1. 开发时是在Mac+MySql, 尝试发布时是在SQL2005+Win 2008 (第一版)
在Startup.cs里,数据库连接要改,分页时netcore默认是用offset关键字分页, 如果用SQL2012之前的版本,就要改
//-----Use SqlServer, 默认用offset分页(这个是sql2012版本之后才有的功能) services.AddDbContextPool<CRMContext>( tt => tt.UseSqlServer(Configuration.GetConnectionString("SqlServerConnection"), opt=>opt.UseRowNumberForPaging()) );
2. SQL2005 没有datetime2类型, EFCore默认处理datetime是用datetime2类型
//如果db是SQL2005,没有datetime2的类型.要手工指定 modelBuilder.Entity<Announcement>().Property(t => t.CreateDate).HasColumnType("datetime"); modelBuilder.Entity<Announcement>().Property(t => t.ModifyDate).HasColumnType("datetime");
要批量修改,可以这样写
foreach (var entity in modelBuilder.Model.GetEntityTypes()) { foreach (var item in entity.GetProperties()) { if(item.ClrType.Name== "DateTime") item.AddAnnotation("Relational:ColumnType", "datetime"); //对应的annotation: [Column(TypeName ="datetime")] } }
SQL2005没有Merge语句, _context.SaveChanges(); 会出错: System.Data.SqlClient.SqlException (0x80131904): 'MERGE' 附近有语法错误。
TODO: 除非你不用批处理SQL语句,不然还是升级到新版本的SQLServer吧.
3. 发布的目录结构和之前的.net不同
4. Kestrel 服务器 如何启动?
我们知道.net core我们可以使用dotnet 命令方式去运行 .net core 应用,这种方式使我们的web不再依赖于iis,实现了跨平台。 我们先了解下命令: > dotnet run [options] [[--] arguments] dotnet run 命令会把我们的项目编译后直接运行,在开发的时候使用,如果是编译好的项目,则使用: > dotnet yourproject.dll [[--] arguments] 如果要更改启动端口,修改Properties\lauchsetting.json的 applicationUrl值
5. 如何发布到IIS7?
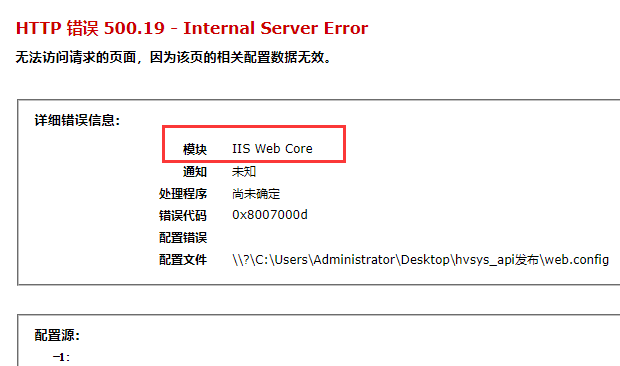
需要检查IIS里有没有这2个模块
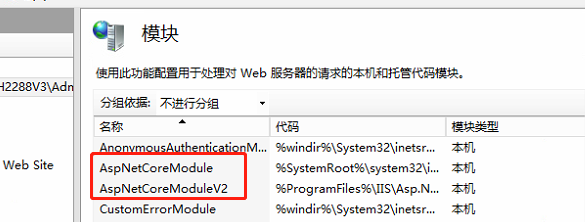
当前 .NET Core 托管捆绑包安装程序(直接下载)
安装 DotNetCore.2.x.x-WindowsHosting(貌似win2008安装不了,要2008R2以上版本才能安装), 安装DotNetCore SDK, 安装VC 2015 Redist http://www.cnblogs.com/tianma3798/p/6947287.html http://www.cnblogs.com/a-dou/p/6685582.html 覆盖发布时,要先停掉之前的站点,才能覆盖
6. NET Core 怎么取得客户端的IP地址呢?
HttpContext.Connection.RemoteIpAddress.ToString();
7. NET Core 没有Server.MapPath, 可以用IHostingEnvironment
using Microsoft.AspNetCore.Hosting;
public class HomeController : Controller { private IHostingEnvironment _host; public HomeController(IHostingEnvironment hostingEnvironment) {
_host= hostingEnvironment;
}
}
_host.ContentRootPath = 项目的根目录 _host.WebRootPath = 项目的WWWRoot目录
另外2种写法: AppDomain.CurrentDomain.BaseDirectory = {项目根目录}\bin\{Debug|Release}\netcoreapp2.0\
PlatformServices.Default.Application.ApplicationBasePath= {项目根目录}\bin\{Debug|Release}\netcoreapp2.0\
8. NLog.config 发布时要改一下里面的路径. 因为debug时,Log文件是在{project}\Log, config文件路径是{project}\bin\Debug\netcoreapp2.0, 发布时把里面内容改成./Logs
<!-- the targets to write to --> <targets> <!-- write logs to file --> <target xsi:type="File" name="allfile" fileName="../../../Logs/nlog-all-${shortdate}.log" layout="${longdate}|${event-properties:item=EventId_Id}|${uppercase:${level}}|${logger}|${message} ${exception:format=tostring}" /> <!-- another file log, only own logs. Uses some ASP.NET core renderers --> <target xsi:type="File" name="ownFile-web" fileName="../../../Logs/nlog-own-${shortdate}.log" layout="${longdate}|${event-properties:item=EventId_Id}|${uppercase:${level}}|${logger}|${message} ${exception:format=tostring}|url: ${aspnet-request-url}|action: ${aspnet-mvc-action}" /> </targets>
9. System.Web.HttpUtility.UrlEncode/UrlDecode 在NET Core的替代?
System.Net.WebUtility.HtmlEncode(myString)
10. 未找到与命令“dotnet-ef”匹配的可执行文件
这个是因为服务器端只安装的.net core SDK2.0,没有安装2.1。 要么是安装2.1的SDK;要么在报错的类库里面的xxx.csproj文件里面的ItemGroup里面加上一句 <DotNetCliToolReference Include="Microsoft.EntityFrameworkCore.Tools.DotNet" Version="2.0.1" />
11. 热更新问题. 之前.net可以直接覆盖旧的dll, 但是现在.net core 网站正在运行的时候,不能热覆盖(指windows服务器). 这个方案之前已经有了好多年了.我居然都不知道
在更新网站时,使用"App_Offline.htm“文件 怎样才能在你想更新一个网站的内容,或是对网站做一些大的变动时,不允许用户访问网站,只有在你的操作完成后,才允许用户访问呢? Asp.Net提供了一个很方便的方法,就是利用”App_Offline.htm"文件。 具体使用方法是,你新建一个名为“App_Offline.htm”的html文档,文档的内容是你在网站关闭时,想让用户看到的内容。 当你更新网站时,如果有用户请求网站,这时Asp.Net会查找有没有App_Offline.html这个文件,如果发现了这个文件,就会关闭网站的应用程序域,并返回App_Offline.html文件的内容。 当你更新完网站后,别忘了把App_Offline.htm文件改名
12. Request.InputStream改成Request.Body
13. Request.UserAgent 改成 request.Headers[HeaderNames.UserAgent].toString()
14. HttpContextBase _httpContext 要改成 IHttpContextAccessor _httpContextAccessor, 并在Startup 里注入.
15. Net Core 控制台输出中文乱码的解决方法:
{
Console.OutputEncoding = System.Text.Encoding.UTF8;//第一种方式:指定编码
//Encoding.RegisterProvider(CodePagesEncodingProvider.Instance);//第二种方式
16. entity 定义要指定decimal的精度,不然会出来warning
2018-11-22 10:34:10.8966|30000|WARN|Microsoft.EntityFrameworkCore.Model.Validation|No type was specified for the decimal column 'OrderShippingInclTax' on entity type 'Order'. This will cause values to be silently truncated if they do not fit in the default precision and scale. Explicitly specify the SQL server column type that can accommodate all the values using 'ForHasColumnType()'.
[Column(TypeName = "decimal(18, 4)")] public decimal OrderShippingInclTax { get; set; }
17. controller 的构造函数,不能使用Session, 这样写会得到null
public EstateController(CRMContext context, PermissionLogic plogic) : base(context,plogic) { SysId = (int)HttpContext.Session.GetInt32("LoginSysId"); }
18. The instance of entity type '{{实体}}' cannot be tracked because another instance with the same key value for {'主键'} is already being tracked. When attaching existing entities, ensure that only one entity instance with a given key value is attached.
这个是因为dbcontext 查询了同一条记录给了不同的变量,但是EF只能跟踪中的一条. 你要把其中的一条查询变成AsNoTrack() var db_Ach = _context.ProjectAchs.Where(t => t.Id == entity.Id).AsNoTracking().FirstOrDefault();