 <property name="windowTitle"> 14 <string>MainWindow</string> 15 </property> 16 <widget class="QWidget" name="centralwidget"> 17 <widget class="QPushButton" name="pushButton"> 18 <property name="geometry"> 19 <rect> 20 <x>470</x> 21 <y>40</y> 22 <width>75</width> 23 <height>23</height> 24 </rect> 25 </property> 26 <property name="text"> 27 <string>播放</string> 28 </property> 29 </widget> 30 <widget class="QPushButton" name="pushButton_2"> 31 <property name="geometry"> 32 <rect> 33 <x>470</x> 34 <y>70</y> 35 <width>75</width> 36 <height>23</height> 37 </rect> 38 </property> 39 <property name="text"> 40 <string>暂停</string> 41 </property> 42 </widget> 43 <widget class="QPushButton" name="pushButton_4"> 44 <property name="geometry"> 45 <rect> 46 <x>470</x> 47 <y>100</y> 48 <width>75</width> 49 <height>23</height> 50 </rect> 51 </property> 52 <property name="text"> 53 <string>次数无限</string> 54 </property> 55 </widget> 56 <widget class="QLineEdit" name="lineEdit"> 57 <property name="geometry"> 58 <rect> 59 <x>30</x> 60 <y>10</y> 61 <width>511</width> 62 <height>20</height> 63 </rect> 64 </property> 65 </widget> 66 </widget> 67 <widget class="QMenuBar" name="menubar"> 68 <property name="geometry"> 69 <rect> 70 <x>0</x> 71 <y>0</y> 72 <width>578</width> 73 <height>23</height> 74 </rect> 75 </property> 76 </widget> 77 <widget class="QStatusBar" name="statusbar"/> 78 </widget> 79 <resources/> 80 <connections/> 81 </ui>
作者:疯狂Delphi
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利.
欢迎关注我,一起进步!扫描下方二维码即可加我
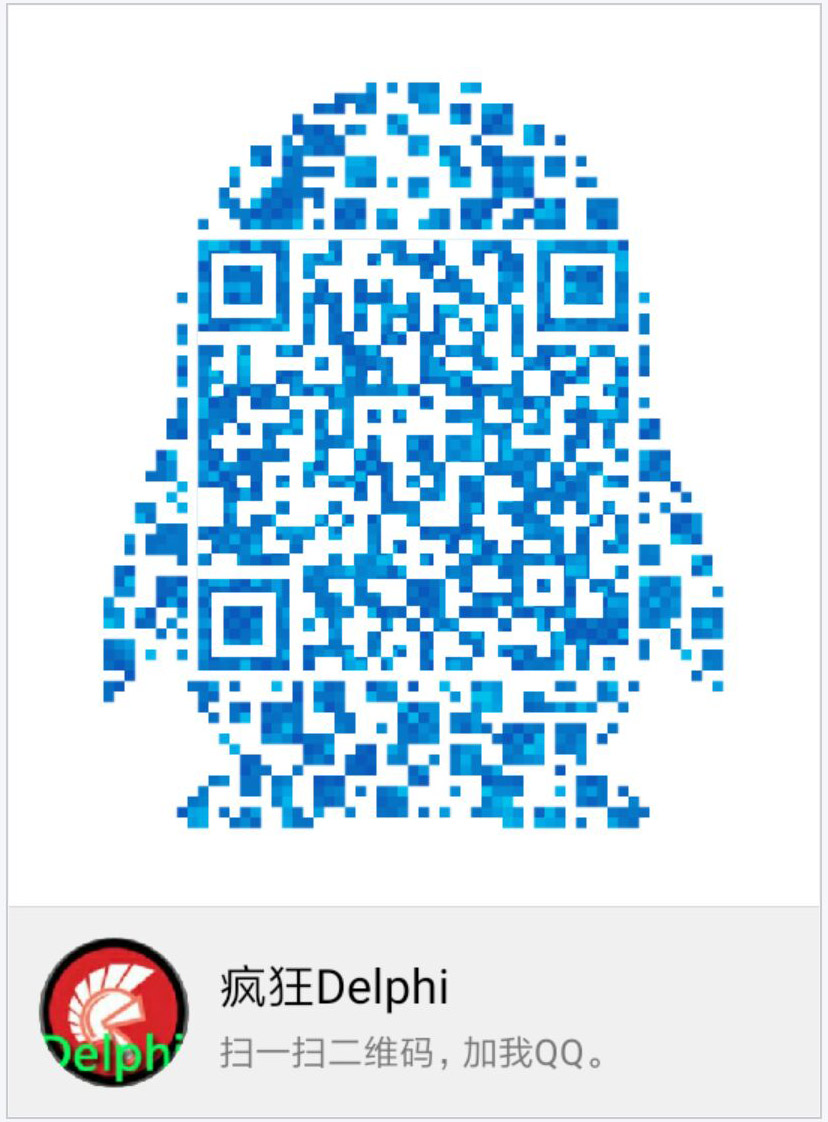
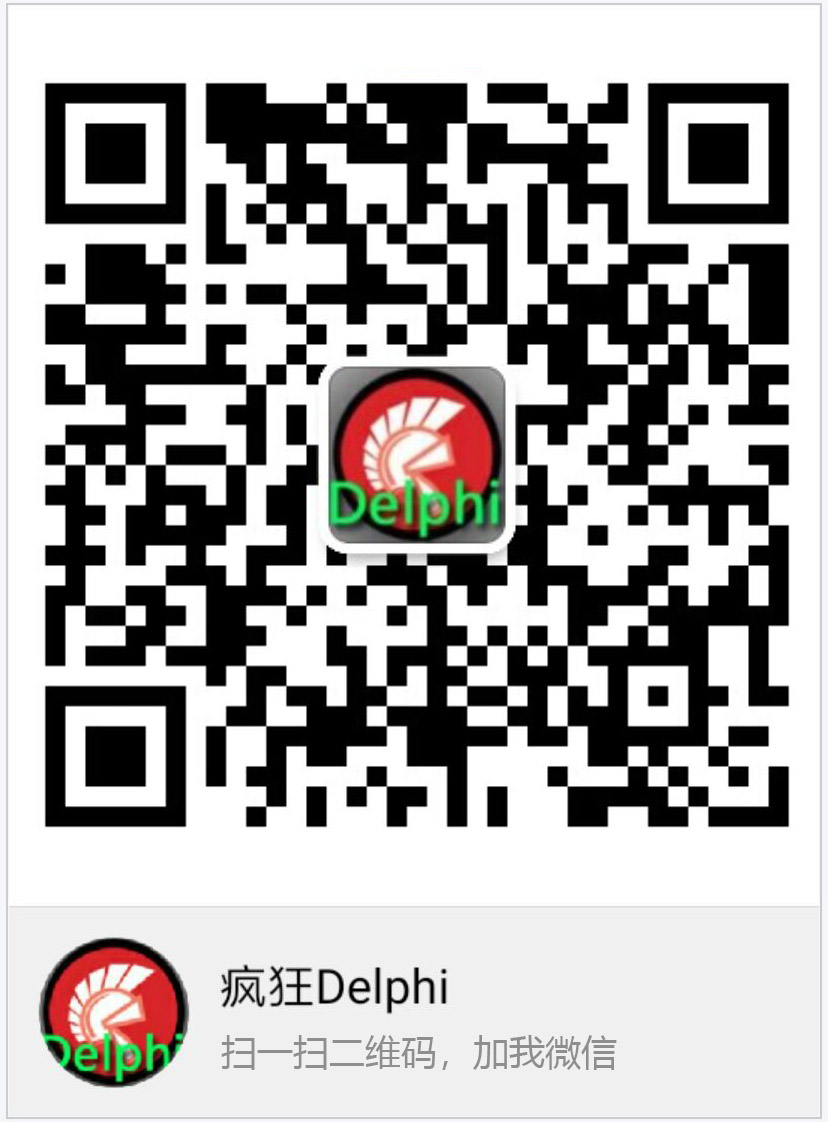