第十一十二周作业
图片一 用内部存储实现文件写入和读取功能
package com.example.innerfilesave; import androidx.appcompat.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.EditText; import android.widget.Toast; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); findViewById(R.id.b1); findViewById(R.id.b2); EditText editText1=findViewById(R.id.et_in); EditText editText2=findViewById(R.id.et_out); } public void click1(View view){ EditText editText1=findViewById(R.id.et_in); EditText editText2=findViewById(R.id.et_out); String filename="data.txt"; String context=editText1.getText().toString(); FileOutputStream fos=null; try { fos=openFileOutput(filename,MODE_PRIVATE); fos.write(context.getBytes()); } catch (Exception e) { e.printStackTrace(); }finally { if (fos!=null) { try { fos.close(); } catch (IOException e) { e.printStackTrace(); } } } Toast.makeText(this,"保存成功",Toast.LENGTH_SHORT).show(); } public void click2(View view){ EditText editText2=findViewById(R.id.et_out); String context=""; FileInputStream fis=null; try { fis=openFileInput("data.txt"); byte[]buffer=new byte[fis.available()]; fis.read(buffer); context=new String(buffer); } catch (Exception e) { e.printStackTrace(); }finally { if (fis!=null) { try { fis.close(); } catch (IOException e) { e.printStackTrace(); } } } editText2.setText(context); } }
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity" android:orientation="vertical"> <EditText android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="输入你想要写入的内容" android:layout_margin="10dp" android:id="@+id/et_in"/> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="写入" android:layout_margin="10dp" android:id="@+id/b1" android:onClick="click1"/> <EditText android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="显示读取的内容" android:layout_margin="10dp" android:id="@+id/et_out"/> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="读取" android:layout_margin="10dp" android:id="@+id/b2" android:onClick="click2"/> </LinearLayout>
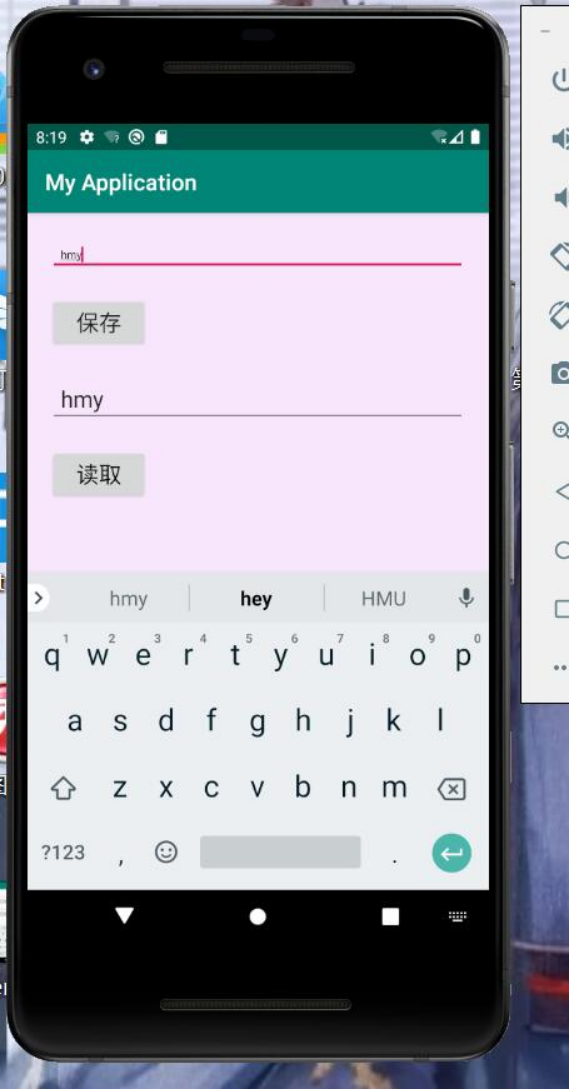
图片二 使用sharedpreference实现记住密码功能
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <!-- <TextView--> <!-- android:layout_width="wrap_content"--> <!-- android:layout_height="wrap_content"--> <!-- android:text="Hello World!"--> <!-- app:layout_constraintBottom_toBottomOf="parent"--> <!-- app:layout_constraintLeft_toLeftOf="parent"--> <!-- app:layout_constraintRight_toRightOf="parent"--> <!-- app:layout_constraintTop_toTopOf="parent" />--> <TextView android:id="@+id/tv1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="账号" android:textSize="25dp" android:layout_margin="10dp"/> <EditText android:id="@+id/et1" android:layout_width="300dp" android:layout_height="wrap_content" android:hint="请输入用户名" android:layout_toRightOf="@id/tv1"/> <TextView android:id="@+id/tv2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="密码" android:textSize="25dp" android:layout_margin="10dp" android:layout_below="@id/tv1"/> <EditText android:id="@+id/et2" android:layout_width="300dp" android:layout_height="wrap_content" android:hint="请输入密码" android:layout_toRightOf="@id/tv2" android:layout_below="@id/et1" android:layout_marginTop="10dp"/> <CheckBox android:id="@+id/cb1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@id/tv2" android:onClick="cbclick"/> <TextView android:id="@+id/tv3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="记住密码" android:layout_toRightOf="@id/cb1" android:layout_below="@id/tv2" android:textSize="20dp"/> <CheckBox android:id="@+id/cb2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@id/tv2" android:layout_toRightOf="@id/tv3"/> <TextView android:id="@+id/tv4" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="自动登录" android:layout_toRightOf="@id/cb2" android:layout_below="@id/tv2" android:textSize="20dp" /> <Button android:id="@+id/bt1" android:layout_width="100dp" android:layout_height="50dp" android:text="登录" android:layout_toRightOf="@id/tv4" android:layout_below="@id/et2" android:onClick="btclick"/> import androidx.appcompat.app.AppCompatActivity; import android.view.View; import android.widget.EditText; import android.content.SharedPreferences; import android.os.Bundle; public class MainAct
</RelativeLayout> package com.example.work5_2;ivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } public void btclick(View view){ String et1=((EditText)(findViewById(R.id.et1))).getText().toString(); String et2=((EditText)(findViewById(R.id.et2))).getText().toString(); SharedPreferences sp=getSharedPreferences("cdate",MODE_PRIVATE); SharedPreferences.Editor editor=sp.edit(); editor.putString("zh", et1); editor.putString("mm", et2); editor.commit(); } public void cbclick(View view){ SharedPreferences sp=getSharedPreferences("cdate", MODE_PRIVATE); String zh=sp.getString("zh", ""); String mm=sp.getString("mm", ""); EditText et1=(EditText)findViewById(R.id.et1); EditText et2=(EditText)findViewById(R.id.et2); et1.setText(zh); et2.setText(mm); } }