LintCode: Two Sum
C++
hash map
把查找2个数的过程转换为查找1个数
借用STL容器 unordered_map
1 class Solution { 2 public: 3 /* 4 * @param numbers : An array of Integer 5 * @param target : target = numbers[index1] + numbers[index2] 6 * @return : [index1+1, index2+1] (index1 < index2) 7 */ 8 vector<int> twoSum(vector<int> &nums, int target) { 9 // write your code here 10 vector<int> result; 11 int n = nums.size(); 12 if (0 == n) { 13 return result; 14 } 15 // first value, second index 16 unordered_map<int, int> hash(n); 17 for (int i = 0; i < n; i++) { 18 if (hash.find(target - nums[i]) != hash.end()) { 19 result.push_back(hash[target - nums[i]]); 20 result.push_back(i + 1); 21 return result; 22 } else { 23 hash[nums[i]] = i + 1; 24 } 25 } 26 } 27 };
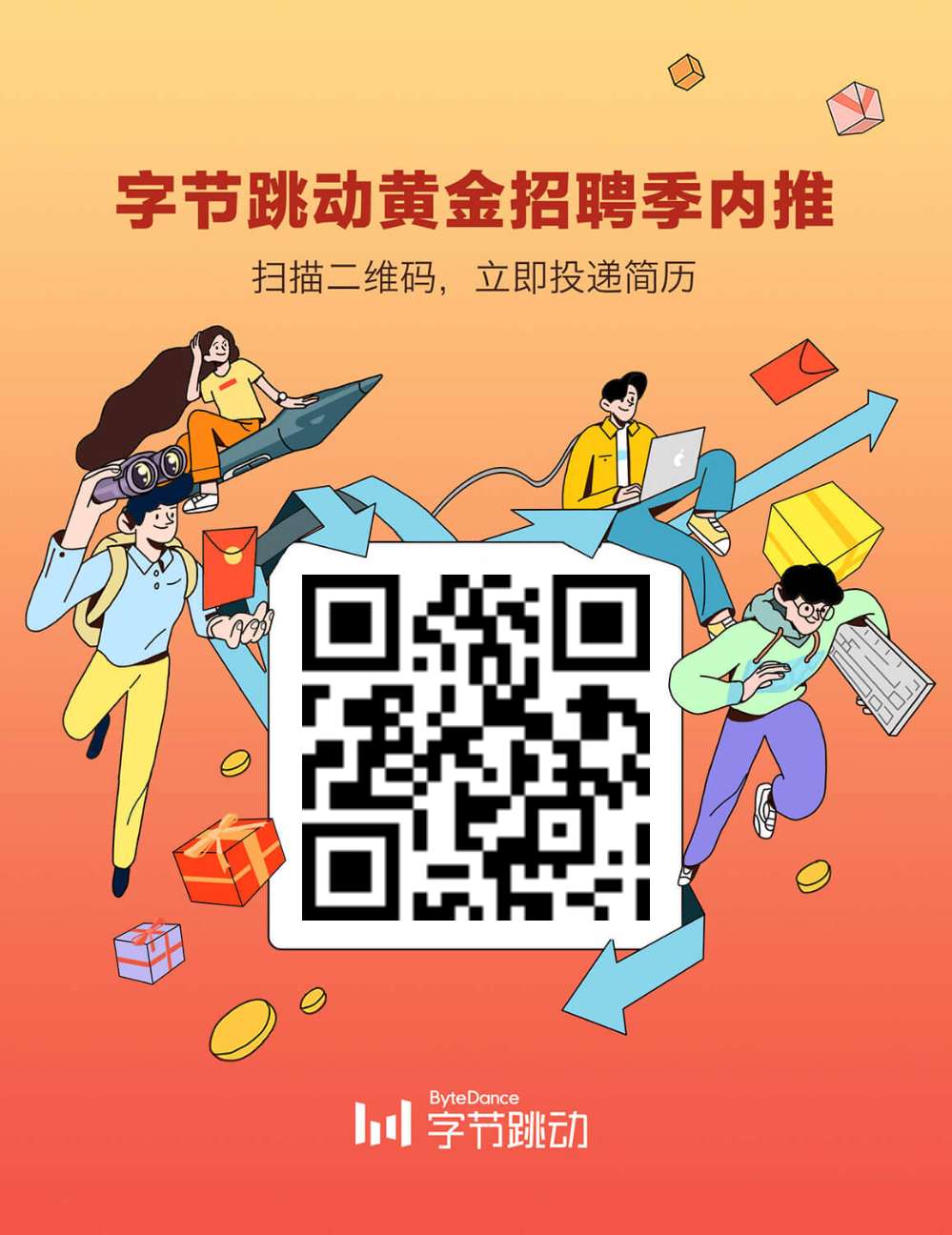
找我内推: 字节跳动各种岗位
作者:
ZH奶酪(张贺)
邮箱:
cheesezh@qq.com
出处:
http://www.cnblogs.com/CheeseZH/
*
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利。