#include "struct.h"
#include "TesSys.h"
typedef enum _SYSTEM_INFORMATION_CLASS // Q S
{
SystemProcessesAndThreadsInformation, // 05 Y N
SystemCallCounts, // 06 Y N
SystemConfigurationInformation, // 07 Y N
SystemProcessorTimes, // 08 Y N
SystemGlobalFlag, // 09 Y Y
SystemNotImplemented2, // 10 Y N
SystemModuleInformation, // 11 Y N
SystemLockInformation, // 12 Y N
SystemNotImplemented3, // 13 Y N
SystemNotImplemented4, // 14 Y N
SystemNotImplemented5, // 15 Y N
SystemHandleInformation, // 16 Y N
SystemObjectInformation, // 17 Y N
SystemPagefileInformation, // 18 Y N
SystemInstructionEmulationCounts, // 19 Y N
SystemInvalidInfoClass1, // 20
SystemCacheInformation, // 21 Y Y
SystemPoolTagInformation, // 22 Y N
SystemProcessorStatistics, // 23 Y N
SystemDpcInformation, // 24 Y Y
SystemNotImplemented6, // 25 Y N
SystemLoadImage, // 26 N Y
SystemUnloadImage, // 27 N Y
SystemTimeAdjustment, // 28 Y Y
SystemNotImplemented7, // 29 Y N
SystemNotImplemented8, // 30 Y N
SystemNotImplemented9, // 31 Y N
SystemCrashDumpInformation, // 32 Y N
SystemExceptionInformation, // 33 Y N
SystemCrashDumpStateInformation, // 34 Y Y/N
SystemKernelDebuggerInformation, // 35 Y N
SystemContextSwitchInformation, // 36 Y N
SystemRegistryQuotaInformation, // 37 Y Y
SystemLoadAndCallImage, // 38 N Y
SystemPrioritySeparation, // 39 N Y
SystemNotImplemented10, // 40 Y N
SystemNotImplemented11, // 41 Y N
SystemInvalidInfoClass2, // 42
SystemInvalidInfoClass3, // 43
SystemTimeZoneInformation, // 44 Y N
SystemLookasideInformation, // 45 Y N
SystemSetTimeSlipEvent, // 46 N Y
SystemCreateSession, // 47 N Y
SystemDeleteSession, // 48 N Y
SystemInvalidInfoClass4, // 49
SystemRangeStartInformation, // 50 Y N
SystemVerifierInformation, // 51 Y Y
SystemAddVerifier, // 52 N Y
SystemSessionProcessesInformation // 53 Y N
} SYSTEM_INFORMATION_CLASS;
typedef
struct _SYSTEM_MODULE_ENTRY
{
ULONG Unknown1;
ULONG Unknown2;
PVOID BaseAddress;
ULONG Size;
ULONG Flags;
ULONG EntryIndex;
USHORT NameLength; // Length of module name not including the path, this field contains valid value only for NTOSKRNL module
USHORT PathLength; // Length of 'directory path' part of modulename
CHAR Name[256];
} SYSTEM_MODULE_ENTRY, * PSYSTEM_MODULE_ENTRY;
typedef
struct _SYSTEM_MODULE_INFORMATION
{
ULONG Count;
SYSTEM_MODULE_ENTRY Module [1];
} SYSTEM_MODULE_INFORMATION, *PSYSTEM_MODULE_INFORMATION;
NTSTATUS
NTAPI
ZwQuerySystemInformation(
IN SYSTEM_INFORMATION_CLASS SystemInformationClass,
IN OUT PVOID SystemInformation,
IN ULONG SystemInformationLength,
OUT PULONG ReturnLength OPTIONAL
);
PEPROCESS g_SysProcess = NULL;
PVOID KernelGetModuleBase(PCHAR pModuleName)
{
PVOID pModuleBase = NULL;
PULONG pSystemInfoBuffer = NULL;
__try
{
NTSTATUS status = STATUS_INSUFFICIENT_RESOURCES;
ULONG SystemInfoBufferSize = 0;
status = ZwQuerySystemInformation(SystemModuleInformation,
&SystemInfoBufferSize,
0,
&SystemInfoBufferSize);
if (!SystemInfoBufferSize)
return NULL;
pSystemInfoBuffer = (PULONG)ExAllocatePool(NonPagedPool, SystemInfoBufferSize*2);
if (!pSystemInfoBuffer)
return NULL;
memset(pSystemInfoBuffer, 0, SystemInfoBufferSize*2);
status = ZwQuerySystemInformation(SystemModuleInformation,
pSystemInfoBuffer,
SystemInfoBufferSize*2,
&SystemInfoBufferSize);
if (NT_SUCCESS(status))
{
PSYSTEM_MODULE_ENTRY pSysModuleEntry =
((PSYSTEM_MODULE_INFORMATION)(pSystemInfoBuffer))->Module;
ULONG i;
for (i = 0; i <((PSYSTEM_MODULE_INFORMATION)(pSystemInfoBuffer))->Count; i++)
{
if (_stricmp(pSysModuleEntry[i].Name +
pSysModuleEntry[i].PathLength, pModuleName) == 0)
{
pModuleBase = pSysModuleEntry[i].BaseAddress;
break;
}
}
}
}
__except(EXCEPTION_EXECUTE_HANDLER)
{
pModuleBase = NULL;
}
if(pSystemInfoBuffer)
{
ExFreePool(pSystemInfoBuffer);
}
return pModuleBase;
}
VOID ProcessNotifyRoutine(HANDLE dwParentId, HANDLE dwProcessId, BOOL bCreate)
{
if ( bCreate )
{
PEPROCESS Process = NULL;
if( NT_SUCCESS(PsLookupProcessByProcessId(dwProcessId, &Process)) && Process )
{
DWORD Type = 0;
if( !_stricmp(PsGetProcessImageFileName(Process), "oll.exe") ) // OD进程
{
Type = 4;
}
else if( !_stricmp(PsGetProcessImageFileName(Process), "cc.exe") ) // CE进程
{
Type = 8;
}
if( Type )
{
if( NT_SUCCESS(PsLookupProcessByProcessId((HANDLE)4, &g_SysProcess)) && g_SysProcess )
{
PBYTE pBase, ppBase;
dprintf("发现exe\n");
pBase = KernelGetModuleBase("TesSafe.sys");
ppBase = pBase;
if ( pBase )
{
dprintf("发现 TesSafe.sys\n");
// 搜索 以下清除debugport的代码
//.text:00011509 xchg eax, [edx]
//.text:0001150B mov ecx, [ecx]
while ( MmIsAddressValid(pBase) )
{
if ( *(PDWORD)pBase == 0x098B0287 && *(PDWORD)(pBase + 4) == 0xED75CF3B )
{
dprintf("debugport:地址 %08X", pBase);
*(PDWORD)*(PDWORD)(pBase - 6) = 0x70; // 修改debugport的偏移
while ( MmIsAddressValid(pBase) )
{
if ( *(PDWORD)pBase == (DWORD)g_SysProcess ) // 搜索 进程为4 的 EPROCESS
{
dprintf("System EPROCESS:地址 %08X", pBase);
while ( MmIsAddressValid(pBase) )
{
if ( *(PDWORD)pBase == 0 )
{
dprintf("白名单地址 %08X", pBase);
*(PDWORD)(pBase - Type) = (DWORD)Process; // 将自身加入白名单
break;
}
pBase += 4;
}
break;
}
pBase ++;
}
break;
}
pBase ++;
}
}
}
}
}
}
}
typedef struct _SERVICE_DESCRIPTOR_TABLE {
ULONG *ServiceTableBase; //指向系统服务程序的地址(SSDT)
ULONG *ServiceCounterTableBase; //指向另一个索引表,该表包含了每个服务表项被调用的次数;不过这个值只在Checkd Build的内核中有效,在Free Build的内核中,这个值总为NULL
ULONG NumberOfServices; //表示当前系统所支持的服务个数
unsigned char *ParamTableBase; //指向SSDT中的参数地址,它们都包含了NumberOfService这么多个数组单元
} SERVICE_DESCRIPTOR_TABLE, *PSERVICE_DESCRIPTOR_TABLE;
typedef struct _SERVICE_DESCRIPTOR_TABLE *PSERVICE_DESCRIPTOR_TABLE;
extern PSERVICE_DESCRIPTOR_TABLE KeServiceDescriptorTable;
ULONG g_NtGetThreadContext = 0;
ULONG g_NtSetThreadContext = 0;
__declspec(naked) NTSTATUS _MyNtGetThreadContext(HANDLE hThread, PCONTEXT pContext)
{
__asm
{
jmp dword ptr[g_NtGetThreadContext]
}
}
__declspec(naked) NTSTATUS _MyNtSetThreadContext(HANDLE hThread, PCONTEXT pContext)
{
__asm
{
jmp dword ptr[g_NtSetThreadContext]
}
}
NTSTATUS MyNtGetThreadContext(HANDLE hThread, PCONTEXT pContext)
{
if ( _stricmp(PsGetProcessImageFileName(PsGetCurrentProcess()), "dnf.exe") )
{
return _MyNtGetThreadContext(hThread, pContext);
}
/*
if ( NT_SUCCESS(st) )
{
if ( !_stricmp(PsGetProcessImageFileName(PsGetCurrentProcess()), "dnf.exe") )
{
if ( MmIsAddressValid(pContext) )
{
pContext->Dr0 = 0;
pContext->Dr1 = 0;
pContext->Dr2 = 0;
pContext->Dr3 = 0;
pContext->Dr7 = 0;
dprintf("清除Drx\n");
}
}
}
*/
return STATUS_UNSUCCESSFUL;
}
NTSTATUS MyNtSetThreadContext(HANDLE hThread, PCONTEXT pContext)
{
if ( _stricmp(PsGetProcessImageFileName(PsGetCurrentProcess()), "dnf.exe") )
{
return _MyNtSetThreadContext(hThread, pContext);
}
DbgPrint("Dr7:%08X\n", pContext->Dr7);
if ( pContext->Dr7 == 0x101 )
{
return _MyNtSetThreadContext(hThread, pContext);
}
return STATUS_UNSUCCESSFUL;
}
VOID DriverUnload(PDRIVER_OBJECT pDriverObj)
{
__asm
{
push eax
cli
mov eax, cr0
and eax, not 0x10000
mov cr0, eax
pop eax
}
KeServiceDescriptorTable->ServiceTableBase[0xD5] = g_NtSetThreadContext;
KeServiceDescriptorTable->ServiceTableBase[0x55] = g_NtGetThreadContext;
__asm
{
push eax
mov eax, cr0
or eax, 0x10000
mov cr0, eax
sti
pop eax
}
PsSetCreateProcessNotifyRoutine(ProcessNotifyRoutine, TRUE);
dprintf("[TesSys] Unloaded\n");
}
NTSTATUS DriverEntry(PDRIVER_OBJECT pDriverObj, PUNICODE_STRING pRegistryString)
{
NTSTATUS status = STATUS_SUCCESS;
UNICODE_STRING ustrLinkName;
UNICODE_STRING ustrDevName;
PDEVICE_OBJECT pDevObj;
dprintf("[TesSys] DriverEntry\n");
pDriverObj->DriverUnload = DriverUnload;
__asm
{
push eax
cli
mov eax, cr0
and eax, not 0x10000
mov cr0, eax
pop eax
}
g_NtGetThreadContext = KeServiceDescriptorTable->ServiceTableBase[0x55];
KeServiceDescriptorTable->ServiceTableBase[0x55] = (ULONG)MyNtGetThreadContext;
g_NtSetThreadContext = KeServiceDescriptorTable->ServiceTableBase[0xD5];
KeServiceDescriptorTable->ServiceTableBase[0xD5] = (ULONG)MyNtSetThreadContext;
__asm
{
push eax
mov eax, cr0
or eax, 0x10000
mov cr0, eax
sti
pop eax
}
PsSetCreateProcessNotifyRoutine(ProcessNotifyRoutine, FALSE);
return STATUS_SUCCESS;
}
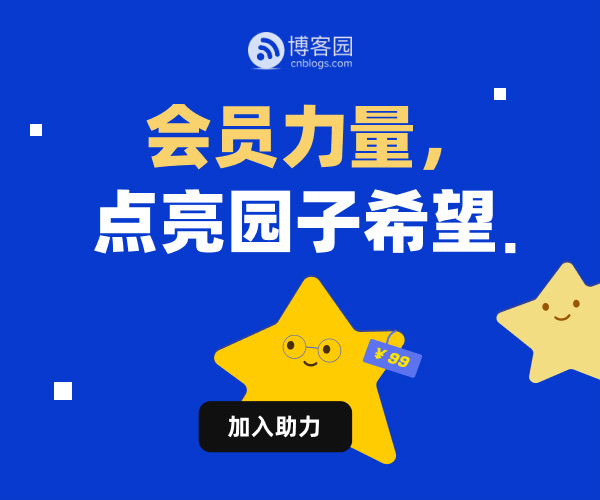
