题目来源:http://acm.hdu.edu.cn/showproblem.php?pid=3529
Bomberman - Just Search!
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)
Total Submission(s): 242 Accepted Submission(s): 112
Problem Description
Bomberman has been a very popular game ever since it was released. As you can see above, the game is played in an N*M rectangular room. Bomberman can go around the room and place bombs. Bombs explode in 4 directions with radius r. To finish a stage, bomberman has to defeat all the foes with his bombs and find an exit behind one of the walls.
Since time is limited, bomberman has to do this job quite efficiently. Now he has successfully defeated all the foes, and is searching for the exit. It's really troublesome to destroy the walls one by one, so he's asking for your help to calculate the minimal number of bombs he has to place in order to destroy all the walls, thus he can surely find the exit.
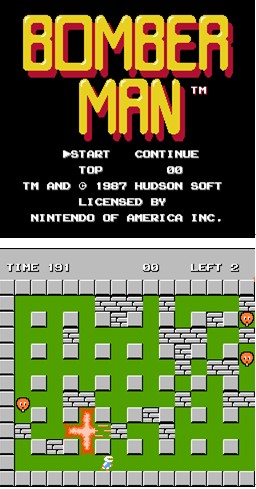
Since time is limited, bomberman has to do this job quite efficiently. Now he has successfully defeated all the foes, and is searching for the exit. It's really troublesome to destroy the walls one by one, so he's asking for your help to calculate the minimal number of bombs he has to place in order to destroy all the walls, thus he can surely find the exit.
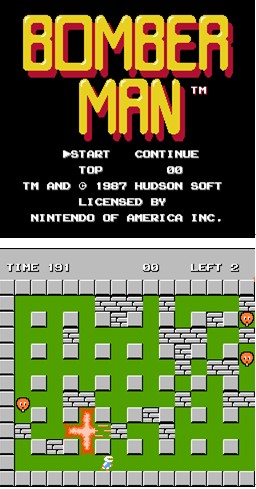
Input
The input contains several cases. Each case begins with two integers: N and M(4 <= N, M <= 15). N lines follow, each contains M characters, describing the room. A '*' means a concrete wall which can never be destroyed, a '#' is an ordinary wall that can be destroyed by a single bomb, a '.' is an empty space where bombs can only be placed. There're at most 30 ordinary walls. The borders of the room is always surrounded by concrete walls, as you can see from the samples. You may assume that the explosion radius r is infinite, so that an explosion can reach as far as possible until it meets a wall and destroys it if it's an ordinary one. Proceed until the end of file.
Output
For each case, output the minimal number of bombs that should be placed to destroy all the ordinary walls. Note that two bombs can't be placed at the same position and all bombs explode simultaneously, which makes the result for the second sample to be 3 instead of 2. You may assume that there's always a solution.
Sample Input
9 11
***********
*#.#...#.#*
*.*.*.*.*.*
*.........*
*.*.*.*.*.*
*....#....*
*.*.*.*.*.*
*....#....*
***********
3 13
*************
*..##...##..*
*************
Sample Output
3
3
题目大意:炸弹超人,不过不是让你炸 foes ,而是 foes 已经被消灭完了,你只需要炸开门就行了,一个炸弹最多威力将无限大,直到他遇到一堵墙,
如果这个墙是ordinary,则能把墙炸开。 也就是说一个炸弹最多能炸开四面墙(上下左右)。让你用最少的炸弹把所有的墙都炸开,所有的炸弹都要同时放,且一个点上只能放
一个炸弹。
思路:因为题上已经说的很明确了,所有的炸弹同时放,所以可以用Dancing Links求解。所有的#当列,然后所有能炸开该墙的“.”在这以列上,构造了一个0-1矩阵,
直接重复覆盖就可以了。
30 | zyzamp | 78MS | 204K | 2228B | C++ | 2011-08-07 20:10:14 |
code:

1 //Problem : 3529 ( Bomberman - Just Search! ) Judge Status : Accepted
2 //RunId : 4355959 Language : C++ Author : zhuyawei
3 //Code Render Status : Rendered By HDOJ C++ Code Render Version 0.01 Beta
4 # include<stdio.h>
5 # include<string.h>
6 # define N 200
7 # define V 900
8 int n,m,size,ak;
9 int U[V],D[V];
10 int L[V],R[V];
11 int C[V];
12 int H[N],S[N];
13 int visit[17][17];
14 int rr,cc;//表示0-1矩阵的行和列
15 char map[17][17];
16 void Link(int r,int c)
17 {
18 S[c]++;C[size]=c;
19 U[size]=U[c];D[U[c]]=size;
20 D[size]=c;U[c]=size;
21 if(H[r]==-1) H[r]=L[size]=R[size]=size;
22 else
23 {
24 L[size]=L[H[r]];R[L[H[r]]]=size;
25 R[size]=H[r];L[H[r]]=size;
26 }
27 size++;
28 }
29 void remove(int c)
30 {
31 int i;
32 for(i=D[c];i!=c;i=D[i])
33 L[R[i]]=L[i],R[L[i]]=R[i];
34 }
35 void resume(int c)
36 {
37 int i;
38 for(i=U[c];i!=c;i=U[i])
39 L[R[i]]=R[L[i]]=i;
40 }
41 int h()
42 {
43 int i,j,k,count=0;
44 bool hash[N];
45 memset(hash,0,sizeof(hash));
46 for(i=R[0];i;i=R[i])
47 {
48 if(hash[i]) continue;
49 hash[i]=1;
50 count++;
51 for(j=D[i];j!=i;j=D[j])
52 for(k=R[j];k!=j;k=R[k])
53 hash[C[k]]=1;
54 }
55 return count;
56 }
57 void Dance(int k)
58 {
59 int i,j,Min,c;
60 if(h()+k>=ak) return;
61 if(!R[0])
62 {
63 if(k<ak) ak=k;
64 return;
65 }
66 for(Min=N,i=R[0];i;i=R[i])
67 if(Min>S[i]) Min=S[i],c=i;
68 for(i=D[c];i!=c;i=D[i])
69 {
70 remove(i);
71 for(j=R[i];j!=i;j=R[j])
72 remove(j);
73 Dance(k+1);
74 for(j=L[i];j!=i;j=L[j])
75 resume(j);
76 resume(i);
77 }
78 }
79 int main()
80 {
81 int i,j,k1,k2;
82 while(scanf("%d%d",&n,&m)!=EOF)
83 {
84 cc=0;
85 memset(visit,0,sizeof(visit));
86 for(i=0;i<n;i++)
87 {
88 scanf("%s",map[i]);
89 for(j=0;map[i][j];j++)
90 if(map[i][j]=='#') visit[i][j]=++cc;
91 }
92 for(i=0;i<=cc;i++)
93 {
94 S[i]=0;
95 D[i]=U[i]=i;
96 L[i+1]=i;R[i]=i+1;
97 }R[cc]=0;
98 size=cc+1;
99 memset(H,-1,sizeof(H));
100 rr=0;
101 for(i=1;i<n-1;i++)
102 {
103 for(j=1;j<m-1;j++)
104 if(map[i][j]=='.')
105 {
106 rr++;
107 k1=i-1;k2=j;
108 while(map[k1][k2]=='.') k1--;
109 if(map[k1][k2]=='#') Link(rr,visit[k1][k2]);
110
111 k1=i+1;k2=j;
112 while(map[k1][k2]=='.') k1++;
113 if(map[k1][k2]=='#') Link(rr,visit[k1][k2]);
114
115 k1=i;k2=j-1;
116 while(map[k1][k2]=='.') k2--;
117 if(map[k1][k2]=='#') Link(rr,visit[k1][k2]);
118
119 k1=i;k2=j+1;
120 while(map[k1][k2]=='.') k2++;
121 if(map[k1][k2]=='#') Link(rr,visit[k1][k2]);
122 //分别向四个方向进行搜索
123 }
124 }
125 ak=N;
126 Dance(0);
127 printf("%d\n",ak);
128 }
129 return 0;
130 }