享元模式(Flyweight Pattern)
享元模式(Flyweight Pattern)
一、定义
享元模式(Flyweight Pattern)主要用于减少创建对象的数量,以减少内存占用和提高性能。这种类型的设计模式属于结构型模式,它提供了减少对象数量从而改善应用所需的对象结构的方式。
运用共享技术有效地支持大量细粒度的对象。
二、优缺点
优点: 大大减少对象的创建,降低系统的内存,使效率提高。
缺点: 提高了系统的复杂度,需要分离出外部状态和内部状态,而且外部状态具有固有化的性质,不应该随着内部状态的变化而变化,否则会造成系统的混乱。
三、具体实现
3.1 原型图
- Flyweight抽象类:描述一个接口,通过这个接口可以接受并作用于外部状态。
- ConcreteFlyweight类:实现Flyweight接口,并为内部状态增加存储空间。ConcreteFlyweight对象必须是可共享的。它所存储的状态必须是内部的。
- UnsharedConcreteFlyweight类:并非所有的Flyweight子类都需要被共享。Flyweight接口使共享成为可能,但它并不强制共享。在Flyweight对象结构的某些层次,UnsharedConcreteFlyweight对象通常将ConcreteFlyweight对象作为子节点(Row和Column)
- FlyweightFactory类:创建并管理flywieght对象,确保合理地共享flyweight。当用户请求一个flyweight时,FlyweightFactory对象提供一个已创建的实例或创建一个(如果不存在的话)
- Client客户端:维持一个对flyweight的引用,计算或存储一个(多个)flyweight的外部状态。
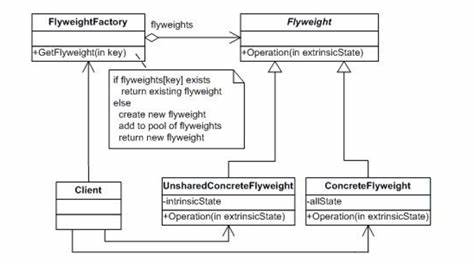
3.2 实现
1、创建一个Flyweight接口。
/**
* @author zhongtao
* @date 2023/5/23 22:10
*/
public interface Shape {
void draw();
}
2、创建实现接口的实体类ConcreteFlyweight。
/**
* @author zhongtao
* @date 2023/5/23 22:10
*/
public class Circle implements Shape {
private String color;
private int x;
private int y;
private int radius;
public Circle(String color) {
this.color = color;
}
public void setX(int x) {
this.x = x;
}
public void setY(int y) {
this.y = y;
}
public void setRadius(int radius) {
this.radius = radius;
}
@Override
public void draw() {
System.out.println("Circle: Draw() [Color : " + color
+ ", x : " + x + ", y :" + y + ", radius :" + radius);
}
}
3、创建一个工厂FlyweightFactory,生成基于给定信息的实体类的对象。
import java.util.HashMap;
/**
* @author zhongtao
* @date 2023/5/23 22:10
*/
public class ShapeFactory {
private static final HashMap<String, Shape> circleMap = new HashMap<>();
public static Shape getCircle(String color) {
Circle circle = (Circle) circleMap.get(color);
if (circle == null) {
circle = new Circle(color);
circleMap.put(color, circle);
System.out.println("Creating circle of color : " + color);
}
return circle;
}
}
4、使用该工厂,通过传递颜色信息来获取实体类的对象。
/**
* @author zhongtao
* @date 2023/5/23 22:11
*/
public class FlyweightPatternDemo {
private static final String colors[] =
{"Red", "Green", "Blue", "White", "Black"};
public static void main(String[] args) {
for (int i = 0; i < 20; ++i) {
Circle circle =
(Circle) ShapeFactory.getCircle(getRandomColor());
circle.setX(getRandomX());
circle.setY(getRandomY());
circle.setRadius(100);
circle.draw();
}
}
private static String getRandomColor() {
return colors[(int) (Math.random() * colors.length)];
}
private static int getRandomX() {
return (int) (Math.random() * 100);
}
private static int getRandomY() {
return (int) (Math.random() * 100);
}
}
5、执行程序,输出结果:
Creating circle of color : Green
Circle: Draw() [Color : Green, x : 22, y :58, radius :100
Circle: Draw() [Color : Green, x : 12, y :57, radius :100
Circle: Draw() [Color : Green, x : 17, y :42, radius :100
Circle: Draw() [Color : Green, x : 2, y :40, radius :100
Creating circle of color : Blue
Circle: Draw() [Color : Blue, x : 88, y :40, radius :100
Circle: Draw() [Color : Blue, x : 81, y :17, radius :100
Creating circle of color : Red
Circle: Draw() [Color : Red, x : 84, y :50, radius :100
Creating circle of color : White
Circle: Draw() [Color : White, x : 78, y :61, radius :100
Circle: Draw() [Color : Green, x : 4, y :49, radius :100
Creating circle of color : Black
Circle: Draw() [Color : Black, x : 83, y :81, radius :100
Circle: Draw() [Color : Blue, x : 42, y :29, radius :100
Circle: Draw() [Color : Black, x : 39, y :87, radius :100
Circle: Draw() [Color : Green, x : 57, y :94, radius :100
Circle: Draw() [Color : Red, x : 2, y :55, radius :100
Circle: Draw() [Color : Red, x : 17, y :30, radius :100
Circle: Draw() [Color : White, x : 80, y :57, radius :100
Circle: Draw() [Color : Red, x : 48, y :35, radius :100
Circle: Draw() [Color : Blue, x : 5, y :42, radius :100
Circle: Draw() [Color : White, x : 71, y :75, radius :100
Circle: Draw() [Color : Green, x : 3, y :23, radius :100