类的声明
(1)记录
//记录的定义形式
type
MyRec=record
aa:double;
bb:Integer;
cc:Char;
end;
//使用记录的方法如下:
var
Rec:MyRec; //先说明
begin
Rec.aa:=123.45; //记录赋值
Rec.bb:=12;
Rec.cc:='C';
end;
(2)集合
集合可以说是记录的一种特殊形式,它也是由多个数据组成,但要求数据是有序的,如有序的数、字符、枚举等,它规定集合元素最多不超过256个。集合用关键字set of声明:
type
I_Set=set of 1..100; //集合元素从1~100
C_Set=set of 'A'..'Z'; //集合元素从'A'-'Z'
N_Set=set of Char; //集合元素从#0..#255
TEnum=(aal,aa2,aa3,aa4,aa5);
E_Set=set of TEnum; //集合元素是枚举中的元素
以上的声明都是合法的,再看看下面的声明:
type
My_Set1=set of 1..1000;
My_Set2=set of Integer;
My_Set3=set of double;
My_Set4=set of string;
这4种声明都是不合法的,第一种与第二种的错误是,超过了集合规定的范围256,第三种与第四种不是有序类型
procedure TForm1.Buttonlclick(Sender:Tobject);
type
TA_Set=set of 'A'..'Z';
var
A_Set:TA_Set;
begin
A_Set:=['A'..'M']; //集合元素初始化
//关系运算第一种情况,是否在集合中
if 'C' in A_Set then
ShowMessage('C在集合中');
else
ShowMessage('C不在集合中');
//关系运算第二种情况,是否不在集合中
if not ('Z' in A Set)then
ShowMessage('Z不在集合中');
else
ShowMessage('z在集合中');
Include(A_Set,'O'); //集合中加元素的第一种方法,用Include
A_Set:=A_Set+['P']; //集合中加元素的第二种方法,用+号
Exclude(A_Set,'B'); //集合中减元素第一种方法,用Exclude
A_Set:=A_Set-['C','D']; //集合中减元素第二种方法,用-号
if ['E','F','G','H']*A_Set=['E','E','G','H']then //交集运算,用*号
ShowMessage('交集中有E、F、G、H');
else
ShowMessage('交集中没有E、F、G、H');
end;
(3)类
面向对象的方法是一种打包法,现在什么东西都讲究包装,编程序也不例外,面向对象的方法就是将完成某一种功能的变量、函数进行包装,变成一个统一的整体,当调用这个整体时,就完成该整体所赋予的功能。
type
TMyC1ass=class(祖先类)
private
aa,bb:Integer; //私有变量
function GetItem(Index:Integer):ListColumn: //私有函数
procedure SetItem(Index:Integer;Value:TListColumn); //私有过程
public
constructor Create(Owner:TComponent);override; //创建过程
destructor Destroy;override; //毁坏过程
P_aa,P_bb:Integer; //公有变量
function P GetItem(Index:Integer):TListColumn; //公有函数
procedure P SetItem(Index:Integer;Value:TListColumn);//公有过程
end;
类是一种数据类型,其内部可以有自己的元素(域)、自己的过程、自己的函数等,这些统称为类的成员。
类声明语法:
type
类名称=class
元素名:数据类型;
Procedure过程名称(参数列表);
Function函数名称(参数列表):函数返回值数据类型;
end;
实现:
procedure 类名称.过程名称(参数列表);
Begin
//过程内要执行的代码
End;
Function类名称.函数名称(参数列表):函数返回值数据类型;
Begin
//函数内要执行的代码
Result:=返回值;
end;
type
daxuesheng=class
mingzi:String;
nianling:Integer;
Procedure kaoshi();
Function fangxue()Integer;
end:
var
Form1:TForm1;
implementation
($R *.dfm)
Procedure daxuesheng.kaoshi();
begin
ShowMessage('要考试啦!');
end;
Function daxuesheng.fangxue()Integer;
begin
ShowMessage('我要放学了);
result :=0;
end;
(4)对象
通过类声明的变量,我们往往称为对象,或者类的实例。对象在使用前需要通过类.Create方法创建,使用完毕后通过对象.Free释放。
对象成员使用语法:
对象.成员
procedure TForm1.Button1click(Sender:TObjet);
var
i:xuesheng;
begin
i:= xuesheng.Create;
i.mingzi:= '呵呵';
i.nianling:= 12;
i.Free;
end;
procedure TForm1.Button2click(Sender:TObjet);
var
i: Array[2..10] of xuesheng;
n: Integer;
begin
for n:=2 to 10 do
begin
i[n]:=xuesheng.Create;
end;
i[2].mingzi:= '小刚';
i[2].nianling:= 13;
i.Free;
end;
(5)类的成员
类的成员(元素、过程、函数),均可以在类的定义内相互访问。
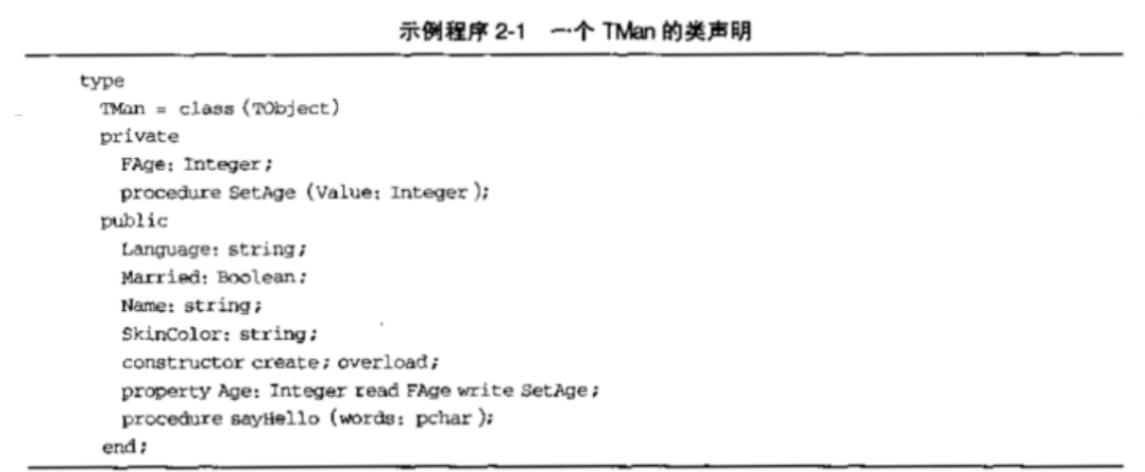
TMan类中:
- 数据成员(字段)有:Name、Language、SkinColor等,用于存储人的姓名、语言和肤色等信息;
- 属性有:Age,用于年龄的读写;
- 方法有:sayHello和SetAge,其中SetAge用于对Age属性的设置(写),以保证年龄合法(比如:没有负的年龄,或年龄都小于140)。
type
TMan = class (TObject)
private
FAge: Integer;
procedure SetAge(Value: Integer);
public
Language: string;
Married: Boolean;
Name: string;
SkinColor: string;
constructor create; overload;
property Age: Integer read FAge write SetAge;
procedure sayHello(words:pchar);
end;
implementation
{ ********************* TMan ***************************}
constructor TMan.create;
begin
Name:='张三';
Language:='中文';
SkinColor:='黄色';
FAge:=20;
Married:=True;
end;
procedure TMan.SetAge(Value: Integer);
begin
if Value<2 then FAge:=0
else FAge:=Value;
end;
procedure TMan.SayHello(Words:PChar);
begin
showmessage(words);
end;
end.
type
xuesheng =class
mingzi:String;
procedure GetMingzi();
end;
var
Form1:TForm1;
implementation
{sR*.dfm}
procedure xuesheng.GetMingzi();
begin
ShowMesssage('你好');
end;
procedure TForm1.Button1Click(Sender:TObject);
var
A:xuesheng;
B:xuesheng;
begin
A :xuesheng.Create;
B :xuesheng.Create;
A.mingzi:='小明';
A.GetMingzi();
B.mingzi:='小刚';
B.GetMingzi();
B.Free;
A.Free;
end;
//Unit2定义函数
unit Unit2;
interface
Function jisuan(a:Integer;b:Integer):Integer;
implementation
Function jisuan(a:Integer;b:Integer):Integer;
begin
Result :a+b;
end;
//Unit1可以调用Unit2中的函数
uses Unit2;
{$R*.dfm}
procedure TForm1.Buttoniclick(Sender:TObjet);
var
i:Integer;
begin
i:=jisuan(12,13);
ShowMessage (IntToStr(i));
end;
//Unit2定义类
unit Unit2;
interface
type
xuesheng Class
mingzi:String;
nianling:Integer;
end;
//Unit1可以调用Unit2中的类
implementation
uses Unit2;
{$R *dfm}
procedure TForm1.Button1click(Sender:TObject);
var
i:xuesheng;
begin
i:=xuesheng.Create;
i.mingzi:='小明';
ShowMessage(i.mingzi);
i.Free;
end;
(6)类成员访问权限
Private:该类型的成员只能在声明类中被访问。
Public:该类型的成员可以在声明堡中访问的同时,也可以在声明类外被访问。
Public成员往往被称为类的接口,而Private成员被隐藏起来,形成了“封装”。
type
xuesheng=Class
Private
mingzi:String;
Public
nianling:Integer;
end;
//Unit2定义类
type
xuesheng=class
mingzi:String;
procedure SetMingzi(s:String);
function GetMingzi():String;
end;
implementation
procedure xuesheng.SetMingzi(s:String);
begin
mingzi := s;
end;
function xuesheng.GetMingzi():String;
begin
Result := mingzi;
end;
//Unit1可以调用Unit2中的类
implementation
uses Unit2:
{$R*.dfm}
procedure TForm1.Button1click(Sender:TObject);
var
i:xuesheng;
begin
i := xuesheng.Create;
i.SetMingZi('中国'):
ShouMessage(i.GetMingzi());
i.Free;
end;
(7)过程的嵌套、递归
过程定义:
Procedure过程名称(参数列表);
Begin
//过程内要执行的代码
End;
- 过程的嵌套:某个过程在执行中,可以调用另外一个过程。
- 过程的递归:某个过程在执行中,可以调用过程本身。
implementation
{sR*.dfm}
procedure A();
begin
ShowMessage('我被执行了!');
end;
procedure B();
begin
ShowMessage('我是过程B');
A();
end;
procedure TForm1.Button1click(Sender:TObje
begin
B();
end;
或者n的阶乘程序实现:
implementation
{sR*.dfm}
procedure A(n:Integer);
begin
if n>0 then begin
ShowMessage('参数的值为:'+IntToStr(n));
A(n-1);
end;
end;
procedure TForm1.Button1click(Sender:TObject);
begin
A(3):
end;