实验6 模板类和文件I/O
实验任务3
#include <iostream> #include <fstream> #include <array> #define N 5 int main() { using namespace std; array<int, N> x{ 97, 98, 99, 100, 101 }; ofstream out; out.open("data1.dat", ios::binary); if (!out.is_open()) { cout << "fail to open data1.dat\n"; return 1; } // 把从地址&x开始连续sizeof(x)个字节的数据块以字节数据块方式写入文件data1.txt out.write(reinterpret_cast<char*>(&x), sizeof(x)); out.close(); }
#include <iostream> #include <fstream> #include <array> #define N 5 int main() { using namespace std; array<int, N> x; ifstream in; in.open("data1.dat", ios::binary); if (!in.is_open()) { cout << "fail to open data1.dat\n"; return 1; } // 从文件流对象in关联的文件data1.dat中读取sizeof(x)字节数据写入&x开始的地址单元 in.read(reinterpret_cast<char*>(&x), sizeof(x)); in.close(); for (auto i = 0; i < N; ++i) cout << x[i] << ", "; cout << "\b\b \n"; }
把task3_2.cpp代码中line7的对象类型从 array<int, N> 改成 array<char, N> 后运行结果
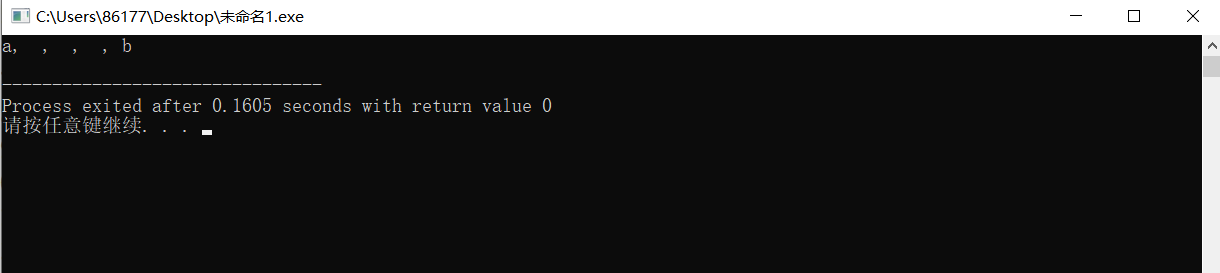
分析:task3_2是从文件流对象in关联的文件data1.dat中读取sizeof(x)字节数据写入&x开始的地址单元,改动前的int类型占四个字节,而改动后的char类型只占一个字节,所以第二次读取97时实际读取的是a与三个空字节,读取98时刚好是第五个字节b。
实验任务4
#include <iostream> #include "Vector.h" using namespace std; void text3() { int n; cin >> n; Vector<double>x1(n); for (auto i = 0; i < n; i++) x1.at(i) = i * 0.7; output(x1); Vector<int>x2(n, 42); Vector<int>x3(x2); output(x2); output(x3); x2.at(0) = 77; output(x2); x3[0] = 999; output(x3); } int main() { text3(); }
#pragma once #include<iostream> #include<cassert> using namespace std; template<typename T> class Vector { public: Vector(int n) :size{ n }{ p = new T[n]; } Vector(int n, T value); Vector(const Vector<T>& vp); int get_size()const; T& at(int i)const; T& operator[](int i)const; ~Vector<T>(); template<typename T1> friend void output(Vector<T1>& v); private: int size; T* p; }; template<typename T> Vector<T>::Vector(int n, T value):size{n} { p = new T[n]; for (auto i = 0; i < size; ++i) p[i] = value; } template<typename T> Vector<T>::Vector(const Vector<T>& vp) :size{ vp.size } { p = new T[size]; for (auto i = 0; i < size; ++i) p[i] = vp.p[i]; } template<typename T> int Vector<T>::get_size()const { return size; } template<typename T> T& Vector<T>::at(int i)const { return p[i]; } template<typename T> T& Vector<T>::operator[](int i)const { return p[i]; } template<typename T1> void output(Vector<T1>& v) { for (auto i = 0; i < v.size; ++i) cout << v.p[i] << " "; cout << endl; } template<typename T> Vector<T>::~Vector() { delete[] p; }
实验任务5
#include<iostream> #include<iomanip> #include<fstream> using namespace std; void output(ofstream&out) { int i,j,t,k; char w[27][27]; for (t = 0,j=0; j < 26; j++,t++) w[0][j] = 'a' + t; for(i=1,k=0;i<27;i++,k++) { char c = 'B' + k; if (c > 'Z') c = c - 26; for (j = 0, t = 0; j < 26; j++, t++) { w[i][j] = c + t; if (w[i][j] > 'Z') w[i][j] -= 26; } } cout << " "; out << " "; for (j = 0; j < 26; j++) { cout << setw(2) << w[0][j]; out << setw(2) << w[0][j]; } cout << endl; out << endl; for (i = 1; i < 27; i++) { cout << left << setw(3) << i; out << left << setw(3) << i; for (j = 0; j < 26; j++) { cout << left << setw(2) << w[i][j]; out << left<<setw(2) << w[i][j]; } cout << endl; out << endl; } } int main() { ofstream out; out.open("cipher_key.txt"); if (!out.is_open()) { cout << "fail to open data1.dat\n"; return 1; } output(out); out.close(); }